I have just learned the basic character display. Just character display requires a large number of various instruction formats. Let's write a program at this stage and summarize it:
Program function: Display characters in the center of the LCD: "Hello World", "By Fate"
Note: Use of LCD 1602: http://gaebolg.blog.163.com/blog/static/198269068201231665524137/
Attached is the source code: (I am a rookie, so there are inevitably some poorly written parts. It is only for backup purposes. Welcome to give me some advice, and please go away if you hate me...)
/*------------------------------------------------------------------------------------
LCD 1602 displays characters, "Hello World", "By Fate" are displayed in the center
-------------------------------------------------------------------------------------*/
#include
#include
sbit RS = P2^4;
sbit RW = P2^5;
sbit EN = P2^6;
#define DataPort P0
void Init_LCD(void); // Initialize LCD display
void LCD_Write_Com(unsigned char c); // write command to LCD
void LCD_Write_Data(unsigned char d); // write data to LCD
bit LCD_Busy(void); // LCD busy detection function, busy returns 1, idle returns 0
void LCD_Clear(void); // Clear screen
/*-----------------------------------------------------------------------------------------------------
LCD parameter setting: DL 0 = Data bus is 4 bits 1 = Data bus is 8 bits
N 0 = Display 1 line 1 = Display 2 lines
F 0 = 5x7 dots/each character 1 = 5x10 dots/each character
--------------------------------------------------------------------------------------*/
void LCD_Set_Argument(unsigned char DL, unsigned char N, unsigned charF);
/*---------------------------------------------------------------------------------------------------------------------------------------------------
LCD input mode setting: ID 0 = Cursor moves left after writing new data 1 = Cursor moves right after writing new data
0 = Display does not move after writing new data 1 = Display moves right by 1 word after writing new data
------------------------------------------------------------------------------------------------------------------------------------*/
void LCD_Set_InputMode(unsigned char ID, unsigned char S);
/*---------------------------------------------------------------------------------------
LCD display settings: D 0 = Display function off 1 = Display function on
C 0 = No cursor 1 = With cursor
B 0 = Cursor does not blink 1 = Cursor blinks
--------------------------------------------------------------------------*/
void LCD_Set_DisplayMode(unsigned char D, unsigned char C, unsigned char B);
/*-----------------------------------------------------------
Display character c at row, column col
------------------------------------------------------------*/
void LCD_Write_Char(unsigned char row, unsigned char col, unsigned charc);
/*-----------------------------------------------------------
Starting from row, column col, display string s
------------------------------------------------------------*/
void LCD_Write_String(unsigned char row, unsigned char col, unsigned char *s);
void Delay(unsigned int t);
void Delay_ms(unsigned int t); // 延迟 t ms
void main (void)
{
Init_LCD();
LCD_Write_Char(1, 3, 'H');
LCD_Write_Char(1, 4, 'e');
LCD_Write_Char(1, 5, 'l');
LCD_Write_Char(1, 6, 'l');
LCD_Write_Char(1, 7, 'o');
LCD_Write_String(1, 9, "World!");
LCD_Write_String(2, 5, "By Fate!");
while (1);
}
void Init_LCD(void)
{
LCD_Set_Argument(1, 1, 0); // Set basic parameters: 8-bit data bus, 2-line display, 5x7 dot matrix/character
LCD_Set_DisplayMode(0, 0, 0); // Set display mode: turn off display, turn off cursor display, turn off cursor blinking
LCD_Set_InputMode(1, 0); // Set input mode: for each character input, the cursor moves right and the screen does not move
LCD_Clear(); // Clear the screen
LCD_Set_DisplayMode(1, 0, 0); // Turn on display
}
void LCD_Write_Com(unsigned char c)
{
while (LCD_Busy());
RS = 0;
RW = 0;
EN = 1;
DataPort = c;
_nop_(); // Small delay, much smaller than Delay(), purpose: to make the data on the bus stable
EN = 0; // Write operation is driven by EN falling edge
}
void LCD_Write_Data(unsigned char d)
{
while (LCD_Busy());
RS = 1;
RW = 0;
EN = 1;
DataPort = d;
_nop_();
EN = 0;
}
bit LCD_Busy(void)
{
DataPort = 0xFF;
RS = 0;
RW = 1;
EN = 0;
_nop_();
EN = 1; // Read operation is EN = 1 drive
return (bit)(DataPort & 0x80); // When forced to convert to bit, unless the original data is equal to 0, bit = 0, otherwise bit = 1
}
void LCD_Clear(void)
{
LCD_Write_Com(0x01);
Delay_ms(5);
}
void LCD_Set_Argument(unsigned char DL, unsigned char N, unsigned charF)
{
DL <<= 4; // The instruction format determines the shift, see the instruction format requirements
N <<= 3;
F <<= 2;
LCD_Write_Com(0x20 | DL | N | F);
Delay_ms(5);
}
void LCD_Set_InputMode(unsigned char ID, unsigned char S)
{
ID <<= 1;
LCD_Write_Com(0x04 | ID | S);
Delay_ms(5);
}
void LCD_Set_DisplayMode(unsigned char D, unsigned char C, unsigned char B)
{
D <<= 2;
C <<= 1;
LCD_Write_Com(0x08 | D | C | B);
Delay_ms(5);
}
void LCD_Write_Char(unsigned char row, unsigned char col, unsigned charc)
{
if (row == 1) LCD_Write_Com(0x80 + col - 0x01); // From the instruction format, we can see that DB7 = 1, so add 80H
else if (row == 2) LCD_Write_Com(0xC0 + col - 0x01);
LCD_Write_Data(c);
}
void LCD_Write_String(unsigned char row, unsigned char col, unsigned char *s)
{
if (row == 1) LCD_Write_Com(0x80 + col - 0x01);
else if (row == 2) LCD_Write_Com(0xC0 + col - 0x01);
while (*s) {
LCD_Write_Data(*s);
s++;
}
}
void Delay(unsigned int t)
{
while (t--);
}
void Delay_ms(unsigned int t) // According to the test, t ms can be represented fairly closely
{
while (t--) {
Delay(245);
Delay(245);
}
}
Previous article:Tip 2 -- Summary of MCU C51 & A51 Programming Key Points
Next article:【C51】Source code 4 -- Speaker plays the theme song "You" of "Higurashi When They Cry"
Recommended ReadingLatest update time:2024-11-16 20:56
![[C51 self-study notes] Serial communication + RS-232C interface + RS-422A/RS-485 interface](https://6.eewimg.cn/news/statics/images/loading.gif)
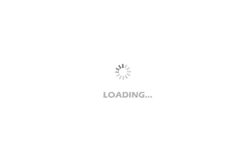
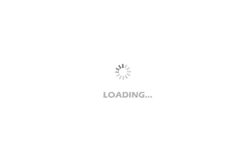
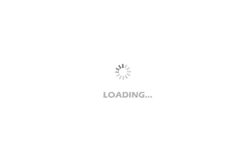
- Popular Resources
- Popular amplifiers
-
西门子S7-12001500 PLC SCL语言编程从入门到精通 (北岛李工)
-
Siemens Motion Control Technology and Engineering Applications (Tongxue, edited by Wu Xiaojun)
-
How to read electrical control circuit diagrams (Classic best-selling books on electronics and electrical engineering) (Zheng Fengyi)
-
MCU C language programming and Proteus simulation technology (Xu Aijun)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- FPGA Implementation of ECT Image Reconstruction Algorithm
- Designing Current Loops Using the MSP430 MCU Smart Analog Combo
- The difference between HISPI and MIPI protocols.
- Can the analog watchdog of stm32f103ve be used for power-off detection?
- 【CH579M-R1】+ Construction and use of development environment
- [National Technology N32G457 Review] 4. Transplanting the small embedded task manager MillisTaskManager
- About the "edge" of the pulse
- Design of CAN-USB Converter Based on ARM7
- MicroPython enhances WB55 functionality
- [Jianan K510 Review 1] One-stop compilation and burning experience