A friend once asked me on the BBS what does {} mean? What is its function? There are many brackets in C, such as {}, [], (), etc., which really confuse some beginners. In some languages such as VB, the same () sign can have different functions. It can be used to combine several statements to form a function block, and can be used as an array subscript, etc. In C, the division of labor of brackets is more obvious. The {} sign is used to combine several statements together to form a function block. This kind of statement composed of several statements is called a compound statement. Compound statements are separated by {}, and each statement inside it still needs to end with a semicolon ";". Compound statements are allowed to be nested, that is, the {} in {} is also a compound statement. When the compound statement is running, the single statements in {} are executed in sequence. In the C language of the microcontroller, the compound statement can be regarded as a single statement, that is, it is grammatically equivalent to a single statement. For a function, the function body is a compound statement. You may know that compound statements can be composed not only of executable statements, but also of variable definition statements. It should be noted that the variables defined in a compound statement are called local variables. The so-called local variables mean that their effective scope is only in the compound statement. Functions are also compound statements, so the effective scope of variables defined in a function is also only within the function. The following is a simple example to illustrate the use of compound statements and local variables.
#include
#include
void main(void)
{
unsigned int a,b,c,d; //Will this definition be in the entire main function?
SCON = 0x50; //Serial port mode 1, allow receiving TMOD = 0x20; //Timer 1 timing mode 2
TH1 = 0xE8; //11.0592MHz 1200 baud rate TL1 = 0xE8;
TI = 1;
TR1 = 1; //Start the timer
a = 5; b = 6; c = 7;
d = 8; //This will be valid throughout the function
printf("0: %d,%d,%d,%dn",a,b,c,d);
{ //Compound statement 1
unsigned int a,e; //Only valid in compound statement 1
a = 10,e = 100;
printf("1: %d,%d,%d,%d,%dn",a,b,c,d,e);
{ //Compound statement 2
unsigned int b,f; //Only valid in compound statement 2
b = 11,f = 200;
printf("2: %d,%d,%d,%d,%d,%dn",a,b,c,d,e,f);
}//Compound statement 2 ends
printf("1: %d,%d,%d,%d,%dn",a,b,c,d,e);
}//Compound statement 1 ends
printf("0: %d,%d,%d,%dn",a,b,c,d);
while(1);
}
Operation results:
0:5,6,7,8
1: 10,6,7,8,100
2: 10,11,7,8,100,200
1: 10,6,7,8,100
0:5,6,7,8 Based on the above explanations, think about why the result is like this.
After reading the previous article, everyone will have a general understanding of the concept of conditional statements, right? Yes, just like learning conditional statements in Chinese, C language is also "if XX then XX" or "if XX then XX otherwise XX". That is, when the condition is met, the statement is executed. Conditional statements are also called branch statements, and some people call them judgment statements. The keyword is composed of if, which is basically the same in most high-level languages. C language provides 3 forms of conditional statements:
1: if (conditional expression) statement: When the result of the conditional expression is true, the statement is executed, otherwise it is skipped. For example, if (a==b) a++; when a is equal to b, a is increased by 1
2: if (conditional expression) statement 1
else statement 2
When the conditional expression is true, statement 1 is executed, otherwise statement 2 is executed, such as if (a==b)
a++;
else
a--;
When a is equal to b, a increases by 1, otherwise a decreases by 1.
3: if (conditional expression 1) statement 1
else if (conditional expression 2) statement 2
else if (conditional expression 3) statement 3
else if (conditional expression m) statement n else statement m
This is a nested if else statement, used to implement multi-directional conditional branches. When using it, you should pay attention to the pairing of if and else. If one is missing, there will be a syntax error. Remember that else is always paired with the closest if. Generally, conditional statements are only used for a single condition or a small number of branches. If there are more branches, the switch statement in the next article will be used more. If you use conditional statements to write a program with more than 3 branches, it will make the program less clear and easy to read.
Previous article:MCU C language tutorial: C51 switch branch statement
Next article:Microcontroller C language tutorial: C51 expression statement and simulator
Recommended ReadingLatest update time:2024-11-16 16:23
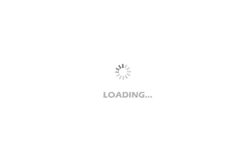
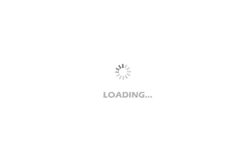
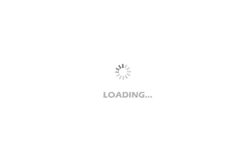
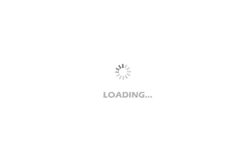
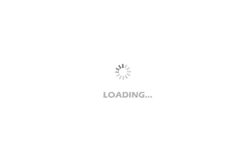
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- MSP430F5438A instruction cycle and clock cycle
- AVR assembler, paid help
- Allegro software design has been updated with the latest patch, but why can't I do 3D simulation?
- EEWORLD University ---- Embedded Linux Development Introduction Video
- What is a smart WiFi module? What are the types and functions of wireless router gateway control modules?
- Case! EMI rectification of power supply
- Reasons for choosing 4-20mA for flow meter
- I want to ask why this motor cannot start? The voltage from B+ to B- is normal, but after starting, the voltage from B+ to M- jumps and the motor vibrates.
- Summary of equivalent circuits of common electronic components
- Comparison of several MicroPython IDEs