STM8S105S4 I2C can send data from the machine with this configuration, but it cannot receive data from the machine. What's the problem? //I2C port initialization GPIO_Init(GPIOE,GPIO_PIN_1|GPIO_PIN_2, GPIO_MODE_OUT_OD_HIZ_SLOW); void I2C_init(void) { I2C_CCRH = 0; //Standard I2C interface I2C_CCRL = 80 ; I2C_FREQR = 16; I2C_OARL = (I2CAddr<<1); I2C_OARH = 0x40 ; I2C_ITR = 0x06; I2C_CR1 = 0x01; I2C_CR2 |= 0x04; } @far @interrupt void I2C_Handler (void) { u8 state1,state2,state3; state1 = I2C_SR1; state2 = I2C_SR2; state3 = I2C_SR3; //Address matchif ((state1&0x02)!=0){I2C_CR2 |= 0x04;} //Data received, need to be processedif ( (state1& 0x40) != 0) { I2C_CR2 |= 0x04; temp1 = I2C_DR; SetOutput(temp1); } //Slave needs to send dataif ((state1 & 0x80) != 0) { I2C_DR = num++; } if((state2 & 0x04) != 0)I2C_SR2 &= ~0x04; //Response failed, clear this bitif ((state1 & 0x10) != 0) { I2C_CR2 = 0x02; } } I wrote it like this, and found that there is no problem with the slave sending data, but the slave receiving data fails and no interrupt is entered. |
After successfully transmitting the correct data once, I can't enter. The program is still running normally
#define I2CAddr 0x01
in the host
//The host writes data, and the slave receives it. This does not work.
Soft_I2C_Start();
Soft_I2C_Write(0x02);
Soft_I2C_Write(0xAA);
Soft_I2C_Stop();
//The host receives data. Without running the previous program, the host can receive data. Running the previous program, the data received by the host is 255.
Soft_I2C_Start();
Soft_I2C_Write(0x03);
var0=Soft_I2C_Read(1);
Soft_I2C_Stop();
This soft I2C has no problem controlling other devices. The chip used by the host is STM32. There should be no problem.
Solve the problem:
That's it. After you succeeded for the first time, if you turned off the response, how can you still receive it? You should be more careful when configuring the register in the future.
The problem is here I2C_CR2 = 0x02; Modify to I2C_CR2 |= 0x02; The reason is that after the modification, there is no reply after the first success |
1. PC0, PC1 initialization, GPIO_Init(GPIOC, GPIO_Pin_1 | GPIO_Pin_0, GPIO_Mode_Out_OD_HiZ_Fast);
2. Hardware must be connected
3. The bus cannot be busy, it must be idle
The initialization code is as follows:
GPIO_Init(GPIOC, GPIO_Pin_0, GPIO_Mode_Out_OD_HiZ_Slow);
GPIO_Init(GPIOC, GPIO_Pin_1, GPIO_Mode_Out_OD_HiZ_Slow);
CLK_PeripheralClockConfig(CLK_Peripheral_I2C1, ENABLE);
I2C_Init(I2C1, 50000, 0xA5, I2C_Mode_I2C, I2C_DutyCycle_2,
I2C_Ack_Enable, I2C_AcknowledgedAddress_7bit);
//Note: I2C is already enabled in I2C_Init(), so I2C_Cmd(I2C1, ENABLE);
while(I2C_GetFlagStatus(I2C1, I2C_FLAG_BUSY));
The built-in I2C of stm8 was finally debugged successfully.
1. This debugging of I2C has benefited me a lot. First of all, it proves that the official program for writing EEPROM is correct.
2. The reason why the debugging of TW8816 failed at the beginning was that I mistakenly thought that the register address was 16 bits (the chip company's business also said so, and I believed it), which made me see different data, but I think that there is data, and the program runs through using the WHILE waiting method, which means that it is connected to the slave.
3. Do not configure the GPIO of I2C. I saw some colleagues configure the GPIO.
4. In order for everyone to use the I2C of STM8 well, I baked the program.
void IIC_Init(void)
{
UCHAR temp;
CLK_PCKENR1 |= 0x01;
I2C_FREQR |= 0x10; //Input peripheral clock frequency is 1MHz
I2C_CR1 = 0x00; //Disable I2C peripherals
I2C_CCRH &= ~0xcf;
I2C_CCRL &= ~0xff;
I2C_TRISER = 0x11;
I2C_CCRL = 0x10;
I2C_CCRH = 0x00;
I2C_CR1 |= 0x01; //Enable I2C peripherals
I2C_CR2 |= 0x04; //Current received byte returns response
I2C_CR2 &= 0x08;
I2C_OARL = 0x86; //Own address
I2C_OARH = 0x40;
}
void Read_8816(UCHAR *pBuffer, UCHAR index, UCHAR NumByteToRead)
{
UCHAR temp;
while(I2C_SR3 & 0x02); //Wait for bus to be free
I2C_CR2 |= 0x01; //Generate start bit
while(!(I2C_SR1 & 0x01)); //Wait for START to be sent
I2C_DR = 0x8a; //Send 8816 device address
while(!(I2C_SR1 & 0x02)); //Wait for special 7-bit device address to be sent
temp = I2C_SR1;
temp = I2C_SR3;
I2C_DR = (UCHAR)(index);
while(!(I2C_SR1 & 0x84));
I2C_CR2 |= 0x01; //Generate repeated start bit
while(!(I2C_SR1 & 0x01)); //Wait for START to be sent
I2C_DR = 0x8b; //Read
while(!(I2C_SR1 & 0x02)); //Wait for the 7-bit device address to be sent
temp = I2C_SR1;
temp = I2C_SR3;
while(NumByteToRead) //How many bytes to read
{
if(NumByteToRead == 1)
{
I2C_CR2 &= ~0x04; //Do not return a response
I2C_CR2 |= 0x02; //Generate a stop bit
}
if(I2C_SR1 & 0x40)
{
temp = I2C_SR1;
Buff[8-NumByteToRead]=I2C_DR;
*pBuffer = Buff[8-NumByteToRead];
pBuffer++;
NumByteToRead--;
}
}
I2C_CR2 |= 0x04;
I2C_CR2 &= ~0x08; //Enable response for the next receive
}
Previous article:STM8S interrupt priority setting
Next article:STM8S-Discovery third program - DS18B20
Recommended ReadingLatest update time:2024-11-16 13:30
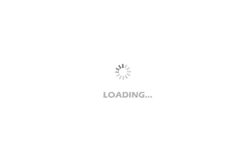
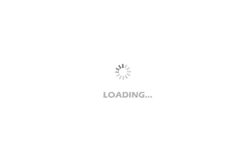
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Hard disk control and encryption based on FPGA
- Redeem mooncakes with your core points during the Mid-Autumn Festival! Exchange your core points for core points and you will get endless surprises!
- I don't understand the GPIO operation statements of the HAL library. Please help me
- High-definition smart camera based on TMS320DM8127
- Causes of color difference between different batches of LCDs
- Is there no one in the forum?
- What exactly are S parameters?
- Can the current direction of a MOS tube be reversed? How much current can a body diode carry?
- EEWORLD University ---- TI Battery Management In-depth Analysis Series
- Introduction to FPGA CLB LUT to implement logic functions