The board is lazy, or it is called making full use of the pull-up function of the IO port, and no common pull-up resistor is added here. When programming, just enable the pull-up of the IO~ Look at the interface below to know that KEY0 is connected to PA13 of STM32!
CZZ once told me in a dream that any IO of STM32 can be used as an external interrupt input. Wow, it’s super powerful!
The general procedure steps are as follows:
1. System initialization, such as system clock initialization, so that it enters 72MHZ main frequency;
2. GPIO configuration, be sure to turn on the AFIO clock when turning on the GPIO clock.
3. EXTI configuration, here configure which pin to select as the interrupt pin.
4. NVIC configuration, this is also an extra part compared to the microcontroller. We must enable the corresponding channel in NVIC and set the priority level.
5. Use while(1) to perform an infinite loop, and write in the interrupt program how to handle the interrupt when it occurs.
Follow the above method step by step to realize the function~
First, define the relevant structure.
GPIO_InitTypeDef GPIO_InitStructure;
EXTI_InitTypeDef EXTI_InitStructure;
NVIC_InitTypeDef NVIC_InitStructure;
The second step is to configure IO and its functions.
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_8; //Here is the code to configure the LED light for easy observation!
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_Init(GPIOA, &GPIO_InitStructure);
GPIO_WriteBit(GPIOA,GPIO_Pin_8,Bit_SET); //Turn off the LED immediately after power on
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_13; //Configure PA13 pull-up input
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IPU; //Pull-up input
GPIO_Init(GPIOA, &GPIO_InitStructure);
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA|RCC_APB2Periph_AFIO,ENABLE); //Make sure to turn on the GPIOA and AFIO clocks!!!
The third step is to configure external interrupts, which is equivalent to the interrupt settings of the microcontroller.
GPIO_EXTILineConfig(GPIO_PortSourceGPIOA,GPIO_PinSource13); //Configure pin 13 of port A as interrupt
EXTI_ClearITPendingBit(EXTI_Line13); //Clear interrupt, it seems that there is no problem without adding it, but it is safe!
EXTI_InitStructure.EXTI_Mode = EXTI_Mode_Interrupt; //External interrupt
EXTI_InitStructure.EXTI_Trigger = EXTI_Trigger_Falling; //Falling edge trigger
EXTI_InitStructure.EXTI_Line = EXTI_Line13;
EXTI_InitStructure.EXTI_LineCmd = ENABLE;
EXTI_Init(&EXTI_InitStructure);
The fourth step is to configure NVIDIA, which is also a feature of STM32.
NVIC_PriorityGroupConfig(NVIC_PriorityGroup_0);
NVIC_InitStructure.NVIC_IRQChannel = EXTI15_10_IRQn; //Channel 13 belongs to 15-10, they are shared!
NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 0; //Preemptive priority 0
NVIC_InitStructure.NVIC_IRQChannelSubPriority = 0; //Sub-priority 0
NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE;
NVIC_Init(&NVIC_InitStructure);
while(1); //wait for interrupt to occur
The fifth step is to write the interrupt processing function ISR and add the following code in stm32f10x_it.c.
void EXTI15_10_IRQHandler(void)
{
if ( EXTI_GetITStatus(EXTI_Line13) == SET) //Judge whether it is the interrupt of pin 13
{
EXTI_ClearITPendingBit(EXTI_Line13); //Clear the interrupt flag!
GPIO_WriteBit(GPIOA,GPIO_Pin_8,Bit_RESET); //Turn on the LED
}
}
OK, the program is complete. Let’s test it!
Previous article:Causes and solutions for Flash download failed-Cortex-M3
Next article:Design of comprehensive monitoring system for urban rail energy-feed power supply system based on ARMCortex-A8
Recommended ReadingLatest update time:2024-11-16 14:28
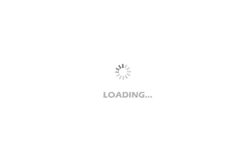
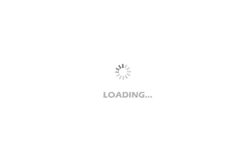
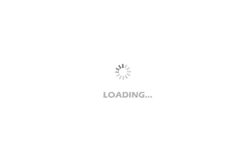
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- The difference between various debugging interfaces (SWD, JTAG, Jlink, Ulink, STlink)
- [SAMR21 new gameplay] 35. HCSR04 ultrasonic sensor
- The evaluation plan for the current probe and signal generator winners is open! The instruments have been distributed, and we look forward to the sharing of the 6 little ones
- How to use stm to make lcd12864 display
- Thank you + my son, my daughter, and my wife
- How to set breakpoints when debugging a program in keil4?
- 【Qinheng Trial】3. System clock and TIMER0
- [SAMR21 New Gameplay] 32. CPU-related functions
- C2000 Power-on Boot Mode Analysis
- EEWORLD University Hall----Electromagnetic Compatibility Principles and Design