1 Basic principles
Error checking of serial data is a necessary means to ensure the correctness of data. Odd-even check and cyclic redundancy check are usually used. Both methods provide necessary information through redundant data. Parity check is applicable to data transmission in bytes. For example, when transmitting an ASCII character with even check, a check bit is added so that the number of "1" in all 9 bits is an even number. Parity check is simple and easy to implement, but when data collapses or multiple errors occur, it is often impossible to detect them, so the reliability is not high.
The cyclic redundancy code check method uses the loop and feedback mechanism. The check code is obtained by a relatively complex operation of the input data and the historical data. Therefore, the redundant code contains more abundant information between the data and has higher reliability. The calibrated cyclic redundancy code can check the following errors: ① any odd number of all data bits are wrong; ② any consecutive 2 bits of all data bits are wrong; ③ any 1 to 8 bits of data within an 8-bit time window are wrong.
When using the cyclic redundancy code check method to communicate, the sender first calculates the check code of the data to be sent, and then sends the data together with the check code; the receiver calculates the cyclic redundancy code while receiving the data, and compares the calculation result with the check code from the sender. If they are different, it means that an error occurred during the transmission process, and the receiver must notify the sender to send the group of data again.
Assume that 64 bits of data are to be transmitted (the last 8 bits are the check code), and use the polynomial x8+x5+x4+1 to generate an 8-bit cyclic redundancy check code (hereinafter referred to as CRC code). Its logical structure can be represented by an XOR gate and a shift register, as shown in Figure 1. The value of the register is the CRC code of the input data. First, input the XOR value of the data and the lowest bit. If it is "0", just shift the current CRC code right by 1 bit (fill the first bit with zero) to get a new CRC code; if it is "1", XOR the current CRC code with 18H, and then shift it right by 1 bit. The check code has the following characteristics: ① When the input 8-bit data (low bit first) is the same as the current CRC code, the output CRC code will be zero. Therefore, when all 64 bits of data including the 8-bit CRC code are input, the output CRC code should be zero. ② As long as there is a non-zero bit, the transmission error can be judged, and complex check technology is not necessary.
2 Generate cyclic redundancy check code using assembly language
On the 8051 microcontroller, the following code can be used to obtain the 8-bit CRC code (stored in the variable CRC), and the 8-bit input data is temporarily stored in ACC.
DO_CRC: PUSH ACC; save input data
PUSH B ; save register B [page]
PUSH ACC ; Save again
MOV B, #8; total 8 bits of data
CRC_LOOP: XRL A, CRC
RRC A ; Place the XOR value of the lowest bit and the input data into the carry flag
MOV A, CRC
JNC ZERO
XRL A, #18H; the current CRC code is XORed with 18H
ZERO: RRC A; right shift 1 bit
MOV CRC, A; save the new CRC code
POP ACC
RR A ; take out the second bit of input data
PUSH ACC
DJNZ B,CRC_LOOP ; loop
POP ACC
POP B
POP ACC; restore each register
RET
The above program performs a series of operations for each bit of input data, which requires a lot of calculations, but takes up little memory, so it is suitable for situations where memory is tight. When memory is sufficient, a more efficient table lookup method can be used.
3 Use the table lookup method to find the CRC code
Separate the input data into bytes, and each byte value is between 0 and 255. Let the current CRC code be 00H. When 0 to 255 are input respectively, 256 CRC codes are obtained. Arrange them in sequence to form a cyclic redundancy check code table. Use the value of the current CRC code and the input byte after XOR as the subscript, and look up the table to find the new CRC code. In the following example, crc stores the CRC code, ACC stores the input byte, and crc_table is the entry address of the cyclic redundancy check table. The code is as follows:
XRL A, crc; current CRC code and input data XOR
PUSH DPH
PUSH DPL ; save data pointer
MOV DPTR #crc_table
MOVC A, @A+DPTR; look up the table
MOV crc,A
POP DPL
POP DPH ; Restore data pointer [page]
RET
crc_table:
DB 00H,5EH,BCH,E2H,61H,3FH,DDH,83H
DB C2H,9CH,7EH,20H,A3H,FDH,1FH,41H
DB 9DH,C3H,21H,7FH,FCH,A2H,40H,1EH
…
4 CPLD Implementation of Cyclic Redundancy Check Code
Using CPLD to implement the cyclic redundancy check code hardware is faster, has better performance, and only occupies very few resources in the CPLD. I used Xilinx's XCV9536 chip and implemented an 8-bit CRC code generation circuit based on the following VHDL code. The VHDL code below implements an 8-bit CRC code generation circuit. In the code, s_in is the input serial data, q is the output CRC code, and d_new is the current CRC code.
Library IEEE;
use IEEE.std_logic_1164.all;
entity crc is
port(clk,s_in,reset:in STD_LOGIC,q:out STD_LOGIC_VECTOR (7 downto 0));
end crc;
architecture crc_arch of crc is
signal t1,t2,t3:std_logic;
signal d_new:std_logic_vector(7 downto 0);
begin
t1<=d_new(0)xor s_in; --t1 is the lowest bit XORed with the input value
t2<=d_new(4)xor '1';
t3<=d_new(3)xor'1';
process(clk,reset)
begin
if clk event and clk='1'then
if reset='1'then
d_new<=x"0"; --When reset, the CRC code is set to zero
elsif t1='1'then
d_new<=t1&d_new(7 downto 5)&t2&t3&td_new(2 downto 1);--New CRC code when t1 is "1"
elsif t1='0'then
d_new<=t1&d_new(7 downto 1); --New CRC code when t1 is "0"
end if;
end if;
end process;
q<=d_new;--output CRC code
end crc_arch;
5 Conclusion
Based on the software and hardware implementation methods of the 8-bit cyclic redundancy check code introduced above, various types of cyclic redundancy check methods can be designed. From the above routines, it can be seen that the cyclic redundancy check is a highly reliable and easy-to-implement check method.
Previous article:Application of W77E58 in RTU Remote Signaling Unit
Next article:Design of high reliability system based on 32-bit single-chip microcomputer MC68HC376
Recommended ReadingLatest update time:2024-11-16 16:40
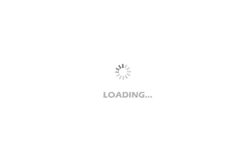
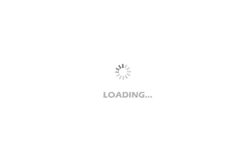
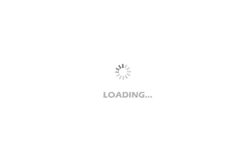
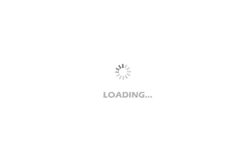
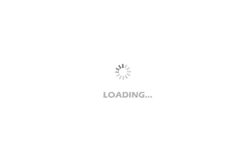
- Popular Resources
- Popular amplifiers
-
Learn CPLD and Verilog HDL programming technology from scratch_Let beginners easily learn CPLD system design technology through practical methods
-
Practical Electronic Components and Circuit Basics (4th Edition)_Explanation of basic circuit principles, introduction to electronic components, design of various circuits and practical circuit analysis
-
FPGA Principle and Structure (Zhao Qian)
-
EDA Technology Practical Tutorial--Verilog HDL Edition (Sixth Edition) (Pan Song, Huang Jiye)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- MSP430: Input Capture
- High Voltage Impedance Tuning Quick Guide
- K210 face recognition environment construction process
- Charge up! The latest generation of ACF UCC28782 ultra-small fast charging adapter!
- Proteus simulation experiment of single chip microcomputer communication
- 【TI recommended course】#Power system design tools#
- Question about username
- NeoPixel simulates fluid physics motion
- BMP library management based on FPGA.pdf
- Making some preparations for the flag I set up on the forum this year (first time shopping at the ghost market)