Introduction to DS3231
DS3231 is a high-precision I2C real-time clock chip, and the I2C bus address is fixed to 0xD0
Built-in temperature compensated crystal oscillator (TCXO) to reduce crystal frequency drift caused by temperature changes, with an error of ±0.432s/Day in the range of [-40°C, 85°C].
Seconds, minutes, hours, weekday, date, month, year, leap year compensation, counting year interval is [1990, 2190]
Two programmable alarms that can repeat weekly or daily
Square wave output
Power supply 2.3V – 5.5V (typical: 3.3V)
Operating current 200 – 300 μA
Standby current 110 – 170 μA
Battery operating current 70 – 150 μA
Time hold battery current 0.84 – 3.5 μA
DS3231 pinout and typical circuit
32KHz - 32.768KHz output (50% duty cycle), open drain output, requires a pull-up resistor, can be left open if not used.
VCC
INT/SQW - Active low interrupt or square wave output (1Hz, 4kHz, 8kHz or 32kHz)
RST - Active low reset pin
GND
VBAT - Backup Power Supply
SDA - I2C Data
SCL - I2C Clock
ZS-042 Module
The most common DS3231 on Taobao is the ZS-042 module, which integrates a CR2032 battery holder and an AT24C32 8K-byte EEPROM storage, the latter of which can be accessed through the same I2C bus.
CR2032 Battery Holder
Provides backup power to the DS3231 when power is interrupted
Onboard AT24C32 EEPROM
The memory chip is AT24C32, with a capacity of 32K Bit = 4K Byte. The address can be modified by shorting A0/A1/A2. According to the data sheet of 24C32, these three bits correspond to the fifth to seventh bits of the 7-bit I2C address.
1 0 1 0 A2 A1 A0 R/W
A0 to A2 are pulled up by internal resistors. Open circuit is 1, short circuit is 0. Different combinations can generate 8 different addresses. The default address corresponding to full open circuit is 0xAE.
Using STC8H3K to drive DS3231
wiring
All three pairs of contacts of AT24C32 remain open
P32 -> SCL
P33 -> SDA
GND -> GND
3.3V -> VCC
Sample Code
Code download address
Gitee https://gitee.com/iosetting/fw-lib_-stc8/tree/master/demo/i2c/ds3231
GitHub https://github.com/IOsetting/FwLib_STC8/tree/master/demo/i2c/ds3231
The code will set the DS3231 time to 2022-07-10 14:21:10, and then display the time every second in hexadecimal format.
20-07-0A 0E:15:1E 00 00␍␊
20-07-0A 0E:15:1F 00 00␍␊
20-07-0A 0E:15:20 00 00␍␊
20-07-0A 0E:15:21 00 00␍␊
20-07-0A 0E:15:22 00 00␍␊
Initialize the I2C interface
Using P32 and P33
void I2C_Init(void)
{
// Master mode
I2C_SetWorkMode(I2C_WorkMode_Master);
/**
* I2C clock = FOSC / 2 / (__prescaler__ * 2 + 4)
*/
I2C_SetClockPrescaler(0x1F);
// Switch alternative port
I2C_SetPort(I2C_AlterPort_P32_P33);
// Start I2C
I2C_SetEnabled(HAL_State_ON);
}
void GPIO_Init(void)
{
// SDA
GPIO_P3_SetMode(GPIO_Pin_3, GPIO_Mode_InOut_QBD);
// SCL
GPIO_P3_SetMode(GPIO_Pin_2, GPIO_Mode_Output_PP);
}
Basic I2C interface reading and writing methods
#define DS3231_I2C_ADDR 0xD0
uint8_t DS3231_Write(uint8_t reg, uint8_t dat)
{
return I2C_Write(DS3231_I2C_ADDR, reg, &dat, 1);
}
uint8_t DS3231_MultipleRead(uint8_t reg, uint8_t *buf, uint8_t len)
{
return I2C_Read(DS3231_I2C_ADDR, reg, buf, len);
}
Conversion between BCD code and HEX
uint8_t DS3231_Hex2Bcd(uint8_t hex)
{
return (hex % 10) + ((hex / 10) << 4);
}
uint8_t DS3231_Bcd2Hex(uint8_t bcd)
{
return (bcd >> 4) * 10 + (bcd & 0x0F);
}
Reading time
Read the time and convert it to HEX, using a uint8_t array with the following structure:
/**
uint8_t year;
uint8_t month;
uint8_t week;
uint8_t date;
uint8_t hour;
uint8_t minute;
uint8_t second;
DS3231_HourFormat_t format;
DS3231_AmPm_t am_pm;
*/
Reading the time from the DS3231
uint8_t DS3231_GetTime(uint8_t *t)
{
uint8_t res;
res = I2C_Read(DS3231_I2C_ADDR, DS3231_REG_SECOND, buff, 7);
if (res != HAL_OK)
{
return res;
}
t[0] = DS3231_Bcd2Hex(buff[6]) + ((buff[5] >> 7) & 0x01) * 100; // year
t[1] = DS3231_Bcd2Hex(buff[5] & 0x1F); // month
t[2] = DS3231_Bcd2Hex(buff[3]); // week
t[3] = DS3231_Bcd2Hex(buff[4]); // date
t[7] = (buff[2] >> 6) & 0x01; // 12h/24h
t[8] = (buff[2] >> 5) & 0x01; // am/pm
if (t[7] == DS3231_FORMAT_12H)
{
t[4] = DS3231_Bcd2Hex(buff[2] & 0x1F); // hour
}
else
{
t[4] = DS3231_Bcd2Hex(buff[2] & 0x3F); // hour
}
t[5] = DS3231_Bcd2Hex(buff[1]); // minute
t[6] = DS3231_Bcd2Hex(buff[0]); // second
return HAL_OK;
}
Setting the time
First check the time values, then write them in by address
uint8_t DS3231_SetTime(uint8_t *t)
{
uint8_t res, reg;
// Time validation
if (t[0] > 200) t[0] = 200; // year
if (t[1] == 0) t[1] = 1; // month
else if (t[1] > 12) t[1] = 12;
if (t[2] == 0) t[2] = 1; // week
else if (t[2] > 7) t[2] = 7;
if (t[3] == 0) t[3] = 1; // date
else if (t[3] > 31) t[3] = 31;
if (t[7] == DS3231_FORMAT_12H)
{
if (t[4] > 12) t[4] = 12; // hour
}
else if (t[7] == DS3231_FORMAT_24H)
{
if (t[4] > 23) t[4] = 23; // hour
}
if (t[5] > 59) t[5] = 59; // minute
if (t[6] > 59) t[6] = 59; // second
res = DS3231_Write(DS3231_REG_SECOND, DS3231_Hex2Bcd(t[6]));
if (res != HAL_OK) return res;
res = DS3231_Write(DS3231_REG_MINUTE, DS3231_Hex2Bcd(t[5]));
if (res != HAL_OK) return res;
if (t[7] == DS3231_FORMAT_12H)
{
reg = (uint8_t)((1 << 6) | (t[8] << 5) | DS3231_Hex2Bcd(t[4]));
}
else
{
reg = (0 << 6) | DS3231_Hex2Bcd(t[4]);
}
res = DS3231_Write(DS3231_REG_HOUR, reg);
if (res != HAL_OK) return res;
res = DS3231_Write(DS3231_REG_WEEK, DS3231_Hex2Bcd(t[2]));
if (res != HAL_OK) return res;
res = DS3231_Write(DS3231_REG_DATE, DS3231_Hex2Bcd(t[3]));
if (res != HAL_OK) return res;
if (t[0] >= 100)
{
res = DS3231_Write(DS3231_REG_MONTH, DS3231_Hex2Bcd(t[1]) | (1 << 7));
if (res != HAL_OK) return res;
return DS3231_Write(DS3231_REG_YEAR, DS3231_Hex2Bcd(t[0] - 100));
}
else
{
res = DS3231_Write(DS3231_REG_MONTH, DS3231_Hex2Bcd(t[1]));
if (res != HAL_OK) return res;
return DS3231_Write(DS3231_REG_YEAR, DS3231_Hex2Bcd(t[0]));
}
}
Read and write AT24C32 in ZS-042 module
Refer to the previous article STC8H Development (XII): I2C drive AT24C08, AT24C32 series EEPROM storage
reference
Discussion of DS3231 module ZS-042, analyzing the battery charging problem and modification when powered by 5V https://forum.arduino.cc/t/zs-042-ds3231-rtc-module/268862/24
AT24C reading and writing https://www.likecs.com/show-204385163.html
Previous article:STC8H Development (XIV): I2C driver for RX8025T high-precision real-time clock chip
Next article:STC8H Development (XII): I2C drive AT24C08, AT24C32 series EEPROM storage
Recommended ReadingLatest update time:2024-11-23 06:47
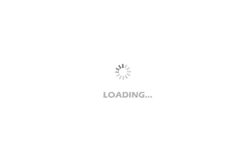
- Popular Resources
- Popular amplifiers
-
Siemens Motion Control Technology and Engineering Applications (Tongxue, edited by Wu Xiaojun)
-
MCU C language programming and Proteus simulation technology (Xu Aijun)
-
100 Examples of Microcontroller C Language Applications (with CD-ROM, 3rd Edition) (Wang Huiliang, Wang Dongfeng, Dong Guanqiang)
-
Principles and Applications of Single Chip Microcomputers 3rd Edition (Zhang Yigang)
- Naxin Micro and Xinxian jointly launched the NS800RT series of real-time control MCUs
- How to learn embedded systems based on ARM platform
- Summary of jffs2_scan_eraseblock issues
- Application of SPCOMM Control in Serial Communication of Delphi7.0
- Using TComm component to realize serial communication in Delphi environment
- Bar chart code for embedded development practices
- Embedded Development Learning (10)
- Embedded Development Learning (8)
- Embedded Development Learning (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Intel promotes AI with multi-dimensional efforts in technology, application, and ecology
- ChinaJoy Qualcomm Snapdragon Theme Pavilion takes you to experience the new changes in digital entertainment in the 5G era
- Infineon's latest generation IGBT technology platform enables precise control of speed and position
- Two test methods for LED lighting life
- Don't Let Lightning Induced Surges Scare You
- Application of brushless motor controller ML4425/4426
- Easy identification of LED power supply quality
- World's first integrated photovoltaic solar system completed in Israel
- Sliding window mean filter for avr microcontroller AD conversion
- What does call mean in the detailed explanation of ABB robot programming instructions?
- STMicroelectronics discloses its 2027-2028 financial model and path to achieve its 2030 goals
- 2024 China Automotive Charging and Battery Swapping Ecosystem Conference held in Taiyuan
- State-owned enterprises team up to invest in solid-state battery giant
- The evolution of electronic and electrical architecture is accelerating
- The first! National Automotive Chip Quality Inspection Center established
- BYD releases self-developed automotive chip using 4nm process, with a running score of up to 1.15 million
- GEODNET launches GEO-PULSE, a car GPS navigation device
- Should Chinese car companies develop their own high-computing chips?
- Infineon and Siemens combine embedded automotive software platform with microcontrollers to provide the necessary functions for next-generation SDVs
- Continental launches invisible biometric sensor display to monitor passengers' vital signs
- Request RF amplifier design solution
- IAR creates a new MSP430 project
- Development and application of mobile phone intelligent antenna test system
- About LDO voltage regulator chip
- Issue 014 // Low RCS Accurate Measurement and New Structure Millimeter Wave Test Field // Li Zhiping
- Correct Selection of Capacitors in Modern Power Supply Technology
- Bluenrg-2N master receives slave data
- Xu Qi, Li Dong: MCU Music Spectrum
- What are the maskable interrupts of MSP430?
- Differential Input and Differentiating Circuit