The RTC unit operates on an
external 32.768kHz crystal oscillator and can perform alarm functions.
RTC operation is relatively simple, and the register settings are described in detail in the data sheet, so I will not write about them here. I will post an RTC code. This program refers to Tianxiang's RTC program code, mainly because his code is better and modularized. I modified his code to have an alarm (using a buzzer) and it can run on the TQ2440 board. Program functions: The serial port displays the time once per second and LED1 flashes once. In the alarm setting, when the second is 20, the alarm time is displayed and the buzzer sounds for a few seconds.
- #include "2440addr.h"
- #include "Option.h"
- #include "2440lib.h"
- #include "def.h"
- #define LED1_ON (rGPBDAT &=~(1<<5))
- #define LED1_OFF (rGPBDAT |=(1<<5) )
- void __irq RTC_tickHandler(void);
- void __irq RTC_alarmHandler(void);
- U8 alarmflag=0;
- typedef struct Date //Define a structure representing date and time
- { U16 year;
- U8 month;
- U8 day;
- U8 week_day;
- U8 hour;
- U8 minute;
- U8 second;
- }date;
- date C_date;
- char *week_num[7]={ "SUN","MON","TUES","WED","THURS","FRI","SAT" }; //define a pointer array
- void Beep_Freq_Set( U32 freq )
- {
- rGPBCON &=~3;
- rGPBCON |= 2; //Set GPB0 to OUT0
- rGPBUP=0x0; //Enable pull-up
- rTCFG0 &=~0xff;
- rTCFG0 |=15; //Prescaler value is 15
- rTCFG1 &=~0x0f;
- rTCFG1 |=0x02; //Division value is 8
- rTCNTB0 = (PCLK>>7)/freq; //Set the value of timer 0 count buffer
- rTCMPB0 = rTCNTB0>>1; // Timer 0 compares the buffer value, PWM output duty cycle 50%
- rTCON &= ~0x1f;
- rTCON |= 0xb; //Auto reload, turn off phase shift, manually update, turn on timer 0
- rTCON &= ~2; // Clear the manual update bit
- }
- void Beep_Stop( void )
- {
- rGPBCON &= ~3; //set GPB0 as output
- rGPBCON |= 1;
- rGPBDAT &= ~1; //output low level
- }
- void delay(int x)
- {
- int i,j;
- for(i=0;i
- for(j=0;j<1000000;j++);
- }
- /**********************************
- *
- * Set the real-time clock date and time
- *
- *********************************/
- void RTC_setdate(date *p_date)
- {
- rRTCCON=0x01; //RTC read and write enable, BCD clock, counter, no reset
- rBCDYEAR = p_date->year;
- rBCDMON = p_date->month;
- rBCDDATE = p_date->day;
- rBCDDAY = p_date->week_day; //Set date and time
- rBCDHOUR = p_date->hour;
- rBCDMIN = p_date->minute;
- rBCDSEC = p_date->second;
- rRTCCON=0x00; //RTC read and write disabled, BCD clock, counter, no reset
- }
- /**********************************
- *
- * Read real-time clock date and time
- *
- *********************************/
- void RTC_getdate(date *p_date)
- {
- rRTCCON=0x01; //RTC read and write enable, BCD clock, counter, no reset
- p_date->year = rBCDYEAR+0x2000;
- p_date->month = rBCDMON;
- p_date->day = rBCDDATE;
- p_date->week_day = rBCDDAY; //Read date and time
- p_date->hour = rBCDHOUR;
- p_date->minute = rBCDMIN;
- p_date->second = rBCDSEC;
- rRTCCON=0x00; //RTC read and write disabled, BCD clock, counter, no reset
- }
- /**********************************
- *
- * TICK interrupt initialization
- *
- *********************************/
- void RTC_tickIRQ_Init(U8 tick)
- {
- ClearPending(BIT_TICK); // Clear the flag
- EnableIrq(BIT_TICK); //Enable interrupt source
- pISR_TICK=(unsigned)RTC_tickHandler; //interrupt function entry address
- rRTCCON=0x00;
- rTICNT=(tick&0x7f)|0x80; //Enable interrupt
- }
- /**********************************
- *
- * Set alarm date, time and alarm wake-up mode
- *
- *********************************/
- void RTC_alarm_setdate(date *p_date,U8 mode)
- {
- rRTCCON = 0x01;
- rALMYEAR = p_date->year;
- rALMMON = p_date->month;
- rALMDATE = p_date->day;
- rALMHOUR = p_date->hour;
- rALMMIN = p_date->minute;
- rALMSEC = p_date->second;
- rRTCALM = mode; //RTC alarm control register
- rRTCCON = 0x00;
- ClearPending(BIT_RTC); //Clear flag
- EnableIrq(BIT_RTC); //open RTC alarm INTERRUPT
- pISR_RTC = (unsigned)RTC_alarmHandler;
- }
- void Main(void)
- {
- SelectFclk(2); //Set the system clock to 400M
- ChangeClockDivider(2, 1); //Set the frequency division to 1:4:8
- CalcBusClk(); //Calculate bus frequency
- rGPHCON &=~((3<<4)|(3<<6));
- rGPHCON |=(2<<4)|(2<<6); //GPH2--TXD[0];GPH3--RXD[0]
- rGPHUP=0x00; //Enable pull-up function
- Uart_Init(0,115200);
- Uart_Select(0);
- rGPBCON &=~((3<<10)|(3<<12)|(3<<14)|(3<<16)); // Clear GPBCON[10:17]
- rGPBCON |=((1<<10)|(1<<12)|(1<<14)|(1<<16)); //Set GPB5~8 to output
- rGPBUP &=~((1<<5)|(1<<6)|(1<<7)|(1<<8)); //Set the pull-up function of GPB5~8
- rGPBDAT |=(1<<5)|(1<<6)|(1<<7)|(1<<8); //Turn off LED
- Beep_Stop(); //The buzzer stops sounding and is used as an alarm
- C_date.year = 0x12;
- C_date.month = 0x05;
- C_date.day = 0x09;
- C_date.week_day = 0x03; //Set the current date and time
- C_date.hour = 0x12;
- C_date.minute = 0x00;
- C_date.second = 0x10;
- RTC_setdate(&C_date);
- C_date.second=0x20;
- RTC_alarm_setdate(&C_date,0x41); //0x41 means enabling the RTC alarm and the second clock alarm
- RTC_tickIRQ_Init(127); // Set the tick to occur once per second
- Uart_Printf("\n ---Real-time clock test program---\n");
- while(Uart_GetKey()!= ESC_KEY)
- {
- LED1_OFF;
- RTC_getdate(&C_date);
- if(alarmflag)
- {
- alarmflag=0;
- Uart_Printf("\nRTC ALARM %02x:%02x:%02x \n",C_date.hour,C_date.minute,C_date.second);
- Beep_Freq_Set(1000);
- delay(5);
- Beep_Stop();
- }
- }
- }
- /**********************************
- *
- * TICK interrupt
- *
- *********************************/
- void __irq RTC_tickHandler(void)
- {
- ClearPending(BIT_TICK);
- LED1_ON; //Refresh LED1
- Delay(500);
- RTC_getdate(&C_date);
- Uart_Printf("RTC TIME: %04x-%02x-%02x %s %02x:%02x:%02x\n", C_date.year,C_date.month,C_date.day,week_num[C_date.week_day], C_date.hour , C_date.minute, C_date.second );
- }
- /**********************************
- *
- * TICK interrupt
- *
- *********************************/
- void __irq RTC_alarmHandler(void)
- {
- alarmflag = 1;
- ClearPending(BIT_RTC);
- }
Previous article:S3C2440 IIC bus interface
Next article:S3C2440 Interrupt Controller
Recommended ReadingLatest update time:2024-11-16 17:40
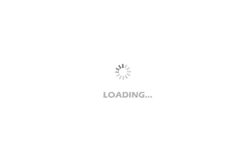
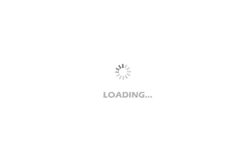
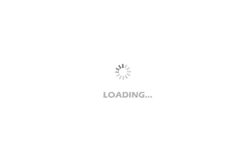
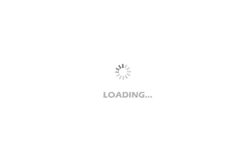
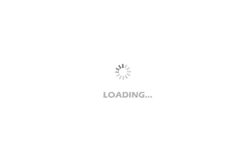
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- May I ask if Mr. Yi Zhongtian mentioned in a certain program that he talked about "have you learned it?", "have you learned it thoroughly?", "have you learned it thoroughly?", in which program did he say this?
- Is it as shown in the picture?
- PCB file conversion
- 5G indoor base stations will be released soon, and it is expected that each household will have a small base station, which will subvert the existing home Internet access methods.
- How to consider the impact of audio analog signals after being transmitted through a 100m long cable?
- Angle sensor
- Transistor Selection
- NUCLEO_G431RB Review - RTC Real-time Clock
- 【New Year's Taste Competition】+ Spring Festival Customs
- ESD electrostatic protection classic book - "ESD Secrets: Electrostatic Protection Principles and Typical Applications"