At this point, you should have a certain understanding of the operation process of ucos. For the process of semaphore transmission, you can refer to another post. Here we only focus on the event identification group itself.
First, applying event identification groups requires four steps:
- Declare a pointer variable to identify the group.
void *SemGrp_Task_LED1;
This stores the pointer to the event control block, and is a global variable that needs to be globally declared (extern) in the corresponding header file. When the identification group is initialized, the event control block is initialized. The event control block is the module for managing and allocating this resource.
-
Initialize the event identification group,
SemGrp_Task_LED1 = OSFlagCreate(0,&err);
Parameter 0 is the result of the final logic operation, the initial value is 0, and parameter &err is the error message;
-
Set the waiting function in the user task,
OSFlagPend( SemGrp_Task_LED1, // Flag group pointer
0x03, // Flag is the last two digits
OS_FLAG_WAIT_SET_ALL + OS_FLAG_CONSUME, // Set and operate and clear the flag after the operation
0,&err // No wait timeout
);There are 5 parameters in total. The first parameter sets the identification group pointer, indicating which identification group is being waited for; the second parameter is which operation bits are in the identification group. 0x03 means that there are 2 semaphores participating in this group and they are the lowest two bits of a byte; the third parameter is the combination of these semaphores to form a valid message, which is the two operations of "AND" and "OR", that is, all single semaphores are triggered when they are 1 or as long as one semaphore sends 1, and the corresponding operation relationship is to clear the single semaphore after the operation; the fourth parameter is the waiting time, the unit is the number of system heartbeats, and 0 means waiting without time limit; the fifth parameter is the error type.
-
Set the sending semaphore function in different user tasks or trigger tasks.
OSFlagPost(SemGrp_Task_LED1,0x01,OS_FLAG_SET,&err); // Set the first bit of the group flag
There are four parameters in total. The first parameter is the identification group pointer, which indicates which identification group you are sending to; the second parameter indicates the number of bits you are operating on. The specific valid bit is set in the waiting function. In the sending function, it indicates which bit you are sending in the waiting function. In the code example, it is the lowest bit sent; the third parameter is whether to set it to one or zero, OS_FLAG_SET is set to one, and OS_FLAG_CLR is cleared to zero; the fourth parameter is the error type.
================================================== ===================================
Here is the actual code inside the system:
Step 2 and Step 3
{
(void) p_arg ;
SemGrp_Task_LED1 = OSFlagCreate(0,&err);
while(1)
{
OSFlagPend( SemGrp_Task_LED1, // Flag group pointer
0x03, // Flag is the last two digits
OS_FLAG_WAIT_SET_ALL + OS_FLAG_CONSUME, // Set and operate and clear the flag after the operation
0,&err // No wait timeout
);
LED1_HIGH;
OSTimeDlyHMSM(0,0,1,0);
LED1_LOW;
OSTimeDlyHMSM(0,0,1,0); // Delay to allow other tasks to run
}
}
Two step 4 functions, respectively in the external interrupt service function:
void Interrupt_Handle_KEY2(void)
{
OSIntEnter();
// If you call the ucos system function in the interrupt service function, you must add the interrupt system function when you enter and exit the interrupt system function.
OSFlagPost(SemGrp_Task_LED1,0x01,OS_FLAG_SET,&err); // Set the first bit of the group flag EXTI_ClearITPendingBit
(EXTI_Line4); // Clear the flag bit
OSIntExit();
}
void Interrupt_Handle_KEY3(void)
{
OSIntEnter();
// If you call the ucos system function in the interrupt service function, you must add the interrupt system function when you enter and exit the interrupt system function.
OSFlagPost(SemGrp_Task_LED1,0x02,OS_FLAG_SET,&err); // Set the second bit of the group flag
EXTI_ClearITPendingBit(EXTI_Line3); // Clear the flag bit
OSIntExit();
}
Summarize:
For the operation of event identification group, the idea is very simple. Add a waiting function in a task of the final result. When the system runs here, it will suspend this task, and then wait for each single semaphore according to the logic. There is a sending function in other tasks. According to the operation bit set in the waiting function, each bit is set high or low, so as to achieve the purpose of synchronizing multiple tasks with one task.
Previous article:STM32 uCOS_II practice message mailbox
Next article:About the global reference of STM32 Systick delay function variables
Recommended ReadingLatest update time:2024-11-16 21:28
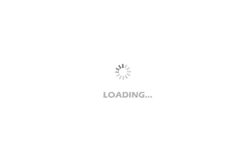
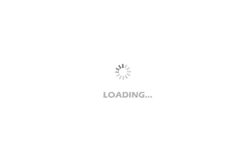
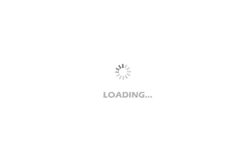
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- [Silicon Labs BG22-EK4108A Bluetooth Development Evaluation] 3. Lighting Experiment
- CC1310 Two-wire Serial Bootloader Solution
- Huawei mobile phone case fill light
- Filter applications for different scenarios - harmonics
- 4.3-inch screen design
- Random functions: usage of rand and srand
- How does AD19 transfer a BMP format logo image to PCB?
- [Image recognition classification & motion detection & analog signal processing system based on Raspberry Pi 400, Part 3] Using QT program...
- How to see, TI official application manual - problems in the boost principle
- ZigBee serial communication experimental code