The 8051 microcontroller uses timer 1 working in mode 2 as the serial port baud rate generator. Its baud rate = (2smod /32) × (timer T1 overflow rate), where smod is PCON<7>, indicating whether the baud rate is doubled, and Fsoc is the system crystal size.
In the baud rate formula: T1 overflow rate = the inverse of the overflow period; overflow period = (256-TH1) × 12/Fosc;
Final formula:
Baud rate: Baud = (2smod × Fsoc) / (32 × 12 × (256-TH1))
We generally do not pay much attention to the calculation of the baud rate, but are concerned about the selected transmission speed (baud rate) to reversely calculate the initial value (TH1) of timer 1 (auto-reload mode), so we can derive the above formula to get the formula for TH1:
TH1=256-(Fsoc×2smod)/(12×32×Baud)
Below is a sample program for the serial port sending program, Shui Han wrote it down for your reference. (I used STC12C5A40S2 for debugging, in principle it can be successful on 8051 core microcontrollers such as STC89C5x and AT89C5x. Since the program is relatively simple, I did not try it, but it should be no problem)
#include "Reg52.H"
/****************************************************** ******************
Please calculate the maximum speed that the selected crystal can reach in advance. The baud rate cannot exceed the maximum speed.
(1) Double the baud rate (SMOD=1): Max_Baud = FOSC/12/16
(2) Baud rate is not doubled (SMOD=0): Max_Baud = FOSC/12/32
For example: 22.1184MHz crystal oscillator, when the baud rate is doubled, the maximum baud rate = 22118400/12/16 = 115200
*************************************************** *******************/
#define FOSC 22118400 //Oscillator frequency
#define BAUD 9600 //Baud rate
#define SMOD 1 //Whether to double the baud rate
#if SMOD
#define TC_VAL (256-FOSC/16/12/BAUD)
#else
#define TC_VAL (256-FOSC/32/12/BAUD)
#endif
typedef unsigned char uint8;
typedef unsigned int uint16;
code const char str1[] = "Ther string is transmitted from 80C51!rn";
code const char str2[] = "Author: xqlu(at)ysu.edu.cnrn";
/***************Function declaration********************/
void InitUART(void);
void SendOneByte(uint8);
void SendrStr(const uint8 *ptr);
/********************Main function************************/
void main(void)
{
uint8 i=0;
InitUART();
while(str2[i]!='')
{
SendOneByte(str2[i++]);
}
SendrStr(str1);
while(1);
}
/****************Interrupt service function****************/
void UART_ISR(void) interrupt 4
{
uint8 RX_Data;
//Only respond to the "receive" interrupt, and erase it directly when the "send" interrupt comes
if(RI)
{
RI = 0; //The serial port interrupt flag cannot be cleared by itself and needs to be cleared manually
RX_Data=SBUF;
SendOneByte(RX_Data);
}
else
TI = 0; //Serial port interrupt is generated after the buffer data is sent
}
/****************Serial port initialization function*************/
void InitUART(void)
{
TMOD = 0x20;
SCON = 0x50;
TH1 = TC_VAL;
TL1 = TH1;
PCON = 0x80; // double the transmission rate
ES = 1;
EA = 1;
TR1 = 1;
}
/**************Serial port sending character function*************/
void SendOneByte(uint8 c)
{
ES = 0; //Disable sending interrupt
SBUF = c;
while(!TI);
TI = 0;
ES = 1;
}
/**************Serial port sending string function*************/
void SendrStr(const uint8 *ptr)
{
do
{
SendOneByte(*ptr);
}while(*ptr++!='');
}
Previous article:51 single chip microcomputer principle and design scheme
Next article:Coexistence of Keil C51 and Keil MDK
Recommended ReadingLatest update time:2024-11-16 09:36
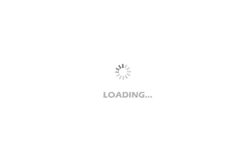
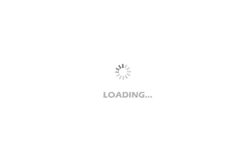
- Popular Resources
- Popular amplifiers
-
MCU C language programming and Proteus simulation technology (Xu Aijun)
-
Principles and Applications of Single Chip Microcomputers 3rd Edition (Zhang Yigang)
-
Principles and Applications of Single Chip Microcomputers and C51 Programming (3rd Edition) (Xie Weicheng, Yang Jiaguo)
-
STC32G Series MCU Technical Reference Manual
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- How to improve battery safety while increasing accuracy and runtime
- An Algorithm for Simultaneous Operation of RF Multi-channels
- Find the period of the following TCL555 output square wave
- PIC18F26K80 INT0 interrupt does not work, see where the problem is
- Application design of MCU in blood analyzer
- GD32E231 DIY Competition (8) - Complete the driver of timer 2
- 4-20mA in industry?
- [NXP Rapid IoT Review] + First Rapid IOT Studio Project
- At 10 am today, Datang NXP will broadcast a live broadcast of [New energy lithium battery management solution with impedance detection function]
- Can anyone help me figure out what chip model this is?