/* File name: UART.c
/* Function: The most basic UART sending and receiving
/* Author: Wujianqi
/* Version: 1.0
#include "2440addr.h" //Includes the settings of 2440 related registers
#include "def.h"
//The four LEDs correspond to GPB5.6.7.8.
#define LED1 5
#define LED2 6
#define LED3 7
#define LED4 8
#define BAUD 115200 //Baud rate
#define Bit(x) (1《x) //Set a bit
#define Output(x) (1《2*x) //Set the corresponding IO as output
#define LED_On(x) rGPBDAT=~Bit(x) //Turn on the corresponding LED
* Name: Clk_Set
* Function: About initialization of system clock
* Input parameter: None
* Output parameter: None
void Clk_Set(void)
{
int count;
rUPLLCON=(56《12)|(2《4)|2; //UCLK is 48MHZ
rMPLLCON=(92《12)|(1《4)|1; //FCLK is 400MHZ
rCLKDIVN=(0《3)|(2《1)|1;//HCLK is 100MHZ, PCLK is 50MHZ
rCAMDIVN=(0《9); //PCLK=HCLK/4
}
* Name: IO_init
* Function: Port initialization for LED
* Input parameters: None
* Output parameters: None
void IO_init(void)
{
rGPBCON=Output(LED1)|Output(LED2)|Output(LED3)|Output(LED4); //Set the IO port of LED to output
rGPBDAT=0xffff; //Turn off all LEDs
}
* Name: UART0_init
* Function: Initialization work related to UART0
* Input parameters: None
* Output parameters: None
void UART0_init(void)
{
rGPHCON=0xa0; //IO port enables UART0 function
rGPHUP=0xff; //Pull-up disabled
rULCON0=0x03; // 8-bit data, no parity, 1 stop bit
rUCON0=0x05; //pclk clock, interrupt request mode is Tx-level, Rx-pulse
rUBRDIV0=26; //Set baud rate
rUFCON0=0x00; //Do not use FIFO
rUMCON0=0x00; //Do not use flow control
}
* Name: Send_Byte
* Function: Send a character
* Input parameter: Waiting for the character to be sent
* Output parameter: None
void Send_Byte(char data)
{
while(!(rUTRSTAT0&0x2)); //Wait for the send buffer to be empty
rUTXH0=data;
}
* Name: Send_String
* Function: Send string
* Input parameter: Waiting for the string to be sent
* Output parameter: None
void Send_String(char* pt)
{
while(*pt)
{
Send_Byte(*pt++);
}
}
* Name: Uart_Getch
* Function: Receive a character
* Input parameter: None
* Output parameter: Received character
char Uart_Getch(void)
{
while(!(rUTRSTAT0&0x1)); //Wait for the receive buffer to have data
return (rURXH0); //Read out data
}
* Name: Main
* Function: Test UART sending and receiving functions
* Input parameter: None
* Output parameter: None
void Main(void)
{
char temp;
IO_init();
UART0_init();
Clk_Set();
Send_String("HelloWorld"); //Send string
while(1)
{
temp=Uart_Getch(); //Receive character
if(temp==0x01)
{
LED_On(LED1);
}
}
}
Previous article:Analysis of RTC clock driver development example on S3C2440
Next article:S3C2440 clock settings
Recommended ReadingLatest update time:2024-11-16 09:35
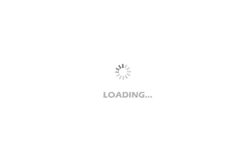
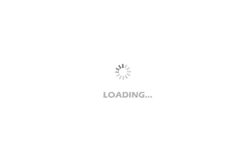
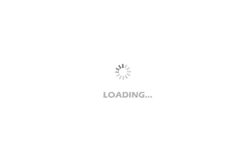
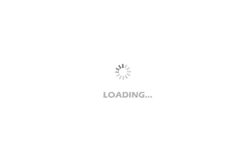
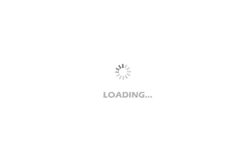
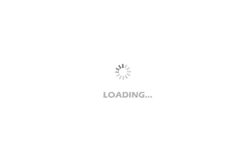
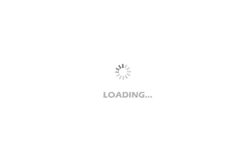
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- [Anxinke UWB indoor positioning module NodeMCU-BU01] 03. Transplantation preparation: hardware schematic analysis
- What happens when the motor suddenly stops running during operation?
- Vishay Online Library, technical information is waiting for you!
- [National Technology Low Power Series N32L43x Review] 02. Create a template project
- Altium Designer copper patch teardrop setting problem
- [Sipeed LicheeRV 86 Panel Review] 2- Board Resource Introduction and Data Collection
- How much do you know about fast charging? A collection of learning materials on fast charging technology and solutions
- Internal clock and RTOS system
- [Xianji HPM6750 Review 1] Experience with two IDE (SES and RS) development platforms
- BlueNRG-x Documentation - Copy and create your own projects