Windows 10 20H2
HLK-W806-V1.0-KIT
WM_SDK_W806_v0.6.0
Excerpted from "W806 Chip Design Guide V1.0", "W806 MCU Chip Specification V2.0"
7816/UART Controller
The device side complies with the APB bus interface protocol
Support interrupt or polling working mode
Support DMA transfer mode, send and receive are stored in 32-byte FIFO
DMA can only operate on bytes, with a maximum of 16-burst byte DMA operations
Compatible with UART and 7816 interface functions
Serial port function
Programmable baud rate
5-8bit data length and parity polarity configurable
1 or 2 stop positions configurable
Support RTS/CTS flow control
Support Break frame sending and receiving
Overrun,parity error,frame error,rx break frame 中断指示
7816 Interface Function
Compatible with ISO-7816-3 T=0.T=1 mode
Compatible with EVM2000 protocol
Configurable guard time (11 ETU-267 ETU)
Forward/reverse convention software configurable
Support send/receive parity check and retransmission function
Supports 0.5 and 1.5 stop bit configurations
Download Port
The W806 chip uses UART0 as the download port by default. When there is no firmware for the initial download, directly connect to the UART0 interface and download the firmware through the relevant download software. When there is firmware in the chip, enter the download mode again by pulling down BOOTMODE and then powering on to enter the download mode. After the download is complete, remove the operation of pulling down BOOTMODE and restart the firmware to run.
Library Functions
function
Open wm_uart.h, there is the following function declaration:
// Initialize the baud rate, data bit, stop bit, check bit, mode, etc. of the serial port used
HAL_StatusTypeDef HAL_UART_Init(UART_HandleTypeDef *huart);
//Restore the serial port to the default state after initialization - the value of each register when it is reset
HAL_StatusTypeDef HAL_UART_DeInit(UART_HandleTypeDef *huart);
//Serial port sends data, using timeout management mechanism
HAL_StatusTypeDef HAL_UART_Transmit(UART_HandleTypeDef *huart, uint8_t *pData, uint16_t Size, uint32_t Timeout);
//Serial port receives data and uses timeout management mechanism
HAL_StatusTypeDef HAL_UART_Receive(UART_HandleTypeDef *huart, uint8_t *pData, uint16_t Size, uint32_t Timeout);
//Serial port interrupt mode sending
HAL_StatusTypeDef HAL_UART_Transmit_IT(UART_HandleTypeDef *huart, uint8_t *pData, uint16_t Size);
/**
* Receive data of a certain length in interrupt mode.
* Note: The address pointed to by pData must have a space length greater than or equal to 32 bytes
* If Size is greater than 0, then receive data of sufficient length and execute HAL_UART_RxCpltCallback(huart);
* If Sized is 0, HAL_UART_RxCpltCallback(huart) will be executed once when receiving data of indefinite length;
* In both cases, the data is stored in huart->pRxBuffPtr or pData, and the data length is stored in huart->RxXferCount
*/
HAL_StatusTypeDef HAL_UART_Receive_IT(UART_HandleTypeDef *huart, uint8_t *pData, uint16_t Size);
//Serial port interrupt service function, used to respond to various serial port interrupts.
//The function entity has two functions, one is to clear the interrupt flag, the other is to call the corresponding callback function,
//如UART_Receive_IT、UART_Transmit_IT、UART_EndTransmit_IT
void HAL_UART_IRQHandler(UART_HandleTypeDef *huart);
//Serial port send interrupt callback function, called by UART_EndTransmit_IT
void HAL_UART_TxCpltCallback(UART_HandleTypeDef *huart);
//Serial port receive interrupt callback function, called by UART_Receive_IT
void HAL_UART_RxCpltCallback(UART_HandleTypeDef *huart);
parameter
Structure and enumeration types
Seeing that STM32 can't hold it anymore
typedef struct
{
uint32_t BaudRate; /*!< This member configures the UART communication baud rate.
The baud rate is computed using the following formula:
- IntegerDivider = ((PCLKx) / (16 * (huart->Init.BaudRate)))
- FractionalDivider = ((IntegerDivider - ((uint32_t) IntegerDivider)) * 16) + 0.5 */
uint32_t WordLength; /*!< Specifies the number of data bits transmitted or received in a frame.
This parameter can be a value of @ref UART_Word_Length */
uint32_t StopBits; /*!< Specifies the number of stop bits transmitted.
This parameter can be a value of @ref UART_Stop_Bits */
uint32_t Parity; /*!< Specifies the parity mode.
This parameter can be a value of @ref UART_Parity
@note When parity is enabled, the computed parity is inserted
at the MSB position of the transmitted data (9th bit when
the word length is set to 9 data bits; 8th bit when the
word length is set to 8 data bits). */
uint32_t Mode; /*!< Specifies whether the Receive or Transmit mode is enabled or disabled.
This parameter can be a value of @ref UART_Mode */
uint32_t HwFlowCtl; /*!< Specifies whether the hardware flow control mode is enabled or disabled.
This parameter can be a value of @ref UART_Hardware_Flow_Control */
uint32_t OverSampling; /*!< Specifies whether the Over sampling 8 is enabled or disabled, to achieve higher speed (up to fPCLK/8).
This parameter can be a value of @ref UART_Over_Sampling. This feature is only available
on STM32F100xx family, so OverSampling parameter should always be set to 16. */
} UART_InitTypeDef;
typedef enum
{
HAL_UART_STATE_RESET = 0x00U, /*!< Peripheral is not yet Initialized
Value is allowed for gState and RxState */
HAL_UART_STATE_READY = 0x20U, /*!< Peripheral Initialized and ready for use
Value is allowed for gState and RxState */
HAL_UART_STATE_BUSY = 0x24U, /*!< an internal process is ongoing
Value is allowed for gState only */
HAL_UART_STATE_BUSY_TX = 0x21U, /*!< Data Transmission process is ongoing
Value is allowed for gState only */
HAL_UART_STATE_BUSY_RX = 0x22U, /*!< Data Reception process is ongoing
Value is allowed for RxState only */
HAL_UART_STATE_BUSY_TX_RX = 0x23U, /*!< Data Transmission and Reception process is ongoing
Not to be used for neither gState nor RxState.
Value is result of combination (Or) between gState and RxState values */
HAL_UART_STATE_TIMEOUT = 0xA0U, /*!< Timeout state
Value is allowed for gState only */
HAL_UART_STATE_ERROR = 0xE0U /*!< Error
Value is allowed for gState only */
} HAL_UART_StateTypeDef;
typedef struct __UART_HandleTypeDef
{
USART_TypeDef *Instance; /*!< UART registers base address */
UART_InitTypeDef Init; /*!< UART communication parameters */
uint8_t *pTxBuffPtr; /*!< Pointer to UART Tx transfer Buffer */
uint16_t TxXferSize; /*!< UART Tx Transfer size */
__IO uint16_t TxXferCount; /*!< UART Tx Transfer Counter */
uint8_t *pRxBuffPtr; /*!< Pointer to UART Rx transfer Buffer */
uint16_t RxXferSize; /*!< UART Rx Transfer size */
__IO uint16_t RxXferCount; /*!< UART Rx Transfer Counter */
HAL_LockTypeDef Lock; /*!< Locking object */
__IO HAL_UART_StateTypeDef gState; /*!< UART state information related to global Handle management
and also related to Tx operations.
This parameter can be a value of @ref HAL_UART_StateTypeDef */
__IO HAL_UART_StateTypeDef RxState; /*!< UART state information related to Rx operations.
This parameter can be a value of @ref HAL_UART_StateTypeDef */
__IO uint32_t ErrorCode; /*!< UART Error code */
}UART_HandleTypeDef;
typedef enum
{
UART_FIFO_TX_NOT_FULL,
UART_FIFO_TX_EMPTY,
UART_FIFO_RX_NOT_EMPTY,
} HAL_UART_WaitFlagDef;
Macro Parameters
#define UART0 ((USART_TypeDef *)UART0_BASE)
#define UART1 ((USART_TypeDef *)UART1_BASE)
#define UART2 ((USART_TypeDef *)UART2_BASE)
#define UART3 ((USART_TypeDef *)UART3_BASE)
#define UART4 ((USART_TypeDef *)UART4_BASE)
#define UART5 ((USART_TypeDef *)UART5_BASE)
#define UART_FIFO_FULL 32
#define HAL_UART_ERROR_NONE 0x00000000U /*!< No error */
#define HAL_UART_ERROR_FE 0x00000040U /*!< Frame error */
#define HAL_UART_ERROR_PE 0x00000080U /*!< Parity error */
#define HAL_UART_ERROR_ORE 0x00000100U /*!< Overrun error */
#define UART_WORDLENGTH_5B ((uint32_t)UART_LC_DATAL_5BIT)
#define UART_WORDLENGTH_6B ((uint32_t)UART_LC_DATAL_6BIT)
#define UART_WORDLENGTH_7B ((uint32_t)UART_LC_DATAL_7BIT)
#define UART_WORDLENGTH_8B ((uint32_t)UART_LC_DATAL_8BIT)
#define UART_STOPBITS_1 0x00000000
#define UART_STOPBITS_2 ((uint32_t)UART_LC_STOP)
#define UART_PARITY_NONE 0x00000000
#define UART_PARITY_EVEN ((uint32_t)UART_LC_PCE)
#define UART_PARITY_ODD ((uint32_t)(UART_LC_PCE | UART_LC_PS))
#define UART_HWCONTROL_NONE 0x00000000U
#define UART_HWCONTROL_RTS ((uint32_t)UART_FC_AFCE)
#define UART_HWCONTROL_CTS ((uint32_t)UART_FC_AFCE)
#define UART_HWCONTROL_RTS_CTS ((uint32_t)UART_FC_AFCE)
#define UART_MODE_RX ((uint32_t)UART_LC_RE)
#define UART_MODE_TX ((uint32_t)UART_LC_TE)
#define UART_MODE_TX_RX ((uint32_t)(UART_LC_RE | UART_LC_TE))
#define UART_STATE_DISABLE 0x00000000U
#define UART_STATE_ENABLE ((uint32_t)(UART_LC_RE | UART_LC_TE))
Macro
#define __HAL_UART_ENABLE(__HANDLE__) ((__HANDLE__)->Instance->LC |= (UART_LC_RE | UART_LC_TE))
#define __HAL_UART_DISABLE(__HANDLE__) ((__HANDLE__)->Instance->LC &= ~(UART_LC_RE | UART_LC_TE))
Previous article:[Lianshengde W806 Hands-on Notes] VII. I2C
Next article:[Lianshengde W806 Hands-on Notes] 5. TIM Timer
Recommended ReadingLatest update time:2024-11-16 12:51
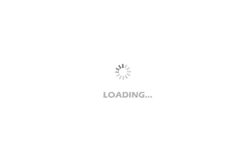
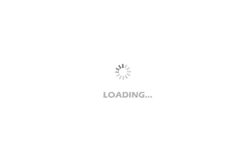
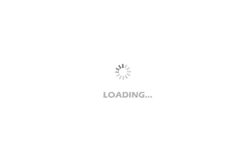
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- EEWORLD University Hall - Altium Designer 22 Electronic Design Introduction Practice 56 Lectures
- ztool cannot connect
- #Idle Market# Exchange Downloader
- ADS1278 data acquisition chip data distortion problem
- USB power supply and DC power supply issues
- Fundamentals of MOSFET and IGBT Gate Driver Circuits
- TI_DSP_SRIO - DirectIO Operations - Basics
- stm32f103vet6 industrial control board and 485 protocol sensor
- EEWORLD University - Cadence Allegro 17.4 Quadcopter Full Zero-Based Introductory Course
- How to find MSP430 program examples on TI's official website