Key input subsystem driver:
#include #include #include #include #include #include #include #include #include #include #include #include #include #include #include #include #include #include #include struct pin_desc { int irq; int pin; int pin_state; char *name; int key_val; int pin_val; }; static struct pin_desc buttons_pin[] = { {IRQ_EINT8, S3C2410_GPG(0), S3C2410_GPG0_EINT8, "KEY1", BTN_0}, {IRQ_EINT11, S3C2410_GPG(3), S3C2410_GPG3_EINT11, "KEY3", BTN_1}, {IRQ_EINT13, S3C2410_GPG(5), S3C2410_GPG5_EINT13, "KEY2", BTN_2}, {IRQ_EINT14, S3C2410_GPG(6), S3C2410_GPG6_EINT14, "KEY4", BTN_3}, {IRQ_EINT15, S3C2410_GPG(7), S3C2410_GPG7_EINT15, "KEY5", BTN_4}, {IRQ_EINT19, S3C2410_GPG(11), S3C2410_GPG11_EINT19, "KEY6", BTN_5}, }; static struct input_dev *buttons_input; static irqreturn_t buttons_interrupt(int irq,void *dev_id) { struct pin_desc *pin_desc = (struct pin_desc *)dev_id; int val; val = s3c2410_gpio_getpin(pin_desc->pin); input_event(buttons_input,EV_KEY,pin_desc->key_val, !val); input_sync(buttons_input); return IRQ_HANDLED; } static int __init buttons_init(void) { int error; int i; buttons_input = input_allocate_device(); if(!buttons_input) return -ENOMEM; #if 0 buttons_input->evbit[0] = BIT(EV_KEY); buttons_input->keybit[BITS_TO_LONGS(KEY_L)] = BIT(KEY_L); buttons_input->keybit[BITS_TO_LONGS(KEY_S)] = BIT(KEY_S); buttons_input->keybit[BITS_TO_LONGS(KEY_ENTER)] = BIT(KEY_ENTER); buttons_input->keybit[BITS_TO_LONGS(KEY_LEFTSHIFT)] = BIT(KEY_LEFTSHIFT); #endif set_bit(EV_KEY,buttons_input->evbit); for(i = 0;i set_bit(buttons_pin[i].key_val,buttons_input->keybit); } error = input_register_device(buttons_input); if(error){ input_free_device(buttons_input); return -1; } for(i = 0;i s3c2410_gpio_cfgpin(buttons_pin[i].pin,buttons_pin[i].pin_state); request_irq(buttons_pin[i].irq,buttons_interrupt, IRQF_SHARED | IRQF_TRIGGER_RISING | IRQF_TRIGGER_FALLING,buttons_pin[i].name,(void *)&buttons_pin[i]); } return 0; } static void __exit buttons_exit(void) { int i; for(i = 0; i< sizeof(buttons_pin)/sizeof(buttons_pin[0]); i++) { free_irq(buttons_pin[i].irq, (void *)&buttons_pin[i]); } input_unregister_device(buttons_input); input_free_device(buttons_input); } module_init(buttons_init); module_exit(buttons_exit); MODULE_LICENSE("GPL"); MODULE_AUTHOR("RopenYuan"); Key input subsystem test program #include #include #include #include #include #include #include #include #include #include #include int main(void) { int buttons_fd; int key_value,i=0,count; struct input_event ev_key; buttons_fd = open("/dev/input/event0", O_RDWR); if (buttons_fd < 0) { perror("open device buttons"); exit(1); } for (;;) { count = read(buttons_fd,&ev_key,sizeof(struct input_event)); for(i=0; i<(int)count/sizeof(struct input_event); i++) if(EV_KEY==ev_key.type) printf("type:%d,code:%d,value:%dn", ev_key.type,ev_key.code,ev_key.value); if(EV_SYN==ev_key.type) printf("syn eventnn"); } close(buttons_fd); return 0; } MAKEFILE ifneq ($(KERNELRELEASE),) obj-m := buttons.o else #KDIR := /home/yuanpengjun/home/linuxeditorkernelfilesystem/kernal/linux-2.6.32/linux-2.6.32.2/ KDIR := /home/yuanpengjun/mini2440/kernel/linux-2.6.32.2/ all: # make -C $(KDIR) M=$(PWD) modules ARCH=arm CROSS_COMPILE=arm-linux- make -C $(KDIR) M=$(PWD) modules /home/yuanpengjun/mini2440/editor/4.4.3/bin/arm-none-linux-gnueabi-gcc -o button button_app.c clean: rm -f *.ko *.o *.mod.o *.mod.c *.symvers modul* endif The above driver code does not include fault tolerance processing, just to verify whether it can run successfully. Input appears when loading the module: Unspecified device as /devices/virtual/input/input0 The reason is that the member name in struct input_dev is not set in the driver. If there is a member named XXXX->name = "yyyyyyy", the following situation will occur after the driver module is loaded: input: yyyyyyy as /devices/virtual/input/input0 When uninstalling the module, rmmod: chdir(2.6.32.2): No such file or directory appears, and the module cannot be uninstalled from the system; The reason is: When the current kernel module is inserted and uninstalled, it will be transferred to the directory /lib/modules/kernel version number/, in the format of /lib/modules/2.6.32.2/. In this case, the module will no longer appear. Unable to uninstall;
Previous article:mini2440 touch screen driver----implementation method of non-input subsystem
Next article:s3c2410 touch screen driver (2.6 kernel) analysis - interrupt the lower half
Recommended ReadingLatest update time:2024-11-16 12:24
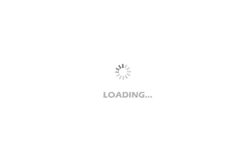
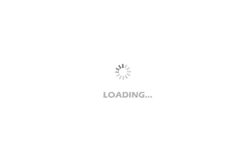
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- DGUS T5UID1 implements modbus protocol
- 【Goodbye 2021, Hello 2022】+ My summary of 2021
- Super detailed teaching you to use HFSS to design and simulate inverted F antenna 3
- Keil's optimization is really a rip-off
- [Zero-knowledge ESP8266 tutorial] Quick Start 2- Light up the external LED
- [Embedded Linux] RK3568 Android 11 screen point process
- Xiaoyi H8 camera, V831 vest QG2101
- Negative feedback bandwidth broadening
- Cyclone pin constraints
- 【Smart Network Desk Lamp】5. ESP32-S2 uses sntp for network time synchronization