1 DDS Principle
1.1 Explanation in the book
DDS (Direct Digital Synthesizer) technology is a new frequency synthesis method. It is a frequency synthesis technology that directly synthesizes the required waveform based on the phase concept. It is a frequency synthesis method that directly generates various frequencies and waveform signals by controlling the speed of phase change.
The heart of the system is the phase accumulator, whose contents are updated at every clock cycle (system clock). Each time the phase accumulator is updated, the digital word M stored in the Δ phase register is added to the number in the phase register. Assume that the numbers in the Δ phase register are 00…01 (i.e., M=1), and the initial contents of the phase accumulator are 00…00. The phase accumulator is updated at 00…01 (M=1) at every clock cycle. If the accumulator is 32 bits wide, it will take 2^32 (over 4 billion) clock cycles before the phase accumulator returns to 00…00, and the cycle will repeat.
The truncated output of the phase accumulator is used as the address of the sine (or cosine) lookup table. Each address in the lookup table corresponds to a sine
A phase point of a wave from 0° to 360°. The lookup table contains the corresponding digital amplitude information for a complete sine wave cycle.
(In reality, only the 90° data is needed because the two MSBs contain quadrature data.) Therefore, the lookup table can convert the phase
The phase information from the accumulator is mapped to a digital amplitude word, which in turn drives the DAC. Figure 3 shows this graphically as a “phase wheel.”
One situation.
Consider the case where n = 32 and M = 1. The phase accumulator will step through each of the 2^32 (2^n/M) possible outputs until it overflows and starts over. The corresponding output sine wave frequency is equal to the input clock frequency divided by 2^32. If M=2, the phase accumulator register will "roll" twice as fast and the output frequency will double. The above can be summarized as follows:
1.2 My own understanding
The DDS system is mainly composed of four large structures: phase accumulator, waveform memory, digital-to-analog (D/A) converter and low-pass filter. Its structural block diagram is shown in the figure below.
Reference Clock:
In the figure, the reference frequency f_clk is a fixed value. Generally, we choose the system clock, which is set to 100MHz here.
Frequency control word:
Used to adjust the frequency of the output signal. The official document of DDS IP provides the corresponding formula for how to get the output frequency based on the reference frequency.
Phase control word:
Phase Accumulator:
It consists of an N-bit adder and an N-bit accumulator register. It completes the accumulation of phase values according to the frequency control word k and inputs the accumulated value into the waveform memory.
Waveform memory:
The value of the phase accumulator is used as the current address to find the signal data corresponding to the phase value and output it to the D/A converter.
D/A Converter:
Convert the digital quantity output by the waveform memory into the corresponding analog quantity.
Low pass filter:
Due to the quantization error of the D/A converter, there is aliasing in the output waveform, and a low-pass filter is needed at the output to improve the output performance of the signal.
2 DDS IP parameter settings
It should be noted that the frequency resolution value above is calculated and must be consistent with the frequency resolution of summer. 100M/(2^20)=95.36743
3 Source Code
Program Structure
3.1 Top-level files
`timescale 1ns / 1ps
module top(
input sys_clk , // system clock
input rst_n , // system reset
input [1:0] key_PINC , //key corresponding to the frequency control word
input [1:0] key_POFF //Key corresponding to the phase control word
);
//-----------Frequency control word module
wire [23:0] PINC ; //frequency word
Fword_set Fword_set_inst(
//input
.clk (sys_clk ),
.rst_n (rst_n ),
.key_PINC (key_PINC ),
//output
.PINC (PINC )
);
//-----------Phase control word module
wire [23:0] POFF ; //Phase word
POFF_set POFF_set_inst(
//input
.clk (sys_clk ) ,
.rst_n (rst_n ) ,
.key_POFF (key_POFF ) ,
//output
.POFF (POFF )
);
//------------DDS module
//input
wire [0:0] fre_ctrl_word_en ;
//output
wire [0:0] m_axis_data_tvalid ;
wire [47:0] m_axis_data_tdata ;
wire [0:0] m_axis_phase_tvalid ;
wire [23:0] m_axis_phase_tdata ;
assign fre_ctrl_word_en=1'b1;
dds_sin dds_sin_inst (
.aclk (sys_clk ), // input wire aclk
.s_axis_config_tvalid (fre_ctrl_word_en ), // input wire s_axis_config_tvalid
.s_axis_config_tdata ({POFF,PINC} ), // input wire [47 : 0] s_axis_config_tdata
.m_axis_data_tvalid (m_axis_data_tvalid ), // output wire m_axis_data_tvalid
.m_axis_data_tdata (m_axis_data_tdata ), // output wire [47 : 0] m_axis_data_tdata
.m_axis_phase_tvalid (m_axis_phase_tvalid ), // output wire m_axis_phase_tvalid
.m_axis_phase_tdata (m_axis_phase_tdata ) // output wire [23 : 0] m_axis_phase_tdata
);
endmodule
3.2 Frequency Control Word Module
`timescale 1ns / 1ps
//
// Use the button to select the corresponding frequency control word, and then select the corresponding signal frequency
//
module Fword_set(
input clk ,
input rst_n ,
input [1:0] key_PINC ,
output reg [23:0] PINC
);
//always@(posedge clk or negedge rst_n)
//begin
// if(!rst_n)
// key_sel <= 4'd0;
// else
// key_sel <= key_sel;
//end
// The output frequency(f_out ) , of the DDS waveform is a function of the system clock frequency(f_clk ) .
// the phase width, that is, number of bits (B ) in the phase accumulator
// and the phase increment value (deta_theta) .
// The output frequency in Hertz is defined by:f_out=f_clk*deta_theta/(2^B)
// How is fre_ctrl_word determined?
//According to the summery of IP core, phase width=20bits Frequency per channel=100MHz
// Output frequency calculation formula f_out=f_clk*deta_theta/(2^B)=100M* 104857/(2^20 )= 10M
always@(*)
begin
case(key_PINC)
0: PINC <= 'h28f5; //1Mhz 10485 The value of each phase increase deta_theta
1: PINC <= 'h51eb; //2Mhz 20971
2: PINC <= 'ha3d7; //4Mhz 41943
3: PINC <= 'h19999; //10Mhz 104857
endcase
end
endmodule
3.3 Phase Control Word Module
`timescale 1ns / 1ps
//
// Use the button to select the corresponding phase control word, and then select the corresponding signal initial phase
/
module POFF_set(
input clk ,
input rst_n ,
input [1:0] key_POFF ,
output [23:0] POFF
);
//always@(posedge clk or negedge rst_n)
//begin
// if(!rst_n)
// key_sel <= 4'd0;
// else
// key_sel <= key_sel;
//end
//According to the summery of IP core, phase_width=20bits Frequency per channel=100MHz
// Output phase calculation formula: POFF=phase*phase_modulus/360
// phase: The phase you want to output, enter 0~360
// phase_modulus: The phase coefficient is 2^phase_width-1=2^20-1,
// phase_width is the phase width, which can be viewed in the summary of the generated IP
reg [8:0] phase; //0-360
always@(*)
begin
case(key_POFF)
0: phase <= 'h0; //0
1: phase <= 'h5a; //90
2: phase <= 'hb4; //180
3: phase <= 'h10e; //270
endcase
end
assign POFF=phase*1048575/360;
endmodule
3.4 testbench file
`timescale 1ns / 1ps
module sim_top;
reg sys_clk ;
reg rst_n ;
reg [1:0] key_PINC;
reg [1:0] key_POFF;
//Instantiate source file
top top_inst(
.sys_clk (sys_clk ),
.rst_n (rst_n ),
.key_PINC (key_PINC ),
.key_POFF (key_POFF )
);
initial
begin
//initialization
sys_clk=0;
rst_n=0;
key_PINC=2'd0;
key_POFF=2'd0; //30
#100
rst_n=1;
key_PINC=0; //1Mhz 10485 The value of each phase increase deta_theta
#4000
key_PINC=1; //2Mhz 20971
#4000
key_PINC=2; //4Mhz 41943
#4000
key_PINC=3; //10Mhz 104857
#4000
key_POFF=1; //60
#4000
key_POFF=2; //90
#4000
key_POFF=3; //180
end
// create clock;
always #5 sys_clk=~sys_clk; //Each time interval is 5ns, take an inversion, that is, the period is 10ns, so the frequency is 100MHz
endmodule
4 Results
Previous article:MATLAB Signal Generator Simulation
Next article:Design of a Simple DDS Signal Generator Based on FPGA
Recommended ReadingLatest update time:2024-11-16 20:35
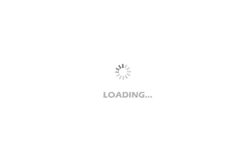
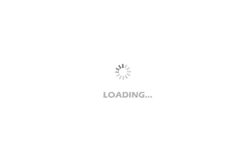
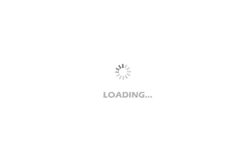
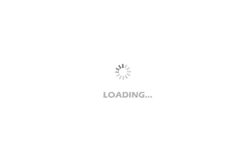
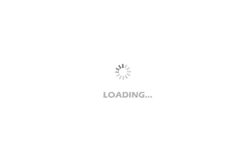
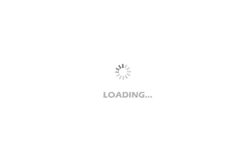
- Popular Resources
- Popular amplifiers
-
Analysis and Implementation of MAC Protocol for Wireless Sensor Networks (by Yang Zhijun, Xie Xianjie, and Ding Hongwei)
-
MATLAB and FPGA implementation of wireless communication
-
Intelligent computing systems (Chen Yunji, Li Ling, Li Wei, Guo Qi, Du Zidong)
-
Summary of non-synthesizable statements in FPGA
- Keysight Technologies Helps Samsung Electronics Successfully Validate FiRa® 2.0 Safe Distance Measurement Test Case
- From probes to power supplies, Tektronix is leading the way in comprehensive innovation in power electronics testing
- Seizing the Opportunities in the Chinese Application Market: NI's Challenges and Answers
- Tektronix Launches Breakthrough Power Measurement Tools to Accelerate Innovation as Global Electrification Accelerates
- Not all oscilloscopes are created equal: Why ADCs and low noise floor matter
- Enable TekHSI high-speed interface function to accelerate the remote transmission of waveform data
- How to measure the quality of soft start thyristor
- How to use a multimeter to judge whether a soft starter is good or bad
- What are the advantages and disadvantages of non-contact temperature sensors?
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- pybCN's UF2 bootloader
- [RVB2601 Creative Application Development] 6. Get weather information
- DIY Wireless Charging Cool Light
- USB Type-C and USB Power Delivery Power Path Design Considerations
- xds510 simulator transfer
- TVS Tube
- How to detect whether two points in a circuit are short-circuited to each other?
- How to handle different tasks at the same time in the time slice polling method
- Simple voice-controlled light circuit schematic and PCB wiring method
- LPS22HH air pressure sensor PCB package and code