FSMC includes 4 modules: (1) AHB interface (including FSMC configuration register) (2) NOR flash memory and PSRAM controller (when driving LCD, LCD is like a PSRAM with only 2 16-bit storage spaces, one is DATA RAM and the other is CMD RAM) (3) NAND flash memory and PC card controller (4) External device interface Each module is controlled by chip select signal --------------------------------------------------------------------------------------------------- Hardware circuit (non-multiplexed connection is given here) can be connected like this: address line A0 connects to RS NE connects to CS NEW – WR NOE – RD After FSMC is connected to the peripherals, it is equivalent to shielding the user from the specific operation of the external device. As long as the corresponding type of mapped address space is written with data, these data + addresses will be automatically translated and written to the peripheral storage device. For writing LCD, there are some common writing methods in the STM official library: #define LCD_BASE ((u32)(0x60000000 | 0x0C000000)) //Let me explain, the address is the fourth block of BANK1 #define LCD ((LCD_TypeDef *) LCD_BASE) void LCD_WriteReg(u8 LCD_Reg,u16 LCD_RegValue) { LCD->LCD_REG = LCD_Reg; LCD->LCD_RAM = LCD_RegValue; } u16 LCD_ReadReg(u8 LCD_Reg) { LCD->LCD_REG = LCD_Reg; return (LCD->LCD_RAM); } Of course, you can also do it yourself, it's entirely up to you. -------------------------------------------------------------------------------------------------- Initialization problem: 1. Bit width: mainly AHB to NOR/PSRAM bit width, for example, if AHB is set to 32 bits and NOR is 16 bits, it will be transmitted twice. 2. Set address. The manual says: In NOR/PSRAM mode, HADDR[27:26] (HADDR are internal AHB address lines that are translated to external memory) are used to chip select the four partitions of NOR/PSRAM. A[25:0] is the address line, because HADDR is a byte address but access is word addressing, so according to the different bit widths, there are the following situations. (1) When the storage data is set to 8 bits, each address bit corresponds to FSMC_A[25:0], and the data bit corresponds to FSMC_D[7:0] (storage size 64MB * 8 = 512MB) (2) When the storage data is set to 16 bits, each address bit corresponds to FSMC_A[25:1]>>1 (i.e. FSMC_A[24:0]), and the data bit corresponds to FSMC_D[15:0] (storage size (64MB / 2) * 16 = 512MB) Note: Under the 16-bit external storage width, FSMC will use A[25:1] to generate A[24:0]. Regardless of 8 or 16 bits, FSMC_A[0] must be connected to A[0] of the external storage. -------------------------------------------------------------------------------------------------- -------------------------------------------------------------------------------------------------- The advantage of FSMC is that once you set it up, the control lines WR, RD, DB0-DB15 and data lines are all automatically controlled by FSMC. For example, when you write in the program: (volatile unsigned short int*)(0x60000000)=val; then the FSMC will automatically perform a write operation, and the corresponding WE and RD pins of the main control chip will show the write timing (ie WE=0, RD=1), and the value of the data val will also be automatically presented through DB0-15 (ie FSMC-D0:FSMC-D15=val). The address 0x60000000 will be presented on the data line (ie A0-A25=0, the correspondence of the address line is the most troublesome, it depends on the specific situation, take a good look at the FSMC manual). After the connection is completed, the read and write timing will be automatically completed by the FSMC. But there is still a very critical problem, that is, RS is not connected, and CS is not connected. Because in the FSMC, there is no corresponding RS and CS pins. What should I do? At this time, there is a good way, which is to use a certain address line to connect RS. For example, if we choose A16 as the address line, then when we want to write to the register, we need RS, that is, A16 is set high. How to do it in software? That is, change the address that FSMC wants to write to 0x60020000, as follows: (volatile unsigned short int*)(0x60020000)=val; At this time, A16 will be pulled high while executing other FSMCs, because A0-A18 will present the address 0x60020000. Bit17=1 in 0x60020000 will cause A16 to be 1. [page] When reading data, the address is changed from 0x60020000 to 0x60000000, and A16 is 0 at this time. --------------------------------------------------------------------------------------------------
Next is the FSCM timing issue, which everyone has discussed:
1. When FSMC selects NOR and PSRAM modules:
it is divided into two types: 1. Asynchronous transactions 2. Synchronous burst transactions
Asynchronous transactions are divided into normal mode (mode 1, mode 2) and 4 extended modes of mode 1 and mode 2 (mode A, B, C, D);
2. General timing rules:
All controller output signals change on the rising edge of the internal clock (HCLK) 2. In synchronous read
and write mode, the output data changes on the falling edge of the
memory clock (FSMC_CLK). 3. Sampling rules The FSMC always samples the data before de-asserting the chip select signal NE. This guarantees that the memory data-hold timing constraint is met (chip enable high to data transition, usually 0 ns min.) (FSMC always samples data before canceling the chip select signal NE, which ensures that the storage data retention time constraint can be met.) IV: Timing calculation The set values are all based on Hclk as the basic unit Formula: What is not fully understood now is that because it is an asynchronous mode, the data is notified to the other end by delaying one HCLK, so how does the signal on the address line notify the other end that it is valid? -------------------------------------------------------------------------------------------------- The following are some data from the information to estimate whether the time roughly meets the application requirements: -------------------------------------------------------------------------------------------------- FAQ for some common problems:
1.Does STM32F103 FSMC exist in all models?
ANS: VC, VD, VE, ZC, ZD, ZE are the only models available.
-------------------------------------------------- -------------------------------------------------- --------------------------
2. Multiplexing or non-multiplexing?
The FSMC of STM32 supports two modes: multiplexing or non-multiplexing of data and address lines.
Non-multiplexing mode: 16-bit data lines and 26-bit address lines are used separately. This mode is recommended for STM32 products with 144 pins or above.
Multiplexing mode: lower 16-bit data/address line multiplexing. In this mode, it is recommended to use an address latch to distinguish between data and address.
If latch is not used: when NADV is low, address signal Ax appears on ADx (x=0…15), and when NADV becomes high, data signal Dx appears on ADx.
If latch is used: both Ax and Dx can be obtained on ADx at the same time.
Unused data or address lines in FSMC can be configured as GPIO
For a 16-bit width external memory, FSMC will use HADDR[25:1] internally to generate the address FSMC_A[24:0] of the external memory. Therefore, the actual access address is the address after right shifting by one bit.
-------------------------------------------------- -------------------------------------------------- --------------------------
3. Timing diagram (extended mode)
I think the main purpose of the difference between normal mode and extended mode is to set the read and write timings to different ones. The so-called modes A, B, C, and D are actually not much different.
Quoting the manual:
The differences with mode1 are the toggling of NADV and the independent read and write timings when extended mode is set.
-------------------------------------------------- -------------------------------------------------- --------------------------
4. FSMC_NWAIT and FSMC_NE1/FSMC_NCE2. I don't understand how to use these two?
ANS: NBL0, NBL1, the IO is specified in the STM32F103 data sheet and cannot be changed casually. (Used when using PSRAM)
NWAIT should be used for FLASH operation. NE1 and NCE2 are some chip select signals. The FSMC of STM32 supports hanging multiple devices at the same time, and each device must have a CS (that is, NEx, NCEx).
NADV (NL) is the stored signal in multiplexing mode. It controls the PSRAM output to be valid in non-multiplexing mode.
After reading English documents and forums for a day, I summarized some random information. Since I am new to embedded development and my foundation is too weak, I will save these for future use. The
experimental board will arrive next Monday. I estimate that I will spend some time to adjust the FSMC and add the DMA function, hoping to improve the product.
PS: I have been struggling for almost a week. Finally, I have done it
![[Reprint] STM32 FSMC study notes [Reprint] STM32 FSMC study notes](https://www.eeworld.com.cn/uploadfile/2015/0909/20150909031601541.gif)
![[Reprint] STM32 FSMC study notes [Reprint] STM32 FSMC study notes](https://www.eeworld.com.cn/uploadfile/2015/0909/20150909031601541.gif)
Previous article:In-depth study of STM32 FSMC bus
Next article:STM32 bit band operation
Recommended ReadingLatest update time:2024-11-16 18:02
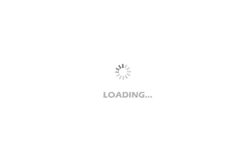
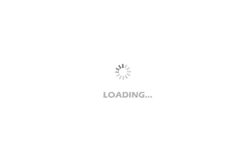
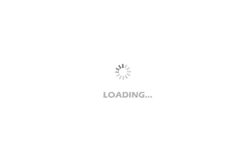
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Need a stereo audio amplifier circuit to replace TI's LM4666
- [Modelsim FAQ] Error loading design
- Principle of oscillometric blood pressure signal detection (a common method of measuring blood pressure)
- LDO problems found in AD2S1210 application
- German electrician VS Indian electrician, one has obsessive-compulsive disorder, the other drives the obsessive-compulsive disorder to death
- Newbies to power supplies, look here! Everything you want to know is here, and you will definitely gain something after reading this
- When you want to improve performance and reduce emissions, Allegro wants you to have the best of both worlds!
- Help, how to fix the low output of the boost circuit?
- A company's power supply interview questions, let's see how many you know? ?
- Single-phase electrical appliance analysis and monitoring device (2017 Electric Competition)