* * Software function: timer interrupt experiment * */ #include "stm32f10x.h" #include "delay.h" void RCC_Configuration(void); void GPIO_Configuration(void); void NVIC_Configuration(void); void TIM3_Configuration(u16 arr,u16 psc); /* Function: int main(void) Function: main function Parameters: None Returns: None / int main(void) { RCC_Configuration(); GPIO_Configuration(); NVIC_Configuration(); TIM3_Configuration(4999,7199); //10Khz counting frequency, 500ms when counting to 5000 //delay_init(72); GPIO_ResetBits(GPIOB,GPIO_Pin_0); while(1); } /* Function: void RCC_Configuration(void) Function: Reset and clock control configuration Parameters: None Returns: None / void RCC_Configuration(void) { ErrorStatus HSEStartUpStatus; //Define the external high-speed crystal startup status enumeration variable RCC_DeInit(); //Reset RCC external device registers to default values RCC_HSEConfig(RCC_HSE_ON); //Turn on the external high-speed crystal oscillator HSEStartUpStatus = RCC_WaitForHSEStartUp(); //Wait for the external high-speed clock to be ready if(HSEStartUpStatus == SUCCESS) //The external high-speed clock is ready { FLASH_PrefetchBufferCmd(FLASH_PrefetchBuffer_Enable); // Enable the FLASH pre-read buffer function to speed up the reading of FLASH. Required usage in all programs. Location: in the RCC initialization sub-function, after the clock starts FLASH_SetLatency(FLASH_Latency_2); //Flash operation delay RCC_HCLKConfig(RCC_SYSCLK_Div1); //Configure AHB (HCLK) clock equal to == SYSCLK RCC_PCLK2Config(RCC_HCLK_Div1); //Configure APB2(PCLK2) clock==AHB clock RCC_PCLK1Config(RCC_HCLK_Div2); //Configure APB1(PCLK1) clock == AHB1/2 clock RCC_PLLConfig(RCC_PLLSource_HSE_Div1, RCC_PLLMul_9); //Configure PLL clock == external high-speed crystal clock * 9 = 72MHz RCC_PLLCmd(ENABLE); //Enable PLL clock while(RCC_GetFlagStatus(RCC_FLAG_PLLRDY) == RESET) //Wait for PLL clock to be ready { } RCC_SYSCLKConfig(RCC_SYSCLKSource_PLLCLK); //Configure system clock = PLL clock while(RCC_GetSYSCLKSource() != 0x08) //Check if PLL clock is used as system clock { } } RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB | RCC_APB2Periph_AFIO, ENABLE); //Enable GPIOB and AFIO clocks RCC_APB1PeriphClockCmd(RCC_APB1Periph_TIM3, ENABLE); //Clock enable } /* Function: void GPIO_Configuration(void) Function: GPIO configuration Parameters: None Returns: None / void GPIO_Configuration(void) { GPIO_InitTypeDef GPIO_InitStructure; //Define GPIO initialization structure GPIO_InitStructure.GPIO_Pin = GPIO_Pin_0; GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz; GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP; GPIO_Init(GPIOB, &GPIO_InitStructure); //PB is used to output and control the LED light } void NVIC_Configuration(void) //Interrupt grouping and priority configuration For details, see "STM32 Function Description (Chinese).pdf" P165 { NVIC_InitTypeDef NVIC_InitStructure; NVIC_InitStructure.NVIC_IRQChannel = TIM3_IRQn; //TIM3 interrupt NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 0; //Preempt priority level 0 NVIC_InitStructure.NVIC_IRQChannelSubPriority = 3; //From priority level 3 NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE; //IRQ channel is enabled NVIC_Init(&NVIC_InitStructure); //Initialize NVIC registers } void TIM3_Configuration(u16 arr,u16 psc) //TIM3 timer configuration { TIM_TimeBaseInitTypeDef TIM_TimeBaseStructure; TIM_TimeBaseStructure.TIM_Period = arr; //Set the value of the auto-reload register period to load the activity at the next update event TIM_TimeBaseStructure.TIM_Prescaler =psc; //Set the prescaler value used as the TIMx clock frequency divisor TIM_TimeBaseStructure.TIM_ClockDivision = TIM_CKD_DIV1; //Set clock division: TDTS = Tck_tim TIM_TimeBaseStructure.TIM_CounterMode = TIM_CounterMode_Up; //TIM up counting mode TIM_TimeBaseInit(TIM3, &TIM_TimeBaseStructure); //Initialize the time base unit of TIMx according to the specified parameters /*((1+7199[TIM_Prescaler] )/72M)*(1+4999[TIM_Period] )=500,000us=500ms */ TIM_ITConfig(TIM3,TIM_IT_Update,ENABLE); //Enable the specified TIM3 interrupt and allow update interrupt TIM_Cmd(TIM3, ENABLE); //Enable TIMx } //Timer 3 interrupt service routine void TIM3_IRQHandler(void) //TIM3 interrupt { if (TIM_GetITStatus(TIM3, TIM_IT_Update) != RESET) //Check whether TIM3 update interrupt occurs { TIM_ClearITPendingBit(TIM3, TIM_IT_Update ); //Clear TIMx update interrupt flag if(!GPIO_ReadInputDataBit(GPIOB,GPIO_Pin_0)) GPIO_SetBits(GPIOB,GPIO_Pin_0); else GPIO_ResetBits(GPIOB,GPIO_Pin_0); } }
Previous article:STM32 MCU Learning (11) DS18B20 Temperature Sensor Experiment
Next article:STM32 MCU learning (10) Digital tube output experiment supplement static (common anode) + dynamic (common cathode)
Recommended ReadingLatest update time:2024-11-16 19:40
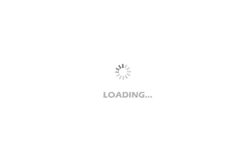
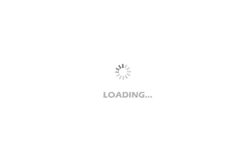
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Understanding of USB-Serial, USB to Serial, USB-UART, PL2303, CH340, etc.
- [RVB2601 Creative Application Development] Design of a Farmland Pest Control Device
- [RVB2601 Creative Application Development] 2. Light up the LED
- Level shifter reverse circuit
- Please advise, is it necessary to consider the safety power when calculating the LED resistance?
- [RVB2601 creative application development] u8g2+IP
- What are the screw holes in this package for?
- Summary of learning about state machine scheduling design
- After adding a product to the ONENET platform, when adding a device, the automatic subscription option allows the device to subscribe to ONENET messages?
- Power chip static current