#include "reg52.h"
#include "intrins.h"
#include "math.h"
#include "stdio.h"
sbit BT_SND =P1^5;
sbit BT_REC =P1^6;
sbit LED =P1^7;
bit LED_flage=1;
//MCU IO port simulates 232 serial port communication program
//C program using two methods to occupy timer 0
#define MODE_QUICK
#define F_TM F0
#define TIMER0_ENABLE TL0=TH0; TR0=1;
#define TIMER0_DISABLE TR0=0;
sbit ACC0= ACC^0;
sbit ACC1= ACC^1;
sbit ACC2= ACC^2;
sbit ACC3= ACC^3;
sbit ACC4= ACC^4;
sbit ACC5= ACC^5;
sbit ACC6= ACC^6;
sbit ACC7= ACC^7;
void IntTimer0() interrupt 1
{
F_TM=1;
}
//Send a character
void PSendChar(unsigned char inch)
{
#ifdef MODE_QUICK
ACC=inch;
F_TM=0;
BT_SND=0; //start bit
TIMER0_ENABLE; //Start
while (!F_TM);
BT_SND=ACC0; //Send the low bit first
F_TM=0;
while(!F_TM);
BT_SND=ACC1;
F_TM=0;
while(!F_TM);
BT_SND=ACC2;
F_TM=0;
while(!F_TM) );
BT_SND=ACC3;
F_TM=0;
while(!F_TM);
BT_SND=ACC4;
F_TM=0;
while(!F_TM);
BT_SND=ACC5;
F_TM=0;
while(!F_TM);
BT_SND=ACC6;
F_TM= 0;
while(!F_TM);
BT_SND=ACC7;
F_TM=0;
while(!F_TM);
BT_SND=1;
F_TM=0;
while(!F_TM);
TIMER0_DISABLE; //Stop timer
#else
unsigned char ii;
ii=0;
F_TM=0;
BT_SND= 0; //start bit
TIMER0_ENABLE; //Start
while(!F_TM);
while(ii<8)
{
if(inch&1)
{
BT_SND=1;
}
else
{
BT_SND=0;
}
F_TM=0;
while(!F_TM) ;
ii++;
inch>>=1;
}
BT_SND=1;
F_TM=0;
while(!F_TM);
#endif
TIMER0_DISABLE; //Stop timer
}
//Receive a character
unsigned char PGetChar()
{
#ifdef MODE_QUICK
TIMER0_ENABLE;
F_TM =0;
while(!F_TM); //Wait until the start bit
ACC0=BT_REC;
TL0=TH0;
F_TM=0;
while(!F_TM);
ACC1=BT_REC;
F_TM=0;
while(!F_TM);
ACC2=BT_REC;
F_TM =0;
while(!F_TM);
ACC3=BT_REC;
F_TM=0;
while(!F_TM);
ACC4=BT_REC;
F_TM=0;
while(!F_TM);
ACC5=BT_REC;
F_TM=0;
while(!F_TM) ;
ACC6=BT_REC;
F_TM=0;
while(!F_TM);
ACC7=BT_REC;
F_TM=0;
while(!F_TM)
{
if(BT_REC)
{
break;
}
}
TIMER0_DISABLE; //Stop timer
return ACC;
#else
unsigned char rch,ii;
TIMER0_ENABLE;
F_TM=0;
ii=0;
rch=0;
while(!F_TM); //Wait for the start
bitwhile(ii<8)
{
rch>>=1;
if(BT_REC)
{
rch|=0x80;
}
ii++;
F_TM=0;
while(!F_TM);
}
F_TM=0;
while(!F_TM)
{
if(BT_REC)
{
break;
}
}
TIMER0_DISABLE; //Stop timer
return rch;
#endif
}
//Check if there is a start
bit StartBitOn()
{
return (BT_REC==0);
}
//Timer 1 initialization
void Time1_Init(void)
{
TMOD=0x22; //Timer 1 is working mode 2 (8-bit automatic reload), 0 is mode 2 (8-bit automatic reload)
PCON=00;
TR0=0; //Start using it when sending or receiving
TF0=0;
TH0=(256-96); //9600bps means the timer is executed in 1000000/9600=104.167 microseconds//104.167*11.0592/12= 96
TL0=TH0;
ET0=1;
EA=1;
}
//Send string
void Send_Char(char *byte)
{
int i=0;
for(i=0;*(byte+i)!='';i++)
{
PSendChar(*(byte+i));
}
}
//void delay(int x)
//{
// int a,b;
// for(a=x;a>0;a--)
// for(b=10;b>0;b--);
//}
//void main()
//{
// unsigned char gch;
// Time1_Init();
// LED=0;
// // Send_Char("S00.0C00.0%E00.0C00.0%L00000lx");
// while(1)
// {
//
// PSendChar('1');
// delay(1000);
//// if(StartBitOn())
//// {
//// gch=PGetChar();
//// if(gch=='1')
//// {
//// LED=LED_flage;
//// delay(1000);
//// LED_flage=~LED_flage;
//// }
////
//// }
//
// }
//
//}
Previous article:51 MCU + 315M wireless RF module receiving program
Next article:Experience in designing and debugging an "electronic calendar" based on the DS1302 digital chip
Recommended ReadingLatest update time:2024-11-16 13:40
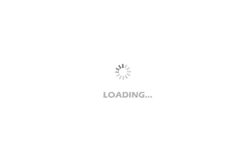
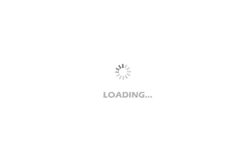
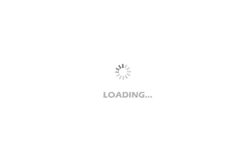
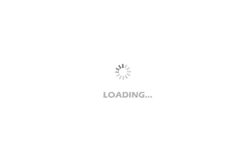
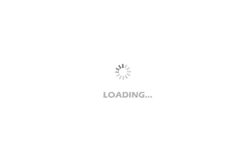
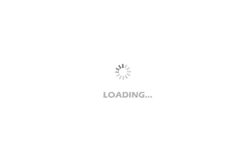
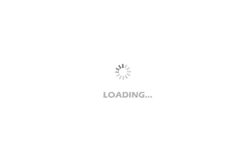
- Popular Resources
- Popular amplifiers
-
Wireless Sensor Network Technology and Applications (Edited by Mou Si, Yin Hong, and Su Xing)
-
Modern Electronic Technology Training Course (Edited by Yao Youfeng)
-
Modern arc welding power supply and its control
-
Small AC Servo Motor Control Circuit Design (by Masaru Ishijima; translated by Xue Liang and Zhu Jianjun, by Masaru Ishijima, Xue Liang, and Zhu Jianjun)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- 【Silicon Labs BG22-EK4108A Bluetooth Development Review】I. Hardware Appreciation and Development Environment Introduction
- [ESP32-S2-Kaluga-1 Review] 4. LCD example compilation pitfalls
- HuaDa MCU FLASH operation instructions and precautions
- I am working on a product for traffic security equipment recently. The project has high safety requirements and heavy tasks. I am having a headache recently...
- Several issues on key-controlled 8X8LED dot matrix screen displaying graphics program
- FAQ: PolarFire SoC FPGA Secure Boot | Microchip Security Solutions Seminar Series 12
- Some Problems on Measuring AC Current with Current Transformer
- Simulation of staying up late is harmful to health
- [RVB2601 Creative Application Development] + RTC Clock Display Experiment
- From traditional substation to smart substation