//Since the ms interrupt time is very short, if the interrupt and display delay are not handled properly, the stopwatch will not run accurately.
#include
#define uchar unsigned char
#define uint unsigned int
uchar code table[]={0x 3f,0x06,0x5b,0x4f,0x66,0x6d,0x7d,0x07,0x7f,0x6f};
uchar code table1[]={0xbf,0x86,0xdb,0xcf,0xe6,0xed,0xfd,0x87,0xff,0xef};
uchar ms,s,m,h,count,count1;
sbit k1=P3^0;
sbit k3=P3^2;
void delay(uint z)
{
}
void displays(uchar temp)
{
}
void displayms(uchar temp)
{
}
void displaym(uchar temp)
{
}
void displayh(uchar temp)
{
}
void keyscan()
{
}
void main()
{
}
void timer0() interrupt 1
{
}
Previous article:Summary of electrical design work - MSP430G2553 study notes - 1
Next article:Design clock using timer interrupt and display using 1602 LCD
Recommended ReadingLatest update time:2024-11-15 17:00
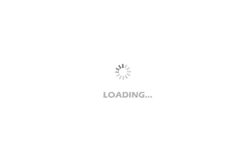
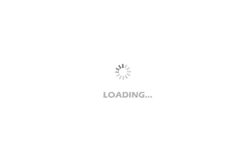
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- LED chemical incompatibility test to see which chemicals LEDs can be used with
- Application of ARM9 hardware coprocessor on WinCE embedded motherboard
- What are the key points for selecting rotor flowmeter?
- LM317 high power charger circuit
- A brief analysis of Embest's application and development of embedded medical devices
- Single-phase RC protection circuit
- stm32 PVD programmable voltage monitor
- Introduction and measurement of edge trigger and level trigger of 51 single chip microcomputer
- Improved design of Linux system software shell protection technology
- What to do if the ABB robot protection device stops
- Huawei's Strategic Department Director Gai Gang: The cumulative installed base of open source Euler operating system exceeds 10 million sets
- Download from the Internet--ARM Getting Started Notes
- Learn ARM development(22)
- Learn ARM development(21)
- Learn ARM development(20)
- Learn ARM development(19)
- Learn ARM development(14)
- Learn ARM development(15)
- Analysis of the application of several common contact parts in high-voltage connectors of new energy vehicles
- Wiring harness durability test and contact voltage drop test method
- Salt spray test chamber shows the solution to the problem
- "Playing with the board" + The first article #Running ModbusTcp on EK-TM4C1294XL
- trinamic open source KICAD stepper motor control board
- [Bluesun AB32VG1 RISC-V Evaluation Board] Settings and selections for program development
- Get a gift when you grab a building | Infineon Tmall store gives you a surprise!
- Why can't this circuit achieve bistable state?
- Power Management Guide
- Can the Unix version of MicroPython call system commands?
- These two sources contradict each other.
- 【ST NUCLEO-G071RB Review】_03_Breathing light experiment