With the rapid development of information technology with computer technology, communication technology and software technology as the core, embedded systems have been widely used in various industries, greatly promoting the industry's penetrating applications.
Embedded system is a "special computer system centered on application, based on computer technology, with tailorable software and hardware to meet the strict requirements of application system on function, reliability, cost, volume and power consumption". It consists of two parts: embedded hardware and embedded software. Embedded software includes embedded operating system and embedded application software. Microsoft's desktop operating system is already familiar and used by people, and the embedded operating system Windows CE.net is also becoming increasingly popular. Windows CE.net is a powerful, compact, efficient and scalable 32-bit embedded operating system launched by Microsoft, mainly for various embedded systems and products. The multi-threaded, multi-tasking and fully preemptive features of this system are designed for various hardware systems with strict resource constraints. In order to connect the operating system and hardware devices, it is very important to connect the drivers of hardware and software.
The following mainly analyzes the Samsung ARM9 core chip S3C2410, introduces the method of developing low-level device drivers under the Windows CE.net system, and provides an example of I2C communication.
1 Introduction to I2C communication protocol and S3C2410 chip
|
The I2C bus complies with the synchronous serial transmission protocol, that is, each bit is sent serially (one bit after another), and the clock line indicates the time to read the data line. Each data packet is preceded by an address to indicate which device receives the data.
S3C2410 is a 16/32-bit RISC microprocessor based on ARM920T, mainly used in handheld devices, with high cost performance, low power consumption and other characteristics, and is also one of the processors for embedded development boards that appear more frequently on the market. The chip has 16 KB of instruction and data buffers, a memory management unit (MMU), LCD controller, 3 serial ports, 4-way DMA, 4 clock timers, 8-way 10-bit A/D conversion; supports I2C, I2S, SPI, master-slave USB and other interfaces as well as SD/MMC cards.
The I2C bus of the S3C2410 microprocessor can be in the following four modes: master receiving mode, master transmitting mode, slave receiving mode and slave transmitting mode. The processor's operation on I2C is mainly to read/write the following registers:
- IIC control register, IICCON (physical address 0X54000000, virtual address after memory mapping);
- IIC control/status register, IICSTAT (physical address 0X54000004);
- IIC data register, IICDS (physical address 0X54000008);
- IIC address register, IICADD (physical address 0X5400000C).
This design mainly uses the CPU working in master mode to communicate with the I2C interface device below.
2 Windows CE system driver features
There are two models of Windows CE.net drivers: native device drivers and stream interface drivers. Native device drivers are suitable for integration into devices based on the Windows CE.net platform. These device drivers are necessary for some hardware and are created by original equipment manufacturers to drive devices such as keyboards, touch screens, audio devices, etc. They are often not replaced after the device is sold. For example, general LED drivers, power drivers, keyboard drivers, and display drivers are all native device drivers. For native device drivers, Platform Builder provides some driver samples to facilitate developers to quickly develop their own drivers. When the Win CE system starts, the local device driver will be loaded into the system's memory. The development of local drivers is divided into layered drivers and monolithic drivers. Layered drivers use the upper layer provided by Microsoft to communicate with applications, called the module driver layer MDD (Model Device Driver). The MDD layer communicates with applications through the device driver interface DDI (Device Driver Interface). Driver development usually does not modify the MDD layer, but mainly focuses on the lower layer related to specific hardware, the platform-dependent device driver layer PDD (Platform Dependent Driver). The PDD layer directly manages the hardware through the device driver service interface (Device Driver Service Provider Interface). Stream interface device drivers (installable boot programs) can be provided by third-party manufacturers to support devices added to the system. Device drivers under Windows CE work at the same protection level as applications. When the system starts, most drivers are loaded by the device management process (DEVICE.EXE), and all these drivers will share the same process address space.
3 I2C bus bottom
Layer-driven design
The I2C bus driver is a real driver placed in the lower layer of the Windows CE operating system kernel, located in the OEM Adaptation Layer (OAL) layer.
3.1 Initialize I2C interrupt and write ISR routine
I2C communication is performed by operating I2C registers. In I2C communication, the four registers introduced above are mainly read and written. By reading and writing the command status words in these registers, the behavior of the I2C bus can be detected and controlled. Under Windows CE.net, first add the macro definition of the I2C interrupt number in the file oalintr.h:
#defineSYSINTR_I2C(SYSINTR_FIRMWARE+19)
Then add the initialization, disabling and resetting of I2C interrupt in the file cfw.c. The specific code is as follows:
Add in OEMInterruptEnable function
case SYSINTR_IIC:
s2410INT->rSRCPND=BIT_IIC;
if (s2410INT->rINTPND & BIT_IIC) s2410INT->rINTPND = BIT_IIC;
s2410INT->rINTMSK&=
|
break;
Add in OEMInterruptDisable function
case SYSINTR_IIC:
s2410INT->rINTMSK|= BIT_IIC;
break;
Add an ISR program in the armint.c file to return the defined interrupt number after processing the interrupt. The specific code is as follows:
Add in OEMInterruptHandler function
else if (IntPendVal == INTSRC_IIC) {
s2410INT->rSRCPND= BIT_IIC; /* Clear interrupt*/
if (s2410INT->rINTPND & BIT_IIC) s2410INT->rINTPND= BIT_IIC;
s2410INT->rINTMSK|= BIT_IIC; /* I2C interrupt disabled*/
return (SYSINTR_RTC_ALARM);
}[page]
3.2 Writing a stream driver
The I2C bus driver uses the standard form of Win CE stream driver. In the IIC_Init function, firstly, the physical address of the chip for I2C is linked to the virtual memory space of the operating system through the functions VirtualAlloc() and VirtualCopy(). The operation of the virtual address space is equivalent to the operation of the physical address of the chip. The address mapping code is as follows:
reg = (PVOID)VirtualAlloc(0, sz, MEM_RESERVE, PAGE_NOACCESS);
if (reg) {
if (!VirtualCopy(reg, addr, sz, PAGE_READWRITE | PAGE_NOCACHE )) {
RETAILMSG( DEBUGMODE,( TEXT( "Initializing interrupt \\
\\
" ) ) );
VirtualFree(reg, sz, MEM_RELEASE);
reg = NULL;
}
}
Among them, sz is the length of the application, and addr is the mapping address of the actual physical address of the virtual address space in Win CE.
Then operate the applied virtual address, install the stream driver model in Windows to write the driver, mainly including the writing of the following functions.
In the
IIC_Init()
function, the main purpose is to initialize I2C. The main statements are as follows:
v_pIICregs = ( volatile IICreg *)IIC_RegAlloc((PVOID)
IIC_BASE, sizeof(IICreg)); v_pIOPregs = ( volatile IOPreg *)IOP_RegAlloc((PVOID)IOP_BASE, sizeof(IOPreg));
v_pIOPregs->rGPEUP|= 0xc000;
v_pIOPregs->rGPECON |= 0xa00000;
v_pIICregs->rIICCON = (1<<7) | (0<<6) | (1<<5) | (0xf);
v_pIICregs->rIICADD= 0x10;
v_pIICregs->rIICSTAT = 0x10;
VirtualFree((PVOID )v_pIOPregs,sizeof( IOPreg ),MEM_RELEASE );
v_pIOPregs = NULL;
if ( !S
startDispatchThread( pIIcHead) )
{ IIC_Deinit( pIIcHead );return ( NULL );} In the StartDispatchThread() function, the main tasks are to create threads, associate events and interrupts. The main statements are as follows:
InterruptInitialize( 36,pIicHead->hIicEvent,NULL,0 );//Associate time and interrupt
CreateThread( NULL,0,IicDispatchThread,pIicHead,0,NULL );//Create thread waiting time
In the IicDispatchThread() function, the main task is to wait for the interrupt to occur, and then execute: WaitReturn = WaitForSingleObject( pIicHead->hIicEvent,INFINITE );
IicEventHandler( pIicHead );//Event processing function
InterruptDone( 36 );
Finally, in the functions IIC_Open, IIC_Read, and IIC_Write, each register is operated to assign data and obtain I
|
4 I2C driver packaging and adding to Windows CE
Through the above work, a DLL function can be compiled, but it cannot be called a stream interface driver. Because its interface function has not been exported, it is necessary to tell the linker what kind of function needs to be exported. To do this, you need to create your own def file. You can use Notepad to create one and name it mydrive.Def:
LIBRARY MyDriver
EXPORTS
IIC_Close
IIC_Deinit
IIC_Init
IIC_IOControl
IIC_Open
IIC_PowerDown
IIC_PowerUp
IIC_Read
IIC_Seek
IIC_Write
Then use Notepad to write a registry file and name it mydrive.reg:
[HKEY_LOCAL_MACHINE\\Drivers\\BuiltIn\\STRINGS]
"Index"=dword:1
"Prefix"="IIC"
"Dll"="MyDriver.dll"
"Order"=dword:0
Finally, write your own CEC file. The main thing is to add a Build Method, whose task is to copy the registry to the Win CE system directory. Add a Bib File, whose main function is to add the compiled mydrive.dll file to the system kernel. Save the written CEC file. Open Platform Builder, open the "File" menu, and add the CEC feature you just wrote to the system options. When building the system, add your own CEC feature, and you can include the I2C driver you just wrote.
The above introduces the driver structure of Win CE and gives some source code of I2C driver based on Win CE. The experiment proves that the design is feasible.
References
[1]. ARM920T datasheet http://www.dzsc.com/datasheet/ARM920T_139814.html.
[2]. RISC datasheet http://www.dzsc.com/datasheet/RISC_1189725.html.
[3]. Chen Xiangqun, et al. Windows CE.NET System Analysis and Experimental Tutorial. Beijing: Machinery Industry Press, 2003
[4]. Zhou Yulin, et al. Windows CE.net kernel customization and application development. Beijing: Electronic Industry Press, 2005
[5]. Microsoft. Windows CE Device Driver Development Guide. Beijing: Beijing Hope Electronics Press, 1999
Previous article:Design of automotive electronic control system unit based on ARM
Next article:How to deal with the Middle-Endian problem of floating point numbers in the ARM system
Recommended ReadingLatest update time:2024-11-16 22:28
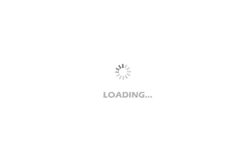
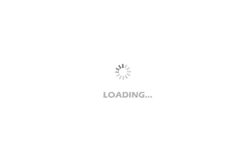
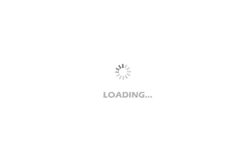
- Popular Resources
- Popular amplifiers
-
Siemens Motion Control Technology and Engineering Applications (Tongxue, edited by Wu Xiaojun)
-
MCU C language programming and Proteus simulation technology (Xu Aijun)
-
100 Examples of Microcontroller C Language Applications (with CD-ROM, 3rd Edition) (Wang Huiliang, Wang Dongfeng, Dong Guanqiang)
-
Principles and Applications of Single Chip Microcomputers 3rd Edition (Zhang Yigang)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- [Support eSports] Invite your friends to join the 19 National Competition Exchange Group and share a thousand-yuan cash gift!
- What is a hysteresis comparator?
- Eliminate distractions
- 【Maturity Cycle】Maturity Cycle of Automotive Electronics Engineers
- A collection of ESP32 experience posts. Netizens who are learning ESP32 can take a look.
- 883 Q What does Q stand for in the device identification?
- Received the prize, I feel the delivery speed is very fast now
- LM358 op amp problem, please give me some advice
- How to Measure Circuit Current Using a Shunt Resistor
- TI's ultra-low power solution gives birth to TWS true wireless