1 Basic skills of C51 language programming
C language is a high-level programming language that provides a very complete standardized process control structure. Therefore, when using C51 language to design single-chip microcomputer application system programs, the structured programming method should be adopted as much as possible, so that the entire application system program structure can be clear and easy to debug and maintain. For a larger program, the entire program can be divided into several modules according to function, and different modules perform different functions. For different functional modules, the corresponding entry parameters and exit parameters are specified respectively, and some frequently used programs are preferably compiled into functions, which will not cause confusion in the management of the entire program, but also enhance readability and portability.
In the process of program design, make full use of the preprocessing commands of the C51 language. For some commonly used constants, such as TRUE, FALSE, PI and various special function registers, or some important constants in the program that are variable according to external conditions, you can use macro definitions "#define" or concentrate them in a header file for definition, and then use the file include command "#include" to add them to the program. In this way, when you need to modify a certain parameter, you only need to modify the corresponding include file or macro definition, and you don't have to modify each program file that uses them, which is conducive to file maintenance and updating. The following examples are given:
Example 1 For different single-chip crystal oscillators, the program takes different delay times, and the length of the delay time can be modified according to changes in external conditions. For such a program, macro definitions and conditional compilation can be used to implement it. The program is as follows:
#define flag 1
#ifdef flag==1
#define fosc 6M
delay=10;
#elif flag = = 0
#define fosc 8M
delay=12;
#else
#define fosc 12M
delay=20;
#endif
main()
{
for(I=0;I
In this way, the source program can be applied to microcontroller systems with different clock frequencies without any modification, and different delay values can be taken according to different situations to achieve different purposes.
2 Mixed programming of C51 language and assembly language programs
The C51 compiler can compile C language source programs efficiently and generate efficient and concise codes. In most cases, C language programming can achieve the expected purpose. However, sometimes, for intuitive programming or the processing of some special addresses, certain assembly language programming must be used. In other cases, for some purposes, assembly language can also call C language. In this mixed programming, the key is the parameter transfer and the return value of the function. They must have complete agreements, otherwise the data exchange may go wrong. The following uses Liyuan's 10-bit serial A/D converter TLC1549 as an example to illustrate the calling of C language programs and assembly language programs. [page]

The pin diagram and timing diagram of 1549 are shown in Figure 1 and Figure 2 respectively. Assume that DATA OUT is connected to P1.0, CS is connected to P1.1, and CLOCK is connected to P1.2.
Please refer to the relevant information for the specific characteristics of 1549.
Figure 2 TLC1549 timing diagram
Example 2 Calling C language program and assembly language program, the subroutine is as follows:
PUBLIC AD; Entry address
SEG_AD SEGMENT CODE; Program segment
RSEG SEG_AD
USING 0
AD: MOV R6,#00
MOV R7,#00
SETB P1.1
ACALL DELAY
CLR P1.1
ACALL DELAY
MOV R0,#10
RR0: SETB P1.2
NOP
CLR P1.2
DJNZ R0,RR0
ACALL DELAY
MOV 30H,R6; A/D conversion high
; two bits are stored in R6
ACALL CIR
MOV R6,30H
SETB P1.2
NOP
CLR P1.2
MOV 30H,R6
ACALL CIR
MOV R6,30H
MOV R0,#8; A/D conversion low
; 8 bits are stored in R7
RR2: SETB P1.2
NOP
CLR P1.2
MOV 30H,R7
ACALL CIR
MOV R7,30H
DJNZ R0,RR2
RET
CIR: CLR C
MOV C,P1.0
MOV A,30H
RLC A
MOV 30H,A
RET
END [page]
In the above program, the return value of the function is an unsigned integer. According to the calling rules, the high bit of the return value must be in R6 and the low bit in R7, so as to ensure that the data transmission is error-free. In addition, during the calling process, attention must be paid to the stacking of registers. In this way, when A/D conversion is used in the future, the assembly language subroutine AD() can be called in C language.
3 C51 interrupt processing process
The C51 compiler supports the development of interrupt procedures directly in the C source program, thus reducing the tedious work of using assembly language and improving development efficiency. The complete syntax of the interrupt service function is as follows:
void function name (void) [mode]
[reentry] interrupt n [using r]
where n (0~31) represents the interrupt number. The C51 compiler allows 32 interrupts, and the specific interrupt to be used is determined by the 80C51 series chip. r (0-3) represents the rth group of registers. When calling an interrupt function, the register group used by the function called by the interrupt procedure must be the same as that of the interrupt function. "Reentry" is used to indicate whether the interrupt handling function has the "reentry" capability. The C51 compiler and its extension of the C language allow programmers to control all aspects of interrupts and use register groups. This support enables programmers to create efficient interrupt service programs. Users only need to care about interrupts and necessary register group switching operations in the C language.
Example 3 Assume that the FOSC of the microcontroller is 12MHz, and it is required to program using T0 mode 1 to output a square wave with a period of 2ms at the P1.0 pin.
The interrupt service program written in C language is as follows:
#include
sbit P1_0=P1^0;
void timer0(void)interrupt 1 using 1 {
/*T0 interrupt service program entry*/
P1_0=!P1_0;
TH0=-(1000/256); /*Reload the initial count value*/
TL0=-(1000%256);
}
void main(void)
{
TMOD=0x01; /*T0 works in timer mode 1*/
P1_0=0;
TH0=-(1000/256); /*Preset the initial count value*/
TL0=-(1000%256);
EA=1; /*CPU turns on interrupt*/
ET0=1; /*T0 turns on interrupt*/
TR0=1; /*Start T0*/
do{}while(1);
}
When writing interrupt service routines, it is important to note that parameters cannot be passed and there cannot be return values.
4 Conclusion
C51 compiler can not only shorten the development cycle of single-chip control systems, but also facilitate debugging and maintenance. In addition, C51 language has many powerful functions, such as providing rich library functions for users to call directly, complete compilation control instructions to provide necessary symbol information for program debugging, etc. In short, C51 language is a powerful tool for a large number of single-chip developers.
Previous article:Using 51 single chip microcomputer to realize the conversion between Gregorian calendar and lunar calendar
Next article:Several useful modules for single-chip C51 programming
Recommended ReadingLatest update time:2024-11-16 19:36
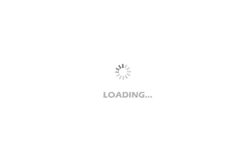
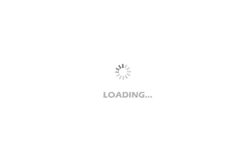
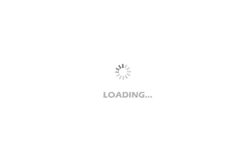
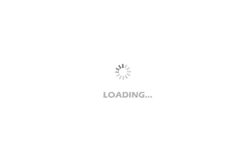
- Popular Resources
- Popular amplifiers
-
西门子S7-12001500 PLC SCL语言编程从入门到精通 (北岛李工)
-
Siemens Motion Control Technology and Engineering Applications (Tongxue, edited by Wu Xiaojun)
-
How to read electrical control circuit diagrams (Classic best-selling books on electronics and electrical engineering) (Zheng Fengyi)
-
MCU C language programming and Proteus simulation technology (Xu Aijun)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Help
- Failed experience of using BlueNRG-1 to make a BLE chicken-eating tool
- Exploiting software vulnerabilities! 19-year-old boy remotely hacked into Tesla
- Gizwits GoKit 3 Hardware Manual
- TMS320C6678 ZYNQ Dual-core ARM Communication Case Development Manual - matrix_multiply Case
- Monochrome screen upgrade: How to quickly upgrade the DWIN T5UIC1 color screen
- Intelligent management tools used by power operation and maintenance personnel
- Precautions for using C/C++ language to write programs based on TMS320 series DSP
- [Automatic clock-in walking timing system based on face recognition] K210 MAIXBIT uses QR code recognition as user information
- Several types of Bluetooth antennas are summarized. Which one is more familiar with Bluetooth signals? Which type of Bluetooth is used for the same chip...