- 8051 memory types and memory areas
- Storage Mode
- Memory Type Declaration
- Variable type declaration
- Bit variables and bit addressing
- Special Function Registers (SFRs)
- C51 pointer
- Function Properties
The specific instructions are as follows (8031 is the default CPU).
Section 1 Keil C51 Extended Keywords
C51 V4.0 version has the following extended keywords (a total of 19):
_at_ idata sfr16 alien interrupt small
bdata large _task_ Code bit pdata
using reentrant xdata compact sbit data sfr
Section 2 Memory Areas:
1. Program Area:
The code indicates that there can be up to 64kBytes of program memory.
2. Internal Data Memory:
The internal data memory can be described using the following keywords:
data: directly addressable area, which is the lower 128 bytes of the internal RAM 00H to 7FH
idata: indirectly addressable area, including the entire internal RAM area 00H to FFH
bdata: bit addressable area, 20H to 2FH
3. External Data Memory
The external RAM can be identified by the following keywords depending on usage:
xdata: can specify up to 64KB of external direct addressing area, address range 0000H ~ 0FFFFH
pdata: can access 1 page (25bBytes) of external RAM, mainly used in compact model.
4. Speciac Function Register Memory
8051 provides 128Bytes of SFR addressing area, which can be bit-addressable, byte-addressable or word-addressable to control timers, counters, serial ports, I/O and other components. It can be described by the following keywords:
sfr: byte addressing, such as sfr P0=0x80; the PO port address is 80H, and the constant between H and FFH after "=".
sfr16: word addressing, such as sfr16 T2=0xcc; specifies the Timer2 port address T2L=0xcc T2H=0xCD
sbit: bit addressing, such as sbit EA=0xAF; specifies the 0xAF bit as EA, that is, interrupt enable
There may also be the following definition methods:
sbit 0V=PSW^2; (defining 0V as the second bit of PSW)
sbit 0V=0XDO^2; (same as above)
or bit 0V-=0xD2 (same as above).
Section 3 Storage Modes
The storage mode determines the default storage area for variables, function parameters, etc. that do not have an explicitly specified storage type. There are three types in total:
- Small mode:
All default variable parameters are loaded into the internal RAM. The advantage is fast access speed, but the disadvantage is limited space, so it is only suitable for small programs. - Compact mode
All default variables are located in one page (256Bytes) of the external RAM area. The specific page can be specified by P2 port, described in the STARTUP.A51 file, or specified by pdata. The advantage is that the space is more spacious than Small, the speed is slower than Small, and faster than Large. It is an intermediate state. - Large Mode
All default variables can be placed in up to 64KB of external RAM. The advantage is that the space is large and many variables can be stored. The disadvantage is that the speed is slow.
Tip: The storage mode is selected in the C51 compiler options.
Section 4 Storage Type Declaration
The storage type of a variable or parameter can be specified by the default type specified by the storage mode, or can be directly specified by a keyword declaration. Each type is described by: code, data, idata, xdata, pdata, for example:
data uar1
char code array[ ] = "hello!";
unsigned char xdata arr[10][4][4];
Section 5 Variables or Data Types
C51 provides the following extended data types:
bit: bit variable value is 0 or 1
sbit: bit variable defined from byte 0 or 1
sfr: byte address 0 to 255
sfr16: word address 0 to 65535
Other data types such as char, enum, short, int, long, float, etc. are the same as ANSI C.
Section 6 Bit Variables and Declarations
1. Bit Variables
Bit variables can be used as variable types, function declarations, function return values, etc., and are stored in internal RAM 20H to 2FH.
Note:
(1) Functions declared with #pragma dISAble and functions specified with "usign" cannot return bit values.
(2) A bit variable cannot be declared as a pointer, such as bit *ptr; it is wrong
(3) There cannot be a bit array, such as: bit arr[5]; it is wrong.
2. The bit addressable area 20H-2FH
can be defined as follows:
int bdata i;
char bdata arr[3],
then:
sbit bito=in0; sbit bit15=I^15;
sbit arr07=arr[0]^7; sbit arr15=arr[i]^7;
Section 7 Keil C51 Pointer
C51 supports Generic Pointer and Memory_Specific Pointer.
1. General pointers
The declaration and use of general pointers are the same as those in standard C, but the storage type of the pointer can also be specified. For example:
long * state; is a pointer to a long integer, and state itself is stored according to the storage mode.
char * xdata ptr; ptr is a pointer to char data, and ptr itself is placed in the external RAM area. The data pointed to by the above long, char and other pointers can be stored in any memory.
The general pointer itself is stored in 3 bytes, which are the memory type, high offset, and low offset.
2. Memory pointer
When describing a memory-based pointer, the storage type is specified, for example:
char data * str; str points to the char data in the data area
int xdata * pow; pow points to the int integer in the external RAM.
When storing this type of pointer, only one byte or two bytes are needed, because only the offset is needed.
3. Pointer conversion
That is, the pointer is converted between the above two types:
- When a memory-based pointer is passed as an argument to a function that expects a general pointer, the pointer is automatically converted.
- If you do not declare the prototype of an external function, the memory-based pointer will be automatically converted to a general pointer, causing an error. Therefore, please declare all function prototypes using "#include".
- The pointer type can be forcibly changed.
Section 8 Keil C51 Function
C51 function declaration extends ANSI C, including:
1. Interrupt function declaration:
The interrupt declaration method is as follows:
void serial_ISR () interrupt 4 [using 1]
{
/* ISR */
}
To improve the fault tolerance of the code, an iret statement is generated at the unused interrupt entry to define the unused interrupt.
/* define not used interrupt, so generate "IRET" in their entrance */
void extern0_ISR() interrupt 0{} /* not used */
void timer0_ISR () interrupt 1{} /* not used */
void extern1_ISR() interrupt 2{} /* not used */
void timer1_ISR () interrupt 3{} /* not used */
void serial_ISR () interrupt 4{} /* not used */
2. General storage work area
3. Select the general storage work area and declare it by using x, as shown in the above example.
4. Specify the storage mode
Declared by small, compact and large, for example:
void fun1(void) small { }
Tip: All internal variables of the function described by small use internal RAM. Key and frequently time-consuming places can be declared in this way to increase the running speed.
5. #pragma dISAble
is declared before the function and is only valid for one function. The function cannot be interrupted during the call.
6. Recursive or reentrant function specification
Functions that can be called in both the main program and the interrupt are prone to problems. Because 51 is different from PC, PC uses the stack to pass parameters, and internal variables other than static variables are all in the stack; while 51 generally uses registers to pass parameters, and internal variables are generally in RAM. When the function is reentered, the data of the last call will be destroyed. The following two methods can be used to solve the problem of function reentry:
a. Use the aforementioned "#pragma dISAble" declaration before the corresponding function, that is, only the main program or interrupt is allowed to call the function;
b. Describe the function as reentrant. As follows:
void func(param...) reentrant;
After KeilC51 is compiled, a reentrant variable stack will be generated, and then the method of passing variables through the stack can be simulated.
Since reentrant functions are generally called by the main program and interrupts, interrupts usually use a different R register group from the main program.
In addition, for reentrant functions, add the switch "#pragma noaregs" in front of the corresponding function to prohibit the compiler from using absolute register addressing, so that code that does not depend on the register group can be generated.
7. Specify PL/M-51 functions
Specified by alien
Previous article:The difference between data, idata, xdata, and pdata in the 51 series
Next article:How to set ROM using keil c51 to generate hex
Recommended ReadingLatest update time:2024-11-15 17:50
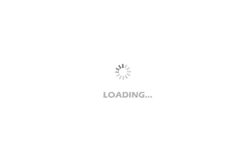
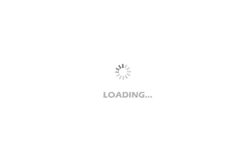
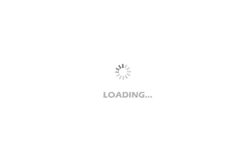
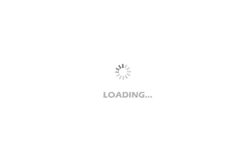
- Popular Resources
- Popular amplifiers
-
西门子S7-12001500 PLC SCL语言编程从入门到精通 (北岛李工)
-
Siemens Motion Control Technology and Engineering Applications (Tongxue, edited by Wu Xiaojun)
-
How to read electrical control circuit diagrams (Classic best-selling books on electronics and electrical engineering) (Zheng Fengyi)
-
MCU C language programming and Proteus simulation technology (Xu Aijun)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- LED chemical incompatibility test to see which chemicals LEDs can be used with
- Application of ARM9 hardware coprocessor on WinCE embedded motherboard
- What are the key points for selecting rotor flowmeter?
- LM317 high power charger circuit
- A brief analysis of Embest's application and development of embedded medical devices
- Single-phase RC protection circuit
- stm32 PVD programmable voltage monitor
- Introduction and measurement of edge trigger and level trigger of 51 single chip microcomputer
- Improved design of Linux system software shell protection technology
- What to do if the ABB robot protection device stops
- Keysight Technologies Helps Samsung Electronics Successfully Validate FiRa® 2.0 Safe Distance Measurement Test Case
- Innovation is not limited to Meizhi, Welling will appear at the 2024 China Home Appliance Technology Conference
- Innovation is not limited to Meizhi, Welling will appear at the 2024 China Home Appliance Technology Conference
- Huawei's Strategic Department Director Gai Gang: The cumulative installed base of open source Euler operating system exceeds 10 million sets
- Download from the Internet--ARM Getting Started Notes
- Learn ARM development(22)
- Learn ARM development(21)
- Learn ARM development(20)
- Learn ARM development(19)
- Learn ARM development(14)
- Chestnut Development Board-TI5708 First Hands-On Exploration
- Review summary: National Technology low-power series, N32L43x is now available for testing
- Some information about mobile power supplies (power banks)
- 【CH579M-R1】+U disk read/write
- Unboxing of Materials - STM32F767 & ESP32
- Prize Review: 50 Rapid IoT Kits from NXP to Proof of Concept in Minutes
- Download Keysight Technologies' e-book "X-Apps Treasure Map: Essential Measurement Apps for Signal Analyzers That Accelerate Tests" and receive a gift!
- [Evaluation of EC-01F-Kit, the EC-01F NB-IoT development board] Power-on and serial port test
- ELEXCON 2022 Shenzhen International Electronics Exhibition will open on November 6 (new schedule), hurry up and get your tickets! There are also many gifts waiting for you!
- Registration reminder: In the last few hours, 100 sets of Pingtouge RISC-V ecological development boards worth 390 yuan will be given away for free~