/********************************************************************
[File name] lcd12864.h
[Function description] lcd12864 header file
**********************************************************************/
#ifndef __LCD12864_H
#define __LCD12864_H
//****************************************************************
#include "stm32f10x_gpio.h"
#include "systick_delay.h"
//************************************************************************
//Pin definition
#define GPIO_LCD GPIOE
#define RCC_APB2Periph_GPIO_LCD RCC_APB2Periph_GPIOE
//片选
#define LCD_CS_1 GPIO_LCD->BSRR = GPIO_Pin_8
#define LCD_CS_0 GPIO_LCD->BRR = GPIO_Pin_8
//数据
#define LCD_SID_1 GPIO_LCD->BSRR = GPIO_Pin_9
#define LCD_SID_0 GPIO_LCD->BRR = GPIO_Pin_9
//同步时钟
#define LCD_CLK_1 GPIO_LCD->BSRR = GPIO_Pin_10
#define LCD_CLK_0 GPIO_LCD->BRR = GPIO_Pin_10
//复位 低电平复位
#define LCD_RET_1 GPIO_LCD->BSRR = GPIO_Pin_11
#define LCD_RET_0 GPIO_LCD->BRR = GPIO_Pin_11
#define LCD_Write_Con_Cmd (uc32)0xf8000000 // 1111 1000 0000 0000 0000 0000 0000 0000
#define LCD_Write_Dis_Data (uc32)0xfa000000 // 1111 1010 0000 0000 0000 0000 0000 0000
//********************Function declaration****************************** **********
void LCD_Port_Config(void);
void LCD_Init(void);
void LCD_Write(u32 inst, u8 ddata);
void LCD_Dis_Str(u8 row, u8 col, u8 *str);
void LCD_Dis_Digital(u8 row, u8 col,u32 Dig_Data);
void LCD_Clear(void);
void LCD_Reset(void);
void LCD_Dis_Frame(void);
void LCD_Clear_GDRAM(void);
void LCD_Clear_Graphics(u8 row, u8 col, u8 row_Pixel, u8 col_Pixel);
void LCD_Dis_Graphics(u8 row, u8 col, u8 row_Pixel, u8 col_Pixel, u8 *Dis_Data);
/****************************** *************************************
LCD module instruction set definition
************ *************************************************** *****
0x01 // Clear display command
0x06 // Set input mode
0x0c // Set display control
0x30 // Function setting (basic command)
0x34 // Function setting (extended command)
0x36 // Open drawing (extended command)
** ************************************************** *************/
#endif
/********************************************************************************
【File name】lcd12864.c
【Function description】lcd12864 driver
【Author】shifu
********************************************************************************/
/**************************************************************************/
#include "lcd12864.h"
#include "stm32f10x_lib.h"
/********************************************************************************
【Function description】I/O port function, direction setting
****************************************************************************/
void LCD_Port_Config(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
/* Enable port clock */
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIO_LCD, ENABLE);
/* Configure the pins used for push-to-play output and the port speed to 50MHz*/
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_8 | GPIO_Pin_9 | GPIO_Pin_10 | GPIO_Pin_11;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIO_LCD, &GPIO_InitStructure);
}
/****************************************************************************
【Function description】 LCD write byte function
Entry parameter inst=cmd/data: command/data flag (data: write display data cmd: write control instruction)
x: command/data byte
****************************************************************************/
void LCD_Write(u32 inst, u8 ddata)
{
u32 temp = inst;
u32 i,t;
temp |= ((u32)(ddata & (u8)0xf0) << 16) + ((u32)(ddata & (u8)0x0f) << 12);
LCD_CS_1; //Select 12864
//Pull down the CLK pin to prepare for a rising edge
LCD_CLK_0;
//Serially transmit 24 binary bits
for(i=0;i<24;i++)
{
if(temp & 0x80000000) LCD_SID_1;
else LCD_SID_0;
//Pull up the CLK pin to generate a rising edge, and the highest bit is transmitted to the LCD module
LCD_CLK_1;
t = 0x10; while(t--); //Delay LCD to read data
//Pull down the CLK pin to prepare for a rising edge
LCD_CLK_0;
//Shift left one bit to prepare the next bit to be transmitted
temp = temp<<1;
}
LCD_CS_0; //Uncheck 12864
}
/****************************************************************************
【Function description】
LCD string display function: void LCD_Dis_Str(u8 x, u8 y, u8 *str);
Input parameter row: The row where the string starts to be displayed
col: The column where the string starts to be displayed
str: The character pointer pointing to the string to be displayed, the string ends with '\0'
Note: The encoding of CGRAM and Chinese fonts can only appear at the start position of adress counter
DDRAM 8*16 bytes space
Line1 80H 81H 82H 83H 84H 85H 86H 87H
Line2 90H 91H 92H 93H 94H 95H 96H 97H
Line3 88H 89H 8AH 8BH 8CH 8DH 8EH 8FH
Line4 98H 99H 9AH 9BH 9CH 9DH 9EH 9FH
Line5 A0H A1H A2H A3H A4H A5H A6H A7H
Line6 B0H B1H B2H B3H B4H B5H B6H B7H
Line7 A8H A9H AAH ABH ACH ADH AEH AFH
Line8 B8H B9H BAH BBH BCH BDH BEH BFH
********************************************************************************/
void LCD_Dis_Str(u8 row, u8 col, u8 *str)
{
u8 addr, i = 0;
//Prevent misoperation (debugging)
// if(row > 3) row = 3;
// if(col > 7) col = 7;
//Determine the display buffer address according to the x,y coordinatesswitch
(row)
{
case 0: addr = 0x80 + col; break;
case 1: addr = 0x90 + col; break;
case 2: addr = 0x88 + col; break;
case 3: addr = 0x98 + col; break;
}
//Set the DDRAM address
LCD_Write(LCD_Write_Con_Cmd, addr);
while(*str)
{
//Next line: Write characters from the set DDRAM address and prepare a pointer to the next character
LCD_Write(LCD_Write_Dis_Data, *str++);
i++;
if(i==2)
{
i = 0;
//The DDRAM address will automatically increment every time two bytes are written to track the address changes of DDRAM so that the line can be adjusted
addr++;
//When addr=88H,90H,98H,a0H, it means that the DRAM address needs to be reassignedif
( (addr&0x07) == 0 )
{
switch(addr)
{
case 0x88: addr = 0x90; break;
case 0x98: addr = 0x88; break;
case 0x90: addr = 0x98; break;
case 0xa0: addr = 0x80; break;
}
//Set the reassigned DDRAM address
LCD_Write(LCD_Write_Con_Cmd, addr);
}
}
}
}
/****************************************************************************
【Function description】 LCD clear screen
************************************************************************/
void LCD_Clear(void)
{
LCD_Write(LCD_Write_Con_Cmd, 0x01);
ST_Delay_Ms(5);
}
/****************************************************************************
【Function description】 LCD reset
************************************************************************/
void LCD_Reset(void)
{
LCD_RET_0;
ST_Delay_Ms(50);
LCD_RET_1;
ST_Delay_Ms(100);
}
/****************************************************************************
【Function description】 Initialize LCD subroutine
**********************************************************************/
void LCD_Init(void)
{
//Port configuration
LCD_Port_Config();
//LCD reset
LCD_Reset();
//Basic instruction set 8-bit data
LCD_Write(LCD_Write_Con_Cmd,0x30);
ST_Delay_Ms(1);
//Basic instruction set 8-bit data
LCD_Write(LCD_Write_Con_Cmd,0x30);
ST_Delay_Ms(1);
//Display on, cursor off, reverse off
LCD_Write(LCD_Write_Con_Cmd,0x0C);
ST_Delay_Ms(1);
//Clear screen
LCD_Clear();
//DDRAM address counter (AC) plus 1
LCD_Write(LCD_Write_Con_Cmd,0x06);
}
/**********************************************************************************
【Function description】 Display decimal number
entry parameter row: row
col: column
Dig_Data: displayed decimal number
**************************************************************************/
void LCD_Dis_Digital(u8 row, u8 col,u32 Dig_Data)
{
u8 dd[11];
u8 i,j=9;
u32 temp;
temp = Dig_Data;
dd[10]=0;
for(i=10;(i>j)&(i>0);i--)
{
dd[i-1] = temp%10 + '0';
temp=temp/10;
if(temp) j--;
}
LCD_Dis_Str(row,col,&dd[i]);
}
/********************************************************************************
【Function description】 LCD display frame graphics mode
******************************************************************************/
void LCD_Dis_Frame(void)
{
u8 x,y;
//LCD clear drawing RAM
LCD_Clear_GDRAM();
LCD_Write(LCD_Write_Con_Cmd,0x34);
LCD_Write(LCD_Write_Con_Cmd,0x36);
for(x=0;x<9;x += 8)
{
for(y=0;y < 32;y++)
{
//left vertical
LCD_Write(LCD_Write_Con_Cmd, y+0x80);//row address
LCD_Write(LCD_Write_Con_Cmd, x+0x80);//column address
LCD_Write(LCD_Write_Dis_Data,0x80);
LCD_Write(LCD_Write_Dis_Data,0x00 );
//Right vertical
LCD_Write(LCD_Write_Con_Cmd, y+0x80);//Row address
LCD_Write(LCD_Write_Con_Cmd, x+0x87);//Column address
LCD_Write(LCD_Write_Dis_Data,0x00);
LCD_Write(LCD_Write_Dis_Data,0x01);
}
}
for(y=0;y<2;y++ )
{
for(x=0;x<8;x++)
{
LCD_Write(LCD_Write_Con_Cmd, y*31+0x80);//row address
LCD_Write(LCD_Write_Con_Cmd, x+0x80+8*y);//column address
LCD_Write(LCD_Write_Dis_Data,0xff);
LCD_Write(LCD_Write_Dis_Data,0xff);
}
}
LCD_Write(LCD_Write_Con_Cmd,0x30);
}
/********************************************************************************
【Function description】 LCD clears all drawing RAM GDRAM 64*32 bytes space
0 1 2****13 14 15
1****************
2****************
*****************
*
*
62
63
****************************************************************************/
void LCD_Clear_GDRAM(void)
{
u8 x,y;
LCD_Write(LCD_Write_Con_Cmd,0x34);
for(y=0;y<64;y++)
{
for(x=0;x<16;x++)
{
LCD_Write(LCD_Write_Con_Cmd, y+0x80);//row address
LCD_Write(LCD_Write_Con_Cmd, x+0x80);//column address
LCD_Write(LCD_Write_Dis_Data,0x00);
LCD_Write(LCD_Write_Dis_Data,0x00);
}
}
LCD_Write(LCD_Write_Con_Cmd,0x30);
}
/**********************************************************************************
【Function Description】 Display custom graphics in the specified rows and columns (defined as 64X8 pixels)
【Entry Parameters】 u8 row: row coordinate value range (0-63)
u8 col: column coordinate value range (0-7)
u8 row_Pixel: row pixel number value range (1-64)
u8 col_Pixel: Column pixel number range (1-8)
u8 *Dis_Data: display data pointer
[Note]: row coordinate plus row offset cannot exceed 63
column coordinate plus column offset cannot exceed 7
**************************************************************************/
void LCD_Dis_Graphics(u8 row, u8 col, u8 row_Pixel, u8 col_Pixel, u8 *Dis_Data)
{
u8 r,c,r_count,c_count;
//Open drawing mode
LCD_Write(LCD_Write_Con_Cmd,0x34);
LCD_Write(LCD_Write_Con_Cmd,0x36);
for(r_count = row; r_count < row + row_Pixel; r_count++)
{
if(r_count > 31) r = r_count - 32;
else r = r_count;
for(c_count = col; c_count < col + col_Pixel; c_count++)
{
if(r_count > 31) c = c_count + 8;
else c = c_count;
LCD_Write(LCD_Write_Con_Cmd, r+0x80);//Row address
LCD_Write(LCD_Write_Con_Cmd, c+0x80);//Column address
LCD_Write(LCD_Write_Dis_Data,*Dis_Data++);
LCD_Write(LCD_Write_Dis_Data,*Dis_Data++) ;
}
}
LCD_Write(LCD_Write_Con_Cmd,0x30);
}
/***************************************************************************
【Function description】 Clear the custom graphics in the specified row and column (defined as 64X8 pixels)
【Entry parameters】 u8 row: row coordinate value range (0-63)
u8 col: column coordinate value range (0-7)
u8 row_Pixel: row pixel number value range (1-64)
u8 col_Pixel: column pixel number value range (1-8)
【Notes】 : The row coordinate plus the row offset cannot exceed 63,
the column coordinate plus the column offset cannot exceed 7
******************************************************************************/
void LCD_Clear_Graphics(u8 row, u8 col, u8 row_Pixel, u8 col_Pixel)
{
u8 r,c,r_count,c_count;
//Extended instruction
LCD_Write(LCD_Write_Con_Cmd,0x34);
for(r_count = row; r_count < row + row_Pixel; r_count++)
{
if(r_count > 31) r = r_count - 32;
else r = r_count;
for(c_count = col; c_count < col + col_Pixel; c_count++)
{
if(r_count > 31) c = c_count + 8;
else c = c_count;
LCD_Write(LCD_Write_Con_Cmd, r+0x80);//行地址
LCD_Write(LCD_Write_Con_Cmd, c+0x80);//列地址
LCD_Write(LCD_Write_Dis_Data,0x00);
LCD_Write(LCD_Write_Dis_Data,0x00);
}
}
LCD_Write(LCD_Write_Con_Cmd,0x30);
}
Previous article:stm32: firmware library file description
Next article:STM32 output comparison error and solution
Recommended ReadingLatest update time:2024-11-15 17:03
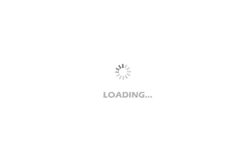
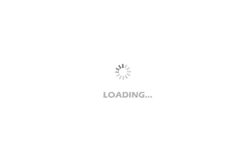
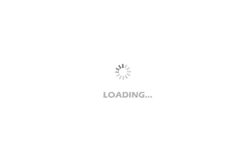
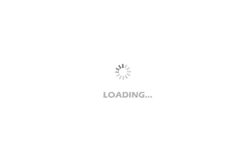
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- LED chemical incompatibility test to see which chemicals LEDs can be used with
- Application of ARM9 hardware coprocessor on WinCE embedded motherboard
- What are the key points for selecting rotor flowmeter?
- LM317 high power charger circuit
- A brief analysis of Embest's application and development of embedded medical devices
- Single-phase RC protection circuit
- stm32 PVD programmable voltage monitor
- Introduction and measurement of edge trigger and level trigger of 51 single chip microcomputer
- Improved design of Linux system software shell protection technology
- What to do if the ABB robot protection device stops
- Huawei's Strategic Department Director Gai Gang: The cumulative installed base of open source Euler operating system exceeds 10 million sets
- Download from the Internet--ARM Getting Started Notes
- Learn ARM development(22)
- Learn ARM development(21)
- Learn ARM development(20)
- Learn ARM development(19)
- Learn ARM development(14)
- Learn ARM development(15)
- Analysis of the application of several common contact parts in high-voltage connectors of new energy vehicles
- Wiring harness durability test and contact voltage drop test method
- Please tell me about the ADS1252 reading data question
- Where to connect the external ± of 485?
- The difference between FPGA and DSP (rough summary)
- gcc cannot find library
- Modification + Portable Ion Air Purifier Disassembly and Modification of Coal Stove Lighter
- [Smart Kitchen] Unboxing - STM32F750
- Is it feasible to automatically turn off the backlight under the key switch?
- 4~20mA current loop acquisition circuit
- Several common communication protocols for microcontrollers
- 使用SVN保持 封装库 同步的方法【轉載】