Use applet to understand what parallel port structure is
Last two times we did two experiments, both of which used the pin P1.0 to turn on the light. We can imagine: since P1.0 can turn on the light, can other pins not? Take a look at Figure 1 , which is the description of the pins of the 8031 microcontroller. Next to P1.0 are P1.1, P1.2...P1.7. Can they all turn on the light? In addition to those starting with P1, there are also those starting with P0, P2, and P3. Count them, there are 32 pins in total. We have learned 7 pins before, and with these 32, there are 39. They all start with the letter P, but the numbers after them are different. Is there any connection between them? Can they all turn on the light? On our experimental board, in addition to P10, there are also P11 -> P17, which are all connected to the LED. Let's do an experiment below. The procedure is as follows:
MAIN: MOV P1,#0FFH
LCALL DELAY
MOV P1,#00H
LCALL DELAY
LJMP MAIN
DELAY:MOV R7,#250
D1:MOV R6,#250
D2:DJNZ R6,D2
DJNZ R7,D1
RET
END
Convert this program into machine code and use a programmer to write it into the microcontroller. What is the result? After power is turned on, we can see that all 8 LEDs are flashing. Therefore, P10->P17 can all light up the lamp. In fact, all 32 pins starting with P can light up the lamp, that is to say: these 32 pins can be used as outputs. If they are not used to light up LEDs, they can be used to control relays and other actuators.
Program analysis: This program is different from the previous one in only two places: the first sentence: originally SETB P1.0, now changed to MOV P1, #0FFH, the third sentence: originally CLR P1.0, now changed to MOV P1.0, #00H. It can be seen that P1 is the representative of P1.0->P1.7 as a whole, and one P1 represents all eight pins. Of course, the instruction used is also different, using the MOV instruction. Why use this instruction? Look at Figure 2, we take P1 as a whole, and treat it as a memory unit. To send a number to a unit, we can use the MOV instruction.
2. The fourth experiment
In addition to being used as output, what else can these 32 pins do? Let's do a microcontroller experiment. The source code is as follows:
MAIN: MOV P3, #0FFH
LOOP: MOV A, P3
MOV P1, A
LJMP LOOP
Let's look at the result of this experiment: all lights are off, then I press a button, and the (1st) light comes on, then I press another button, and the (2nd) light comes on, and when I release the button, the light goes off. Let's analyze the program based on this experimental phenomenon and the circuit.
From the wiring of the hardware circuit, we can see that there are four buttons connected to P3 port P32, P33, P34, P35. We can guess the purpose of the first instruction: to make all P3 ports high level. The second instruction is MOV A, P3. As we know, MOV means to send a number. This instruction means to send the number in P3 port to A. We can regard A as an intermediate unit (see Figure 3). The third sentence is to send the number in A to P1 port again. The fourth sentence is a loop, which is to repeat this process continuously. We have seen this. When we press the first button, the (3)th light is on, so P12 port should output a low level. Why does P12 port output a low level? Let's see what is sent to P1 port. Only the number from P3 port is sent to A and then sent to P1 port. Therefore, it must be the number from P3 port that makes P12 output a high level. The button of the P32 bit of the P3 port is pressed, making the level of the P32 bit low. Through the program, the P12 port outputs a low level, so the P3 port plays an input role. Verification: Pressing the second, third, and fourth buttons, and pressing 2, 3, and 4 buttons at the same time can get the same conclusion, so the P3 port does play an input role. In this way, we can see that the pins starting with the letter P can be used not only as outputs, but also as inputs. Can other pins be used? Yes, they can. These 32 pins are called parallel ports. Let's analyze the structure of the parallel port and see how it realizes input and output.
Parallel port structure analysis:
1. Output structure
First, let's look at the structural diagram of one bit of P1 port (only the output part is drawn): As can be seen from the figure, the opening and closing of the switch represents the high and low output of the pin. If the switch is closed, the pin output is low, and if the switch is opened, the output is high. This switch is controlled by a line. This data bus comes from the CPU. Let's recall that the data bus is a common line for everyone. Many devices are connected to it. At different times, different devices certainly need different signals. For example, at a certain moment, we let this pin output a high level and require it to be maintained for a certain period of time. During this period of time, the computer is of course busy and communicating with other devices. The level on this control line may not be able to maintain the original value, and the output will change. How to solve this problem? We learned in the memory section that the memory can store charges. We might as well add a small memory unit and add a switch in front of it. When this bit is to be output, turn on the switch, the signal enters the memory unit, and then immediately turn off the switch. In this way, the state of this bit is saved until the next command to turn the switch on again. In this way, the state of this bit is independent of other devices. We give such a small unit a very vivid name, called "latch".
2. Input structure
This is a schematic diagram of the output structure of a parallel port. In addition to the output, there are two lines, one connected from the external pin and the other connected from the output of the latch, marked as read pin and read latch respectively. These two lines are used to receive signals from the outside, why two? It turns out that there are two ways to input in the 51 microcontroller, called "read pin" and "read latch". The first way is to use the pin as input, which is to actually read the input value from the external pin. The second way is that when the pin is in the output state, sometimes the state of this bit needs to be changed. In this case, it is not necessary to actually read the pin state, but only read the state of the latch, and then make some changes before outputting.
Please pay attention to the input structure diagram. If this lead is used as an input port, we cannot guarantee that we can get the correct result at any time (why?). Refer to the input diagram in Figure 2. If the switch connected to the outside is open, it should be input 1, and if the switch is closed, it should be input 0. However, if the switch inside the microcontroller is closed, then no matter whether the external switch is open or closed, the data received by the microcontroller is 0. It can be seen that in order to use this port as an input, a "preparation" must be done first, that is, to disconnect the internal switch first, that is, to let the port output "1". Because of this preparation, we call it a "quasi-bidirectional I/O port".
The above is the structure of one bit of port P1. The structures of the other bits of port P1 are the same. The other three ports: P0, P2, and P3 have other uses besides input and output, so the structure is slightly more complicated, but the structure for input and output is the same. See the figure (). For us, these additional functions do not need to be controlled by us, so we don't care about it.
Previous article:51 MCU Tutorial from Scratch - Part 6 Delay Program Analysis
Next article:51 MCU Tutorial from Scratch - Part 8 Special Function Registers
Recommended ReadingLatest update time:2024-11-16 15:50
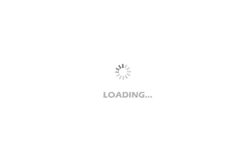
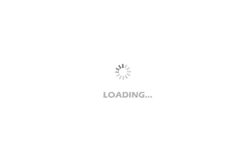
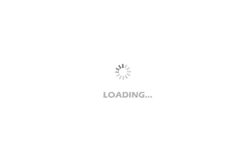
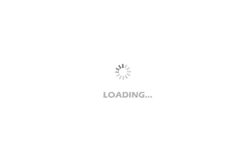
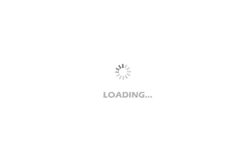
- Popular Resources
- Popular amplifiers
-
Wireless Sensor Network Technology and Applications (Edited by Mou Si, Yin Hong, and Su Xing)
-
Modern Electronic Technology Training Course (Edited by Yao Youfeng)
-
Modern arc welding power supply and its control
-
Small AC Servo Motor Control Circuit Design (by Masaru Ishijima; translated by Xue Liang and Zhu Jianjun, by Masaru Ishijima, Xue Liang, and Zhu Jianjun)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- How to Make DSP Digital Oscillator Generate Phase-Shifted Sine Wave
- About the problem of modifying the frequency offset of CC2650 chip
- Zigbee packet capture analysis - Introduction
- About STM32 sampling pressure sensor value
- Open source Linux phone designed with KiCad
- MSP430F5529 MCP4725 Program
- [STM32WB55 Review] This Nucleo is a little special
- HIFU ultrasonic knife (high energy focusing control circuit)
- ARM transplantation encounters a long wait for a solution
- Single bus temperature sensor DS18B20 reading and writing example