1. System plan
This design uses AT89C52 microcontroller as the main controller. The LCD 1602 displays the temperature and humidity. LabView communicates with the host computer. The host computer displays the temperature. At the same time, the host computer can set the upper and lower temperature limits. When the measured temperature is lower than or higher than the upper limit, the temperature Alarm, the upper computer can control the starting and shutting down of the heating equipment of the lower computer, and realize the exchange of data between the upper computer and the lower computer.
2. The hardware design
schematic diagram is as follows:
3. Microcontroller software design
1. The microcontroller code is mainly serial port initialization and serial port interrupt service routine. The first is the serial port initialization:
void uart_init()
{
TMOD |= 0x20;//Timer 1, working mode 2 8-bit automatic reloading
TH1 = 0xfd;
TL1 = 0xfd; //Set the bit rate 9600
SM0 = 0;
SM1 = 1 ;//Serial port working mode 1, 8-bit UART baud rate variable
TR1 = 1; //Start timer 1
REN = 1;
EA = 1; //Open total interrupt
ES = 1; //Open serial port interrupt
}
2 , paste the two functions of the 51 microcontroller responsible for serial port transmission:
void SendByte(unsigned char dat) //Send one byte of data, the formal parameter dat is the data to be sent.
{
SBUF = dat; //Write data to the serial port buffer
while(!TI); //Wait for the sending to complete
TI = 0;
}
void SendArray(unsigned char *Array, unsigned char Size)//Send an array through the serial port, Construct a for loop, change the index and send
{
unsigned char i;
for(i = 0; i < Size; i++)
{
SendByte(Array[i]);
}
}
Process the received data in the serial port interrupt:
void uart(void ) interrupt 4 //Serial port interrupt
{
unsigned char Res;
static unsigned char Rec_state = 0;
if(RI) //Data received
{
RI = 0; // Clear interrupt request
Res = SBUF;
if(Res == 0xFF) // Frame header received
{
Rec_state = 1;
}
else if(Rec_state == 1 && Res == 0xEE)//Received end of frame
{
Rec_state = 0;
}
else if(Rec_state == 1)
{
switch(Res)//Received data, relay control
{
case 0x02: RY2 = 0; break;
case 0x03 : RY2 = 1; break;
default : RY2 =1; break;
}
}
}
else //After sending one byte of data
{
//TI = 0;
}
}
3. Paste the DHT22 temperature and humidity reading function
unsigned char Read_Sensor(void )
{
unsigned char i;
//Host pulls low (Min=800US Max=20Ms)
DHT_PIN = 0;
Delay_N1ms(18); //Delay 18Ms
//Release bus delay (Min=30us Max=50us)
DHT_PIN = 1;
Delay_N10us(2); //Delay 30us
//The host is set to input the sensor response signal
DHT_PIN = 1;
Sensor_AnswerFlag = 0; // Sensor response flag
//Judge whether the slave has a low-level response signal. If it does not respond, it will jump out. If it responds, it will run downward
if(DHT_PIN ==0)
{
Sensor_AnswerFlag = 1; //Receive the start signal
Sys_CNT = 0;
//Judge the slave. Whether the machine sends out a low-level response signal of 80us and ends
while((!DHT_PIN))
{
if(++Sys_CNT>300) //Prevent entering an infinite loop
{
Sensor_ErrorFlag = 1;
return 0;
}
}
Sys_CNT = 0;
// Determine whether the slave sends a high level of 80us. If it does, it will enter the data receiving state
while((DHT_PIN))
{
if(++Sys_CNT>300) //Prevent entering an infinite loop
{
Sensor_ErrorFlag = 1;
return 0;
}
}
/ / The data receiving sensor sends a total of 40 bits of data
// That is, the high bits of 5 bytes are sent first and the 5 bytes are the high bits of humidity, the low bits of humidity, the high bits of temperature, the low bits of temperature, checksum
// The checksum is: high bits of humidity + low bits of humidity + temperature High bit + temperature low bit
for(i=0;i<5;i++)
{
Sensor_Data[i] = Read_SensorData();
}
}
else
{
Sensor_AnswerFlag = 0; // Sensor response not received
}
return 1;
}
4. Host computer The software design
host computer is developed with the help of LabView. The interface is relatively simple. First, the UI interface is designed on the front panel, and then the software development is completed through graphical programming on the back panel. Here is a screenshot of the front panel of the host computer:
The screenshot of the rear panel is as follows:
Previous article:Comparative analysis of two methods of CAN bus and Ethernet embedded gateway circuit design
Next article:Practical methods of 51 series microcontroller timer
Recommended ReadingLatest update time:2024-11-23 02:44
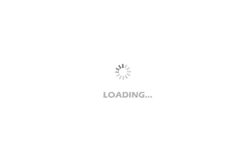
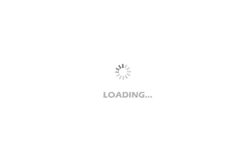
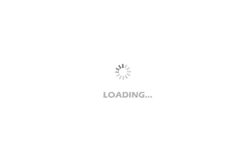
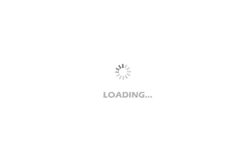
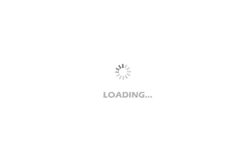
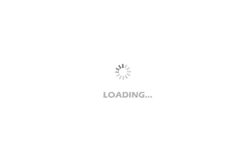
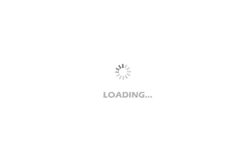
- Popular Resources
- Popular amplifiers
-
100 Examples of Microcontroller C Language Applications (with CD-ROM, 3rd Edition) (Wang Huiliang, Wang Dongfeng, Dong Guanqiang)
-
Arduino Nano collects temperature and humidity data through LabVIEW and DHT11
-
Modern Testing Technology and System Integration (Liu Junhua)
-
Computer Control System Analysis, Design and Implementation Technology (Edited by Li Dongsheng, Zhu Wenxing, Gao Rui)
- Naxin Micro and Xinxian jointly launched the NS800RT series of real-time control MCUs
- How to learn embedded systems based on ARM platform
- Summary of jffs2_scan_eraseblock issues
- Application of SPCOMM Control in Serial Communication of Delphi7.0
- Using TComm component to realize serial communication in Delphi environment
- Bar chart code for embedded development practices
- Embedded Development Learning (10)
- Embedded Development Learning (8)
- Embedded Development Learning (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Intel promotes AI with multi-dimensional efforts in technology, application, and ecology
- ChinaJoy Qualcomm Snapdragon Theme Pavilion takes you to experience the new changes in digital entertainment in the 5G era
- Infineon's latest generation IGBT technology platform enables precise control of speed and position
- Two test methods for LED lighting life
- Don't Let Lightning Induced Surges Scare You
- Application of brushless motor controller ML4425/4426
- Easy identification of LED power supply quality
- World's first integrated photovoltaic solar system completed in Israel
- Sliding window mean filter for avr microcontroller AD conversion
- What does call mean in the detailed explanation of ABB robot programming instructions?
- STMicroelectronics discloses its 2027-2028 financial model and path to achieve its 2030 goals
- 2024 China Automotive Charging and Battery Swapping Ecosystem Conference held in Taiyuan
- State-owned enterprises team up to invest in solid-state battery giant
- The evolution of electronic and electrical architecture is accelerating
- The first! National Automotive Chip Quality Inspection Center established
- BYD releases self-developed automotive chip using 4nm process, with a running score of up to 1.15 million
- GEODNET launches GEO-PULSE, a car GPS navigation device
- Should Chinese car companies develop their own high-computing chips?
- Infineon and Siemens combine embedded automotive software platform with microcontrollers to provide the necessary functions for next-generation SDVs
- Continental launches invisible biometric sensor display to monitor passengers' vital signs
- Silly answers in interviews
- Arduino releases v1.8.11
- Problems with waveform conversion circuits
- What's so special about Tesla's batteries?
- Why do new boards still include serial ports?
- What good things did everyone buy on Double 11? I bought a pair of "quantum long johns"
- ADI Launches High-Accuracy, Flexible Quad Voltage Monitor and Sequencer
- Application of Solid State Relays in High Reliability Systems
- Come together, everyone.
- PIC12C5XX instruction set and programming skills