#includetypedef unsigned char uchar; typedef unsigned char uint; //********************************************NRF24L01 port definition**************************************** sbit MISO = P0^4; sbit MOSI = P0^3; sbit SCK = P0^2; sbit CE =P0^0; sbit CSN = P0^1; sbit IRQ = P0^5; //*********************************************NRF24L01************************************* #define TX_ADR_WIDTH 5 // 5 uints TX address width #define RX_ADR_WIDTH 5 // 5 uints RX address width #define TX_PLOAD_WIDTH 2 // 2 uints TX payload #define RX_PLOAD_WIDTH 2 // 2 uints TX payload uint const TX_ADDRESS[TX_ADR_WIDTH]= {0x34,0x43,0x20,0x10,0x01}; //local address uint const RX_ADDRESS[RX_ADR_WIDTH]= {0x34,0x43,0x20,0x10,0x01}; //Receive address //*******************************************NRF24L01 register instructions******************************************************* #define READ_REG 0x00 // Read register instruction #define WRITE_REG 0x20 // Write register instruction #define RD_RX_PLOAD 0x61 // Read receive data instruction #define WR_TX_PLOAD 0xA0 // Write data to be sent instruction #define FLUSH_TX 0xE1 // Flush transmit FIFO instruction #define FLUSH_RX 0xE2 // Flush receive FIFO instruction #define REUSE_TX_PL 0xE3 // Define repeat loading data instruction #define NOP 0xFF // Reserved //****************************************SPI(nRF24L01) register address**************************************************** #define CONFIG 0x00 //Configure the receiving and sending status, CRC check mode and the receiving and sending status response method #define EN_AA 0x01 //Auto answer function setting #define EN_RXADDR 0x02 // Available channel settings #define SETUP_AW 0x03 // Set the width of the receiving and sending address #define SETUP_RETR 0x04 // Automatic resend function setting #define RF_CH 0x05 // Operating frequency setting #define RF_SETUP 0x06 // Transmit rate, power consumption function settings #define STATUS 0x07 // Status register #define OBSERVE_TX 0x08 // Send monitoring function #define CD 0x09 // Address detection #define RX_ADDR_P0 0x0A // Channel 0 receive data address #define RX_ADDR_P1 0x0B // Channel 1 receive data address #define RX_ADDR_P2 0x0C // Channel 2 receive data address #define RX_ADDR_P3 0x0D // Channel 3 receive data address #define RX_ADDR_P4 0x0E // Channel 4 receive data address #define RX_ADDR_P5 0x0F // Channel 5 receive data address #define TX_ADDR 0x10 // Transmit address register #define RX_PW_P0 0x11 // Receive data length of receiving channel 0 #define RX_PW_P1 0x12 // Receive data length of receiving channel 0 #define RX_PW_P2 0x13 // Receive data length of receiving channel 0 #define RX_PW_P3 0x14 // Receive data length of receiving channel 0 #define RX_PW_P4 0x15 // Receive data length of receiving channel 0 #define RX_PW_P5 0x16 // Receive data length of receiving channel 0 #define FIFO_STATUS 0x17 // FIFO stack entry and exit status register settings //************************************************************************************** void Delay(unsigned int s); void inerDelay_us(unsigned char n); void init_NRF24L01(void); uint SPI_RW(uint uchar); volatile SPI_Read(volatile reg); void SetRX_Mode(void); uint SPI_RW_Reg(uchar reg, uchar value); uint SPI_Read_Buf(uchar reg, uchar *pBuf, uchar uchars); uint SPI_Write_Buf(uchar reg, uchar *pBuf, uchar uchars); unsigned char nRF24L01_RxPacket(unsigned char* rx_buf); void nRF24L01_TxPacket(unsigned char * tx_buf); //********************************************Long delay********************************************* void Delay(unsigned int s) { unsigned int i; for(i=0; i 0;n--) _nop_(); } //**************************************************************************************** /*NRF24L01 initialization //***************************************************************************************/ void init_NRF24L01(void) { inerDelay_us(100); CE=0; // chip enable start chip CSN=1; //Spi disable exit SPI SCK=0; // Spi clock line init high Initialize SPI clock to low level SPI_Write_Buf(WRITE_REG + TX_ADDR, TX_ADDRESS, TX_ADR_WIDTH); // Write local address SPI_Write_Buf(WRITE_REG + RX_ADDR_P0, RX_ADDRESS, RX_ADR_WIDTH); // Write the receiving end address SPI_RW_Reg(WRITE_REG + EN_AA, 0x01); // Channel 0 automatic ACK response enabled SPI_RW_Reg(WRITE_REG + EN_RXADDR, 0x01); // The only allowed receiving address is channel 0. If multiple channels are required, please refer to Page 21 SPI_RW_Reg(WRITE_REG + RF_CH, 0); // Set the channel to work at 2.4GHZ, the send and receive must be consistent SPI_RW_Reg(WRITE_REG + RX_PW_P0, RX_PLOAD_WIDTH); //Set the received data length, this time set to 32 bytes SPI_RW_Reg(WRITE_REG + RF_SETUP, 0x07); //Set the transmission rate to 1MHZ and the transmission power to the maximum value 0dB SPI_RW_Reg(WRITE_REG + CONFIG, 0x0e); // Set the sending mode IRQ receiving and sending completion interrupt response, 16-bit CRC, master send } /**************************************************************************************************** /*Function: uint SPI_RW(uint uchar) /*Function: SPI write timing of NRF24L01 /****************************************************************************************************/ uint SPI_RW(uint uchar) { uint bit_ctr; for(bit_ctr=0;bit_ctr<8;bit_ctr++) // output 8-bit { MOSI = (uchar & 0x80); // output 'uchar', MSB to MOSI Send the highest bit of the lower eight bits of 16-bit data once until the lowest bit uchar = (uchar << 1); // shift next bit into MSB.. prepare to send next bit SCK = 1; // Set SCK high.. Simulate SPI timing uchar |= MISO; // capture current MISO bit SCK = 0; // ..then set SCK low again Receive ACK? } return(uchar); // return read uchar return data and response? ? } /**************************************************************************************************** /*Function: uchar SPI_Read(uchar reg) /*Function: SPI timing of NRF24L01 /****************************************************************************************************/ fly SPI_Read(fly reg) { fly reg_val; CSN = 0; // CSN low, initialize SPI communication... SPI_RW(reg); // Select register to read from.. reg_val = SPI_RW(0); // ..then read registervalue CSN = 1; // CSN high, terminate SPI communication return(reg_val); // return register value }[page] /****************************************************************************************************/ /*Function: NRF24L01 read and write register function writes one data at a time /****************************************************************************************************/ uint SPI_RW_Reg(uchar reg, uchar value) { wint state; CSN = 0; // CSN low, init SPI transaction starts SPI bus status = SPI_RW(reg); // select register Send register address to select register and select read or write SPI_RW(value); // ..and write value to it.. Write data to the register CSN = 1; // CSN high again return(status); // return nRF24L01 status uchar } /****************************************************************************************************/ /* Function: uint SPI_Read_Buf(uchar reg, uchar *pBuf, uchar uchars) /*Function: used to read data, reg: register address, pBuf: address of data to be read, uchars: number of data to be read /****************************************************************************************************/ uint SPI_Read_Buf(uchar reg, uchar *pBuf, uchar uchars) { uint status,uchar_ctr; CSN = 0; // Set CSN low, init SPI tranaction status = SPI_RW(reg); // Select register to write to and read status uchar for(uchar_ctr=0;uchar_ctr(TxBuf+4)) t=TxBuf; SetRX_Mode(); //Set to receive mode while(!nRF24L01_RxPacket(&RxBuf)); //Wait for receiving data P2=RxBuf; Delay(20000); } }
Previous article:Three-axis angle detection (tilt sensor MMA7455 (accelerometer))
Next article:Assembler program to implement square operation
Recommended ReadingLatest update time:2024-11-16 14:40
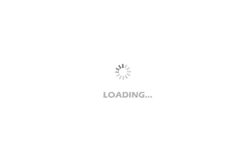
- Popular Resources
- Popular amplifiers
-
Mission-oriented wireless communications for cooperative sensing in intelligent unmanned systems
-
Principles of Digital Communications (Gallagher)
-
Build the Internet of Things with Arduino
-
Electronic competition thesis documents based on MSP430 microcontroller design + source code and some hardware PCB files
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- PCB Design Issues
- Professor Yang's book is here, a five-volume collection of "New Concept Analog Circuits" series by Yang Jianguo of Xi'an Jiaotong University
- 2019, what is your New Year's resolution?
- How does MCU implement part of the code running in RAM?
- STM32SPI interrupt can only accept the first two bytes each time
- BlueNrg-1 and Raspberry Pi communication abnormality
- 28335 Sampling Problems
- Common problems and causes of switching power supplies
- DM6467T calls TI's C6Accel library
- Rules for sending multiple instructions inside CAN protocol devices