main.c
#include #include #include #include #define uchar unsigned char #define uint unsigned int uchar code Write_addr [] = {0x80, 0x82, 0x84, 0x86, 0x88, 0x8a, 0x8c}; // write address uchar code Read_addr[] = {0x81,0x83,0x85,0x87,0x89,0x8b,0x8d}; // read address uchar Time [7] = {0x50, 0x59, 0x23, 0x07, 0x06, 0x02, 0x22}; // Initial value setting // Seconds Minutes Hours Day Month Week Year //-----------------------------------Variable area--------------------------------- uint Temp; // temperature variable //-----------------------------------Function area--------------------------------- void Int_DS1302() { flying i; Write_Ds1302_Byte( 0x8e,0x00 ); for(i = 0;i < 8;i++) { Write_Ds1302_Byte( Write_addr [i],Time [i] ); } Write_Ds1302_Byte( 0x8e,0x80 ); } void Read_DS1302_Time() { flying i; for(i = 0;i<8;i++) { Time [i] = Read_Ds1302_Byte( Read_addr [i] ); } } void Display_Time() { LCD_ShowNum(2,15,Time [0]%16,1); LCD_ShowNum(2,14,Time [0]/16,1); LCD_ShowChar(2 ,13,':'); LCD_ShowNum(2,12,Time [1]%16,1); LCD_ShowNum(2,11,Time [1]/16,1); LCD_ShowChar(2 ,10,':'); LCD_ShowNum(2,9,Time [2]%16,1); LCD_ShowNum(2,8,Time [2]/16,1); LCD_ShowChar(2 ,7,'-'); LCD_ShowNum(2,6,Time [3]%16,1); LCD_ShowNum(2,5,Time [3]/16,1); LCD_ShowChar(2 ,4,'-'); LCD_ShowNum(2,3,Time [4]%16,1); LCD_ShowNum(2,2,Time [4]/16,1); } void main() { Int_DS1302(); LCD_initial(); while(1) { Read_DS1302_Time(); Temp = Read_Temp(); string(0x82,"Temp"); LCD_ShowChar(1 ,7,':'); LCD_ShowChar(1 ,11,'C'); LCD_ShowNum(1,8,Temp,3); //The first row directly displays the temperature, occupying four cells //---------------------------------display time---------------------------------------- Display_Time(); } } onewire.c #include "reg52.h" #include #define uchar unsigned char #define uint unsigned int sbit DQ = P3^3; //single bus interface // Single bus delay function void Delay_OneWire(unsigned int t) //STC89C52RC { while(t--); } //Write a byte to DS18B20 via single bus void Write_DS18B20(unsigned char dat) { unsigned char i; for(i=0;i<8;i++) { DQ = 0; DQ = that&0x01; Delay_OneWire(12); DQ = 1; that >>= 1; } Delay_OneWire(5); } //Read a byte from DS18B20 unsigned char Read_DS18B20(void) { unsigned char i; unsigned char dat; for(i=0;i<8;i++) { DQ = 0; that >>= 1; DQ = 1; if(DQ) { that |= 0x80; } Delay_OneWire(5); } return that; } //DS18B20 device initialization bit init_ds18b20(void) { bit initflag = 0; DQ = 1; Delay_OneWire(12); DQ = 0; Delay_OneWire(80); DQ = 1; Delay_OneWire(10); initflag = DQ; Delay_OneWire(5); return initflag; } uint Read_Temp() { uint high,low,Date_T; init_ds18b20(); Write_DS18B20(0xcc); Write_DS18B20(0x44); Delay_OneWire(225); init_ds18b20(); Write_DS18B20(0xcc); Write_DS18B20(0xbe); low = Read_DS18B20(); high = Read_DS18B20(); Date_T = high<<8; Date_T = Date_T+low; Date_T = Date_T * 0.0625+0.5;// return Date_T; } onewire.h #ifndef __ONEWIRE_H #define __ONEWIRE_H #define uchar unsigned char #define uint unsigned int unsigned char rd_temperature(void); //; ; unsigned char Read_DS18B20(void); void Write_DS18B20(unsigned char dat); bit init_ds18b20(void); uchar Read_Temp(void); #endif LCD.c #include #include #define uchar unsigned char #define uint unsigned int #define out P2 sbit rs = P1^5; sbit rw = P1^4; sbit e = P1^3; void delay(uint j) { fly i=250; for(;j>0;j--) { while(--i); i=249; while(--i); i=250; } } void check_busy(void) { flying dt; do { dt=0xff; e=0; rs=0; rw=1; e=1; dt=out; }while(dt&0x80); e=0; } void write_command(uchar com) //write command { check_busy(); e=0; rs=0; rw=0; out=com; e=1; _nop_(); e=0; delay(1); } void write_data(uchar dat)//write data { check_busy(); e=0; rs=1; rw=0; out=that; e=1; _nop_(); e=0; delay(1); } void LCD_initial(void) //initialization { delay(2); write_command(0x38); delay(1); write_command(0x38); write_command(0x08); write_command(0x01); write_command(0x06); write_command(0x0c); //delay(1); } void string(uchar ad,uchar *s) //display characters { write_command(ad); while(*s>0) { write_data(*s++); delay(100); } } int LCD_Pow(uint x,uint y)// { unsigned char i = 0; int result = 1; for(i=0;i result *= x; } return result; } void LCD_SetCursor(uchar Line,uchar Columu) //Line selection { if(Line == 1) { write_command(0x80|(Columu-1)); } else { write_command(0x80|( Columu-1)+0x40); } } // row grid number length void LCD_ShowNum(uchar Line,uchar Columu,uint Num,uint Length)//Display number { uint i = 0; LCD_SetCursor(Line,Columu); for(i=Length;i>0;i--) { write_data('0'+Num/LCD_Pow(10,i-1)%10); } } void LCD_ShowChar(unsigned char Line, unsigned char Columu, unsigned char Char) //Write a character { LCD_SetCursor(Line,Columu); write_data(Char); } LCD.h #ifndef __LCD_H__ #define __LCD_H__ #define uchar unsigned char #define uint unsigned int void check_busy(void); void write_command(unsigned char com); void write_data(uchar dat); void LCD_initial(void); void string(flyer name ,flyer *s); void lcd_test(void); void delay(uint); void LCD_ShowNum(uchar Line,uchar Columu,uint Num,uint Length);//Display number void LCD_SetCursor(float Line, float Column); int LCD_Pow(volatile x,volatile y); void LCD_ShowChar(unsigned char Line, unsigned char Columu, unsigned char Char); //Write a character #endif ds1302.c #include #include sbit SCK = P3^1; sbit SDA = P3^2; sbit RST = P3^0; // DS1302 reset #define uchar unsigned char #define uint unsigned int //uchar code Write_addr [] = {0x80, 0x82, 0x84, 0x86, 0x88, 0x8a, 0x8c}; //write address //uchar code Read_addr[] = {0x81,0x83,0x85,0x87,0x89,0x8b,0x8d}; //read address //uchar Time [7] = {0x50, 0x59, 0x23, 0x00, 0x00, 0x00, 0x00}; //initial value setting Seconds Minutes Hours Day Month Week Year void Write_Ds1302(unsigned char temp) { unsigned char i; for (i=0;i<8;i++) { SCK=0; SDA=temp&0x01; temp>>=1; SCK=1; } } void Write_Ds1302_Byte( unsigned char address,unsigned char dat ) { RST=0; _nop_(); SCK=0; _nop_(); RST=1; _nop_(); Write_Ds1302(address); Write_Ds1302(that); RST=0; } unsigned char Read_Ds1302_Byte ( unsigned char address ) { unsigned char i,temp=0x00; RST=0; _nop_(); SCK=0; _nop_(); RST=1; _nop_(); Write_Ds1302(address); for (i=0;i<8;i++) { SCK=0; temp>>=1; if(SDA) temp|=0x80; SCK=1; } RST=0; _nop_(); SCK=0; _nop_(); SCK=1; _nop_(); SDA=0; _nop_(); SDA=1; _nop_(); return (temp); } ds1302.h #ifndef __DS1302_H #define __DS1302_H void Write_Ds1302(unsigned char temp); void Write_Ds1302_Byte( unsigned char address,unsigned char dat ); unsigned char Read_Ds1302_Byte( unsigned char address ); #endif Simulation diagram
Previous article:51 MCU connected to ADC0808 to make a simple voltage meter
Next article:Use the timer to make the P1^0 port output a specified duty cycle and periodic rectangular pulse
Recommended ReadingLatest update time:2024-11-23 10:40
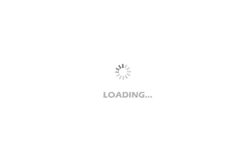
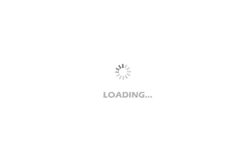
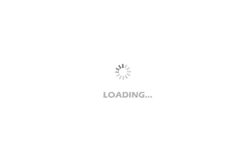
- Popular Resources
- Popular amplifiers
-
Single-chip microcomputer C language programming and simulation
-
100 Examples of Microcontroller C Language Applications (with CD-ROM, 3rd Edition) (Wang Huiliang, Wang Dongfeng, Dong Guanqiang)
-
Principles and Applications of Single Chip Microcomputers and C51 Programming (3rd Edition) (Xie Weicheng, Yang Jiaguo)
-
stm32+lcd1602 example
- Naxin Micro and Xinxian jointly launched the NS800RT series of real-time control MCUs
- How to learn embedded systems based on ARM platform
- Summary of jffs2_scan_eraseblock issues
- Application of SPCOMM Control in Serial Communication of Delphi7.0
- Using TComm component to realize serial communication in Delphi environment
- Bar chart code for embedded development practices
- Embedded Development Learning (10)
- Embedded Development Learning (8)
- Embedded Development Learning (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Intel promotes AI with multi-dimensional efforts in technology, application, and ecology
- ChinaJoy Qualcomm Snapdragon Theme Pavilion takes you to experience the new changes in digital entertainment in the 5G era
- Infineon's latest generation IGBT technology platform enables precise control of speed and position
- Two test methods for LED lighting life
- Don't Let Lightning Induced Surges Scare You
- Application of brushless motor controller ML4425/4426
- Easy identification of LED power supply quality
- World's first integrated photovoltaic solar system completed in Israel
- Sliding window mean filter for avr microcontroller AD conversion
- What does call mean in the detailed explanation of ABB robot programming instructions?
- STMicroelectronics discloses its 2027-2028 financial model and path to achieve its 2030 goals
- 2024 China Automotive Charging and Battery Swapping Ecosystem Conference held in Taiyuan
- State-owned enterprises team up to invest in solid-state battery giant
- The evolution of electronic and electrical architecture is accelerating
- The first! National Automotive Chip Quality Inspection Center established
- BYD releases self-developed automotive chip using 4nm process, with a running score of up to 1.15 million
- GEODNET launches GEO-PULSE, a car GPS navigation device
- Should Chinese car companies develop their own high-computing chips?
- Infineon and Siemens combine embedded automotive software platform with microcontrollers to provide the necessary functions for next-generation SDVs
- Continental launches invisible biometric sensor display to monitor passengers' vital signs
- About steering wheel steering sensor selection
- How much do you know about power supply? Take a look at the following questions to test yourself
- Driving Asphalt 8 with PICO and motion sensors
- Pulse oximeter based on MSP430FG439 development board
- MicroPython Hands-on (40) - Image Basics of Machine Vision 2
- Teach you how to learn DSP step by step - based on TMS320F28335
- 【NXP Rapid IoT Review】
- Development of a new multifunctional membrane/substrate bonding strength tester
- MOC3031
- LPC55S69