Windows 10 20H2
HLK-W806-V1.0-KIT
WM_SDK_W806_v0.6.0
Excerpted from "W806 Chip Design Guide V1.0", "W806 MCU Chip Specification V2.0"
Timer
Microsecond and millisecond timing (counting number configured according to clock frequency) implements six configurable 32-bit counters. When the count configured by the corresponding counter is completed, a corresponding interrupt is generated.
Library Functions
function
Open wm_tim.h, there is the following function declaration:
HAL_StatusTypeDef HAL_TIM_Base_Init(TIM_HandleTypeDef *htim);
// Initialize the timer used and its beat time unit and count value and other basic parameter configurations
HAL_StatusTypeDef HAL_TIM_Base_DeInit(TIM_HandleTypeDef *htim);
//Restore the initialized timer to its default state – the value of each register when it is reset
void HAL_TIM_Base_MspInit(TIM_HandleTypeDef *htim);
//Initialize timer clock, priority and enable corresponding interrupt
void HAL_TIM_Base_MspDeInit(TIM_HandleTypeDef *htim);
//Restore the corresponding timer to the default state
HAL_StatusTypeDef HAL_TIM_Base_Start(TIM_HandleTypeDef *htim);
//Timer starts counting
HAL_StatusTypeDef HAL_TIM_Base_Stop(TIM_HandleTypeDef *htim);
//Timer stops counting
HAL_StatusTypeDef HAL_TIM_Base_Start_IT(TIM_HandleTypeDef *htim);
//Timer interrupt mode starts counting
HAL_StatusTypeDef HAL_TIM_Base_Stop_IT(TIM_HandleTypeDef *htim);
//Timer stops counting in interrupt mode
HAL_TIM_StateTypeDef HAL_TIM_Base_GetState(TIM_HandleTypeDef *htim);
//Get the timer status
void HAL_TIM_IRQHandler(TIM_HandleTypeDef *htim);
//Timer interrupt service function, used to respond to timer interrupt. The function entity has two functions, one is to clear the interrupt flag bit, the other is to call the following callback function.
void HAL_TIM_Callback(TIM_HandleTypeDef *htim);
//The interrupt callback function can be understood as the specific action that the interrupt function responds to.
parameter
Structure and enumeration types
typedef enum
{
HAL_TIM_STATE_RESET = 0x00U,
HAL_TIM_STATE_READY = 0x01U,
HAL_TIM_STATE_BUSY = 0x02U,
HAL_TIM_STATE_TIMEOUT = 0x03U,
HAL_TIM_STATE_ERROR = 0x04U
} HAL_TIM_StateTypeDef;
typedef struct
{
uint32_t Unit;
uint32_t AutoReload;
uint32_t Period;
} TIM_Base_InitTypeDef;
typedef struct
{
uint32_t Instance;
TIM_Base_InitTypeDef Init;
HAL_LockTypeDef Lock;
__IO HAL_TIM_StateTypeDef State;
} TIM_HandleTypeDef;
Macro Parameters
#define TIM ((TIM_TypeDef *)TIM_BASE)
#define TIM0 0
#define TIM1 1
#define TIM2 2
#define TIM3 3
#define TIM4 4
#define TIM5 5
#define TIM_UNIT_US 0x00000000U
#define TIM_UNIT_MS 0x00000001U
//Timer beat time unit
#define TIM_AUTORELOAD_PRELOAD_DISABLE 0x00000001U
#define TIM_AUTORELOAD_PRELOAD_ENABLE 0x00000000U
Macro
#define IS_TIM_INSTANCE(INSTANCE)
(((INSTANCE) == TIM0) ||
((INSTANCE) == TIM1) ||
((INSTANCE) == TIM2) ||
((INSTANCE) == TIM3) ||
((INSTANCE) == TIM4) ||
((INSTANCE) == TIM5))
#define IS_TIM_UNIT(UNIT) (((UNIT) == TIM_UNIT_US) ||
((UNIT) == TIM_UNIT_MS))
#define IS_TIM_AUTORELOAD(PRELOAD) (((PRELOAD) == TIM_AUTORELOAD_PRELOAD_DISABLE) ||
((PRELOAD) == TIM_AUTORELOAD_PRELOAD_ENABLE))
#define __HAL_TIM_ENABLE(__HANDLE__) (TIM->CR |= TIM_CR_TIM_EN((__HANDLE__)->Instance - TIM0))
#define __HAL_TIM_DISABLE(__HANDLE__) (TIM->CR &= ~(TIM_CR_TIM_EN((__HANDLE__)->Instance - TIM0)))
#define __HAL_TIM_ENABLE_IT(__HANDLE__) (TIM->CR |= TIM_CR_TIM_TIE((__HANDLE__)->Instance - TIM0))
#define __HAL_TIM_DISABLE_IT(__HANDLE__) (TIM->CR &= ~(TIM_CR_TIM_TIE((__HANDLE__)->Instance - TIM0)))
#define __HAL_TIM_GET_FLAG(__HANDLE__) ((TIM->CR & TIM_CR_TIM_TIF((__HANDLE__)->Instance - TIM0)) ==
TIM_CR_TIM_TIF((__HANDLE__)->Instance - TIM0))
#define __HAL_TIM_CLEAR_IT(__HANDLE__) (TIM->CR |= TIM_CR_TIM_TIF((__HANDLE__)->Instance - TIM0))
Test program in Demo
main.c
#include #include "wm_hal.h" TIM_HandleTypeDef htim0; TIM_HandleTypeDef htim1; TIM_HandleTypeDef htim2; TIM_HandleTypeDef htim3; TIM_HandleTypeDef htim4; TIM_HandleTypeDef htim5; void Error_Handler(void); static void TIM0_Init(void); static void TIM1_Init(void); static void TIM2_Init(void); static void TIM3_Init(void); static void TIM4_Init(void); static void TIM5_Init(void); int main(void) { SystemClock_Config(CPU_CLK_160M); printf("enter mainrn"); TIM0_Heat(); HAL_TIM_Base_Start_IT(&htim0); TIM1_Heat(); HAL_TIM_Base_Start_IT(&htim1); TIM2_Heat(); HAL_TIM_Base_Start_IT(&htim2); TIM3_Heat(); HAL_TIM_Base_Start_IT(&htim3); TIM4_Heat(); HAL_TIM_Base_Start_IT(&htim4); TIM5_Init(); HAL_TIM_Base_Start_IT(&htim5); while(1) { HAL_Delay(1000); } } static void TIM0_Init(void) { htim0.Instance = TIM0; htim0.Init.Unit = TIM_UNIT_US; htim0.Init.Period = 1000000; htim0.Init.AutoReload = TIM_AUTORELOAD_PRELOAD_ENABLE; if (HAL_TIM_Base_Init(&htim0) != HAL_OK) { Error_Handler(); } } static void TIM1_Init(void) { htim1.Instance = TIM1; htim1.Init.Unit = TIM_UNIT_US; htim1.Init.Period = 1001000; htim1.Init.AutoReload = TIM_AUTORELOAD_PRELOAD_ENABLE; if (HAL_TIM_Base_Init(&htim1) != HAL_OK) { Error_Handler(); } } static void TIM2_Init(void) { htim2.Instance = TIM2; htim2.Init.Unit = TIM_UNIT_US; htim2.Init.Period = 1002000; htim2.Init.AutoReload = TIM_AUTORELOAD_PRELOAD_ENABLE; if (HAL_TIM_Base_Init(&htim2) != HAL_OK) { Error_Handler(); } } static void TIM3_Init(void) { htim3.Instance = TIM3; htim3.Init.Unit = TIM_UNIT_US; htim3.Init.Period = 1003000; htim3.Init.AutoReload = TIM_AUTORELOAD_PRELOAD_ENABLE; if (HAL_TIM_Base_Init(&htim3) != HAL_OK) { Error_Handler(); } } static void TIM4_Init(void) { htim4.Instance = TIM4; htim4.Init.Unit = TIM_UNIT_US; htim4.Init.Period = 1004000; htim4.Init.AutoReload = TIM_AUTORELOAD_PRELOAD_ENABLE; if (HAL_TIM_Base_Init(&htim4) != HAL_OK) { Error_Handler(); } } static void TIM5_Init(void) { htim5.Instance = TIM5; htim5.Init.Unit = TIM_UNIT_US; htim5.Init.Period = 1005000; htim5.Init.AutoReload = TIM_AUTORELOAD_PRELOAD_ENABLE; if (HAL_TIM_Base_Init(&htim5) != HAL_OK) { Error_Handler(); } } void HAL_TIM_Callback(TIM_HandleTypeDef *htim) { printf("%d ", htim->Instance); if (htim->Instance == TIM0) { } if (htim->Instance == TIM1) { } if (htim->Instance == TIM2) { } if (htim->Instance == TIM3) { } if (htim->Instance == TIM4) { } if (htim->Instance == TIM5) { } } void Error_Handler(void) { while (1) { } } void assert_failed(uint8_t *file, uint32_t line) { printf("Wrong parameters value: file %s on line %drn", file, line); } wm_hal_msp.c #include "wm_hal.h" void HAL_MspInit(void) { } void HAL_TIM_Base_MspInit(TIM_HandleTypeDef* htim_base) { __HAL_RCC_TIM_CLK_ENABLE(); HAL_NVIC_SetPriority(TIM_IRQn, 0); HAL_NVIC_EnableIRQ(TIM_IRQn); } void HAL_TIM_Base_MspDeInit(TIM_HandleTypeDef* htim_base) { // Since all TIMs share a clock and interrupt, if only one TIM is used, the clock and interrupt can be turned off during DeInit. However, if multiple TIMs are used, during DeInit of one channel, if // Turning off the clock and interrupt will affect other running TIMs // __HAL_RCC_TIM_CLK_DISABLE(); // HAL_NVIC_DisableIRQ(TIM_IRQn); } wm_it.c #include "wm_hal.h" extern TIM_HandleTypeDef htim0; extern TIM_HandleTypeDef htim1; extern TIM_HandleTypeDef htim2; extern TIM_HandleTypeDef htim3; extern TIM_HandleTypeDef htim4; extern TIM_HandleTypeDef htim5; #define readl(addr) ({unsigned int __v = (*(volatile unsigned int *) (addr)); __v;}) __attribute__((isr)) void CORET_IRQHandler(void) { readl(0xE000E010); HAL_IncTick(); } __attribute__((isr)) void TIM0_5_IRQHandler(void) { HAL_TIM_IRQHandler(&htim0); HAL_TIM_IRQHandler(&htim1); HAL_TIM_IRQHandler(&htim2); HAL_TIM_IRQHandler(&htim3); HAL_TIM_IRQHandler(&htim4); HAL_TIM_IRQHandler(&htim5); } Experimental phenomena
Previous article:[Lianshengde W806 Hands-on Notes] 6. 7816/UART Controller
Next article:[Lianshengde W806 Hands-on Notes] 4. PWM Module
Recommended ReadingLatest update time:2024-11-16 10:22
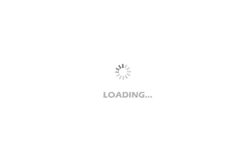
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- 【Arduino】168 sensor module series experiments (219) --- INMP441 omnidirectional microphone
- Technology Live: LPC55S69, designed to prevent hackers, helps you build secure edge nodes at low cost (share and win 20E coins)
- Analysis of EMI radiation signal strength
- Research on the Development of Radio Frequency Filter Materials Industry
- Working Principle of Solar Street Lights
- [RVB2601 Creative Application Development] + A Preliminary Study on Connecting to Alibaba Cloud
- [RVB2601 Creative Application Development] Practice 3 - External DHT11 Display of Temperature and Humidity
- Top 5 Microcontroller Board Features
- MSA-AIAG-Manual 4
- Solution to power supply problem of MCU based on SE8510