In single-chip microcomputer application systems, it is often necessary to communicate with microcomputers through RS-232 serial ports. Among various operating systems, Microsoft's Windows is more common, and most of them are 32-bit platforms such as Windows 95/98. In the past, serial communication on the Windows platform was mostly implemented using the API functions provided by it. This method requires many low-level settings to use, so it is cumbersome and difficult to understand. The ActiveX technology launched by Microsoft provides another way to implement serial communication. This method is not only relatively simple, but also very practical. Especially in the visual object-oriented programming environment such as Visual C++, the serial port can be truly regarded as an object. Only simple settings are required when programming, and it is also easy to understand. The following discusses in detail how to use the serial communication ActiveX control provided by Microsoft. The corresponding file of this control is MSCOMM32.OCX, hereinafter referred to as the MSCOMM control.
1. MSCOMM control
MSCOMM control, namely Microsoft Communication Control, is an ActiveX control provided by Microsoft to simplify serial communication programming under Windows. It provides a series of standard communication command interfaces, which can be used to establish a connection with the serial port, connect to other communication devices (such as modems) through the serial port, issue commands, exchange data, and monitor and respond to events and errors that occur in the serial connection. The MSCOMM control can be used to create telephone dialing programs, serial communication programs, and full-featured terminal programs.
The MSCOMM control provides two ways to handle communication:
(1) Event-driven method. When a communication event occurs, the MSCOMM control triggers the OnComm event. The caller can capture the event and check its CommEvent property to confirm what event or error occurred, so as to handle it accordingly. The advantages of this method are timely response and high reliability.
(2) Query method. After each key function of the program, you can query events and errors by checking the value of the CommEvent property. If the application is small, this method may be more desirable. For example, if you write a simple telephone dialing program, there is no need to generate an event for each character received, because the only character waiting to be received is the "OK" response from the modem.
When using the MSCOMM control, one MSCOMM control can only correspond to one serial port at a time. If the application needs to access and control multiple serial ports, multiple MSCOMM controls must be used.
In VC++, the MSCOMM control corresponds to only one C++ class - CMSComm. Since the MSCOMM control itself does not provide methods, the CMSComm class, except for the Create() member function, has Get/Set function pairs to get or set the properties of the control. The MSCOMM control also has only one OnComm event to notify the caller that a communication event has occurred.
The MSCOMM control has many important properties. Due to space limitations, only a few more important and commonly used properties are given, as listed in Table 1.
Table 1 Important properties of the MSCOMM control:
Property Description
CommPort Communication port number
Settings Baud rate, parity, data bit expressed in string form
PortOpen The status of the communication port, open or close
Input Receive data
Output Send data
InputMode Type of received data: 0 is text; 1 is binary
2. Programming implementation
As can be seen from Table 1, MSCOMM can receive data in two different forms, namely in text form and in binary form. There are many documents and materials on using MSCOMM control to transmit character data. Such an example can be found in Microsoft's MSDN (Microsoft Developer Network), namely VCTERM. However, almost all raw data obtained by measurement systems with single-chip microcomputer as the core are in binary form, so transmitting data in binary form will be the most direct and concise way. In addition, since the MSCOMM control transmits characters in wide character format in text form, additional processing is required to obtain useful information. Therefore, this article mainly discusses the use method in binary form.
In VC++6.0, three types of applications can be generated using APPWizard: single document (SDI), multiple documents (MDI) and dialog-based applications. In order to illustrate the problem and omit unnecessary details, the following takes a dialog-based application as an example.
1. Create a dialog-based application
Open the VC++6.0 integrated development environment, select the menu item File/New, select MFC AppWizard (exe) in the Projects tab in the dialog box that appears, and then fill in MyCOMM (name it as needed) in the Project Name box, and then click the OK button. In the dialog box that appears next, select the Dialog Based item, and then click the NEXT button. The following dialog boxes are set by default, so that a dialog-based application can be generated. Its dialog box template will appear in the resource programmer.
2. Insert the MSCOMM control
Select the menu item Project/Add to project/Components and Controls…, select Microsoft Communications Control, version 6.0 in the Registered ActiveX Controls folder in the pop-up dialog box, and then press the Insert button. Then a dialog box will pop up, prompting the generated class name and file name. Press the OK button to insert the control. At this time, a control icon that looks like a telephone will appear on the control toolbar of the dialog box, and a class CMSComm will also appear in the Classview of the Workspace. [page]
At this time, you can add the MSCOMM control to the dialog box template, and the addition method is the same as other controls. Then you also need to add a member variable to the dialog class accordingly, and here we name it m_comm. To add it: First, in the dialog template, right-click the control, select ClassWizard, and in the Control Ids item of the Member Variables tab of the dialog box that appears, select IDC_MSCOMM1. Then, press the Add Variable… button, and enter m_comm in the Member Variable Name item of the dialog box that appears. Finally, press the OK button.
3. Set properties
You can set the properties of the control in two places:
(1) In the dialog resource editor. On the dialog template, right-click the MSCOMM control and select the Properties… menu item to set various properties. Only the following items are modified here, and the others are accepted by default: Rthershold: 1, InputLen: 1, DTREnable: Unchecked, InputMode: 1-Binary.
(2) In the OnInitDialog() function of the dialog class. : Below is the function implementation of the above settings:
BOOL CMyCOMMDlg::OnlnitDialog()
{
CDialog::OnlnitDialog();
//This is the code automatically generated by the application framework and is not listed
//TODO: Add extra initialization here
m_comm.SetCommPort(1); //Use serial port 1
m_comm.SetSettings("9600, N, 8, 1");
//The baud rate is 9600, no parity, 8 data bits, 1 stop bit
m_comm.SetRThreshold(10); //Trigger a receive event every 10 characters received
m_comm.SetSThreshold(0); //Do not trigger a send event
m_comm.SetInputLen(10); //Each read operation takes 10 characters from the buffer
m_comm.SetInputMode(1); //Binary data transmission form
m_comm.SetPortOpen(TRUE); //Open the serial port
return TRUE; //return TRUE unless you set the focus to a control
}
4. Send binary data
If you need to send binary data, you can process the data as follows. The specific code is as follows:
CByteArray bytOutArr;
bytOutArr.Add(0x0); //Assign values to the array
bytOutArr.Add(0x1);
bytOutArr.Add(0x2);
bytOutArr.Add(0x3);
bytOutArr.Add(0x4);
COleVariant varOut;
varOut=COleVariant(bytOutArr); //Convert the data to the variant data type
m_comm.SetOutput (varOut); //Send data
5. Receive binary data
When you need to receive a large amount of data, it is best to use event-driven programming. The specific steps are as follows:
(1) Respond to the OnComm event. In the dialog resource programmer, double-click the MSCOMM control on the dialog template and fill in the event response function name you want in the pop-up dialog box. Here it is named OnCommMscomm1().
(2) Receive and process data in the event response function. The received data is variant data, so some processing is needed. The specific code is as follows:
void CMyCOMMDlg::OnCommMscomm1()
{
COleVariant varRcv;
CByteArray byt;
int i;
long num;
switch (m_comm.GetCommEvent())
{
cass 1://data sending event
break;
case 2://data receiving event
varRcv=m_comm.GetInput();
varRcv.ChangeType (VT_ARRAY |VT_UI1);
BYTE HUGEP *pbstr;
HRESULT hr;
hr=SafeArrayAccessData (varRcv.parray,(void HUGEP*FAR*)&pbstr);//Get the safe array pointer
if (FAILED (hr)){
AfxMessageBox("Failed to get the array pointer!");
break;}
num=0;
hr=SafeArrayGetUBound (varRcv.parray,1,&num);//Get the upper bound of the arrayif
(FAILED (hr)){
AfxMessageBox("Failed to get the upper bound of the array!");
break;}
for(i=0;i
byt.Add(pbstr [i]);
SafeArrayUnaccessData (varRcv.parray);
//At this point, the data has been saved in the binary array byt, and related processing can be performed as neededbreak
;
default:
break;
}
}
The processing part in the above code can be made into a separate function and called here. After the processing of the above code, the received data has been stored in the binary array byt, and it can be processed according to your needs, such as saving and displaying.
3. Hardware interface
The hardware interface between the microcontroller and the microcomputer can be realized with a MAX232 or ICL232 and a few capacitors. There are many literatures discussing this, so I will not go into details here.
The above method has been applied by the author in practice and feels very simple and convenient, and has strong practical significance.
Previous article:Using MSP430F149 single chip microcomputer to realize universal controller of stepper motor
Next article:Hardware design trivia 1: Low power design
Recommended ReadingLatest update time:2024-11-16 19:51
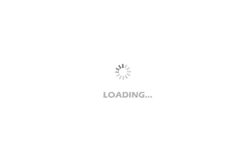
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Award-winning live broadcast: Registration for the introduction of ON Semiconductor's photovoltaic and energy storage products is now open~
- CircuitPython 7.0.0 Alpha 4 released
- How to Erase the Contents of EPCS in Altera FPGA Configuration Device
- [Problem Feedback] Anlu TangDynasty ChipWatcher network sorting confusion issue
- Instrument and control system grounding and shielding training materials
- ART-Pi evaluates the display driver LTDC+SDRAM of H7 RGB screen
- 64 yuan group purchase (5 left): Thumb board NUCLEO-L432KC, limited quantity, come quickly
- CCS uninstallation and installation process and problems encountered
- [Summary] EEWorld invites you to disassemble (fifth issue) - disassemble the power strip and learn to evaluate the circuit
- Why install a mobile base station?