The frequency spectrum of sound ranges from approximately tens to thousands of hertz. If you can use a program to control a certain port of the microcontroller to continuously output "high" or "low" levels, a square wave of a certain frequency can be generated on the port. Connecting the square wave to a speaker can produce a sound of a certain frequency. If you use the program to control the duration of the "high" or "low" levels, you can change the frequency of the output waveform and thus change the tone.
In the music, each note corresponds to a certain frequency. Table 1 shows the frequencies of each note in the key of C. If the frequency of the "high" and "low" levels output by a certain line of the microcontroller is the same as the frequency of a certain note, then the sound of this note can be produced by connecting this line to a speaker.
This system is designed based on this principle. For the AT89C2051 microcontroller, to generate a square wave of a certain frequency, it is roughly to first output a high level on a certain line, then delay for a period of time and then output a low level. In this way, the output of the cycle will generate a square wave of a certain frequency. By changing the delay time, the frequency of the output square wave can be changed. There are two main methods for microcontroller delay:
The first method is to use a loop statement to achieve delay, let the MCU execute a certain instruction in a loop, and then calculate the delay time based on the execution time of each instruction of the MCU and the number of loops. As shown below:
It can be seen from the delay program above that the execution time of the DJNZ instruction is 2 machine cycles, the execution time of the MOV instruction is 1 machine cycle, and the machine cycle is 1μs when the crystal frequency of the single-chip microcomputer is 12MHz. Therefore, according to the execution time of these instructions and the number of cycles of each instruction, the delay time of the above delay program can be calculated to be about 50ms. However, the delay time calculated by this method is not very accurate and very complex calculations must be performed to achieve a certain delay time. Therefore, this method is only used when the delay time requirements are not strict. However, for the electronic piano circuit, since the frequency value of each note is strictly required and the range of variation cannot be too large, the frequency of the square wave is also strictly required, and the delay program cannot be used to generate this square wave.
The second method is to use the timer/counter of the microcontroller to delay. The AT89C2051 microcontroller has two 16-bit timer/counters T0 and T1. The timer/counter of the microcontroller is actually a counting device. It can count the internal crystal oscillator clock of the microcontroller and the external input pulse. When counting the internal crystal oscillator clock of the microcontroller, it is called a timer, and when counting the external clock, it is called a counter. When counting the internal crystal oscillator clock of the microcontroller, the count value of the timer/counter is increased by 1 for each machine cycle. When the count value reaches the maximum count value, the count is completed and the CPU of the microcontroller is notified; when counting the external input clock signal, the count value of the timer/counter is increased by 1 for each rising edge of the external clock. When the count value reaches the maximum count value, the count is completed and the CPU of the microcontroller is notified. Therefore, if the machine cycle of the microcontroller or the cycle of the external input clock signal is known, the microcontroller can calculate the timing time according to the count value of the timer. This method is very accurate in timing. If you want to get a certain delay time, you can assign a certain count initial value to the timer. The timer starts from the preset count initial value and continues to increase by 1. When it reaches the maximum count value, the count is completed. By adjusting the size of the count initial value, the timer timing can be adjusted, thereby achieving accurate delay. This system uses the second method to delay through the timer/counter.
The specific circuit of this system is shown in the figure on the right. In the figure, P1.1-P1.7 are connected to 7 buttons respectively, corresponding to the seven notes 1, 2, 3, 4, 5, 6, and 7 in the music. P3.6 port is connected to the speaker through the power amplifier chip LM386. When one of the buttons of P1.1~P1.7 is pressed, the single-chip microcomputer executes the corresponding subroutine to assign a counting initial value to the timer and make P3.6 port output a high level. When the timer is finished, the value of P3.6 port is inverted and the counting initial value is reassigned to continue counting. When the counting is completed again, the value of P3.6 port is inverted and the initial value is assigned to count again. In this way, a square wave of a certain frequency is generated at P3.6 port. LM386 amplifies this square wave and outputs it through the speaker to produce the sound of the corresponding note. Press different buttons and the single-chip microcomputer will execute different subroutines to assign different initial values to the timer to obtain square waves of different frequencies and output different sounds. Therefore, pressing a button outputs a note.
There are 6 special function registers (TH1, TH0, TL1, TL0, TMOD, TCON) in the microcontroller that are used to control the timer of the microcontroller. By programming the read and write of these special function registers, the two timers T0 and T1 of the microcontroller can be controlled. When the microcontroller is reset, the default values of these 6 registers are 00H.
(I) Working mode and control word of timer/counter
Among the special function registers, TMOD and TCON are the timer mode control registers. Figure 2 shows the internal structure of the TMOD register, and Figure 3 shows the internal structure of the TCON register. TMOD and TCON are the names of the registers. When writing programs, we can directly use these names to specify them. Of course, we can also directly use their addresses 89H and 88H to specify them (actually, using the names means directly using the addresses, and the assembly software will help you translate it).
As can be seen from Figure 2, TMOD is divided into two parts, each with 4 bits, which are used to control T1 and T0 respectively.
As can be seen from Figure 3, TCON is also divided into two parts, the upper 4 bits are used for the timer/counter, and the lower 4 bits are used for interrupts.
The single-chip timer/counter has four working modes: mode 0, mode 1, mode 2, and mode 3. Except for mode 3, T0 and T1 have exactly the same working state. The following takes T1 as an example to describe the characteristics and usage of each mode. Figure 4 is the internal structure of the timer/counter T1 in working mode 0.
From Figure 4, it can be seen that the counting pulse must be 1 to enter the counter TR1 (or TR0), the switch can be closed, and the pulse can come. Therefore, TR1 (O) is called the operation control bit, which can be set by instruction SErB to start the counter and timer operation, and instruction CLR to shut down the timer/counter. When the counting pulse enters the counter, the counting pulse is added to the lower 5 bits of TL1, and it continues to increase by 1 from the preset initial count value. When TL1 is full, it carries to TH1. When TL1 and TH1 are both full, the timer return zero flag TF1 of T1 is set, indicating that the timing time or the number of counts has expired. The microcontroller can judge the state of the timer according to the flag bit, and execute the corresponding program.
1. Working mode 0
The working mode O of the timer and counter is called the 13-bit timer/counter mode. It is composed of the lower 5 bits of TL (1/0) and the 8 bits of TH (0/1) to form a 13-bit counter. At this time, the upper 3 bits of TL (1/0) are not used.
The working mode of the timer can be set according to the timer register TMOD:
①M1M0: The timer/counter has four working modes, which are controlled by M1M0. Two bits have exactly four combinations.
②C/T: The timer/counter can be used for timing or counting. If C/T is 0, it is used as a timer (the switch is turned up), and if C/T is 1, it is used as a counter (the switch is turned down). A timer/counter can be used for either timing or counting at the same time, but not both.
③GATE: When we select the timing or counting working mode, the timing/counting pulse may not reach the counter end. There is a switch in the middle. Obviously, if this switch is not closed, the counting pulse cannot pass. So when does the switch pass? There are two situations.
GATE=0. Analyze the logic. GATE is 1 after being negated and enters the OR gate. The OR gate always outputs 1, which has nothing to do with the other input terminal INT1 of the OR gate. In this case, the opening and closing of the switch only depends on TR1. As long as TR1 is 1, the switch is closed and the counting pulse can pass smoothly. If TR1 is equal to 0, the switch is open and the counting pulse cannot pass. Therefore, whether the timing/counting works depends only on TR1.
GATE=1, in this case, the switch on the counting pulse path is not only controlled by TR1, but also by the INT1 pin. Only when TR1 is 1 and the INT1 pin is also high, the switch is closed and the counting pulse can pass. This feature can be used to measure the width of the high level of a signal.
2. Working method 1
Working mode 1 is a 16-bit timing/counting mode. Just set M1M0 to 01. Other characteristics are the same as working mode 0.
3: Working method 2
8-bit automatic loading time constant mode. TL1 constitutes an 8-bit counter, and TH1 is only used to store the time constant. As shown in Figure 5, whenever the count overflows, the switch between the high and low 8 bits of T(0/1) will be turned on, and the preset count will enter the low 8 bits. This is done automatically by hardware and does not require manual intervention. Usually this mode of operation is used for baud rate generators. When used for this purpose, the timer is to provide a time reference. There is nothing to do after the count overflows. The only thing to do is to reload the preset count and start counting again, and there should be no delay in the middle. It can be seen that this task is best completed using working mode 2.
4. Working method 3
Two 8-bit counters are only suitable for timer 0. In this operation mode, timer/counter 0 is split into two independent timers/counters. Among them, TL0 can form an 8-bit timer or counter operation mode, while THO can only be used as a timer. We know that the control bit TR0 is required to use it as a timer or counter, and the overflow flag TF0 is required when the count is full. T0 is divided into two, so two sets of control and overflow flags are required. TLO still uses the original T0 flag, while TH0 borrows the T1 flag. In this way, T1 has no flag and control is available. Therefore, T0 is generally allowed to work in mode 3 only when T1 is running in mode 2 (when used as a baud rate generator).
(II) Timing/Counting Range of Timer/Counter
Working mode O: 13-bit timing/counting mode, therefore, the maximum time that can be counted is 2^13, that is, 8192 times. For a 12MHz crystal oscillator, the machine cycle of the microcontroller is 1us. The maximum timing time of working mode 0 is 8.192us.
Working mode 1: 16-bit timing/counting mode, therefore, the maximum time that can be counted is 2^16, that is, 65536 times. For a 12MHz crystal oscillator, the maximum timing time of working mode 1 is 65536μs.
Both working modes 2 and 3 are 8-bit timing/counting modes, so they can count up to 2^8, or 256 times. For a 12MHz crystal oscillator, the maximum timing time of working mode 1 is 256μs.
The calculation method of the initial count value is to subtract the required number of counts from the maximum count value. For example: if T0 is running in the timing state, the crystal oscillator of the microcontroller is 12MHz, and the timing is required to be 100us, then the timer needs to count 100 machine cycles. When T0 works in working mode O, the initial count value should be 8192-100=8092; when working in mode 1, the initial count value should be 65536-100=65436; when working in modes 2 and 3, the initial count value should be 256-100=156.
The system software flow chart is shown in the figure
When the machine is turned on, the first step is to initialize timer T0 and set its working state (for this system, T0 is set to working mode O); then determine whether a key is pressed. If no key is pressed, continue to determine. If a key is pressed, determine which key is pressed; then load the initial count value into timer T0 according to the function of the key and start T0. When T0 is finished, reload the initial count value to continue timing and invert P3.6. After the timing is finished again, reload the initial count value to continue timing and invert P3.6. Repeat this operation until the key is released. After the key is released, stop T0 and determine whether another key is pressed again, and continue the previous process.
Timer T0 works in mode 0, 13-bit counting mode, so the maximum number of counts is 2^13=8192 times. Since the crystal oscillator of the microcontroller is 12MHz, the machine cycle is 1μs, that is, the maximum time the timer can count is 8192μs. Half of the cycle of each note, that is, the duration of the high level or low level, is the time the timer needs to count, so the calculation method for the initial value of each note is as follows:
Assume the frequency of the note is f, then the initial count value = 8192-the period of each note = 8192-
The initial count value is shown in Table 2:
Previous article:Design and implementation of CAN bus repeater based on C8051F040
Next article:Design of an External Nerve Stimulator Based on AT89C52
Recommended ReadingLatest update time:2024-11-16 19:51
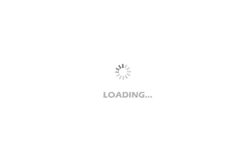
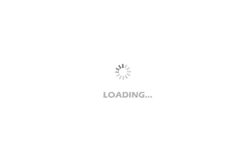
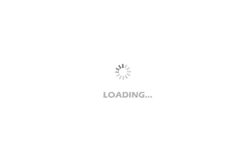
- Popular Resources
- Popular amplifiers
-
Learning MCU Technology from Scratch (Liu Jianqing)
-
Wireless doorbell alarm based on AT89C2051 single chip microcomputer
-
Infrared remote control LED electronic clock using real-time clock chip DS1302+AT89C2051
-
Infrared remote control LED electronic clock using real-time clock chip DS1302+AT89C2051.rar
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- SHT31 evaluation + second proofing expansion board
- [Scientific Epidemic Prevention] How does the infrared body temperature rapid screening device build the first line of defense?
- The live broadcast with prizes will start at 10:00 this morning: "The use of SOI level conversion driver IC in LLC topology"
- [Blood Oximeter] Disassembly Part 3 Background Knowledge
- [Silicon Labs BG22-EK4108A Bluetooth Development Evaluation] Burn Bootloader + Debug Bluetooth Lighting
- Altium Designer 19.0.10 The shortcut key for aligning left is not available?
- The price of domestic chip substitutes is only 1/5 of imported ones. Will domestic companies have a great opportunity?
- TI reference circuit solution for electric vehicle power management
- S digital receiving channel architecture
- The role of adding a reverse diode to the be pole of the transistor