/*===Chip:STC12C5A32S2==================================*/
/*===Software:Keil 4 C51=======================================*/
/*===Author:Liang Peng==============================================*/
/*===Organization:College of Physics and Electronic Engineering,Guangxi University for Nationalities,07 Automation==========================*/
/*===Date:May 26, 2010==================================*/
/*===Version:1.0==========================================*/
/*===Statement: This program is an original work and is only for learning and communication. It may not be used for commercial purposes. If you need to
reprint it, please indicate the source. Thank you for your cooperation! ===============================*/
///*----------File name: Traffic_light.c-------------*/
#include#include #include<LCD1602.H> #define ON 0 #define OFF 1 #define yellow_time 3 //=========================== sbit MODE_KEY=P3^3; //Mode key for adjusting time sbit TIME_ADD=P3^4; //button to increase the time by 5 seconds sbit TIME_DEC=P3^5; //button to reduce the time by 5 seconds sbit LOCK_KEY=P3^6; //Lock/Restore Traffic Button sbit North_turn_R= P0^0; //Red light for north-south left turn sbit North_turn_G= P0^1; //North-South left turn green light sbit North_Y = P0^2; //North and south yellow lights sbit North_R = P0^3; //Red light for north-south straight driving sbit North_G = P1^0; //North-South straight green light sbit East_turn_R = P1^1; //Red light for turning left from east to west sbit East_turn_G = P1^2; //Green light for east-west left turn sbit East_Y = P1^3; //East and West yellow lights sbit East_R = P1^4; //Red light for east-west driving sbit East_G = P1^5; //Green light for east-west straight ahead //=========================== void delay_ms(uint); //delay sub-function void init(); //Program timer interrupt external interrupt initialization void system_on(); //The system is powered on, and the two yellow lights flash for 3 seconds. void display_lcd(); //LCD display sub-function void display_light(); //LED light display void judge(); //judgment function for each state switching void yellow_light(); //Sub-function that flashes the yellow light for 3 seconds void GoStraight(); //Sub-function for the straight path void TurnLeft(); //Sub-function of the left turn //=========================== bit direction; //0->North-South direction; 1->East-West direction; bit flash_flags; // flashing status variable bit change_flags; //Timeout variable bit Lock_flags; //0->Traffic is not blocked; 1->Traffic is blocked uint ovf_cnt; //Timer interrupt count variable uchar mode_flags; //key changes variables uchar run_flags; //Runtime status variables uchar time_temp; //timing time cache uchar north_green_time=10; //initialize the north-south straight green light time uchar east_green_time=10; //initialize the green light time for east-west straight travel /*The left turn green light time is equal to the passing green light time*/ /*-Red light time is green light time plus yellow light time-*/ /*------The yellow light time is fixed at 3 seconds-----*/ //uchar north_red_time; //uchar east_red_time; //uchar north_turn_time; //uchar east_turn_time; //=========================== //When the red light switches to green, the yellow light does not need to flash //When the green light switches to the red light, the yellow light needs to flash //When the yellow light flashes, the green light does not go out //Red light time = green light time + yellow light time //Left turn time = straight green light time //============================================ //******************************************** void main() { init(); //Timed interrupt external interrupt initialization LCD_init(); //LCD1602 initialization /*When running for the first time, the yellow light flashes for three seconds*/ LCD_str(1,1,"System on ..."); time_temp=yellow_time; system_on(); //Two yellow lights flashing sub-function while(1) { judge(); /*LED light display*/ display_light(); /*1602 display*/ display_lcd(); } } //********************************************* //============================================= void init() { TMOD|=0x02; //Timer 0 mode 2, automatic reload TH0=5;TL0=5; //250us interruption ET0=1; //Enable timer 0 interrupt TCON|=0x01; //External interrupt 0 falling edge trigger EX0=1; //Enable external interrupt 0 EA=1; //Enable global interrupt TR0=1; //Start timer 0 } //=========================== void system_on() //After power on, the yellow light at the east, west, south, and north intersections flashes for 3 seconds { do{ if(flash_flags) {North_Y=OFF;East_Y=OFF;} else {North_Y=ON; East_Y=ON; } }while(!change_flags); //The first time is not completed and the status is not changed } //=========================== void display_lcd() { if((!mode_flags)&&(!Lock_flags)){//Normal display status switch(run_flags){ case 0:case 2:case 4:case 6:case 8: //yellow light flashing state if(!direction){ if(flash_flags) {LCD_str(1,1," ");} else {LCD_str(1,1,"Status Change...");} }else{ if(flash_flags) {LCD_str(1,1," ");} else {LCD_str(1,1,"Status Change...");} } break; case 1: case 5: //straight state if(!direction) {LCD_str(1,1,"North GoStraight");} else {LCD_str(1,1,"East GoStraight");} break; case 3: case 7: //Left turn state if(!direction) {LCD_str(1,1,"North Turn Left ");} else {LCD_str(1,1,"East Turn Left ");} break; } LCD_str(2,6," "); //Clear the second line } if((mode_flags==1)&&(!Lock_flags)){//Change the north-south direct time status LCD_str(1,1,"North Green Time"); LCD_str(2,6,"To: "); LCD_int_(north_green_time); LCD_str_("S "); } if((mode_flags==2)&&(!Lock_flags)){//Change the state of the east-west direct time LCD_str(1,1,"East Green Time"); LCD_str(2,6,"To: "); LCD_int_(east_green_time); LCD_str_("S "); } if(!Lock_flags){/*Display the remaining time of the current state*/ LCD_int(2,1,time_temp); LCD_char(' '); LCD_str(2,4,"S ") } if(Lock_flags){ //Display when traffic is blocked if(flash_flags){ LCD_str(1,1," "); LCD_str(2,1," "); }else{ LCD_str(1,1," Please Wait... "); LCD_str(2,1," Locking... "); } } } //=========================== void display_light() { if(!Lock_flags){//When traffic is not blocked, the traffic lights are displayed according to the following procedures /*The following is determined by the run_flags status to determine whether the light is on or off*/ switch(run_flags){ case 0:case 2:case 4:case 6:case 8: yellow_light(); break; case 1:case 5: GoStraight(); break; case 3:case 7: TurnLeft(); break; default: run_flags=1; } /*Red light on and off*/ if(!direction){ //When traveling north and south, all green lights on the east and west sides are off and all red lights are on East_R=ON; East_turn_R=ON; East_G=OFF; East_turn_G=OFF; East_Y=OFF; }else{ //When traveling east-west, all green lights on the north and south sides are off and all red lights are on North_R=ON; North_turn_R=ON; North_G=OFF;North_turn_G=OFF; North_Y=OFF; } }else{//When traffic is blocked, all red lights are on North_R=ON; North_turn_R=ON; North_G=OFF;North_turn_G=OFF; North_Y=OFF;East_Y=OFF; East_R=ON; East_turn_R=ON; East_G=OFF; East_turn_G=OFF; } } //=========================== void yellow_light() /*Sub-function of yellow light flashing*/ { if(!direction){ //North_G=OFF; //Green light for north-south driving is off //North_turn_G=OFF; if(flash_flags) {North_Y=OFF;} else {North_Y=ON; } }else{ //East_G=OFF; //East_turn_G=OFF; if(flash_flags) {East_Y=OFF; } else {East_Y=ON; } } } //=========================== void GoStraight(){ /*Subfunction of the straight path*/ if(!direction){ North_Y=OFF; //North and South yellow lights are off North_turn_R=ON; //Red light on for north-south left turn North_R=OFF; //Red light for north-south driving is off North_G=ON; //Green light for north-south straight driving is on }else{ East_Y=OFF; East_turn_R=ON; East_R=OFF; East_G=ON; } } //=========================== void TurnLeft() /*Subfunction of the left turn*/ { if(!direction){ North_G=OFF; //Green light for north-south driving is off North_R=ON; //Red light for north-south driving is on North_turn_R=OFF; //Red light turns off for north-south left turn North_turn_G=ON; //North-South left turn green light is on }else{ East_G=OFF; East_R=ON; East_turn_R=OFF; East_turn_G=ON; } } //=========================== void judge() /*State switching judgment function*/ { if(change_flags){ //Status timing end flag change_flags=0; //Status flag clear run_flags++; //State switch if((run_flags==5)||(run_flags==9))direction=~direction; switch(run_flags){ //status time assignment case 0:case 2:case 4:case 6:case 8: //yellow light flashes time_temp=yellow_time;break; case 1:time_temp=north_green_time;break;//straight north and south case 5:time_temp=east_green_time;break; //Go east and west case 3:time_temp=north_green_time;break;//Turn left from north to south case 7:time_temp=east_green_time;break; //Turn left default:run_flags=1;time_temp=north_green_time; } } } //=========================== void keys_scan() interrupt 0 /*key scan subfunction*/ { delay_ms(20); if(!INT0){ if(!MODE_KEY){ if(++mode_flags>=3)mode_flags=0; } if(!TIME_ADD){ if(mode_flags==1)north_green_time+=5; if(mode_flags==2)east_green_time+=5; if(north_green_time>90)north_green_time=5; if(east_green_time>90)east_green_time=5; } if(!TIME_DEC){ if(mode_flags==1)north_green_time-=5; if(mode_flags==2)east_green_time-=5; if(north_green_time>90)north_green_time=5; if(east_green_time>90)east_green_time=5; } /*You can also add a status here to change the left turn time*/ if(!LOCK_KEY)Lock_flags=~Lock_flags; } INT0=1; } //=========================== void T0_isr() interrupt 1 { if(ovf_cnt==1846)flash_flags=~flash_flags; if(++ovf_cnt>=3691){ ovf_cnt=0; flash_flags=~flash_flags; if(!Lock_flags)if(time_temp--==1)change_flags=1; } } //=========================== void delay_ms(uint xms) { uint i,j; for(i=0;i /*--------------File name: LCD1602.H----------------*/ #ifndef _LCD1602_H_ #define _LCD1602_H_ #define uint unsigned int #define uchar unsigned char #define LCM_P P2 //LCD control port #define LCM_DATA P0 //LCD data port #define LCM_RS_0 LCM_P&=~(1<<5) #define LCM_RS_1 LCM_P|= 1<<5 #define LCM_RW_0 LCM_P&=~(1<<6) #define LCM_RW_1 LCM_P|= 1<<6 #define LCM_EN_0 LCM_P&=~(1<<7) #define LCM_EN_1 LCM_P|= 1<<7 /*========================================*/ #define LCD_str(x,y,s) Locate(x,y);LCD_str_(s); #define LCD_int(x,y,n) Locate(x,y);LCD_int_(n); /*========================================*/ void LCD_init(); //LCM1602 initialization function, must be called before using 1602 void Locate(uchar,uchar); //Display positioning function void LCD_half(uchar); //send half byte function void LCD_char(uchar); //Write a character function void LCD_cmd(uchar); //write command function void LCD_int_(int); //Write integer data function void LCD_str_(uchar str[]); //Write string data function void LCD_delay(uint); //delay sub-function /******************************************/ /*-Function: LCD usage initialization---------------*/ /*-Entry parameters: None*/ /******************************************/ void LCD_init() { LCD_cmd(0x01); LCD_delay(10); LCD_cmd(0x28); //4-bit data, double-line display, 5*7 (0x38 is eight bits) LCM_EN_1;_nop_();_nop_();_nop_(); LCM_EN_0; /*These two sentences must be added here*/ LCD_delay(10); LCD_cmd(0x28); LCD_cmd(0x06); LCD_cmd(0x0c); LCD_cmd(0x01); LCD_delay(10); } /******************************************/ /*-Function: Display data positioning function-------------*/ /*-Entry parameters: row coordinate x, column coordinate y-------------*/ /******************************************/ void Locate(fly x,fly y) { x&=0x01; LCD_cmd((x==0)?(y+0xbf):(y+0x7f)); } /******************************************/ /*-Function: Send half byte function-----------------*/ /*- Entry parameter: To be written to the LCD instruction or data register -*/ /* The upper or lower four bits of the byte---------*/ /******************************************/ void LCD_half(uchar dataw_) { LCM_DATA=(LCM_DATA&0x0f)|(dataw_); LCM_EN_1;_nop_();_nop_();_nop_(); LCM_EN_0; LCD_delay(1); //Add 10ms delay in actual use } /******************************************/ /*-Function: Write one bit of data function---------------*/ /*-Entry parameters: data content---------------------*/ /******************************************/ void LCD_char(uchar dataw) { LCM_RS_1;LCM_RW_0;LCM_EN_0;_nop_(); LCD_half(dataw&0xf0); LCD_half(dataw<<4); } /*========================================*/ void LCD_cmd(uchar cmd) { LCM_RS_0;LCM_RW_0;LCM_EN_0;_nop_(); LCD_half(cmd&0xf0); LCD_half(cmd<<4); } /*========================================*/ void LCD_str_(uchar *str) { while(*str)LCD_char(*str++); } /*========================================*/ void LCD_int_(int num) { uchar counter=0,num_str[10],neg_flags=0; if(num<0){num=-num,neg_flags=1;} do{ num_str[counter++]=num%10+0x30; num/=10; }while(num!=0);//After the loop ends, the value of counter is 1 more than the number if(neg_flags){num_str[counter++]='-';} while(counter) { LCD_char(num_str[--counter]); }//The value of counter must be reduced by one first } /*========================================*/ void LCD_delay(uint xus) { uint i=0,j=0; for(i=0;i
Previous article:Multiplication formula simulation answering device based on 51 single chip microcomputer
Next article:Program and debugging of driving AD9954 with C51 single chip microcomputer
Recommended ReadingLatest update time:2024-11-16 22:35
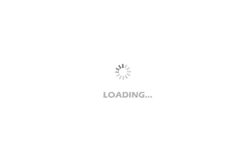
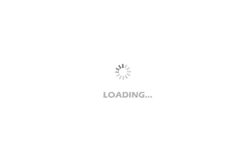
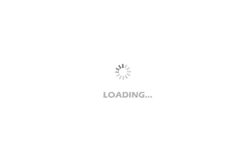
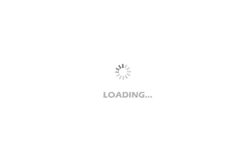
- Popular Resources
- Popular amplifiers
-
Wireless Sensor Network Technology and Applications (Edited by Mou Si, Yin Hong, and Su Xing)
-
Modern Electronic Technology Training Course (Edited by Yao Youfeng)
-
Modern arc welding power supply and its control
-
Small AC Servo Motor Control Circuit Design (by Masaru Ishijima; translated by Xue Liang and Zhu Jianjun, by Masaru Ishijima, Xue Liang, and Zhu Jianjun)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- KiCad 5.1.5 latest stable version Chinese tutorial
- Understand C language pointers in 1 minute
- TMS320F28377S LaunchPad XDS100 V2 driver cannot be installed
- On-policy, its process is the same as the above strategic gradient. Practical analysis
- Answer the questions and win prizes | TDK special reports are waiting for you (Issue 2)
- Today is Chinese Valentine's Day, Alipay has launched an electronic marriage certificate, have you gone to pick up your partner?
- TI MSP430 series microcontroller serial communication baud rate calculation method
- [Atria AT32WB415 Series Bluetooth BLE 5.0 MCU Review] 3.1 MCU generates events and sends commands to Bluetooth APP
- What are the differences in the working principles of military radios and mobile phones? Are their power the same?
- 【Qinheng Trial】Four overall system designs (1/2)——Function integration of ch549 modules