Included Files:
(1)Main
+ Experimental platform: ST official three-in-one kit
+ Hardware: STM32F103C8T6
+ Development platform: IAR For ARM 5.40
+ Emulator: J-Link
+ Date: 2010-10-26
+ Frequency: HSE = 8MHz, main frequency = 72MHz
++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++*/
#include "includes.h"
/*******************************************************************************
== Main function==
***************************************************************************/
int main(void)
{
RCC_Configuration(); //Configure system clock
NVIC_Configuration(); //Configure NVIC and Vector Table
SysTick_Config(); //Configure SysTick's precise delay
GPIO_Configuration();
LED1_HIGH ; LED2_HIGH ; LED3_HIGH ; LED4_HIGH ; //Initialize and turn off all lights
//Main loop
while (1)
{
LED1_LOW ;
Delay_Ms(50);
LED1_HIGH ;
Delay_Ms(50);
}
}
(2)Init_External_Device.c
/*******************************************************************************
== Global variables ==
**************************************************************************/
vu32 TimingDelay; // Precise delay counting variable used in SysTick interrupt
/*******************************************************************************
* Function Name : RCC_Configuration
* Description : Configures the different system clocks.
* Input : None
* Output : None
* Return : None
*******************************************************************************/
void RCC_Configuration(void)
{
ErrorStatus HSEStartUpStatus;
// Reset the peripheral RCC registers to the default values
RCC_DeInit();
// Set external high-speed crystal oscillator (HSE)
RCC_HSEConfig(RCC_HSE_ON);
// Wait for HSE to start
HSEStartUpStatus = RCC_WaitForHSEStartUp();
if(HSEStartUpStatus == SUCCESS)
{
//Enable prefetch buffer
FLASH_PrefetchBufferCmd(FLASH_PrefetchBuffer_Enable);
//Set code delay value
//FLASH_Latency_2 2 delay period
FLASH_SetLatency(FLASH_Latency_2);
//Set AHB clock (HCLK)
//RCC_SYSCLK_Div1 AHB clock = system clock
RCC_HCLKConfig(RCC_SYSCLK_Div1);
//Set high speed AHB clock (PCLK2)
//RCC_HCLK_Div2 APB1 clock = HCLK / 2
RCC_PCLK2Config(RCC_HCLK_Div2);
//Set low speed AHB clock (PCLK1)
//RCC_HCLK_Div2 APB1 clock = HCLK / 2
RCC_PCLK1Config(RCC_HCLK_Div2);
//PLLCLK = 8MHz * 9 = 72 MHz
//Set PLL clock source and frequency multiplierRCC_PLLConfig
(RCC_PLLSource_HSE_Div1, RCC_PLLMul_9);
//Enable or disable PLL
RCC_PLLCmd(ENABLE);
//Wait for the specified RCC flag to be set successfullyWait for PLL initialization to be successfulwhile
(RCC_GetFlagStatus(RCC_FLAG_PLLRDY) == RESET)
{
}
//Set system clock (SYSCLK) Set PLL as system clock sourceRCC_SYSCLKConfig
(RCC_SYSCLKSource_PLLCLK);
//Wait for PLL to be successfully used as the clock source for system clock
// 0x00: HSI as system clock
// 0x04: HSE as system clock
// 0x08: PLL as system clockwhile
(RCC_GetSYSCLKSource() != 0x08)
{
}
}
//RCC_APB2PeriphClockCmd(RCC_APB2Periph_ALL, ENABLE);
//Enable or disable APB2 peripheral clock
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA | RCC_APB2Periph_AFIO, ENABLE);
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB, ENABLE);
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOC, ENABLE);
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOD, ENABLE);
}
/*******************************************************************************
* Function Name : SysTick_Config SysTick设置
* Description : Configures SysTick
* Input : None
* Output : None
* Return : None
*******************************************************************************/
void SysTick_Config(void)
{
/* Disable SysTick Counter */
SysTick_CounterCmd(SysTick_Counter_Disable);
/* Disable the SysTick Interrupt */
SysTick_ITConfig(DISABLE);
/* Configure HCLK clock as SysTick clock source */
SysTick_CLKSourceConfig(SysTick_CLKSource_HCLK_Div8);
/* SysTick interrupt each 1000 Hz with HCLK equal to 72MHz */
SysTick_SetReload(9000);
/* Enable the SysTick Interrupt */
SysTick_ITConfig(ENABLE);
}
/*******************************************************************************
* Function Name : NVIC_Configuration
* Description : Configures NVIC and Vector Table base location.
* Input : None
* Output : None
* Return : None
*******************************************************************************/
void NVIC_Configuration(void)
{
#ifdef VECT_TAB_RAM
/* Set the Vector Table base location at 0x20000000 */
NVIC_SetVectorTable(NVIC_VectTab_RAM, 0x0);
#else /* VECT_TAB_FLASH */
/* Set the Vector Table base location at 0x08000000 */
NVIC_SetVectorTable(NVIC_VectTab_FLASH, 0x0);
#endif
}
/*******************************************************************************
* Function Name : GPIO_Configuration
* Description : Configures the different GPIO ports.
* Input : None
* Output : None
* Return : None
*******************************************************************************/
void GPIO_Configuration(void)
{
GPIO_InitTypeDef GPIO_InitStructure_LED_PORTB;
GPIO_InitTypeDef GPIO_InitStructure_KEY_PORTA;
GPIO_InitTypeDef GPIO_InitStructure_KEY_PORTB;
GPIO_InitTypeDef GPIO_InitStructure_KEY_PORTC;
//==== LED =======================================================
GPIO_InitStructure_LED_PORTB.GPIO_Pin = GPIO_Pin_12 | GPIO_Pin_13 | GPIO_Pin_14 | GPIO_Pin_15 ;
GPIO_InitStructure_LED_PORTB.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure_LED_PORTB.GPIO_Mode = GPIO_Mode_Out_PP; //推挽输出
GPIO_Init(GPIOB, &GPIO_InitStructure_LED_PORTB);
//==== KEY =======================================================
GPIO_InitStructure_KEY_PORTA.GPIO_Pin = GPIO_Pin_0 ;
GPIO_InitStructure_KEY_PORTA.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure_KEY_PORTA.GPIO_Mode = GPIO_Mode_IPU ; //上拉输入
GPIO_Init(GPIOA, &GPIO_InitStructure_KEY_PORTA);
GPIO_InitStructure_KEY_PORTB.GPIO_Pin = GPIO_Pin_7 ;
GPIO_InitStructure_KEY_PORTB.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure_KEY_PORTB.GPIO_Mode = GPIO_Mode_IPU; //上拉输入
GPIO_Init(GPIOB, &GPIO_InitStructure_KEY_PORTB);
GPIO_InitStructure_KEY_PORTC.GPIO_Pin = GPIO_Pin_13 | GPIO_Pin_14 | GPIO_Pin_15 ;
GPIO_InitStructure_KEY_PORTC.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure_KEY_PORTC.GPIO_Mode = GPIO_Mode_IPU; //上拉输入
GPIO_Init(GPIOC, &GPIO_InitStructure_KEY_PORTC);
}
/*******************************************************************************
* Function Name : 精确延时函数
*******************************************************************************/
void Delay_Ms(u32 nTime)
{
/* Enable the SysTick Counter */
SysTick_CounterCmd(SysTick_Counter_Enable);
TimingDelay = nTime;
while(TimingDelay != 0);
/* Disable SysTick Counter */
SysTick_CounterCmd(SysTick_Counter_Disable);
/* Clear SysTick Counter */
SysTick_CounterCmd(SysTick_Counter_Clear);
}
(3)includes.h
#define INCLUDES 1
//================================================================================
// ★★☆☆★★ Include files ★★☆☆★★
//================================================================================
#include "stm32f10x_lib.h"
#include "stm32f10x_type.h"
#include "stm32f10x_it.h"
//=====================================================================================
// ★★☆☆★★ Global variables ★★☆☆★★
//=
... The counting variable used for precise delay in SysTick interrupt
//====================================================================================
// ★★☆☆★★ Calling function ★★☆☆★★
//=
... Clock part#####################################################################
void RCC_Configuration(void); //Configure system clock
//###### System pulse part#####################################################################
void SysTick_Config(void); //Configure system pulse timer
//###### Interrupt part####################################################################
void NVIC_Configuration(void); //Configure NVIC and Vector Table
//###### I/O part###################################################################
void GPIO_Configuration(void); //Configure the GPIO port to be used
//###### Other common functions########################################################################
void Delay_Ms(u32 nTime); //Precise millisecond delay
//=================================================================================
// ★★☆☆★★ IO port definition ★★☆☆★★
//===============================================================================
#define LED1_HIGH ( GPIO_SetBits(GPIOB, GPIO_Pin_12) )
#define LED2_HIGH ( GPIO_SetBits(GPIOB, GPIO_Pin_13) )
#define LED3_HIGH ( GPIO_SetBits(GPIOB, GPIO_Pin_14) )
#define LED4_HIGH ( GPIO_SetBits(GPIOB, GPIO_Pin_15) )
#define LED1_LOW ( GPIO_ResetBits(GPIOB, GPIO_Pin_12) ) #define LED2_LOW
( GPIO_ResetBits(GPIOB , GPIO_Pin_13) )
#define LED3_LOW ( GPIO_ResetBits(GPIOB, GPIO_Pin_14) )
#define LED4_LOW ( GPIO_ResetBits(GPIOB, GPIO_Pin_15) )
#define KEY_UP ( GPIO_ReadInputDataBit(GPIOC, GPIO_Pin_14) )
#define KEY_DOWN (GPIO_ReadInputDataBit(GPIOA, GPIO_Pin_0) )
#define KEY_LEFT (GPIO_ReadInputDataBit(GPIOC, GPIO_Pin_15) )
#define KEY_RIGHT ( GPIO_ReadInputDataBit(GPIOC, GPIO_Pin_13) )
#define KEY_SELECT ( GPIO_ReadInputDataBit(GPIOB, GPIO_Pin_7) )
#endif
(4)stm32f10x_it.c
#include "includes.h"
//#include "stm32f10x_it.h"
// ... ...
/*******************************************************************************
* Function Name : SysTickHandler
* Description : This function handles SysTick Handler.
* Input : None
* Output : None
* Return : None
*******************************************************************************/
//Line :136
void SysTickHandler(void)
{
if (TimingDelay != 0x00)
{
TimingDelay--;
}
}
// ... ...
Previous article:STM32 UART1(1)
Next article:STM32 KEY
Recommended ReadingLatest update time:2024-11-25 13:30
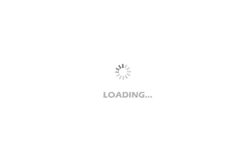
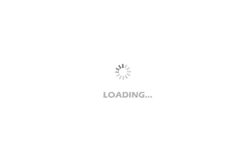
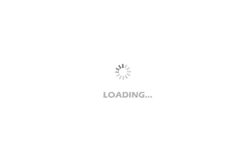
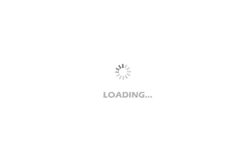
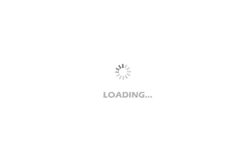
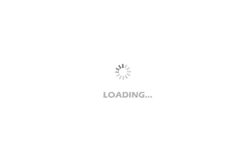
- Naxin Micro and Xinxian jointly launched the NS800RT series of real-time control MCUs
- How to learn embedded systems based on ARM platform
- Summary of jffs2_scan_eraseblock issues
- Application of SPCOMM Control in Serial Communication of Delphi7.0
- Using TComm component to realize serial communication in Delphi environment
- Bar chart code for embedded development practices
- Embedded Development Learning (10)
- Embedded Development Learning (8)
- Embedded Development Learning (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Intel promotes AI with multi-dimensional efforts in technology, application, and ecology
- ChinaJoy Qualcomm Snapdragon Theme Pavilion takes you to experience the new changes in digital entertainment in the 5G era
- Infineon's latest generation IGBT technology platform enables precise control of speed and position
- Two test methods for LED lighting life
- Don't Let Lightning Induced Surges Scare You
- Application of brushless motor controller ML4425/4426
- Easy identification of LED power supply quality
- World's first integrated photovoltaic solar system completed in Israel
- Sliding window mean filter for avr microcontroller AD conversion
- What does call mean in the detailed explanation of ABB robot programming instructions?
- Automechanika Shanghai 2024 to feature a record number of concurrent events, bringing together industry wisdom and planning for future development
- Corporate Culture Sharing: How to cultivate scarce silicon IP professionals? SmartDV's journey of personal growth and teamwork
- Corporate Culture Sharing: How to cultivate scarce silicon IP professionals? SmartDV's journey of personal growth and teamwork
- NXP releases first ultra-wideband wireless battery management system solution
- NXP releases first ultra-wideband wireless battery management system solution
- Beijing Jiaotong University undergraduates explore Tektronix's advanced semiconductor open laboratory and experience the charm of cutting-edge high technology
- Beijing Jiaotong University undergraduates explore Tektronix's advanced semiconductor open laboratory and experience the charm of cutting-edge high technology
- New CEO: Dr. Torsten Derr will take over as CEO of SCHOTT AG on January 1, 2025
- How Edge AI Can Improve Everyday Experiences
- How Edge AI Can Improve Everyday Experiences
- How many PWM waves can the MSP430F149 microcontroller output?
- Keysight N5235A PNA-L Microwave Network Analyzer
- Benefit: Get the classic books on power supplies now!
- CircuitPython 7.3.0 released
- R8-5 Attribute Introduction
- [2022 Digi-Key Innovation Design Competition] Build a blockchain browser service
- POWER PCB software usage training
- Is the maximum power of this 24V voltage regulator only 5W?
- Cheer for the college entrance examination
- Introduction and use of execve function