Experiment 1 - Lighting up an LED
1. Look at the schematic to determine how the hardware is connected
The schematic diagram shows the hardware circuit of the chip controlling the LED and how the chip pins are connected to the LED.
2. Check the main chip manual to determine how to control the pins
Specific: How to make GPF4 output high and low levels?
2.1. Configure GPF4 to output mode (GPFCON)
Set bits [9:8] of the GPFCON register = 0b01.
2.2.Configure GPF4 output high/low level (GPFDAT)
The 4th bit of GPFDAT is 0-low level, 1-high level. (Note: corresponding)
3. Assembler program accesses registers to control LED
3.1. Editing Program
Code language: javascript
@ brief: Light up the LED connected to GPF4
@ author: mculover666
@ date: 2019/3/1
.text
.global _start
_start:
@ Set GPFCON register, GPF4 is output mode
LDR R0,=0x56000050
LDR R1,=0x0100
STR R1,[R0]
@ Set the GPF DAT register, GPF4 outputs low level
LDR R0,=0X56000054
LDR R1,=0
STR R1,[R0]
@Program Pause
halt:
B halt
3.2. Compiling the Program
Assemble to binary object file
Code language: javascript
arm-linux-gcc -c led_on.s -Wall -o led_on.o
Link to executable file elf
Code language: javascript
arm-linux-ld -Ttext 0 led_on.o -o led_on.elf
Convert to bin file
Code language: javascript
arm-linux-objcopy -O binary -S led_on.elf led_on.bin
The entire compilation step can be written as a makefile:
Code language: javascript
TARGET = led_on
# Output all warnings
CFLAGS = -Wall
$(TARGET).bin:$(TARGET).elf
arm-linux-objcopy -O binary -S $(TARGET).elf $(TARGET).bin
$(TARGET).elf:$(TARGET).o
arm-linux-ld -Ttext 0 $(TARGET).o -o $(TARGET).elf
$(TARGET).o:$(TARGET).s
arm-linux-gcc -c $(TARGET).s $(CFLAGS) -o $(TARGET).o
clean:
rm -rf *.o *.elf *.bin
download_to_nand:
#Download to nand flash
oflash 0 1 0 0 0 $(TARGET).bin
3.3. Burning Program
Use oflash to burn the bin file to address 0 of Nand Flash:
Code language: javascript
oflash 0 1 0 0 0 .led_on.bin
3.4. Run the program
-
Set the boot switch to Nand boot;
-
Re-power on;
-
Experimental Results
4.C program accesses registers to control LED
4.1. Prerequisites for running C programs - startup files
-
The C language entry function is the main() function, which is called by the startup file (the assembler program executed when power is just turned on);
-
The stack will be pushed when calling, and the stack will be popped after the call is completed, so the stack top pointer SP needs to be set;
-
After the main function is called, it returns to the startup file calling point.
Code language: javascript
Startup file start.s: Initialize the C language operating environment and introduce C programs
@ brief: S3C2440 startup file
@ author: mculover666
.text
.global _start
_start:
@ Disable watchdog
LDR R0,=0x53000000
MOV R1,#0
STR R1,[R0]
@ Set the stack top pointer SP (start from Nand)
LDR SP,=4096
@ Call the main function, save the return address, and transfer to the C program
BL main
@ The main function returns and the program pauses
halt:
B halt
4.2. Writing C Programs - Pointers + Bit Operations
Code language: javascript
int main(void)
{
/* Set the GPFCON register to configure the GPF4 pin as output mode*/
*(unsigned int *)0x56000050 &= ~(3<<(2*4));
*(unsigned int *)0x56000050 |= 1<<(2*4);
/* Set the GPF DAT register, GPF4 outputs low level, and lights up the LED */
*(unsigned int *)0x56000054 &= ~(1<<4);
/* Program pause */
while(1);
}
4.3. Compilation
Code language: javascript
TARGET = led_on
CFLAGS = -Wall # Output all warnings
$(TARGET).bin:$(TARGET).elf
arm-linux-objcopy -O binary -S $(TARGET).elf $(TARGET).bin
#Note: The startup file must be linked first
$(TARGET).elf:start.o $(TARGET).o
arm-linux-ld -Ttext 0 start.o $(TARGET).o -o $(TARGET).elf
$(TARGET).o:$(TARGET).c
arm-linux-gcc -c $(TARGET).c $(CFLAGS) -o $(TARGET).o
start.o:start.s
arm-linux-gcc -c start.s $(CFLAGS) -o start.o
clean:
rm -rf *.o *.elf *.bin
download_to_nand:
#Download to nand flash
oflash 0 1 0 0 0 $(TARGET).bin
4.4. Burn and run
Code language: javascript
copy
oflash 0 1 0 0 0 .led_on.bin
Experiment 2 - Key Detection
1. Look at the schematic to determine how the hardware is connected
2. Check the main chip manual to determine how to control the pins
2.1. Configure GPF0 to input mode (GPFCON)
2.2. Read the status of GPF0 (high/low level) (GPFDAT)
3.C program accesses register detection button
3.1. Programming
Code language: javascript
int main(void)
{
volatile int GPF0_state;
/* Set GPFCON register */
//Set GPF4 to output
*(unsigned int *)0x56000050 &= ~(3<<(2*4));
*(unsigned int *)0x56000050 |= (1<<(2*4));
//Set GPF0 as input
*(unsigned int *)0x56000050 &= ~(3<<(2*0));
/* Program infinite loop detection key */
while(1)
{
/* Read GPF DAT register */
GPF0_state = *(unsigned int *)0x56000054;
/* Check the status of GPF4 pin */
if(GPF0_state & 0x01)
{
//The button is not pressed, the pull-up resistor is pulled high, and the LED is turned off
*(unsigned int *)0x56000054 |= (1<<4);
}
else
{
//Button pressed, low level, light up the LED
*(unsigned int *)0x56000054 &= ~(1<<4);
}
}
}
3.2. Compiling the Program
Code language: javascript
TARGET = key_scan
CFLAGS = -Wall # Output all warnings
$(TARGET).bin:$(TARGET).elf
arm-linux-objcopy -O binary -S $(TARGET).elf $(TARGET).bin
#Note: The startup file must be linked first
$(TARGET).elf:start.o $(TARGET).o
arm-linux-ld -Ttext 0 start.o $(TARGET).o -o $(TARGET).elf
$(TARGET).o:$(TARGET).c
arm-linux-gcc -c $(TARGET).c $(CFLAGS) -o $(TARGET).o
start.o:start.s
arm-linux-gcc -c start.s $(CFLAGS) -o start.o
clean:
rm -rf *.o *.elf *.bin
download_to_nand:
#Download to nand flash
oflash 0 1 0 0 0 $(TARGET).bin
3.3. Burn the program and run it
Code language: javascript
oflash 0 1 0 0 0 .led_on.bin
Summary of Experiments 1 and 2
Through these two experiments:
-
1. In terms of the S3C2440 processor, we have mastered:
-
How to control the GPIO pins of S3C2440: output high and low levels and detect external input levels (GPFCON register and GPFDAT register)
-
5 commonly used ARM assembly instructions: MOV, LDR, STR, BL, B;
-
The basic format for writing assembler programs;
-
2. In terms of C language, I have mastered:
-
How is assembly converted to C language in the startup file: BL calls the main function;
-
Calling functions requires a lot of stack usage, which shows the importance of setting the stack pointer SP at startup;
-
Use C language pointers to access registers and use C language bit operation syntax to change register data;
-
3. In terms of development tools, we have mastered:
-
Use of arm-linux-gcc series tools and makefile;
Previous article:S3C2440⑤ | S3C2440 clock system architecture and experiments
Next article:S3C2440⑥ | UART Experiment
Recommended ReadingLatest update time:2024-11-25 05:20
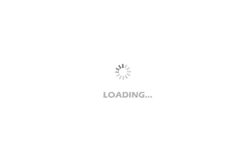
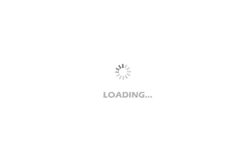
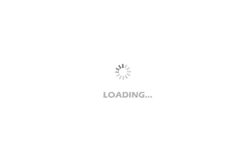
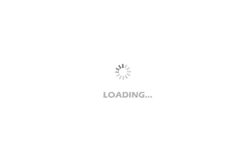
- Naxin Micro and Xinxian jointly launched the NS800RT series of real-time control MCUs
- How to learn embedded systems based on ARM platform
- Summary of jffs2_scan_eraseblock issues
- Application of SPCOMM Control in Serial Communication of Delphi7.0
- Using TComm component to realize serial communication in Delphi environment
- Bar chart code for embedded development practices
- Embedded Development Learning (10)
- Embedded Development Learning (8)
- Embedded Development Learning (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Intel promotes AI with multi-dimensional efforts in technology, application, and ecology
- ChinaJoy Qualcomm Snapdragon Theme Pavilion takes you to experience the new changes in digital entertainment in the 5G era
- Infineon's latest generation IGBT technology platform enables precise control of speed and position
- Two test methods for LED lighting life
- Don't Let Lightning Induced Surges Scare You
- Application of brushless motor controller ML4425/4426
- Easy identification of LED power supply quality
- World's first integrated photovoltaic solar system completed in Israel
- Sliding window mean filter for avr microcontroller AD conversion
- What does call mean in the detailed explanation of ABB robot programming instructions?
- CATL releases October battle report
- Battery industry in October 2024: growth momentum remains unabated!
- Mercedes-Benz will launch the eCitaro equipped with NMC4 batteries to provide high energy density and long life
- Many companies have announced progress on solid-state batteries. When will solid-state batteries go into mass production?
- Xsens Sirius Series Inertial Sensors Enable 3D Inertial Navigation in Harsh Environments
- Infineon's Automotive Landscape: From Hardware to Systems
- STMicroelectronics discloses its 2027-2028 financial model and path to achieve its 2030 goals
- 2024 China Automotive Charging and Battery Swapping Ecosystem Conference held in Taiyuan
- State-owned enterprises team up to invest in solid-state battery giant
- The evolution of electronic and electrical architecture is accelerating
- Allegro Ali Dog Drawing Board. I haven't drawn on the drawing board for a long time.
- BMW Night Vision (Infrared Thermal Imaging) Camera Disassembly
- stm32 usb cdc communication
- [ATmega4809 Curiosity Nano Review] Unboxing
- How to burn MSBL format firmware
- What is the reason for this voltage drop?
- Application of large color serial port screen in non-contact infrared thermometer
- MCS-51 microcontroller reads and writes USB flash drive (CH375 chip)
- [RISC-V MCU CH32V103 Review] Reinterpretation of USB read and write functions
- When using the MINI USB connector to power the board, are D+D- short-circuited or floating?