Directly use the external clock source
Select by setting the pin
FCLK
HCLK
PCLK
PLL increases the system clock speed through the clock control logic PLL
UPLL
MPLL
PLL is not started FCLK=Fin (crystal frequency)
The s3c2410 has five 16-bit timers,
@**********************************************************************************
@ File: head.S
@ Function: Initialize, set the interrupt mode, system mode stack, and interrupt handling function
@******************************************************************************
.extern
.text
.global _start
_start:
@**********************************************************************************
@ Interrupt vector. In this program, except Reset and HandleIRQ, other exceptions are not used
@******************************************************************************
@ 0x04: The vector address of the undefined instruction abort mode
HandleUndef:
@ 0x08: The vector address of the management mode, which is entered by the SWI instruction
HandleSWI:
@ 0x0c: vector address of the exception caused by instruction prefetch termination
HandlePrefetchAbort:
@ 0x10: The vector address of the exception caused by data access termination
HandleDataAbort:
@ 0x14: Reserved
HandleNotUsed:
@ 0x18: interrupt mode vector address
@ 0x1c: Vector address of fast interrupt mode
HandleFIQ:
Reset:
on_sdram:
halt_loop:
HandleIRQ:
int_return:
#include "s3c24xx.h"
void disable_watch_dog(void);
void clock_init(void);
void memsetup(void);
void copy_steppingstone_to_sdram(void);
void init_led(void);
void timer0_init(void);
void init_irq(void);
void disable_watch_dog(void)
{
}
#define S3C2410_MPLL_200MHZ
#define S3C2440_MPLL_200MHZ
void clock_init(void)
{
__asm__(
}
void memsetup(void)
{
}
void copy_steppingstone_to_sdram(void)
{
}
#define GPB5_out
#define GPB6_out
#define GPB7_out
#define GPB8_out
#define GPG11_eint
#define GPG3_eint
#define GPF3_eint
#define GPF2_eint
void init_led(void)
{
}
void timer0_init(void)
{
}
void init_irq(void)
{
}
#include "s3c24xx.h"
void Timer0_Handle(void)
{
}
int main(void)
{
}
Previous article:UART Operation
Next article:Operation of s3c24xx interrupts
Recommended ReadingLatest update time:2024-11-16 07:40
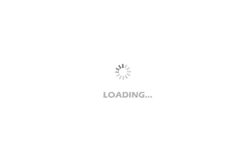
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- GD32E231 Learning 4: External Interrupt EXTI
- The smallest Bluetooth module in the industry? Now available
- Questions about I2C communication
- Bullshit, playing with a 4.2-inch e-ink screen price tag
- ZigBee (CC2530, ZSTACK) transparent transmission example
- Unboxing - ESP32-S2-KALUGA-1
- Shanghai ACM32F070 development board evaluation 2, MDK programming environment
- TMS320C6000-CCS software development environment construction
- [RVB2601 Creative Application Development] Simulating UART 2
- Tips to improve DC-DC dynamic characteristics