Use the program to practice data transfer instructions
Data transfer instructions between the accumulator A of the microcontroller and the external RAM
MOVX A,@Ri
MOVX@Ri,A
MOVX A,@DPTR
MOVX @DPTR,A
Note:
1) In the 51 series MCU, the only accumulator that interacts with the external RAM is the A accumulator. All data that needs to be transferred to the external RAM must be sent through A, and all data that needs to be read from the external RAM must also be read through A. Here we can see the difference between the internal and external RAM. The internal RAM can directly transfer data, but the external RAM cannot. For example, to send a unit in the external RAM (set to the data of the 0100H unit) to another unit (set to the 0200H unit), the content of the 0100H unit must first be read into A, and then transferred to the 0200H unit.
To read or write external RAM, of course, you must know the address of the RAM. In the last two MCU instructions, the address is directly placed in DPTR. In the first two instructions, since Ri (i.e. R0 or R1) is only an 8-bit register, only the lower 8-bit address is provided. Because sometimes the number of extended external RAM is relatively small, less than or equal to 256, it is enough to provide only 8-bit address.
When using it, the address to be read or written should first be sent to DPTR or Ri, and then the read or write command should be used.
Example: Send the content in the 100H unit in the microcontroller's external RAM to the 200H unit in the external RAM.
MOV DPTR, #0100H
MOVX A, @DPTR
MOV DPTR,#0200H
MOVX @DPTR,A
Program memory transfers instruction to accumulator A
MOVC A, @A+DPTR This instruction is to send the number in ROM to A. This instruction is also called the microcontroller table lookup instruction, which is often used to look up a table that has been prepared in ROM. Description:
This instruction introduces a new addressing method: variable addressing. This instruction is to find data in an address unit of ROM. Obviously, the address of this unit must be known. The address of this unit is determined as follows: there is a number in DPTR and a number in A when executing this instruction. When executing the instruction, add the numbers in A and DPTR to get the address of the unit to be found.
The search result is placed in A, so the value in A may not be the same before and after the execution of this instruction.
Example: There is a number in R0, and you need to use a table lookup to determine its square value (the value range of this number is 0-5)
MOV DPTR, #TABLE
MOV A, R0
MOVC A, @A+DPTR
TABLE: DB 0,1,4,9,16,25
Assume that the value in R0 is 2, and is sent to A, and the value in DPTR is TABLE, then the address of the ROM unit finally determined is TABLE+2, that is, the number is taken from this unit, and the number is 4, which is obviously 2 squared. The same can be said for other data.
The real meaning of the label: From this point, we can also see another problem. We use labels to replace specific unit addresses. In fact, the real meaning of the label is the address value. Here it represents the starting position of the data 0, 1, 4, 9, 16, 25 stored in the ROM. In the LCALL DELAY microcontroller instruction we have learned before, DELAY represents the starting address of the program labeled DELAY stored in the ROM. In fact, the CPU finds this program through this address.
You can look at the meaning of the labels through the following routine:
MOV DPTR, #100H
MOV A, R0
MOVC A, @A+DPTR
ORG 0100H.
DB 0,1,4,9,16,25
If the value in R0 is 2, the final address is 100H+2, which is 102H, and the value found in unit 102H is 4. Do you understand this?
Why not write the program like this, using labels? Doesn't it just add to the confusion?
If we write a program in this way, we must determine the specific location of this table in the ROM when writing the program. If we want to insert another program before this program after writing the program, the location of this table will change again, and we have to change the sentence ORG 100H. We often need to modify the program, which is very troublesome, so we use labels instead. As long as the program is compiled, the position will change automatically. We leave this troublesome matter to the computer - referring to the computer we use.
Stack Operations
PUSH direct
POP direct
The first instruction is called push, which is to put the content in direct into the stack, and the second instruction is called pop, which is to put the content in the stack back into direct. The execution process of the push instruction is to first add 1 to the value in SP, then use the value in SP as the address, and put the value in direct into the RAM unit with the value in SP as the address. Example:
MOV SP, #5FH
MOV A, #100
MOV B, #20
PUSH ACC
PUSH B
The execution of the first PUSH ACC instruction is as follows: add 1 to the value in SP, which becomes 60H, and then send the value in A to the 60H unit. Therefore, after executing this instruction, the value of the 60H unit in memory is 100. Similarly, when executing PUSH B, SP+1 is added, which becomes 61H, and then the value in B is sent to the 61H unit. That is, after executing this instruction, the value in the 61H unit becomes 20.
The POP instruction is executed in the microcontroller as follows: first, the value in SP is used as the address, and the number in this address is sent to the direct following the POP instruction, and then SP is reduced by 1.
Continuing from the previous example:
POP B
POP ACC
The execution process is: use the value in SP (now 61H) as the address, take the value in the 61H unit (now 20), and send it to B, so after executing this instruction, the value in B is 20, then reduce SP by 1, so after this instruction is executed, the value of SP becomes 60H, then execute POP ACC, use the value in SP (60H) as the address, take the number from the address (now 100), and send it to ACC, so after executing this instruction, the value in ACC is 100.
What is the meaning of this? The value in ACC is originally 100, and the value in B is originally 20. Yes, in this example, it does not make sense, but in actual work, other instructions are usually executed after PUSH B, and these instructions will change the values in A and B. So at the end of the program, if we want to restore the values in A and B to their original values, then these instructions make sense.
Another question, if I don't use the stack, for example, use MOV 60H, A at the PUSH ACC instruction, use MOV 61H, B at the PUSH B instruction, and then use MOV A, 60H, MOV B, 61H to replace the two POP instructions, isn't it the same? Yes, it is the same in terms of the result, but it is different in terms of the process. The PUSH and POP instructions are single-byte, single-cycle instructions, while the MOV instruction is a double-byte, double-cycle instruction. What's more, the role of the stack is more than that, so there is a stack on general computers, and the same is true for microcontrollers. When we write subroutines and need to save data, we often do not use the latter method, but use the stack method to achieve it.
Example: Write out the running results of the following MCU program
MOV 30H, #12
MOV 31H, #23
PUSH 30H
PUSH 31H
POP 30H
POP 31H
As a result, the value in 30H becomes 23, and the value in 31H becomes 12. The data is exchanged. From this example, we can see that when using a stack, the order of writing into the stack and the order of writing out of the stack must be opposite to ensure that the data is sent back to the original position, otherwise an error will occur.
Homework: Execute the above routine under MCS51 and pay attention to the changes in the memory window and stack window.
Previous article:51 MCU Tutorial from Scratch - 10 Data Transfer Instructions
Next article:51 MCU Tutorial from Scratch - 12 MCU Arithmetic Instructions
Recommended ReadingLatest update time:2024-11-17 12:24
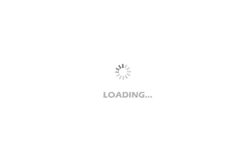
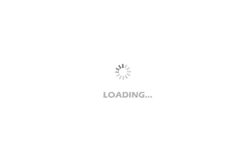
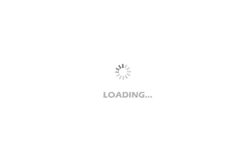
- Popular Resources
- Popular amplifiers
-
Wireless Sensor Network Technology and Applications (Edited by Mou Si, Yin Hong, and Su Xing)
-
Modern Electronic Technology Training Course (Edited by Yao Youfeng)
-
Modern arc welding power supply and its control
-
Small AC Servo Motor Control Circuit Design (by Masaru Ishijima; translated by Xue Liang and Zhu Jianjun, by Masaru Ishijima, Xue Liang, and Zhu Jianjun)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- New breakthrough! Ultra-fast memory accelerates Intel Xeon 6-core processors
- New breakthrough! Ultra-fast memory accelerates Intel Xeon 6-core processors
- Consolidating vRAN sites onto a single server helps operators reduce total cost of ownership
- Consolidating vRAN sites onto a single server helps operators reduce total cost of ownership
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- Introduction to the factors that cause the switching power supply loop instability
- Sonoff WiFi Smart Socket Network Service
- How to convert a DC 5V power supply into ±12V
- [Repost] Understand the difference between PCB and integrated circuit in one article
- SPWM wave generation tool
- How to force the switch state of TI TMS320F28335 EPWM by software
- "Intel SoC FPGA Learning Experience" + My Implementation Method of GDBServer in Lesson 5
- EEWORLD University Hall----Live Replay: The Development and Latest Application of TTI TE Sensors in Industrial Motors
- ISO's simple classification of RFID series standards
- C6455 CSL_EMIF example