Below is the source code:
/**
*********************************************************************************************
* @file main.c
* @author xr
* @date March 18, 2014 20:00:03
* @note Parameters of the stepper motor: Reduction ratio: 1:64 Step angle: 5.625/64 Starting frequency: >=550 Minimum starting time: 1.08ms
* @brief Serial communication control of stepper motor Microcontroller STC89C52RC MCU Crystal oscillator 11.0592MHZ
*************************************************************************************************
*/
#include
typedef unsigned int uint;
typedef unsigned char uchar;
typedef unsigned long ulong;
//Stepper motor eight-beat mode phase value encoding A-AB-B-BC-C-CD-D-DA
uchar code table[] = {
0xE, 0xC, 0xD, 0x9, 0xB, 0x3, 0x7, 0x6
};
uint tmp = 0;
bit dir = 0;
ulong beats = 0; //Number of beats
uchar angle = 0; //Number of turns
void timer0_Config();
void motor_Config(ulong angle);
void Uart_Config(uint baud);
void main()
{
timer0_Config();//The startup time is set to 2ms
Uart_Config(9600);
while (1)
{
switch (angle)
{
case 2:
{
motor_Config(2*360);
angle = 0;
break;
}
case 3:
{
motor_Config(3*360);
angle = 0;
break;
}
case 4:
{
motor_Config(4*360);
angle = 0;
break;
}
case 5:
{
motor_Config(5*360);
angle = 0 ;
break;
}
case 6:
{
motor_Config(6*360);
angle = 0;
break;
}
case 7:
{
motor_Config(7*360);
angle = 0;
break;
}
case 8:
{
motor_Config(8*360) ;
angle = 0;
break;
}
case 9:
{
P1 |= 0x0F;
beats = 0;
angle = 0;
break;
}
default:
break;
}
}
}[page]
/**
* @brief Stepper motor configuration (determine the beats of the rotor according to the number of turns of the output shaft)
* @param None
* @retval None
*/
void motor_Config(ulong angle)
{
//Disable interrupts before calculation
EA = 0;
beats = (angle*64*8*8)/360;
EA = 1;
}
/**
* @brief Timer T0 timing configuration
* @param uint xms
* @retval None
*/
void timer0_Config()
{
TMOD &= 0xF0; // Clear T0 control bit
TMOD |= 0x01; // T1 mode 1
TH0 = 0xF8;
TL0 = 0xCC; // 2ms
TR0 = 1;
ET0 = 1;
EA = 1;
}
/**
* @brief Serial communication configuration
* @param None
* @retval None
*/
void Uart_Config(uint baud)
{
SCON |= 0x50; //SM0-SM2 REN TB8 RB8 TI RI
TMOD &= 0x0F; //Clear T1 control bit
TMOD |= 0x20; //Set timer T1 to eight-bit auto-reload mode, count and set serial port baud rate
TH1 = 256-(11059200/12/32/baud); //Baud rate = 2^SMOD/32 * T1(overflow rate) T1(overflow rate) = 1/[(256-X)*(12/11059200)]
TL1 = TH1;
TR1 = 1;
ET1 = 0; //Disable T1 timer interrupt
ES = 1; //Enable serial port interrupt
EA = 1; //Enable total interrupt
}
/**
* @brief T0 interrupt service subroutine
* @param None
* @retval None
*/
void timer0_Int() interrupt 1 using 3
{
static uchar step = 0;
uchar buf = 0;//Store phase value
TH0 = 0xF8;
TL0 = 0xCC;
if (beats)
{
if (!dir)
{
buf = (P1 & 0xF0);//buf temporarily stores the high byte of P1, clears the low byte
buf |= table[step++];//Store the phase value in buf
step &= 0x07;//And the method to achieve 8 zeroing
P1 = buf;
beats--;
}
else
{
buf = (P1 & 0xF0);
buf |= table[step--];
if (step <= 0)
{
step = 7;
}
P1 = buf;
beats--;
}
}
else
{
P1 |= 0x0F; // Turn off all phases, that is, the stepper motor stops rotating
}
}
/**
* @brief Serial port interrupt service subroutine
* @param None
* @retval None
*/
void Uart_Int() interrupt 4 using 0
{
if (RI)
{
RI = 0;
if (SBUF > 1)
{
angle = SBUF;
}
else
{
dir = SBUF;
}
}
}
Previous article:Three ways to implement dynamic scanning of digital tubes
Next article:MCU matrix key timer debounce program source code
Recommended ReadingLatest update time:2024-11-16 14:35
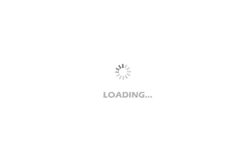
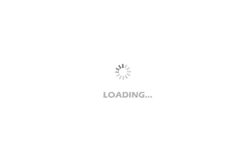
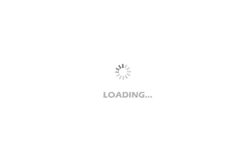
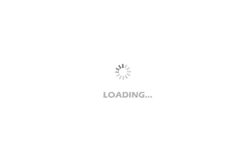
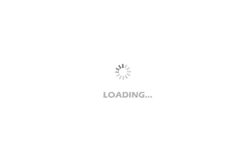
- Popular Resources
- Popular amplifiers
-
Wireless Sensor Network Technology and Applications (Edited by Mou Si, Yin Hong, and Su Xing)
-
Modern Electronic Technology Training Course (Edited by Yao Youfeng)
-
Modern arc welding power supply and its control
-
Small AC Servo Motor Control Circuit Design (by Masaru Ishijima; translated by Xue Liang and Zhu Jianjun, by Masaru Ishijima, Xue Liang, and Zhu Jianjun)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- AVR MCU nrf24l01 driver
- Circuit Design Considerations
- PWM output of MSP430 library timer TA
-
[NXP Rapid IoT Review] +
NXP Rapid IoT, a simplest cloud server prototype - EEWORLD University ---- Computer Control Technology
- Please advise, why do you need to remove the motherboard before burning the flash?
- Antenna packaging issues
- DA output waveform test
- TI High-Speed Signal Conditioning Product Selection Guide
- An important representative enterprise in 70 domestic chip sub-sectors!