First, let's look at the DIR register. Its offset address is 0x8000, and its attributes are readable and writable. It is responsible for the direction of the GPIO pin, that is, whether the pin is used as input or output, which is determined by the setting of this register. Although the DIR register is also a 32-bit structure, since each group of LPC1114 has only 12 pins, only the lower 12 bits are used. When the bit is 0, it is used as input, and when it is 1, it is used as output. The 12 bits correspond to the 12 pins one by one, and each bit setting corresponds to a pin. In the default state, the value of DIR is all 0, so in the default state, all pins are in input state. To change the pin state, you can do it by writing the corresponding bit of the DIR register. For example, to set the 1st, 3rd, and 5th pins of port 0 as inputs and the others as outputs, execute the statement "LPC_GPIO0->DIR = 0xFD5;".
Next, let's look at the IS register. Its offset address is 0x8004, and its attributes are readable and writable. It is responsible for the triggering mode of the external interrupt of the GPIO pin, that is, whether the pin responds to the interrupt as level-triggered or edge-triggered, which is determined by the setting of this register. The IS register also uses only the lower 12 bits of the 32 bits. When the bit is 0, edge triggering is selected, and when it is 1, level triggering is selected. The 12 bits correspond to 12 pins one by one, and each bit setting corresponds to one pin. In the default state, the value of IS is all 0, so in the default state, all pins are edge-triggered when external interrupts occur. To change the triggering mode of the pin interrupt, you can do so by writing the corresponding bit of the IS register. For example, to set the 11th pin of port 1 to level triggering, execute the statement "LPC_GPIO1->IS |= 0x800;". In addition, when selecting the triggering mode, please note that if level triggering is selected, you must consider whether multiple external interrupt responses will be triggered during the period when the trigger level is maintained. Generally, there will be no such problem if edge triggering is selected.
Next, let's look at the IEV register, whose offset address is 0x800C and whose attributes are readable and writable. It is responsible for cooperating with the IS register above to determine the specific trigger mode. That is, if IS is configured as edge trigger mode, IEV is responsible for selecting whether it is an upward jump edge or a downward jump edge; if IS is configured as a level trigger mode, IVE is responsible for selecting whether it is a high level trigger or a low level trigger. The IVE register also only uses the lower 12 bits of the 32 bits. When the bit is 0, it selects the downward jump edge trigger or the low level trigger mode, and when it is 1, it selects the upward jump edge trigger or the high level trigger mode. The 12 bits correspond to the 12 pins one by one, and each bit setting corresponds to a pin. In the default state, the value of IEV is all 0, so in the default state, all pins are in the downward jump edge trigger or the low level trigger mode when the external interrupt occurs. To change the specific trigger mode of the pin interrupt, it can be achieved by writing the corresponding bit of the IEV register. For example, to set the 5th pin of port 2 to high level trigger, execute two sentences "LPC_GPIO1->IS |= 0x020;LPC_GPIO1->IVE |= 0x020;". Another point to emphasize is that the IEV register must be used in conjunction with the IS register. Generally speaking, IS should be configured first, and then IVE.
Next is the IBE register, whose offset address is 0x8008 and whose attributes are readable and writable. It is responsible for selecting whether to use the dual-edge trigger mode when the GPIO is interrupted externally. In some special applications (such as when it is necessary to capture the rising and falling edges of the pulse at the same time), it is necessary to frequently switch the trigger mode in the program. In LPC1114, due to the IBE register, the operation is much simpler. Just enable the dual-edge trigger mode of the corresponding pin by setting the IBE register. The IBE register also only uses the lower 12 bits of the 32 bits. When the bit is 0, the dual-edge trigger is turned off. The trigger mode at this time is determined by the IEV register. When it is 1, the dual-edge trigger is turned on. The 12 bits correspond to the 12 pins one by one, and each bit setting corresponds to one pin. In the default state, the value of IBE is all 0, so in the default state, the dual-edge trigger mode of all pins is turned off when the external interrupt occurs. To enable the dual-edge trigger mode, it can be achieved by writing the corresponding bit of the IBE register. For example, to set the first pin of port 3 to dual-edge trigger mode, execute two statements: "LPC_GPIO2->IS &= ~0x002;LPC_GPIO2->IBE |=0x002;". Another point needs to be emphasized. If a pin is set to dual-edge trigger by IBE, the corresponding IEV setting will not work. In addition, the IBE register must also be used in conjunction with the IS register. Generally speaking, IS should be configured first, and then IBE.
The next one is the IE register, whose offset address is 0x8010 and whose attributes are readable and writable. It is responsible for enabling the external interrupt function of the GPIO pin. This register also uses only the lower 12 bits of the 32 bits. When the bit is 0, the interrupt function is masked (i.e., the interrupt is not enabled), and when it is 1, the interrupt function is not masked (i.e., the interrupt is enabled). The 12 bits correspond to the 12 pins one by one, and each bit setting corresponds to a pin. In the default state, the value of IE is all 0, so in the default state, all pins do not use external interrupts (all interrupts are masked). To enable the external interrupt function of a pin, it can be achieved by writing the corresponding bit of the IE register. For example, to enable the interrupt function of the first pin of port 0, execute the statement "LPC_GPIO0->IE = 0x002;".
The next one is the RIS register, whose offset address is 0x8014 and whose attribute is read-only. It is responsible for querying which GPIO pin has an interrupt request. This register also uses only the lower 12 bits of the 32 bits. When the bit is 0, it indicates that there is no external interrupt request on the corresponding pin. When it is 1, it indicates that there is an external interrupt request on the corresponding pin. The 12 bits correspond to the 12 pins one by one, and each bit setting corresponds to one pin. The value of RIS is all 0 at reset, indicating that no external interrupt request occurs when all pins are reset. If the value corresponding to RIS on a pin is 1, it indicates that a qualified interrupt request occurs on the pin. At this time, if the corresponding IE bit is also 1 (that is, the interrupt function of the pin is turned on), an interrupt response will occur on the pin. However, if the corresponding IE bit is not 1, the interrupt will not be responded.
Next is the MIS register, whose offset address is 0x8018 and whose attribute is read-only. It is basically the same as the RIS register above and can be used to query whether there is an interrupt response. The difference is that for RIS, as long as the interrupt trigger condition is met on the pin, its corresponding bit is set to 1, but it is not necessarily responded to because it may be masked (for example, the corresponding IE bit is not set to 1). Therefore, RIS cannot determine whether the interrupt is responded to, but can only determine whether an interrupt request that meets the conditions occurs; but in the MIS register, if the corresponding bit is set to 1, it means that the interrupt must be responded to, so it is possible to query the MIS register by program to determine whether an interrupt is responded to and which pin is responded to. In fact, the MIS register represents the interrupt status after masking, and it also uses only the lower 12 bits of the 32 bits. When the bit is 0, it indicates that there is no external interrupt response on the corresponding pin, and when it is 1, it indicates that there is an external interrupt response on the corresponding pin. 12 bits correspond to 12 pins one by one, and each bit setting corresponds to one pin.
The last one is the IC register, with an offset address of 0x801C and a write-only attribute. It is used to clear the interrupt edge detection logic. It also uses only the lower 12 bits of the 32 bits. When the bit is 0, it does not work, that is, it has no effect on the external interrupt. When it is 1, it indicates that the edge trigger port bit on the corresponding pin is cleared. The 12 bits correspond to the 12 pins one by one, and each bit setting corresponds to a pin. The reason for setting the IC register is that after the interrupt response (edge-type trigger only), the corresponding bits in the RIS and MIS registers will not be automatically cleared. Therefore, after the interrupt response, it is necessary to write 1 in the corresponding bit of the IC register to clear it to ensure the normal triggering of subsequent interrupts.
The above is a basic introduction to the functions of each register in GPIO. As for the detailed usage, it will be discussed in detail in the interrupt chapter later, so I will not go into details here.
Next, let's discuss the configuration of the GPIO port pins. As we know from the first chapter, many LPC1114 pins are multiplexed. Except for pins 3 in port 0, pins 2 to 10 in port 2, and pins 4 and 5 in port 3, the remaining pins have secondary functions. Even the reset terminal RESET is multiplexed on the GPIO0_0 pin. In order to switch between the multiplexed functions on the pins, a pin configuration register IOCON is specially set up inside the processor. Users can use IOCON to configure the following functional characteristics of each I/O:
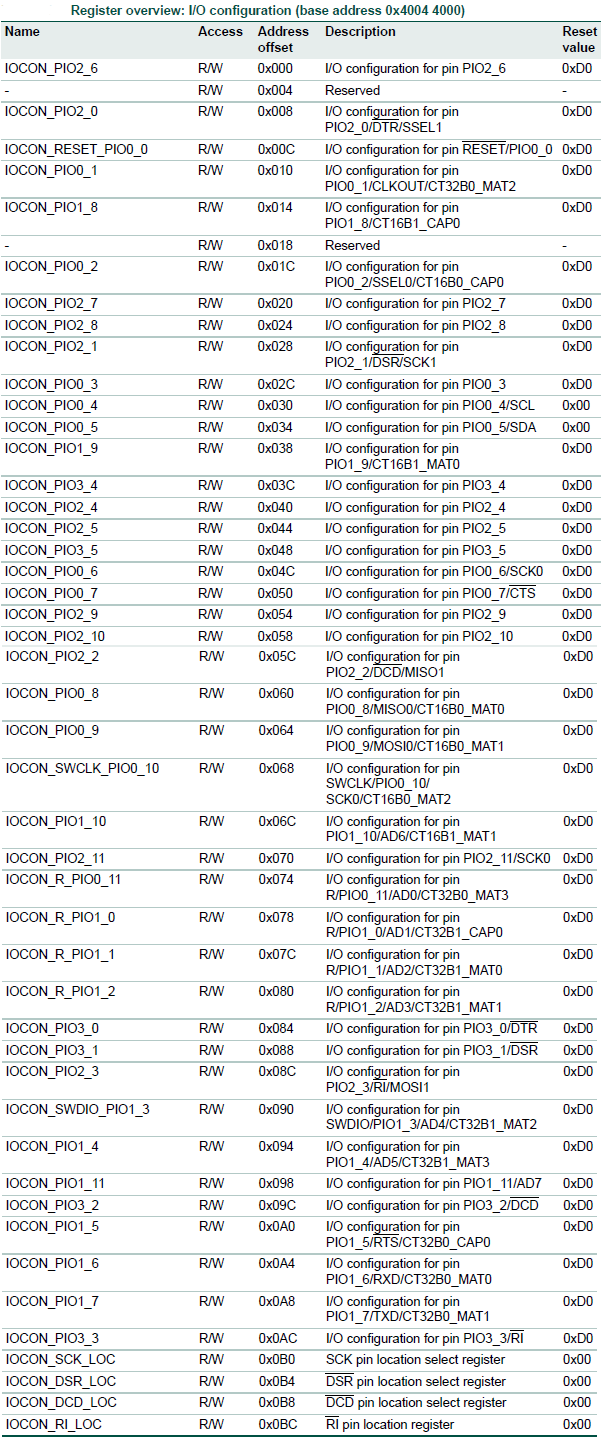
{
__IO uint32_t PIO2_6; /*!< Offset: 0x000 (R/W) I/O configuration for pin PIO2_6 */
uint32_t RESERVED0[1];
__IO uint32_t PIO2_0; /*!< Offset: 0x008 (R/W) I/O configuration for pin PIO2_0/DTR/SSEL1 */
__IO uint32_t RESET_PIO0_0; /*!< Offset: 0x00C (R/W) I/O configuration for pin RESET/PIO0_0 */
__IO uint32_t PIO0_1; /*!< Offset: 0x010 (R/W) I/O configuration for pin PIO0_1/CLKOUT/CT32B0_MAT2 */
__IO uint32_t PIO1_8; /*!< Offset: 0x014 (R/W) I/O configuration for pin PIO1_8/CT16B1_CAP0 */
uint32_t RESERVED1[1];
__IO uint32_t PIO0_2; /*!< Offset: 0x01C (R/W) I/O configuration for pin PIO0_2/SSEL0/CT16B0_CAP0 */
__IO uint32_t PIO2_7; /*!< Offset: 0x020 (R/W) I/O configuration for pin PIO2_7 */
__IO uint32_t PIO2_8; /*!< Offset: 0x024 (R/W) I/O configuration for pin PIO2_8 */
__IO uint32_t PIO2_1; /*!< Offset: 0x028 (R/W) I/O configuration for pin PIO2_1/nDSR/SCK1 */
__IO uint32_t PIO0_3; /*!< Offset: 0x02C (R/W) I/O configuration for pin PIO0_3 */
__IO uint32_t PIO0_4; /*!< Offset: 0x030 (R/W) I/O configuration for pin PIO0_4/SCL */
__IO uint32_t PIO0_5; /*!< Offset: 0x034 (R/W) I/O configuration for pin PIO0_5/SDA */
__IO uint32_t PIO1_9; /*!< Offset: 0x038 (R/W) I/O configuration for pin PIO1_9/ CT16B1_MAT0 */
__IO uint32_t PIO3_4; /*!< Offset: 0x03C (R/W) I/O configuration for pin PIO3_4 */
__IO uint32_t PIO2_4; /*!< Offset: 0x040 (R/W) I/O configuration for pin PIO2_4 */
__IO uint32_t PIO2_5; /*!< Offset: 0x044 (R/W) I/O configuration for pin PIO2_5 */
__IO uint32_t PIO3_5; /*!< Offset: 0x048 (R/W) I/O configuration for pin PIO3_5 */
__IO uint32_t PIO0_6; /*!< Offset: 0x04C (R/W) I/O configuration for pin PIO0_6/SCK0 */
__IO uint32_t PIO0_7; /*!< Offset: 0x050 (R/W) I/O configuration for pin PIO0_7/nCTS */
__IO uint32_t PIO2_9; /*!< Offset: 0x054 (R/W) I/O configuration for pin PIO2_9 */
__IO uint32_t PIO2_10; /*!< Offset: 0x058 (R/W) I/O configuration for pin PIO2_10 */
__IO uint32_t PIO2_2; /*!< Offset: 0x05C (R/W) I/O configuration for pin PIO2_2/DCD/MISO1 */
__IO uint32_t PIO0_8; /*!< Offset: 0x060 (R/W) I/O configuration for pin PIO0_8/MISO0/CT16B0_MAT0 */
__IO uint32_t PIO0_9; /*!< Offset: 0x064 (R/W) I/O configuration for pin PIO0_9/MOSI0/CT16B0_MAT1 */
__IO uint32_t SWCLK_PIO0_10; /*!< Offset: 0x068 (R/W) I/O configuration for pin SWCLK/PIO0_10/SCK0/CT16B0_MAT2 */
__IO uint32_t PIO1_10; /*!< Offset: 0x06C (R/W) I/O configuration for pin PIO1_10/AD6/CT16B1_MAT1 */
__IO uint32_t PIO2_11; /*!< Offset: 0x070 (R/W) I/O configuration for pin PIO2_11/SCK0 */
__IO uint32_t R_PIO0_11; /*!< Offset: 0x074 (R/W) I/O configuration for pin TDI/PIO0_11/AD0/CT32B0_MAT3 */
__IO uint32_t R_PIO1_0; /*!< Offset: 0x078 (R/W) I/O configuration for pin TMS/PIO1_0/AD1/CT32B1_CAP0 */
__IO uint32_t R_PIO1_1; /*!< Offset: 0x07C (R/W) I/O configuration for pin TDO/PIO1_1/AD2/CT32B1_MAT0 */
__IO uint32_t R_PIO1_2; /*!< Offset: 0x080 (R/W) I/O configuration for pin nTRST/PIO1_2/AD3/CT32B1_MAT1 */
__IO uint32_t PIO3_0; /*!< Offset: 0x084 (R/W) I/O configuration for pin PIO3_0/nDTR */
__IO uint32_t PIO3_1; /*!< Offset: 0x088 (R/W) I/O configuration for pin PIO3_1/nDSR */
__IO uint32_t PIO2_3; /*!< Offset: 0x08C (R/W ) I/O configuration for pin PIO2_3/RI/MOSI1 */
__IO uint32_t SWDIO_PIO1_3; /*!< Offset: 0x090 (R/W) I/O configuration for pin SWDIO/PIO1_3/AD4/CT32B1_MAT2 */
__IO uint32_t PIO1_4; /*!< Offset: 0x094 (R/W) I/O configuration for pin PIO1_4/AD5/CT32B1_MAT3 */
__IO uint32_t PIO1_11; /*!< Offset: 0x098 (R/W) I/O configuration for pin PIO1_11/AD7 */
__IO uint32_t PIO3_2; /*!< Offset: 0x09C (R/W) I/O configuration for pin PIO3_2 /nDCD */
__IO uint32_t PIO1_5; /*!< Offset: 0x0A0 (R/W) I/O configuration for pin PIO1_5/nRTS/CT32B0_CAP0 */
__IO uint32_t PIO1_6; /*!< Offset: 0x0A4 (R/W) I/O configuration for pin PIO1_6/RXD/CT32B0_MAT0 */
__IO uint32_t PIO1_7; /*!< Offset: 0x0A8 (R/W) I/O configuration for pin PIO1_7/TXD/CT32B0_MAT1 */
__IO uint32_t PIO3_3; /*!< Offset: 0x0AC (R /W) I/O configuration for pin PIO3_3/nRI */
__IO uint32_t SCK_LOC; /*!< Offset: 0x0B0 (R/W) SCK pin location select Register */
__IO uint32_t DSR_LOC; /*!< Offset: 0x0B4 (R/W) DSR pin location select Register */
__IO uint32_t DCD_LOC; /*!< Offset: 0x0B8 ( R/W) DCD pin location select Register */
__IO uint32_t RI_LOC; /*!< Offset: 0x0BC (R/W) RI pin location Register */
} LPC_IOCON_TypeDef;
The above structure is defined in the header file LPC11xx.h.
Each IOCON register is a 32-bit structure, but it only uses the lower 11 bits at most, because the number of bits occupied by the settings varies according to the functions of the pins. For the convenience of discussion, the following table gives a general bit field definition description of an IOCON register. When actually using different settings of the pins, please consult the data sheet yourself.
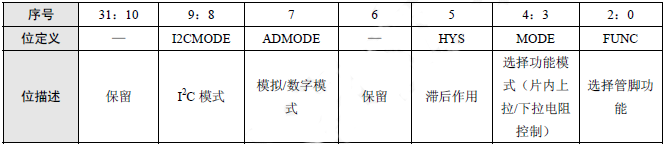
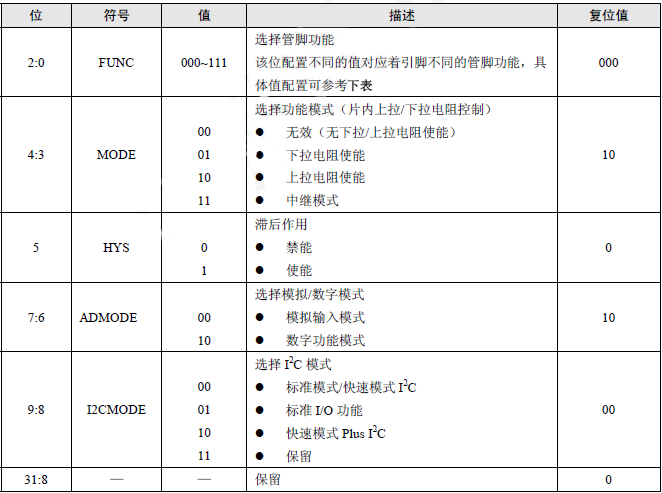
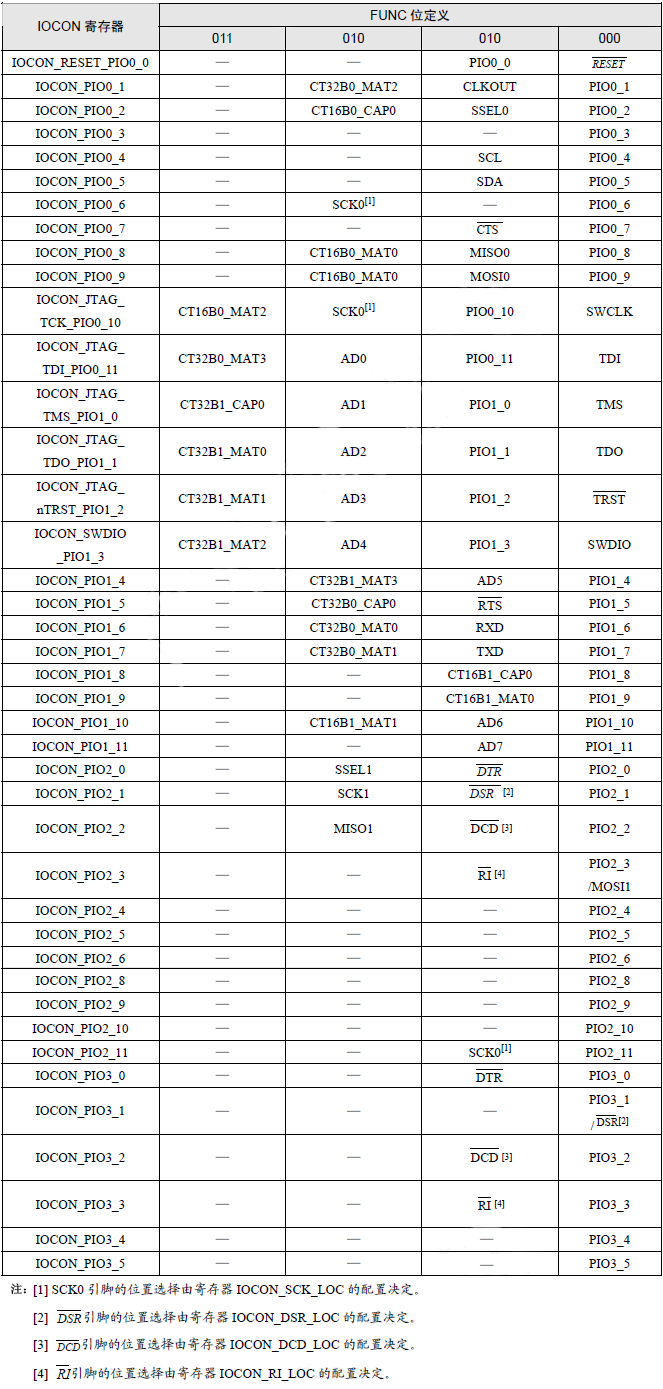
Here are some notes on the pin configuration:
1. If the pin is configured as GPIO, the DIR register can be used to set its direction (i.e. input/output); if it is configured as a non-GPIO function, the DIR setting is invalid.
2. If the pin is configured as GPIO, it defaults to pull-up mode, but whether it is pull-up or pull-down, it is weak pull type (40k resistor on chip). If you want strong pull, you must connect an external resistor.
3. The hysteresis effect is the input buffer, which can only be used when the external pin voltage is 2.5~3.6V and must be disabled when the voltage is lower than 2.5V.
4. When the A/D conversion mode is selected, the internal digital receiving part is disconnected to ensure the accuracy of the input voltage. If only high and low levels are received, it must be set to digital mode, otherwise the level cannot be read.
5. Only some pins have A/D mode and I2C mode (see FUNC table). Other pins are invalid when configured in this mode. If the pin is configured in A/D mode, the hysteresis and pull-up/pull-down configuration are invalid.
6. When the IOCON configured for all pins is reset, the value is 0xD0, which means that the pins are in multiplexing function group 0, pull-up resistor mode, disabled hysteresis characteristics, and digital mode by default.
7. When using program configuration, please note that the 6th bit (reserved bit) of IOCON must be written as 1, because its reset value is 1.
8. The other four special configuration registers (SCK_LOC, DSR_LOC, DCD_LOC, RI_LOC) are mainly used to locate the pins of a certain activation function. Please refer to the manual for specific usage.
9. Before configuring IOCON, the IOCON clock (located at the 16th bit in the AHBCLKCTRL register) must be turned on, otherwise the configuration cannot be performed. After the configuration is completed, turn off the clock to save power.
Finally, let’s look at a few examples:
1. It is required to configure pin 0 of port 0 as a GPIO port, with the direction as output, with pull-up, and the output value as 0.
Execution: LPC_SYSCON->SYSAHBCLKCTRL |= (1<<16);
LPC_IOCON->RESET_PIO0_0 = 0xD1;
LPC_SYSCON->SYSAHBCLKCTRL &= ~(1<<16);
LPC_GPIO0->DIR |= 0x001;
LPC_GPIO0->MASKED_ACCESS[1 ] = 0x000;
2. It is required to configure pin 1 of port 1 as A/D input port.
Execution: LPC_SYSCON->SYSAHBCLKCTRL |= (1<<16);
LPC_IOCON->R_PIO1_1 = 0x42;
LPC_SYSCON->SYSAHBCLKCTRL &= ~(1<<16);
3. It is required to configure the third pin of port 1 to CT32B1_MAT2 mode.
Execution: LPC_SYSCON->SYSAHBCLKCTRL |= (1<<16);
LPC_IOCON->SWDIO_PIO1_3 = 0xD3;
LPC_SYSCON->SYSAHBCLKCTRL &= ~(1<<16);
Previous article:LPC1114 external interrupt
Next article:LPC1114 General Purpose Input/Output Ports (GPIO)
- Popular Resources
- Popular amplifiers
- Learn ARM development(16)
- Learn ARM development(17)
- Learn ARM development(18)
- Embedded system debugging simulation tool
- A small question that has been bothering me recently has finally been solved~~
- Learn ARM development (1)
- Learn ARM development (2)
- Learn ARM development (4)
- Learn ARM development (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- LED chemical incompatibility test to see which chemicals LEDs can be used with
- Application of ARM9 hardware coprocessor on WinCE embedded motherboard
- What are the key points for selecting rotor flowmeter?
- LM317 high power charger circuit
- A brief analysis of Embest's application and development of embedded medical devices
- Single-phase RC protection circuit
- stm32 PVD programmable voltage monitor
- Introduction and measurement of edge trigger and level trigger of 51 single chip microcomputer
- Improved design of Linux system software shell protection technology
- What to do if the ABB robot protection device stops
- Analysis of the application of several common contact parts in high-voltage connectors of new energy vehicles
- Wiring harness durability test and contact voltage drop test method
- From probes to power supplies, Tektronix is leading the way in comprehensive innovation in power electronics testing
- From probes to power supplies, Tektronix is leading the way in comprehensive innovation in power electronics testing
- Sn-doped CuO nanostructure-based ethanol gas sensor for real-time drunk driving detection in vehicles
- Design considerations for automotive battery wiring harness
- Do you know all the various motors commonly used in automotive electronics?
- What are the functions of the Internet of Vehicles? What are the uses and benefits of the Internet of Vehicles?
- Power Inverter - A critical safety system for electric vehicles
- Analysis of the information security mechanism of AUTOSAR, the automotive embedded software framework
- RF System Design Guide
- For CC2640R2f watchdog can be like this
- Please tell me the advantages and disadvantages of these two differential signal amplifications
- Nexperia ESD Webinar Invitation
- What is the four-wire resistance measurement? What are the advantages?
- This circuit for detecting mobile phones is no longer usable, right?
- STM32 stepper motor trapezoidal acceleration and deceleration program
- What is the way to distinguish whether series resonance is good or bad?
- Improved Snowy Night Christmas Tree Program
- National Geographic China and "Beautiful China - China's Most Beautiful Places Selection Event"