/************lcd initialization program**************/
void init_lcd (void)
{
wr_lcd (comm,0x30); /*30---basic instruction set action, 8-bit control interface*/
wr_lcd (comm,0x01); /*clear screen, address pointer points to 00H*/
delay (80);
wr_lcd (comm,0x06); /*cursor movement direction, cursor moves right*/
wr_lcd (comm,0x0c); /*turn on display, turn off cursor*/
}
/******************LCD displays arbitrary quantity program************************/
void eng_disp (uchar x,uchar y,uchar code *eng)
{
uchar i,j;
wr_lcd (comm,0x30);
wr_lcd (comm,0x80); /*The starting address of the first line*/
for(j=0;j
for (i=0;i
if((y==2)&&(j==1))
{wr_lcd (comm,0x90);
for (i=0;i<8;i++)
wr_lcd (dat,eng[j*16+i]);
}
}
}
/*************************LCD displays Chinese (welcome interface) program******************/
void chn_disp (uchar code *chn) /*lcd displays Chinese*/
{
uchar i,j;
wr_lcd (comm,0x30);
wr_lcd (comm,0x80); /*The starting address of the first line*/
j=0;
for (i=0;i<16;i++)
wr_lcd (dat,chn[j*16+i]);
wr_lcd (comm,0x90); //The starting address of the second line
j=1;
for (i=0;i<16;i++)
wr_lcd (dat,chn[j*16+i]);
}
/***************************LCD clear memory program**********************/
void clrram (void) //lcd clear memory
{
wr_lcd (comm,0x30);
wr_lcd (comm,0x01);
delay (120);
}
/*************LCD write data or write command subroutine****************/
void wr_lcd (uchar dat_comm,uchar content) /*lcd write data*/
{
uchar a,i,j;
delay (40);
a=content; //a is the instruction code or data
cs=1; //set cs to start transmitting data
sclk=0; //pull sclk low to prepare for the rising edge, send data
std=1; //set 1
for(i=0;i<5;i++) /*transmit the start byte first, first 5 1s, the rising edge of sclk starts the transmission*/
{
sclk=1;
sclk=0;
}
std=0; //RW=0;
sclk=1;
sclk=0;
if(dat_comm)
std=1; //if it is data, RS is 1
else
std=0; //if it is a control word, RS is 0
sclk=1; //give the rising edge, start the transmission
sclk=0; //pull sclk low
std=0; //the 8th bit is 0, the start byte transmission is completed
sclk=1; //Give rising edge to start transmission
sclk=0;
for(j=0;j<2;j++) //A byte is sent twice. The first time, the high four bits are sent, followed by 4 zeros. The second time, the low four bits are sent, followed by 4 zeros.
{
for(i=0;i<4;i++) //Transmit the high 4 bits first
{
a=a<<1; //Data is cyclically shifted left by one bit, and the low bit is filled with 0
std=CY; //Transmit the carry
sclk=1;
sclk=0;
}
std=0;
for(i=0;i<4;i++) //The last 4 bits of data are 4 0s
{
sclk=1;
sclk=0;
}
}
}
/**********************LCD delay program************************************/
void delay (uint us) //lcd delay time
{
while(us--);
}
void delay1 (uint ms)
{
uint i,j;
for(i=0;i
delay(1);
}
/*************************Display data bit subroutine*******************/
void math_disp(uint math)
{ uint k;
uchar i;
uchar j;
k=math;
dispbuf[0]=k/10000; //get the ten thousand digit
k=k%10000;
dispbuf[1]=k/1000; //get the thousand digit
k=k%1000;
dispbuf[2]=k/100; //get the hundred digit
k=k%100;
dispbuf[3]=k/10; //get the ten digit
dispbuf[4]=k%10; //get the unit digit
wr_lcd (comm,0x90); //give the starting address of the second line so that the data can be displayed in the second line,
for(i=0;i<5;i++)
{
for(j=0;j<2;j++) //continuously display the bytes in two tab2, LCD automatically connects these two bytes to display one data
{
wr_lcd(dat,tab2[xs2*dispbuf[i]+j]); //Call display subfunction
}
}
}
Previous article:Homemade 51 MCU common header files (st7920 parallel mode)
Next article:51 MCU and PC serial communication program and hardware circuit diagram
Recommended ReadingLatest update time:2024-11-16 15:00
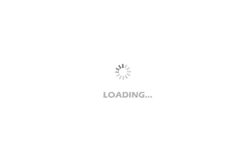
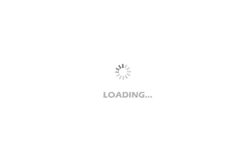
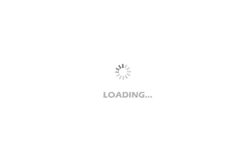
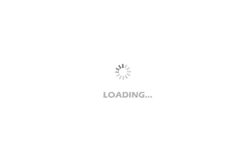
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- A brief introduction to commonly used wireless technologies
- Disassembly of the SPX01W power bank that supports wireless charging
- (1) Unboxing experience based on the STM32L4R9 MEMS sensor board (IKS01A3)
- EEWORLD University ---- Razavi Analog Integrated Circuit Tutorial (English)
- About the uboot compilation problem of am335x
- Here is the motor drive information on the national competition list
- RC snubber circuit design principles
- EEWORLD University ---- Linux driver tutorial (by itop4412)
- DSP 28335 program automatic upgrade solution
- What is the IoT edge? Where is the IoT edge?