When developing embedded systems, the binary code of the target platform is usually generated on the host machine through cross-compilation, and then written into the target machine for execution. One disadvantage of this development method is that it is not easy to debug the generated target code logic. Therefore, many cross-compilation tools currently have the function of debugging source code on the host machine. In order to enable the binary code of the target platform to run on the host machine, a virtual system that can execute the target machine instructions must be provided. This paper takes the 80C51 microcontroller as the target machine and the PC based on the X86 platform as the host machine, and gives a method for constructing a virtual target machine instruction execution system on the host machine.
1 Introduction to the virtual instruction execution system
The 80C51 virtual instruction execution system described in this paper refers to the use of software to simulate the execution process and execution effect of 80C51 instructions. It is mainly composed of a virtual instruction executor and a virtual memory. The virtual instruction executor is the core module of the virtual instruction execution system. It divides the execution process of instructions into three stages: fetching instructions, analyzing instructions, and executing instructions. It simulates the operations of these three stages and virtualizes the execution effect of instructions. The virtual storage system is an essential module of the virtual instruction execution system. It reflects the effect of the virtual instruction executor executing instructions. Based on the memory structure in the 80C51 system, this paper virtualizes the memory space and registers, and provides an interface for the virtual instruction executor to access the virtual memory.
Figure 1 is the overall structure of the virtual 80C51 instruction execution system. At the same time, Figure 1 also shows the three basic processes of the system operation:
(1) Load the binary file into the ROM of the virtual memory
(2) The virtual instruction executor periodically fetches instructions from the ROM of the virtual memory, analyzes the instructions and executes the instructions
(3) During the execution of the instructions, the execution effect of the instructions is reflected by reading and writing the memory and registers in the virtual memory
Obviously, the above process is carried out around the virtual instruction executor and the virtual memory.
2 Design and implementation of virtual memory
Both the loading process and the virtual instruction executor rely on the virtual memory, so it is necessary to first introduce the implementation of the virtual memory. From the perspective of access, registers and memory have the same properties, and similar implementation methods can be used to virtualize them. The scope of virtual memory in this article includes virtual storage space and registers.
2.1 Virtual 80C51 storage space
In addition to ROM and RAM, the storage space of 80C51 is also divided into on-chip and off-chip. The data accessed by the 80C51 instruction during execution can exist in the following four types of storage units: on-chip ROM, off-chip ROM, on-chip RAM, and off-chip RAM. Their address spaces are shown in Table 1.
[page]
The capacity of each storage space is small, and the storage space can be virtualized by opening up different arrays of corresponding sizes:
In addition to virtualizing the storage space, the virtual memory also provides access interfaces: read storage units and write storage units. When reading and writing storage units, it is necessary to indicate the type of storage unit.
Using these two interfaces, the virtual instruction executor can easily access the virtual memory when executing instructions.
2.2 Virtual 80C51 Registers
The registers of 80C51 can be divided into three categories: special registers (SFR), working registers (R0~R7), and program counter (PC). The virtual system has different virtual methods and access methods for these three registers.
1) Virtualization and access of special registers
The address space range of the special registers of 80C51 is 0x80~0xFF. Each special register has a certain address in this address space. From a virtual perspective, it can be considered that special registers and RAM have similar access characteristics. Therefore, the virtual memory method can be used to virtualize special registers:
In this way, the read and write interfaces provided by the virtual memory can be used to access special registers. [page]
2) Virtualization and access of working registers
Unlike special registers, the addresses of working registers R0~R7 are uncertain during instruction execution. Their addresses are determined by the values of RS1 and RS0 bits in special register PSW, and their physical addresses occupy the address space of on-chip RAM, see Table 2. Figure 2 shows the process of accessing working register Rn.
3) Virtualization and access
of PC register The program counter PC of 80C51 is a 16-bit register, which is changed by CPU during instruction execution. The PC register is transparent to the user, that is, the PC register is not mapped to the address space of the memory, and the PC cannot be accessed by the read-write interface of the virtual memory. It is necessary to virtualize a 16-bit PC register separately and provide a read-write interface:
[page]
Generally, the CPU is always fetching instructions, analyzing instructions, and executing instructions. The virtual instruction executor performs the same process. It simulates the operations of the above three stages as the core and simulates the execution effect of each instruction in the 80C51 instruction system. Figure 4 shows the overall design of the virtual instruction executor.
It can be seen that the workflow of the virtual instruction executor is divided into three stages: instruction fetching stage, instruction analysis stage and instruction execution stage.
3.1 Instruction fetching stage
The virtual instruction execution system fetches the instruction to be executed from the virtual ROM according to the value of PC in each cycle. Before the virtual instruction executor executes the instruction, the binary file (hex file format) on the hard disk must be loaded into the virtual ROM as the input of the instruction executor. The loading process is completed as described below: parse the content of the binary file and store the instruction at the specified ROM address. After loading, the value of the PC register needs to be set to the address of the first instruction to be executed. The length of the instruction
fetched by the virtual instruction execution system each time is uncertain. The instruction length of 80C51 is not fixed, and it is divided into three types: single-byte, double-byte and three-byte instructions. Therefore, when fetching an instruction, the length of the instruction needs to be determined. By analyzing the instruction table of 80C51, it can be found that the first byte of any instruction is unique. Using this feature, a mapping table from the byte to the instruction length can be built. When fetching an instruction, first fetch the first byte from the address pointed to by the PC, and then fetch the corresponding number of bytes by querying the mapping table. After the instruction is successfully fetched, the PC value needs to be updated so that the PC points to the address of the next instruction.
For use in the subsequent two stages, the fetched instructions need to be stored in a buffer. The longest instruction of 80C51 is 3 bytes, so a 3-byte array can be used to store the fetched instructions, such as unsigned char inst, and the fetched instructions are filled into inst in order.
3.2 Instruction Analysis Stage
An instruction includes an opcode and an operand. The opcode determines what action the instruction performs on the operand. The instruction analysis stage needs to parse the opcode and operand from the fetched instruction. The parsing process is as follows: assuming that the instruction has been fetched into inst, from the perspective of the virtual instruction executor, the first byte of the instruction can be considered as the opcode, that is, inst[0]. The operands are stored in inst[1] and inst[2] respectively. The number of operands is determined according to the mapping table from opcode to instruction length.
After parsing the instruction code and operand, it is necessary to call the execution function corresponding to the instruction according to the opcode. Each instruction has its own execution action, and an execution function can be designed for each instruction to simulate the execution action of the instruction. Instruction opcodes and instruction execution functions also correspond one to one, so there will be a mapping table from instruction opcodes to instruction execution functions in the virtual system. After analyzing the opcode of the instruction, the execution function of the instruction can be quickly called to simulate the semantic action of the instruction. For example, the instruction fetched is e580. According to the previous description, 0xe5 is the opcode, which is stored in inst[0], and 0x80 is the operand, which is stored in inst[1]. By checking the instruction table, it can be known that the assembly instruction corresponding to 0xe5 is MOV A, direct. An execution function mov_a_direct0 is designed for this instruction. When the virtual instruction execution system executes instruction e580, it actually calls its execution function mov_a_direct 0.
3.3 Execution instruction stage
The execution action of the instruction is completed by calling the execution function of the instruction. The execution function of an instruction is a simulation of the actual execution action of the instruction, mainly including the access to registers and memory, and the impact on the flag bit in the program status word. For example, the instruction with the opcode 0xe5 introduced above has the assembly instruction MOV A, direct, which means storing the memory number in direct in register A and changing the P flag bit in PSW. The execution function mov_a_direct 0 of this instruction is actually the implementation of the above description. The 80C51 instruction
system has a total of 111 instructions, and the virtual instruction executor designs a similar execution function for each instruction. These instructions can be divided into several categories: data transfer instructions, arithmetic operation instructions, logical operations and shift instructions, control transfer instructions, and bit operation instructions. There are some things to pay attention to when designing the execution function: arithmetic operation instructions need to pay attention to the impact of the operation on the flag bit of PSW, and control transfer instructions need to accurately change the value of the PC register.
4 Conclusion
This article introduces the design and implementation of the virtual 80C51 instruction execution system in detail. The method given in the article is also applicable to the microcontroller instruction system with storage space and instruction scale similar to 80C51. The virtual instruction execution system is the basis of other functions of the virtual target machine and has a wide range of applications. For example, a target machine code debugger based on the virtual instruction execution system can be constructed. In addition, according to application needs, the virtual scope of the 80C51 microcontroller can also be increased, such as realizing the virtualization of interrupts and I/O.
Previous article:Design of portable amplitude-frequency characteristic tester based on MC8051 core
Next article:Design of multi-channel data acquisition system based on ADC0809 and 51 single chip microcomputer
Recommended ReadingLatest update time:2024-11-16 19:50
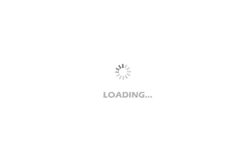
- Popular Resources
- Popular amplifiers
-
西门子S7-12001500 PLC SCL语言编程从入门到精通 (北岛李工)
-
Siemens Motion Control Technology and Engineering Applications (Tongxue, edited by Wu Xiaojun)
-
How to read electrical control circuit diagrams (Classic best-selling books on electronics and electrical engineering) (Zheng Fengyi)
-
MCU C language programming and Proteus simulation technology (Xu Aijun)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- EEWORLD University - Cadence Allegro 17.4 Quadcopter Full Zero-Based Introductory Course
- How to find MSP430 program examples on TI's official website
- Another board unboxing! This time it's the GigaDevice GD32307E-START development board
- FPGA Introduction Course 2-Counter
- Introduction to TI battery failure modes and BMS related solutions
- 2008 transcripts - review
- Help with the feedback control loop of the flyback switching power supply
- LPWAN Alternatives for IoT
- Greenhouse automatic spraying system ---- H743temperature sensor
- Hongmeng Development Board Neptune (Part 1) - Unboxing