DHT11 is a temperature and humidity sensor with calibrated digital signal output. The accuracy is +-5%RH, the temperature is +-2℃, the range is 20-90%RH, and the temperature is 0~50℃.
1. Circuit connection analysis
1. Pin diagram
2. Wiring Diagram
The DHT11 device uses a simplified single bus communication. A single bus has only one data line, and the data exchange and control in the system are all completed by the single bus. The single bus usually requires an external pull-up resistor of about 5.1kΩ, so that when the bus is idle, its state is high. Since they are master-slave junctions, the slave can only respond when the host calls the slave. Therefore, the host must strictly follow the single bus sequence when accessing the device. If the sequence is confused, the device will not respond to the host.
2. Data Collection and Analysis
1. Total data timing
After the user host (MCU) sends a start signal, DHT11 switches from low power mode to high speed mode. After the host start signal ends, DHT11 sends a response signal, sends out 40-bit data, and triggers a signal collection.
2. The host sends a start signal
The I/O port of the microcontroller connected to the DATA pin of DHT11 outputs a low level, and the low level retention time cannot be less than 18ms, and then waits for DHT11 to make a response signal.
3. Detect the slave response signal
When the DATA pin of DHT11 detects a low level external signal, it waits for the low level of the external signal to end. After a delay, the DATA pin of DHT11 is in the output state, outputs a low level for 80 microseconds as a response signal, and then outputs a high level for 80 microseconds to notify the peripheral device to prepare to receive data.
4. Receive data
(1) Data judgment rules
The format of the bit data "0" is: 50 microseconds of low level and 26-28 microseconds of high level, and the format of the bit data "1" is: 50 microseconds of low level plus 70 microseconds of high level.
When receiving data, you can first wait for the low level to pass, that is, wait for the data line to be pulled high, and then delay for 60us, because 60us is greater than 28us and less than 70us, and then detect whether the data line is high at this time. If it is high, the data is judged to be 1, otherwise it is 0.
(2) Data format
40 bits of data are transmitted at a time, high bit first out
8-bit humidity integer data + 8-bit humidity decimal data + 8-bit temperature integer data + 8-bit temperature decimal data + 8-bit check bit.
(3) Data correction
Determine whether the result of "8-bit humidity integer data + 8-bit humidity decimal data + 8-bit temperature integer data + 8-bit temperature decimal data" is equal to the 8-bit check bit. If it is equal, the data is received correctly, otherwise the data should be discarded and received again.
3. Driver
#include #include #define uchar unsigned char #define uint unsigned int sbit Data=P3^6; //define data line uchar rec_dat[9]; //Received data array for display void DHT11_delay_us(uchar n) { while(--n); } void DHT11_delay_ms(uint z) { uint i,j; for(i=z;i>0;i--) for(j=110;j>0;j--); } void DHT11_start() { Data=1; DHT11_delay_us(2); Data=0; DHT11_delay_ms(20); //delay more than 18ms Data=1; DHT11_delay_us(30); } uchar DHT11_rec_byte() //Receive a byte { uchar i,dat=0; for(i=0;i<8;i++) //Receive 8 bits of data from high to low { while(!Data); Wait for 50us low level to pass DHT11_delay_us(8); // Delay 60us, if it is still high, the data is 1, otherwise it is 0 dat<<=1; // Shift to receive 8-bit data correctly, shift directly when the data is 0 if(Data==1) //When data is 1, add 1 to dat to receive data 1 dat+=1; while(Data); //Wait for the data line to be pulled low } return dat; } void DHT11_receive() //Receive 40 bits of data { uchar R_H,R_L,T_H,T_L,RH,RL,TH,TL,revise; DHT11_start(); if(Data==0) { while(Data==0); //Wait for high DHT11_delay_us(40); //Delay 80us after pulling high R_H=DHT11_rec_byte(); //Receive high eight bits of humidity R_L=DHT11_rec_byte(); //Receive the lower eight bits of humidity T_H=DHT11_rec_byte(); //Receive high eight bits of temperature T_L=DHT11_rec_byte(); //Receive the lower eight bits of temperature revise=DHT11_rec_byte(); //Receive correction bit DHT11_delay_us(25); //End if((R_H+R_L+T_H+T_L)==revise) //correction { RH=R_H; RL=R_L; TH=T_H; TL=T_L; } /*Data processing, convenient display*/ rec_dat[0]='0'+(RH/10); rec_dat[1]='0'+(RH%10); rec_dat[2]='R'; rec_dat[3]='H'; rec_dat[4]=' '; rec_dat[5]=' '; rec_dat[6]='0'+(TH/10); rec_dat[7]='0'+(TH%10); rec_dat[8]='C'; } } /* LCD1602 is used here to display the collected data. The LCD1602 driver is explained in detail in the blogger's 51 microcontroller series blog*/ void main() { uchar i; lcd_init(); //lcd1602 initialization while(1) { DHT11_delay_ms(1500); //After DHT11 is powered on, it needs to wait for 1S to get past the unstable state. No instructions can be sent during this period. DHT11_receive(); lcd_write_command(0x80); //Start displaying from the first position of the first line of lcd1602 for(i=0;i<9;i++) lcd_write_data(rec_dat[i]); //display data } }
Previous article:51 MCU AD conversion PCF8591
Next article:Blue Bridge Cup MCU Design and Development Notes (Part 2)
Recommended ReadingLatest update time:2024-11-23 08:33
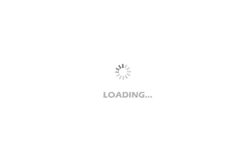
- Naxin Micro and Xinxian jointly launched the NS800RT series of real-time control MCUs
- How to learn embedded systems based on ARM platform
- Summary of jffs2_scan_eraseblock issues
- Application of SPCOMM Control in Serial Communication of Delphi7.0
- Using TComm component to realize serial communication in Delphi environment
- Bar chart code for embedded development practices
- Embedded Development Learning (10)
- Embedded Development Learning (8)
- Embedded Development Learning (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Intel promotes AI with multi-dimensional efforts in technology, application, and ecology
- ChinaJoy Qualcomm Snapdragon Theme Pavilion takes you to experience the new changes in digital entertainment in the 5G era
- Infineon's latest generation IGBT technology platform enables precise control of speed and position
- Two test methods for LED lighting life
- Don't Let Lightning Induced Surges Scare You
- Application of brushless motor controller ML4425/4426
- Easy identification of LED power supply quality
- World's first integrated photovoltaic solar system completed in Israel
- Sliding window mean filter for avr microcontroller AD conversion
- What does call mean in the detailed explanation of ABB robot programming instructions?
- STMicroelectronics discloses its 2027-2028 financial model and path to achieve its 2030 goals
- 2024 China Automotive Charging and Battery Swapping Ecosystem Conference held in Taiyuan
- State-owned enterprises team up to invest in solid-state battery giant
- The evolution of electronic and electrical architecture is accelerating
- The first! National Automotive Chip Quality Inspection Center established
- BYD releases self-developed automotive chip using 4nm process, with a running score of up to 1.15 million
- GEODNET launches GEO-PULSE, a car GPS navigation device
- Should Chinese car companies develop their own high-computing chips?
- Infineon and Siemens combine embedded automotive software platform with microcontrollers to provide the necessary functions for next-generation SDVs
- Continental launches invisible biometric sensor display to monitor passengers' vital signs
- Please help me
- download
- Switch OUT?! Nintendo's latest recruitment may be aimed at the next generation console
- Share some RISC-V resources
- This article explains the differences between EMI, EMS and EMC
- What makes "tomorrow's travel" just around the corner?
- Selection and performance considerations of GPS smart antennas in system integration
- Why is photolithography simulation software used in the semiconductor manufacturing process?
- Pressure sensor controlled motor
- Verilog Basic Syntax - Module