introduction
It is relatively simple to implement a voice recorder using a microcontroller (MCU). Many MCUs have integrated analog-to-digital (A/D) converters. The microphone provides the captured sound to an amplifier, which then feeds the analog input of the A/D converter. The recorded sound can be stored in a memory such as flash or RAM, and the MCU can be triggered to play the recorded sound by pressing a button, which feeds the stored data to a digital-to-analog (D/A) converter and then to the audio power amplifier.
Such a voice recorder can be easily implemented using MSP430. The MSP430 microcontroller uses integrated peripherals to implement the analog signal chain on-chip. In addition, the CPU processing power of the MSP430 is powerful enough to perform the compression of the recorded sound.
Compression and decompression algorithms
For example, the simplest way to implement a voice recorder is to store the A/D converter results (e.g. 12-bit samples) directly in flash memory. Most of the time, the audio data does not use the entire A/D converter range, which means that redundant data is also stored in flash memory. Compression algorithms can remove this redundant information, thereby reducing the size of the stored data.
Adaptive Differential Pulse Code Modulation (ADPCM) is an example of this type of compression algorithm. There are various types of ADPCM algorithms, but all use differential coding of the quantizer and an adaptive quantization step size scheme in the quantizer. Before further discussing the IMA ADPCM algorithm for related codes, we first briefly introduce differential PCM coding.
Differential Pulse Code Modulation (DPCM)
DPCM encodes the analog audio input signal by using the difference between the current sample and the previous sample. Figure 1 shows the block diagram of the DPCM encoder and decoder. In this case, we use the signal estimate Se(n) instead of the previous input to determine the signal difference d(n), ensuring that the encoder uses the same information as the decoder. If the encoder uses the previous input sample, it will cause quantization errors to accumulate, making the reconstructed signal different from the original input signal. By using the signal estimate as shown in Figure 1, we can avoid the reconstructed signal Sr(n) from differing from the original input signal. The reconstructed signal Sr(n) is the input to the predictor, which determines the next signal estimate Se(n+1).
Figure 2 shows a small section of a recorded audio stream and two diagrams comparing the difference between the analog audio input samples (PCM values) and the successive samples (DPCM values).
The range of PCM values is between 26 and 203, for a total of 177 steps. The range of encoded DPCM values is between -44 and 46, for a total of 90 steps. Even with a quantizer step size of only 1, this DPCM encoding already compresses the input data. The range of encoded DPCM values can be further reduced by simply choosing a larger quantizer step size.
Adaptive Differential Pulse Code Modulation (ADPCM)
ADPCM is a variation of DPCM with a different encoder step size. The strength of the speech input signal varies across different speakers and also across the speech and non-speech parts of the speech input signal. The quantizer step size is adaptively adjusted for each sample to ensure the same coding efficiency for both high and low input signal strengths. Figure 3 shows the block diagram of the revised DPCM with step size adjustment.
The ADPCM encoder performs signal estimation (Se) by decoding the ADPCM code, that is, the decoder is part of the ADPCM encoder, so the encoded audio data stream can only be replayed using the decoder, so the decoder must track the encoder.
The initial encoder and decoder signal estimation levels and step size adjustment levels must be defined before starting encoding or decoding, otherwise the encoded or decoded values will be out of range.
MSP430 On-Chip Signal Chain
The MSP430 series of microcontrollers supports a variety of on-chip peripherals. In order to achieve a complete on-chip signal chain solution, the MSP430 must provide at least 1 A/D converter analog input and 1 D/A converter. Below we will introduce two MSP430 solutions.
MSP430F169 Signal Chain Solution on a Chip
The MSP430F169 includes an integrated 12-bit SAR A/D converter, which is a hardware multiplier module that can efficiently support digital filters. In addition, the MSP430F169 also includes an integrated 12-bit D/A converter module. Figure 4 shows the MSP430F169 signal chain circuit diagram.
The above configuration is also suitable for the case of using external serial flash memory, so as to meet the storage requirements of audio data. The external flash memory can be connected through the I2C or SPI interface of MSP430. The MSP430F169 DMA module can automatically transfer the received data to RAM, thus greatly reducing the CPU load.
MSP430FG4618 Signal Chain Solution on a Chip
We can implement another on-chip signal chain solution with the MSP430FG4618. The MSP430F169 supports 60 KB of integrated flash memory, while the MSP430FG4618 supports 116 KB of flash memory. Another advantage of the MSP430FG4618 is that it also integrates an operational amplifier module. The operational amplifier can be used to amplify the input of the amplifier and the analog output of the digital-to-analog converter. Figure 5 shows the MSP430FG4618 signal chain circuit diagram. The specific configuration uses the MSP430FG4618/F2013 experiment board launched by TI. This evaluation board can be used with related code examples.
The output signal of the microphone is very small and must be amplified. The operational amplifiers of the MSP430 can be used in different operation modes. If used in PGA mode, the maximum amplification can only be 15 times, which is not enough for the microphone amplifier. Therefore, it is necessary to increase the gain through external components. The operational amplifier OA0 in Figure 5 is used in the general amplifier mode. The amplifier has 8 settings to achieve the best balance between performance such as gain-bandwidth product and conversion rate and current consumption. All amplifiers OA0, OA1 and OA2 in the figure use the high-performance mode (fast mode).
For more details on the use of the op amp, see the MSP430FG4618/F2013 Experimenter Board User's Guide.
The audio data can be stored in external flash memory using the Universal Serial Communication Interface (USCI). We can also connect to external memory via I2C bus or SPI bus.
MSP430 Performance
There are some sample *.wav files in the associated code files that show the quality of decoded ADPCM data. We can compare these files on a PC using software such as a media player, so that we can experience the actual quality of the ADPCM compression algorithm. Please note that we can further improve the audio quality by increasing the audio sampling rate and the audio sample size (resolution).
Use the relevant code
The related code contains two software projects. Both versions are based on the content introduced in Part 3 and both use the IMA ADPCM algorithm.
The use of ADPCM functions is very simple. First, the ADPCM.h header file must be included in the application code. This header file defines the ADPCM functions of the ADPCM.c file. Before each recording or playback of audio data, the ADPCM_Init() function must be called. This function defines the starting value of the signal estimate (Se) and the step pointer used for quantizer step adjustment. The encoder and decoder can be synchronized through settings. Encoding can be performed by calling the ADPCM_Encoder (int value) function, and playback can be performed by calling the ADPCM_Decoder() function for each audio sample. The following code snippet shows how to complete the above work.
#include "ADPCM.h"
void main(void)
{ // Application software initialization
while(1) // Main loop
{ // Application software
if (P1IN & 0x01)
record();
if (P1IN & 0x02)
play();
}
}
void record(void)
{ // Initialize to allow recording of A/D converter, timer, amplifier, etc.
ADPCM_Init(); // Must be done before recording starts
// Start recording
}
void play(void)
{ // Initialize to allow recording of A/D converter, timer, amplifier, etc.
ADPCM_Init(); // Must be done before recording starts
// Start playback
}
Next, we use IAR Embedded Workbench KickStart version 3.42A to measure the number of times the ADPCM function is executed. The default optimization settings are used for the measurement.
The ADPCM_Encoder() function call requires 114 to 126 cycles.
The ADPCM_Decoder() function call requires 99 to 109 cycles.
Note that this only covers the compression/decompression algorithms. To implement the recording and playback functionality, more code is required.
References:
1. MSP430x4xx Family User's Guide (SLAU056)
2. MSP430F169 Product Manual (SLAS368)
3. MSP430FG4618 Product Manual (SLAS508)
4. 32kbps ADPCM (SPRA131) based on TMS32010
5. Low-cost 12-bit speech codec design based on MSP430F13x (SLAA131)
6. MSP430FG4618/F2013 Experimenter Board User Guide (SLAU213)
Previous article:Proteus virtual simulation software for single chip microcomputer
Next article:Communication method between single chip microcomputer and eView touch screen under Modbus protocol
Recommended ReadingLatest update time:2024-11-16 19:36
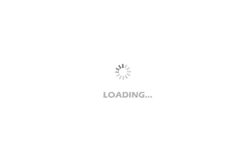
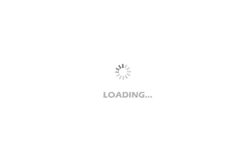
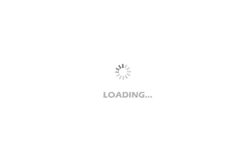
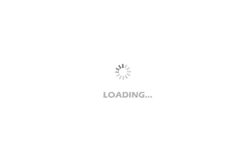
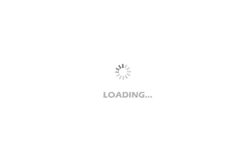
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- NUCLEO_G431RB review->File structure & ST-Link online debugging experience
- Sugar Glider ⑤ Sugar Glider Health Monitoring System Based on RSL10--Project Introduction
- Problems with using the bq28z610EVM development board
- How to create a transcoding table using HZK16 font in micropython?
- 【TI recommended course】#What is I2C design tool? #
- Digital Input Closed-Loop Class-D Audio Amplifier Evaluation Module
- Come and recommend a book!!! April is a great time to read when the flowers are blooming and the grass is green!
- [GD32L233C-START Review] 4. USART uses idle interrupts and DMA to receive data of variable length
- How to convert orcad library into pads library?
- [RVB2601 Creative Application Development] + Portable Instrument Cloud Data System