(3) The source code is explained as follows:
- #include "REG52.H"
- #define const_rc_size 36 //Buffer array size for receiving serial port interrupt data
- #define const_least_size 26 //The size of a string of standard data
- void initial_myself();
- void initial_peripheral();
- void delay_short(unsigned int uiDelayShort);
- void delay_long(unsigned int uiDelaylong);
- //74HC595 driving the digital tube
- void dig_hc595_drive(unsigned char ucDigStatusTemp16_09, unsigned char ucDigStatusTemp08_01);
- void display_drive(); //Drive function for displaying digital tube fonts
- void display_service(); //Display window menu service program
- //74HC595 driving LED
- void hc595_drive(unsigned char ucLedStatusTemp16_09, unsigned char ucLedStatusTemp08_01);
- void usart_service(void); //Serial port receiving service program, in the main function
- void usart_receive(void); //Serial port receive interrupt function
- void T0_time(); //Timer interrupt function
- sbit dig_hc595_sh_dr=P2^0; //74HC595 program of digital tube
- sbit dig_hc595_st_dr=P2^1;
- sbit dig_hc595_ds_dr=P2^2;
- sbit hc595_sh_dr=P2^3; //74HC595 program for LED light
- sbit hc595_st_dr=P2^4;
- sbit hc595_ds_dr=P2^5;
- sbit beep_dr=P2^7; //Buzzer driver IO port
- sbit led_dr=P3^5; //Independent LED light
- //Common cathode digital tube character table obtained based on the schematic diagram
- code unsigned char dig_table[]=
- {
- 0x3f, //0 Sequence number 0
- 0x06, //1 Sequence number 1
- 0x5b, //2 Sequence number 2
- 0x4f, //3 Sequence number 3
- 0x66, //4 Sequence number 4
- 0x6d, //5 Sequence number 5
- 0x7d, //6 Sequence number 6
- 0x07, //7 Serial number 7
- 0x7f, //8 Sequence number 8
- 0x6f, //9 Sequence number 9
- 0x00, //No sequence number 10
- 0x40, //- Sequence number 11
- 0x73, //P serial number 12
- };
- unsigned int uiRcregTotal=0; // represents how much data the current buffer has received
- unsigned int uiRcregTotalTemp=0; //Intermediate variable representing how much data the current buffer has received
- unsigned char ucRcregBuf[const_rc_size]; //Buffer array for receiving serial port interrupt data
- unsigned char ucReceiveFlag=0; //Receive success flag
- unsigned char ucDigShow8; //The content to be displayed on the 8th digital tube
- unsigned char ucDigShow7; //The content to be displayed on the 7th digital tube
- unsigned char ucDigShow6; //The content to be displayed on the 6th digital tube
- unsigned char ucDigShow5; //The content to be displayed on the 5th digital tube
- unsigned char ucDigShow4; //The content to be displayed on the 4th digital tube
- unsigned char ucDigShow3; //The content to be displayed on the third digital tube
- unsigned char ucDigShow2; //The content to be displayed on the second digital tube
- unsigned char ucDigShow1; //The content to be displayed on the first digital tube
- unsigned char ucDigDot8; //Whether the decimal point of digital tube 8 is displayed
- unsigned char ucDigDot7; //Whether the decimal point of digital tube 7 is displayed
- unsigned char ucDigDot6; //Whether the decimal point of digital tube 6 is displayed
- unsigned char ucDigDot5; //Whether the decimal point of digital tube 5 is displayed
- unsigned char ucDigDot4; //Whether the decimal point of digital tube 4 is displayed
- unsigned char ucDigDot3; //Whether the decimal point of digital tube 3 is displayed
- unsigned char ucDigDot2; //Whether the decimal point of digital tube 2 is displayed
- unsigned char ucDigDot1; //Whether the decimal point of digital tube 1 is displayed
- unsigned char ucDigShowTemp=0; //temporary intermediate variable
- unsigned char ucDisplayDriveStep=1; //Dynamic scanning digital tube step variable
- unsigned char ucWd1Part1Update=1; //8-bit digital tube update display flag
- unsigned long ulWeightCurrent=12345; //Display the current actual weight
- void main()
- {
- initial_myself();
- delay_long(100);
- initial_peripheral();
- while(1)
- {
- usart_service(); //Serial port receiving service program
- display_service(); //Display window menu service program
- }
- }
- /* Note 1:
- * This section processes serial port data based on whether the data string is valid based on whether the data tail has 0x0d 0x0a. Once this keyword is found,
- * Then determine whether the total data length is equal to or greater than the fixed length of a string of data. If it is satisfied, set the relevant flag to notify the main function
- * The serial port service program is used for processing. At the same time, the serial port interrupt is also closed in time to avoid interruption of serial port data during the processing of serial port data.
- * Wait until the serial port service program has finished processing before opening it.
- */
- void usart_receive(void) interrupt 4 //Serial port receives data interrupt function
- {
- if(RI==1)
- {
- RI = 0;
- ++uiRcregTotal;
- ucRcregBuf[uiRcregTotal-1]=SBUF; //Cache the data received by the serial port into the receiving buffer
- if(uiRcregTotal>=2&&ucRcregBuf[uiRcregTotal-2]==0x0d&&ucRcregBuf[uiRcregTotal-1]==0x0a) //Once the suffix is found to be 0x0d 0x0a, it will be processed and judged
- {
- if(uiRcregTotal
- {
- uiRcregTotal=0;
- }
- else
- {
- uiRcregTotalTemp=uiRcregTotal; //Pass the total received data to an intermediate variable and process the intermediate variable in the main function
- ucReceiveFlag=1; //Notify the main program of successful reception
- ES=0; // Disable receiving interrupt, and enable serial port interrupt after the main function has processed the received data, to avoid interrupt interference from serial port data during processing of serial port data.
- }
- }
- else if(uiRcregTotal>=const_rc_size) //Exceeds the buffer
- {
- uiRcregTotal=0;
- }
- }
- else //If it is not a serial port receiving interrupt, then it must be a serial port sending interrupt. Clear the send interrupt flag in time, otherwise the send interrupt will continue.
- {
- TI = 0;
- }
- }
- void usart_service(void) //Serial port receiving service program, in the main function
- {
- //After adding the static keyword, this local variable will not be initialized every time the function comes in, which may reduce the time consumed by instructions.
- static unsigned long ulReceiveData10000; //Defined as long type to facilitate the subsequent multiplication operation so that it will not overflow and cause errors.
- static unsigned long ulReceiveData1000;
- static unsigned long ulReceiveData100;
- static unsigned long ulReceiveData10;
- static unsigned long ulReceiveData1;
- if(ucReceiveFlag==1) //Indicates that data has been received successfully and enters data processing and analysis
- {
- ulReceiveData10000=0;
- ulReceiveData1000=0;
- ulReceiveData100=0;
- ulReceiveData10=0;
- ulReceiveData1=0;
- /* Note 2:
- * According to the protocol, the 9th, 10th, 11th, 12th, 13th, and 14th digits from the bottom are valid ASCII code numbers, and the 11th digit from the bottom is a fixed decimal point, so it is omitted.
- */
- if(ucRcregBuf[uiRcregTotalTemp-9]>=0x30)
- {
- ulReceiveData1=ucRcregBuf[uiRcregTotalTemp-9]-0x30; //The received ASCII code number minus 0x30 becomes the actual value.
- }
- if(ucRcregBuf[uiRcregTotalTemp-10]>=0x30)
- {
- ulReceiveData10=ucRcregBuf[uiRcregTotalTemp-10]-0x30;
- ulReceiveData10=ulReceiveData10*10;
- }
- if(ucRcregBuf[uiRcregTotalTemp-12]>=0x30)
- {
- ulReceiveData100=ucRcregBuf[uiRcregTotalTemp-12]-0x30;
- ulReceiveData100=ulReceiveData100*100;
- }
- if(ucRcregBuf[uiRcregTotalTemp-13]>=0x30)
- {
- ulReceiveData1000=ucRcregBuf[uiRcregTotalTemp-13]-0x30;
- ulReceiveData1000=ulReceiveData1000*1000;
- }
- if(ucRcregBuf[uiRcregTotalTemp-14]>=0x30)
- {
- ulReceiveData10000=ucRcregBuf[uiRcregTotalTemp-14]-0x30;
- ulReceiveData10000=ulReceiveData10000*10000;
- }
- ulWeightCurrent=ulReceiveData10000+ulReceiveData1000+ulReceiveData100+ulReceiveData10+ulReceiveData1;
- ucWd1Part1Update=1; //Update display
- uiRcregTotalTemp=0; //Clear the intermediate variable of the actual number of bytes received
- uiRcregTotal=0; // Clear the number of bytes actually received
- ucReceiveFlag=0; //Clear completion flag
- ES = 1; // Enable receive interrupt
- }
- }
- void display_service() //Display window menu service program
- {
- //After adding the static keyword, this local variable will not be initialized every time the function comes in, which may reduce the time consumed by instructions.
- static unsigned char ucTemp5; //intermediate transition variable
- static unsigned char ucTemp4; //intermediate transition variable
- static unsigned char ucTemp3; //intermediate transition variable
- static unsigned char ucTemp2; //intermediate transition variable
- static unsigned char ucTemp1; //intermediate transition variable
- if(ucWd1Part1Update==1) //Update display
- {
- ucWd1Part1Update=0; //Clear the flag in time to avoid continuous scanning
- //First decompose the data to display each bit
- ucTemp5=ulWeightCurrent%100000/10000;
- ucTemp4=ulWeightCurrent%10000/1000;
- ucTemp3=ulWeightCurrent%1000/100;
- ucTemp2=ulWeightCurrent%100/10;
- ucTemp1=ulWeightCurrent%10;
- ucDigDot3=1; //Display the decimal point of the 3rd digital tube, the actual data has 2 decimal points.
- ucDigShow8=10; //The 8th digital tube is not used, so the display is none. 10 means the display is empty.
- ucDigShow7=10; //The 7th digital tube is not used, so the display is none. 10 means the display is empty.
- ucDigShow6=10; //The sixth digital tube is not used, so the display is none. 10 means the display is empty.
- if(ulWeightCurrent<10000)
- {
- ucDigShow5=10; //If it is less than 1000, the thousands place will be displayed
- }
- else
- {
- ucDigShow5=ucTemp5; //The content to be displayed on the 5th digital tube
- }
- if(ulWeightCurrent<1000)
- {
- ucDigShow4=10; //If less than 1000, thousands will be displayed
- }
- else
- {
- ucDigShow4=ucTemp4; //The content to be displayed on the 4th digital tube
- }
- // Because there are 2 decimal places, the first 3 digits are valid numbers and must be displayed. Do not judge and remove the blank display processing of 0.
- ucDigShow3=ucTemp3; //The content to be displayed on the third digital tube
- ucDigShow2=ucTemp2; //The content to be displayed on the second digital tube
- ucDigShow1=ucTemp1; //The content to be displayed on the first digital tube
- }
- }
- void display_drive()
- {
- // The following program can also compress the capacity if some arrays and shifted elements are added. However, what Hongge pursues is not capacity, but clear explanation ideas.
- switch(ucDisplayDriveStep)
- {
- case 1: //Display the first position
- ucDigShowTemp=dig_table[ucDigShow1];
- if(ucDigDot1==1)
- {
- ucDigShowTemp=ucDigShowTemp|0x80; //Display decimal point
- }
- dig_hc595_drive(ucDigShowTemp,0xfe);
- break;
- case 2: //Display the second digit
- ucDigShowTemp=dig_table[ucDigShow2];
- if(ucDigDot2==1)
- {
- ucDigShowTemp=ucDigShowTemp|0x80; //Display decimal point
- }
- dig_hc595_drive(ucDigShowTemp,0xfd);
- break;
- case 3: //Display the third digit
- ucDigShowTemp=dig_table[ucDigShow3];
- if(ucDigDot3==1)
- {
- ucDigShowTemp=ucDigShowTemp|0x80; //Display decimal point
- }
- dig_hc595_drive(ucDigShowTemp,0xfb);
- break;
- case 4: //Display the 4th digit
- ucDigShowTemp=dig_table[ucDigShow4];
- if(ucDigDot4==1)
- {
- ucDigShowTemp=ucDigShowTemp|0x80; //Display decimal point
- }
- dig_hc595_drive(ucDigShowTemp,0xf7);
- break;
- case 5: //Display the 5th digit
- ucDigShowTemp=dig_table[ucDigShow5];
- if(ucDigDot5==1)
- {
- ucDigShowTemp=ucDigShowTemp|0x80; //Display decimal point
- }
- dig_hc595_drive(ucDigShowTemp,0xef);
- break;
- case 6: //Display the 6th digit
- ucDigShowTemp=dig_table[ucDigShow6];
- if(ucDigDot6==1)
- {
- ucDigShowTemp=ucDigShowTemp|0x80; //Display decimal point
- }
- dig_hc595_drive(ucDigShowTemp,0xdf);
- break;
- case 7: //Display the 7th position
- ucDigShowTemp=dig_table[ucDigShow7];
- if(ucDigDot7==1)
- {
- ucDigShowTemp=ucDigShowTemp|0x80; //Display decimal point
- }
- dig_hc595_drive(ucDigShowTemp,0xbf);
- break;
- case 8: //Display the 8th digit
- ucDigShowTemp=dig_table[ucDigShow8];
- if(ucDigDot8==1)
- {
- ucDigShowTemp=ucDigShowTemp|0x80; //Display decimal point
- }
- dig_hc595_drive(ucDigShowTemp,0x7f);
- break;
- }
- ucDisplayDriveStep++;
- if(ucDisplayDriveStep>8) //After scanning 8 digital tubes, restart scanning from the first one
- {
- ucDisplayDriveStep=1;
- }
- }
- //74HC595 driver function of digital tube
- void dig_hc595_drive(unsigned char ucDigStatusTemp16_09, unsigned char ucDigStatusTemp08_01)
- {
- unsigned char i;
- unsigned char ucTempData;
- dig_hc595_sh_dr=0;
- dig_hc595_st_dr=0;
- ucTempData=ucDigStatusTemp16_09; //Send the high 8 bits first
- for(i=0;i<8;i++)
- {
- if(ucTempData>=0x80)dig_hc595_ds_dr=1;
- else dig_hc595_ds_dr=0;
- dig_hc595_sh_dr=0; //The rising edge of the SH pin sends data to the register
- delay_short(1);
- dig_hc595_sh_dr=1;
- delay_short(1);
- ucTempData=ucTempData<<1;
- }
- ucTempData=ucDigStatusTemp08_01; //Send the lower 8 bits first
- for(i=0;i<8;i++)
- {
- if(ucTempData>=0x80)dig_hc595_ds_dr=1;
- else dig_hc595_ds_dr=0;
- dig_hc595_sh_dr=0; //The rising edge of the SH pin sends data to the register
- delay_short(1);
- dig_hc595_sh_dr=1;
- delay_short(1);
- ucTempData=ucTempData<<1;
- }
- dig_hc595_st_dr=0; //The ST pin updates the data of the two registers and outputs them to the output pin of 74HC595 and latches them
- delay_short(1);
- dig_hc595_st_dr=1;
- delay_short(1);
- dig_hc595_sh_dr=0; //Pull low to enhance anti-interference
- dig_hc595_st_dr=0;
- dig_hc595_ds_dr=0;
- }
- //74HC595 driver function for LED lamp
- void hc595_drive(unsigned char ucLedStatusTemp16_09, unsigned char ucLedStatusTemp08_01)
- {
- unsigned char i;
- unsigned char ucTempData;
- hc595_sh_dr=0;
- hc595_st_dr=0;
- ucTempData=ucLedStatusTemp16_09; //Send the high 8 bits first
- for(i=0;i<8;i++)
- {
- if(ucTempData>=0x80)hc595_ds_dr=1;
- else hc595_ds_dr=0;
- hc595_sh_dr=0; //The rising edge of the SH pin sends data to the register
- delay_short(1);
- hc595_sh_dr=1;
- delay_short(1);
- ucTempData=ucTempData<<1;
- }
- ucTempData=ucLedStatusTemp08_01; //Send the lower 8 bits first
- for(i=0;i<8;i++)
- {
- if(ucTempData>=0x80)hc595_ds_dr=1;
- else hc595_ds_dr=0;
- hc595_sh_dr=0; //The rising edge of the SH pin sends data to the register
- delay_short(1);
- hc595_sh_dr=1;
- delay_short(1);
- ucTempData=ucTempData<<1;
- }
- hc595_st_dr=0; //The ST pin updates the data of the two registers and outputs them to the output pin of 74HC595 and latches them
- delay_short(1);
- hc595_st_dr=1;
- delay_short(1);
- hc595_sh_dr=0; //Pull low to enhance anti-interference
- hc595_st_dr=0;
- hc595_ds_dr=0;
- }
- void T0_time() interrupt 1
- {
- TF0=0; //Clear interrupt flag
- TR0=0; //Disable interrupt
- display_drive(); //Drive function of digital tube font
- TH0=0xfe; //Reinstall initial value (65535-500)=65035=0xfe0b
- TL0=0x0b;
- TR0=1; //Open interrupt
- }
- void delay_short(unsigned int uiDelayShort)
- {
- unsigned int i;
- for(i=0;i
- {
- ; //A semicolon is equivalent to executing an empty statement
- }
- }
- void delay_long(unsigned int uiDelayLong)
- {
- unsigned int i;
- unsigned int j;
- for(i=0;i
- {
- for(j=0;j<500;j++) //Number of empty instructions in the embedded loop
- {
- ; //A semicolon is equivalent to executing an empty statement
- }
- }
- }
- void initial_myself() //Initialize the MCU in the first zone
- {
- beep_dr=1; //Use PNP transistor to control the buzzer, it will not sound when the output is high level.
- led_dr=0; //Turn off the independent LED light
- hc595_drive(0x00,0x00); //Turn off all LED lights driven by the other two 74HC595
- TMOD=0x01; //Set timer 0 to working mode 1
- TH0=0xfe; //Reinstall initial value (65535-500)=65035=0xfe0b
- TL0=0x0b;
- //Configure the serial port
- SCON=0x50;
- TMOD=0X21;
- /* Comment 3:
- * In order to ensure that the data received by the serial port interrupt is not lost, you must set IP = 0x10, which is equivalent to setting the serial port interrupt to the highest priority.
- * At this time, the serial port interrupt can interrupt any other interrupt service function to achieve nesting,
- */
- IP =0x10; //Set the serial port interrupt to the highest priority, required.
- TH1=TL1=-(11059200L/12/32/9600); //The serial port baud rate is 9600.
- TR1=1;
- }
- void initial_peripheral() // Initialize the periphery of the second zone
- {
- ucDigDot8=0; //Initialize all decimal points without displaying them
- ucDigDot7=0;
- ucDigDot6=0;
- ucDigDot5=0;
- ucDigDot4=0;
- ucDigDot3=0;
- ucDigDot2=0;
- ucDigDot1=0;
- EA=1; //Open the general interrupt
- ES=1; //Enable serial port interrupt
- ET0=1; //Enable timer interrupt
- TR0=1; //Start timing interrupt
- }
Previous article:Section 89: Using the internal timer of a microcontroller to make a clock
Next article:Section 87: Source code of industrial control project donated by Zheng Wenxian
Recommended ReadingLatest update time:2024-11-15 15:42
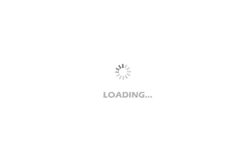
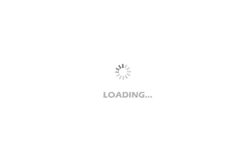
- Popular Resources
- Popular amplifiers
-
Wireless Sensor Network Technology and Applications (Edited by Mou Si, Yin Hong, and Su Xing)
-
Modern Electronic Technology Training Course (Edited by Yao Youfeng)
-
Modern arc welding power supply and its control
-
Small AC Servo Motor Control Circuit Design (by Masaru Ishijima; translated by Xue Liang and Zhu Jianjun, by Masaru Ishijima, Xue Liang, and Zhu Jianjun)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- LED chemical incompatibility test to see which chemicals LEDs can be used with
- Application of ARM9 hardware coprocessor on WinCE embedded motherboard
- What are the key points for selecting rotor flowmeter?
- LM317 high power charger circuit
- A brief analysis of Embest's application and development of embedded medical devices
- Single-phase RC protection circuit
- stm32 PVD programmable voltage monitor
- Introduction and measurement of edge trigger and level trigger of 51 single chip microcomputer
- Improved design of Linux system software shell protection technology
- What to do if the ABB robot protection device stops
- Huawei's Strategic Department Director Gai Gang: The cumulative installed base of open source Euler operating system exceeds 10 million sets
- Download from the Internet--ARM Getting Started Notes
- Learn ARM development(22)
- Learn ARM development(21)
- Learn ARM development(20)
- Learn ARM development(19)
- Learn ARM development(14)
- Learn ARM development(15)
- Analysis of the application of several common contact parts in high-voltage connectors of new energy vehicles
- Wiring harness durability test and contact voltage drop test method
- Answer questions to win JD.com card | PI InnoSwitch Product Series Learning Center
- Discussion: 5G NSA vs SA? (Reply to receive 20-100 ChipCoins)
- DSP external device connection interface HPI
- How GaN has changed the market (Part 1)
- Thermistor detection method
- How to configure GPIO interrupt for TIC6000?
- Sharing practical experience of "ESD protection circuit design" for PCB boards!!!
- Detailed explanation of the usage of volatile in DSP programming
- Can we use 3.6V connected by two diodes in series to replace 3.3V?
- C2000 Piccolo Workshop