The SysTick timer is bundled in the NVIC and is used to generate the SysTick exception (exception number: 15). In the past, the operating system and all systems that used a time base had to have a hardware timer to generate the required "tick" interrupt as the time base for the entire system. Tick interrupts are particularly important for operating systems. For example, the operating system can grant different numbers of time slices to multiple tasks to ensure that no task can monopolize the system; or grant a certain time range of each timer cycle to a specific task, etc., and the various timing functions provided by the operating system are all related to this tick timer. Therefore, a timer is needed to generate periodic interrupts, and it is best to prevent user programs from arbitrarily accessing its registers to maintain the rhythm of the operating system's "heartbeat".
The
Cortex-M3 processor contains a simple timer. Because all CM3 chips have this timer, software migration between different CM3 devices is simplified. The clock source of this timer can be an internal clock (FCLK, the free-running clock on the CM3) or an external clock (the
STCLK signal on the CM3 processor). However, the specific source of STCLK is determined by the chip designer, so the clock frequency between different products may vary greatly. Therefore, it is necessary to check the device manual of the chip to decide what to choose as the clock source.
The SysTick timer can generate an interrupt, and CM3 has a special exception type for it, and it has a place in the vector table. It makes the porting of operating systems and other system software between CM3 devices much simpler, because the SysTick processing method is the same in all CM3 products.
There are 4 registers controlling the SysTick timer as shown in the following table.
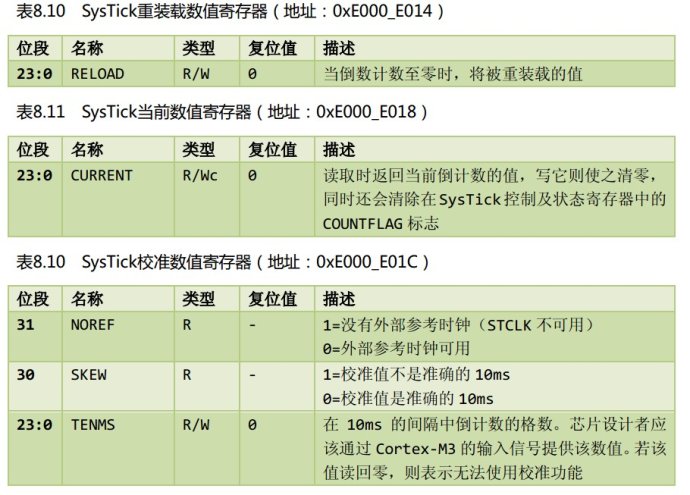
In addition to serving the operating system, the SysTick timer can also be used for other purposes: as an alarm, for measuring time, etc. It should be noted that when the processor is halted during debugging, the SysTick timer will also be suspended.
In the 3.5 firmware library, SysTick is defined in core_cm3.h:
static __INLINE uint32_t SysTick_Config(uint32_t ticks)
{
if (ticks > SysTick_LOAD_RELOAD_Msk) return (1);
SysTick->LOAD = (ticks & SysTick_LOAD_RELOAD_Msk) - 1;
NVIC_SetPriority (SysTick_IRQn, (1<<__NVIC_PRIO_BITS) - 1);
SysTick->VAL = 0;
SysTick->CTRL = SysTick_CTRL_CLKSOURCE_Msk |
SysTick_CTRL_TICKINT_Msk |
SysTick_CTRL_ENABLE_Msk;
return (0);
}
In the 3.5 library, it is very simple to use SysTick. Just call the SysTick_Config() function to write the reload beat number during initialization. For example, set the SysTick timer to generate an interrupt:
void Delay(u32 nTime)
{
TimingDelay = nTime;
while(TimingDelay); //Wait for Sys Tick to generate an interrupt
}
int main(void)
{
GPIO_conf();
while(1)
{
while(SysTick_Config(3600000)!=0); //72MHz frequency, reload register to one twentieth
LED_on();
Delay(10); //Delay 0.5s //Interrupt 10 times
LED_off();
Delay(10); //Delay 0.5s
}
}
Among them, TimingDelay is a global variable, which is decremented in the SysTick interrupt service routine:
void SysTick_Handler()
{
TimingDelay--;
}
The program compilation result is displayed as a 1Hz flashing light.
Keywords:STM32 Sys Tick Timer
Reference address:
STM32 Learning Road (IV) - Sys Tick Timer
Recommended ReadingLatest update time:2024-11-16 17:43
STM32 beginner's LED button interrupt
First, we select the specific pins that need to be controlled. Then configure specific functions for it. Finally, you can control the on and off of the LED by pressing the button. The initialization of LEDs and buttons is similar, both are configured through the GPIO_InitTypeDef structure. typedef struct { u
[Microcontroller]
NSS pin signal of stm32 spi
The NSS pin is also known as the chip select signal. As the master device, the NSS pin is high, and the slave device NSS pin is low. When the NSS pin is low, the SPI device is selected and can communicate with the master device. In STM32, the NSS signal pin of each SPI controller has two functions, namely input and ou
[Microcontroller]
STM32 interrupt nesting controller
Priority concept in STM32 (Cortex-M3) There are two priority concepts in STM32 (Cortex-M3): preemptive priority and response priority. The response priority is also called "sub-priority" or "sub-priority". Each interrupt source needs to be assigned these two priorities. 1. What is pre-emption priority? Interrupt
[Microcontroller]
STM32 printf redirection
Just one step:
In the Target page of Options, check Use MicroLIB.
In order to redirect the printf() function, we need to rewrite the fputc() C standard library function.
Because printf() is actually a macro in the C standard library function, it ultimately calls the fputc() function.
of.
int
[Microcontroller]
STM32 PWM output experiment
First, some necessary declarations #include stm32f10x.h
#include "pwm.h"u32 Sys_Clk=1000000;u16 pwm1_2_Freqz;//Frequency of pwm wave 1, 2 output port u16 pwm3_4_Freqz;//Frequency of pwm wave 3, 4 output port u16 TIM2_PERIOD;//Number of timer jump cycles u16 TIM4_PERIOD;u16 CCR_VAL1;//Value of the timer comparison reg
[Microcontroller]
Using STM32 external interrupt to drive four-way digital touch sensor module
First, let me introduce the external interrupts of STM32. Of course, this is not my summary. This is a learning document that I found among so many blogs and I think it is a good summary. Let's learn it first! In STM32, each GPIO can trigger an external interrupt, but the GPIO interrupt is a unit of group bit, and o
[Microcontroller]
STM32 study notes: FSMC details
FSMC (Flexible Static Memory Controller) is a new memory expansion technology used by the STM32 series. It has unique advantages in external memory expansion and can easily expand different types of large-capacity static memories according to system application needs . After using the FSMC controller, the FSMC_A provi
[Microcontroller]
Differences between eight IO port modes of STM32
Differences between eight IO port modes of STM32 (1) GPIO_Mode_AIN analog input (2) GPIO_Mode_IN_FLOATING floating input (3) GPIO_Mode_IPD pull-down input (4) GPIO_Mode_IPU pull-up input (5) GPIO_Mode_Out_OD open drain output (6) GPIO_Mode_Out_PP push-pull output (7) GPIO_Mode_AF_OD multiplexed open-drain output (8) G
[Microcontroller]