The external structure of the microcontroller:
1. DIP40 dual in-line socket;
2. P0, P1, P2, P3 four 8-bit quasi-bidirectional I/O pins; (When used as I/O input, high level must be output first)
3. Power supply VCC (PIN40) and ground GND (PIN20);
4. High level reset RESET (PIN9); (10uF capacitor connected to VCC and RESET can achieve power-on reset)
5. Built-in oscillation circuit, externally just connect crystal to X1 (PIN18) and X0 (PIN19); (the frequency is 12 times of the main frequency)
6. Program configuration EA (PIN31) connected to high level VCC; (run the program in the internal ROM of the microcontroller)
7. P3 supports the second function: RXD, TXD, INT0, INT1, T0, T1
MCU internal I/O components: (the so-called learning MCU is actually programming and controlling the following I/O components to complete the specified task)
1. 1. Four 8-bit general-purpose I/O ports, corresponding to pins P0, P1, P2 and P3;
2. Two 16-bit timer counters; (TMOD, TCON, TL0, TH0, TL1, TH1)
3. One serial communication interface; (SCON, SBUF)
4. One interrupt controller; (IE, IP)
For the AT89C52 microcontroller, the header file AT89x52.h gives the definitions of all ports of the SFR special function registers. Page 160 of the textbook gives the C language extended variable types for the MCS51 series microcontrollers.
Basics of MCU C language programming
1. Hexadecimal representation of byte 0x5a: binary is 01011010B; 0x6E is 01101110.
2. If a 16-bit binary number is assigned to an 8-bit byte variable, it is automatically truncated to the lower 8 bits and the upper 8 bits are discarded.
3. ++var means to increase the variable var by one first; var— means to decrease the variable by one later.
4. x |= 0x0f; is represented as x = x | 0x0f;
5. TMOD = ( TMOD & 0xf0 ) | 0x05; means to assign 0x5 to the lower four bits of the variable TMOD without changing the upper four bits of TMOD.
6. While( 1 ); means to execute the statement indefinitely, that is, an infinite loop. The semicolon after the statement indicates an empty loop body, that is, {;}
Programming method to output high level on a certain pin: (such as P1.3 (PIN4) pin)
#include //This header document contains symbolic definitions of the microcontroller's internal resources, including P1.3
void main( void ) //void means no input parameters and no function return value. This is the reset entry for the microcontroller to run
{
P1_3 = 1; //Assign 1 to P1_3, and pin P1.3 can output a high level VCC
While( 1 ); //Infinite loop, equivalent to LOOP: goto LOOP;
}
Note: When each pin of P0 is to output a high level, an external pull-up resistor (such as 4K7) must be connected to the VCC power supply.
Programming method to output low level on a certain pin: (such as P2.7 pin)
#include //This header document contains symbolic definitions of the microcontroller's internal resources, including P2.7
void main( void ) //void means no input parameters and no function return value. This is the reset entry for the microcontroller to run
{
P2_7 = 0; //Assign 0 to P2_7, and pin P2.7 can output a low level GND
While( 1 ); //Infinite loop, equivalent to LOOP: goto LOOP;
}
How to program a square wave output on a certain pin: (such as P3.1 pin)
#include //This header document contains symbolic definitions of the internal resources of the microcontroller, including P3.1
void main( void ) //void means no input parameters and no function return value. This is the reset entry for the microcontroller to run
{
While( 1 ) //Non-zero means true. If true, execute the following loop statement
{
P3_1 = 1; //Assign 1 to P3_1, and pin P3.1 can output a high level VCC
P3_1 = 0; //Assign 0 to P3_1, and pin P3.1 can output a low level GND
} //Since it is always true, it continuously outputs high, low, high, low..., thus forming a square wave
}
After inverting the input level of a pin, output it from another pin: (for example, P0.4 = NOT( P1.1) )
#include //This header document contains symbolic definitions of the microcontroller's internal resources, including P0.4 and P1.1
void main( void ) //void means there are no input parameters and no function return values. This is the reset entry for the microcontroller to run
{
P1_1 = 1; //Initialization. As an input, P1.1 must output a high level.
While( 1 ) //Non-zero indicates true. If true, execute the following loop statements
{
if( P1_1 == 1 ) //Read P1.1, which means P1.1 is considered as an input. If P1.1 inputs a high level VCC
{ P0_4 = 0; } //Assign 0 to P0_4, and pin P0.4 can output a low level GND
else //Otherwise, P1.1 input is a low level GND
//{ P0_4 = 0; } //Assign 0 to P0_4, and pin P0.4 can output a low level GND
{ P0_4 = 1; } //Assign 1 to P0_4, and pin P0.4 can output a high level VCC
} //Since it is always true, the output level of P0.4 is constantly changed according to the input of P1.1
}
The input level of 8 pins of a port is inverted, and the lower four bits are output from 8 pins of another port: (for example, P2 = NOT( P3 ) )
#include //This header document contains symbolic definitions of the microcontroller's internal resources, including P2 and P3
void main( void ) //void means no input parameters and no function return value. This is the reset entry for the microcontroller to run
{
P3 = 0xff; //Initialization. As an input, P3 must output a high level, and at the same time output a high level to the 8 pins of the P3 port
While( 1 ) //Non-zero means true. If true, execute the following loop statements
{ //The method of negation is XOR 1, and the method of non-negation is XOR 0
P2 = P3^0x0f //Read P3, that is, consider P3 as input, XOR the lower four bits to 1, that is, negate, and then output
} //Since it is always true, P3 is continuously negated and output to P2
}
Note: 8-bit D7, D6 to D0 of a byte are output to P3.7, P3.6 to P3.0 respectively. For example, if P3=0x0f, the four pins P3.7, P3.6, P3.5 and P3.4 will all output low level, while the four pins P3.3, P3.2, P3.1 and P3.0 will all output high level. Similarly, inputting a port P2 means reading P2.7, P2.6 to P2.0 into 8-bit D7, D6 to D0 of a byte.
1. Connect power supply: VCC (PIN40), GND (PIN20). Add decoupling capacitor 0.1uF
2. Connect crystal: X1 (PIN18), X2 (PIN19). Note that the crystal frequency (select 12MHz) and auxiliary capacitor 30pF are marked
3. Connect reset: RES (PIN9). Connect the power-on reset circuit and the manual reset circuit, and analyze the reset working principle
4. Connect configuration: EA (PIN31). Explain the reason.
LED control: MCU I/O output
Connect the positive pole (anode) of a light-emitting diode LED to P1.1, and the negative pole (cathode) of the LED to the ground GND. As long as P1.1 outputs a high level VCC, the LED will be forward-conducted (the voltage drop on the LED is greater than 1V when it is turned on), and current will flow through the LED until the LED lights up. In fact, since the high-level output resistance of P1.1 is 10K, it plays the role of output current limiting, so the current flowing through the LED is less than (5V-1V)/10K = 0.4mA. As long as P1.1 outputs a low level GND, which is actually less than 0.3V, the LED cannot be turned on, and the LED will not light up. [page]
Switch double key input: input first, output high
One button KEY_ON is connected between P1.6 and GND, and the other button KEY_OFF is connected between P1.7 and GND. When KEY_ON is pressed, the LED is on, and when KEY_OFF is pressed, the LED is off. When both buttons are pressed, the LED is half-lit, and when the buttons are released, the LED remains on, that is, ON is on and OFF is off.
#include
#define LED P1^1 //Replace P1_1 with the symbol LED
#define KEY_ON P1^6 //Replace P1_6 with the symbol KEY_ON
#define KEY_OFF P1^7 //Replace P1_7 with the symbol KEY_OFF
void main( void ) //The execution entry after the MCU is reset, void means empty, no input parameters, no return value
{
KEY_ON = 1; //As input, first output high, then KEY_ON, P1.6 is grounded to 0, otherwise the input is 1
KEY_OFF = 1; //As input, first output high, then KEY_OFF, P1.7 is grounded to 0, otherwise the input is 1
While( 1 ) //Always true, so always loop and execute all the statements in the following brackets
{
if( KEY_ON==0 ) LED=1; //Yes, KEY_ON is connected, as shown, P1.1 outputs high and LED is
onif( KEY_OFF==0 ) LED=0; //When KEY_OFF is pressed, P1.1 output is low and LED is off
} //After releasing the key, no value is assigned to the LED, so the LED maintains the last key state.
//When pressed at the same time, the LED is constantly on and off, each taking half the time, and the alternation frequency is very fast. Due to the inertia of the human eye, it looks half-lit
}
Digital tube connection and driving principle and single chip microcomputer programming
A seven-segment digital tube is actually composed of 8 LEDs, 7 of which form the seven strokes of the number 8, so it is called a seven-segment digital tube, and the remaining LED is used as a decimal point. As a habit, the 8 LEDs are marked with symbols: a, b, c, d, e, f, g, h. Corresponding to the top stroke of 8, arranged in a clockwise direction, the middle stroke is g, and the decimal point is h.
We usually correspond each diode to the 8 bits of a byte, a(D0), b(D1), c(D2), d(D3), e(D4), f(D5), g(D6), h(D7), and the corresponding 8 light-emitting diodes are connected to the 8 pins of a port Pn of the microcontroller, so that the microcontroller can control the brightness of the 8 light-emitting diodes through the high and low levels output by the pins, thereby displaying various numbers and symbols; corresponding to the byte, the pin connection method is: a(Pn.0), b(Pn.1), c(Pn.2), d(Pn.3), e(Pn.4), f(Pn.5), g(Pn.6), h(Pn.7).
If the negative poles (cathodes) of the 8 light-emitting diodes are connected together as a pin of the digital tube, this digital tube is called a common cathode digital tube, the common pin is called a common cathode, and the 8 positive poles are segment poles. Otherwise, if the positive pole (anode) is connected together and led out, it is called a common anode digital tube, the common pin is called a common anode, and the 8 negative poles are segment poles.
Taking a single common cathode digital tube as an example, the segment pole can be connected to a port Pn, and the common cathode can be connected to GND, then the seven-segment code table byte data corresponding to the hexadecimal code can be written as shown on the right:
16-key code display single chip program
We connect a common cathode digital tube SLED to the P1 port, and connect 16 keys to the P2 and P3 ports, numbered KEY_0, KEY_1 to KEY_F respectively. Only one key can be pressed during operation, and after the key is pressed, the SLED displays the corresponding key number.
#include
#define SLED P1
#define KEY_0 P2^0
#define KEY_1 P2^1
#define KEY_2 P2^2 #define KEY_3 P2^3
#define
KEY_4 P2^4
#define KEY_5 P2^5
#define KEY_6 P2^6
#define KEY_7 P2^7
#define KEY_8 P3^0
#define KEY_9 P3^
1
#define KEY_A P3^2 #define KEY_B P3^3
#define KEY_C P3^4
#define
KEY_D P3^5
#define KEY_E P3^6
#define KEY_F P3 ^7
Code unsigned char Seg7Code[16]= //Use hexadecimal as array subscript to directly obtain the corresponding seven-segment code byte
// 0 1 2 3 4 5 6 7 8 9 A b C d EF
{0x3f, 0x06, 0x5b, 0x4f, 0x66, 0x6d, 0x7d, 0x07, 0x7f, 0x6f, 0x77, 0x7c, 0x39, 0x5e, 0x79, 0x71};
void main( void )
{
unsigned char i=0; //As array subscript
P2 = 0xff; //P2 is used as input, initialize output high
P3 = 0xff; //P3 is used as input, initialize output high
While( 1 )
{
if( KEY_0 == 0 ) i=0; if( KEY_1 == 0 ) i=1;
if( KEY_2 == 0 ) i=2; if( KEY_3 == 0 ) i=3;
if( KEY_4 == 0 ) i=4; if( KEY_5 == 0 ) i=5;
if( KEY_6 == 0 ) i=6; if( KEY_7 == 0 ) i=7;
if( KEY_8 == 0 ) i=8; if( KEY_9 == 0 ) i= 9;
if( KEY_A == 0 ) i=0xA; if( KEY_B == 0 ) i=0xB;
if( KEY_C == 0 ) i=0xC; if( KEY_D == 0 ) i=0xD;
if( KEY_E == 0 ) i=0xE; if( KEY_F == 0 ) i=0xF;
SLED = Seg7Code[ i ]; // Display 0 at the beginning, and get the seven-segment code according to i
}
}
Previous article:The software and hardware design of the minimum system of single chip microcomputer
Next article:MCU LCD display code
Recommended ReadingLatest update time:2024-11-16 17:52
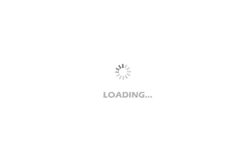
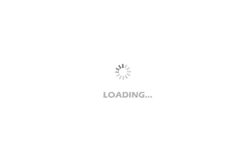
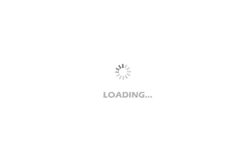
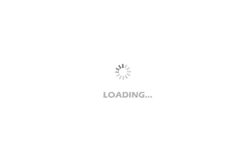
- Popular Resources
- Popular amplifiers
-
Wireless Sensor Network Technology and Applications (Edited by Mou Si, Yin Hong, and Su Xing)
-
Modern Electronic Technology Training Course (Edited by Yao Youfeng)
-
Modern arc welding power supply and its control
-
Small AC Servo Motor Control Circuit Design (by Masaru Ishijima; translated by Xue Liang and Zhu Jianjun, by Masaru Ishijima, Xue Liang, and Zhu Jianjun)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- MSP430 MCU simulation example: LCD1602 liquid crystal display
- [Atria Development Board AT32F421 Review] Timer PWM Output
- "Date in Spring" + Where the peach blossoms are in full bloom
- TI Selected Chinese Reference Design Industrial Applications (Full Book)
- EEWORLD University Hall ---- Learn FPGA with you ---- Hao Xushuai team of Sanxin Intelligent
- Introduction to the internal structure of C2000
- 【IoT Development】Zhengdian Atom STM32 Battleship v3+Gizwits AIoT+APP Control
- Let me express my feelings and talk about phone calls and scammers
- MC33063A boost circuit, burning IC and electrolytic capacitor? With pictures, help!
- Could anyone help me see what is wrong with my amplifier circuit?