Usually after installing the exception vector table, we jump to the entry of the handler we defined ourselves. At this time, we have not saved the scene of the interrupted program, so we must first save the interrupted program scene in the entry of the exception handler.
3. Save the execution scene
At the beginning of the exception handling program, it is necessary to save the execution scene of the interrupted program. The execution scene of the program is nothing more than saving the data in the current operation register. The following stack operation instructions can be used to save the scene:
STMFD SP_excep!, {R0 – R12, LR_excep}
Note: LR_abt and SP_excep correspond to LR and SP in the abnormal mode respectively. _abt is added for the convenience of readers to understand
It should be noted that when jumping to the exception handler entry, it has already switched to the corresponding exception mode, so the SP here is SP_excep in the exception mode, so the interrupted program scene (register data) is saved in the stack in the exception mode. The above instructions save all R0~R12 to the exception mode stack, and finally save the modified return address of the interrupted program into the stack. The reason for saving the return address is that in the future, it can be returned to the user program through instructions similar to: MOV PC, LR to continue execution.
After an exception occurs, it must be processed according to the exception type. Therefore, each exception has its own exception handler. The interrupt exception handling process is analyzed through the system interrupt handling in the next section.
5. Return of exception handling
After the exception handling is completed, the interrupted program is returned to continue execution. The specific operations are as follows:
Restore register data when the program is interrupted
Restore the program runtime state CPSR
Return to the interrupted program and continue execution by returning to the return address saved when entering the exception
1. Exception return address
The execution of an instruction is divided into three main stages: instruction fetch, decoding, and execution. Because the CPU uses pipeline technology, the address of the currently executed instruction should be PC-8 (four bytes for one instruction on a 32-bit machine), so the next instruction to be executed should be PC-4. When an exception occurs, the CPU will automatically save the value of PC-4 to LR, but whether the value is correct depends on the type of exception.
The return address of each mode is described as follows:
Normal/Fast Interrupt Request:
The return processing of fast interrupt request and general interrupt request is the same. Usually, after the processor executes the current instruction, it queries the FIQ/IRQ interrupt pin and checks whether the FIQ/IRQ interrupt is allowed. If an interrupt pin is valid and the system allows the interrupt to occur, the processor will generate a FIQ/IRQ exception interrupt. When the FIQ/IRQ exception interrupt occurs, the value of the program counter pc has been updated, and it points to the third instruction after the current instruction (for ARM instructions, it points to the current instruction address plus 12 bytes; for Thumb instructions, it points to the current instruction address plus 6 bytes). When the FIQ/IRQ exception interrupt occurs, the processor saves the value (pc-4) to the register lr_irq/lr_irq in the FIQ/IRQ exception mode, which points to the second instruction after the current instruction. Therefore, the correct return address can be calculated by the following instructions:
SUBS PC, LR_irq, #4; general interrupt
SUBS PC, LR_fiq, #4; Fast interrupt
Note: LR_irq/LR_fiq are LRs in general interrupt and fast interrupt exception modes respectively. There is no LR_xxx register. _xxx is added for the convenience of readers. The same applies below.
Prefetch abort exception:
During instruction prefetching, if the target address is illegal, the instruction is marked as a problematic instruction. At this time, the instructions before the instruction on the pipeline continue to execute. When the instruction marked as problematic is executed, the processor generates an instruction prefetch abort exception interrupt. When an instruction prefetch abort exception occurs, the program must return to the problematic instruction, re-read and execute the instruction. Therefore, the instruction prefetch abort exception interrupt should return to the instruction that generated the instruction prefetch abort exception interrupt, rather than the next instruction of the current instruction.
The instruction prefetch abort exception interrupt is generated when the currently executed instruction is executed in the ALU. When the instruction prefetch abort exception interrupt occurs, the value of the program counter pc has not been updated. It points to the second instruction after the current instruction (for ARM instructions, it points to the current instruction address plus 8 bytes; for Thumb instructions, it points to the current instruction address plus 4 bytes). At this time, the processor saves the value (pc-4) to lr_abt, which points to the next instruction of the current instruction, so the return operation can be implemented by the following instruction:
SUBS PC, LR_abt, #4
Undefined instruction exception:
The undefined instruction exception interrupt is generated when the currently executed instruction is executed in the ALU. When the undefined instruction exception interrupt occurs, the value of the program counter pc has not been updated. It points to the second instruction after the current instruction (for ARM instructions, it points to the current instruction address plus 8 bytes; for Thumb instructions, it points to the current instruction address plus 4 bytes). When the undefined instruction exception interrupt occurs, the processor saves the value (pc-4) to lr_und. At this time, (pc-4) points to the next instruction of the current instruction, so returning from the undefined instruction exception interrupt can be achieved through the following instructions:
MOV PC, LR_und
Soft interrupt instruction (SWI) exception:
The SWI exception interrupt is the same as the undefined exception interrupt instruction. It is also generated when the currently executed instruction is executed in the ALU. When the SWI instruction is executed, the value of pc has not been updated. It points to the second instruction after the current instruction (for ARM instructions, it points to the current instruction address plus 8 bytes; for Thumb instructions, it points to the current instruction address plus 4 bytes). When the undefined instruction exception interrupt occurs, the processor saves the value (pc-4) to lr_svc. At this time, (pc-4) points to the next instruction of the current instruction. Therefore, the implementation method of returning from the SWI exception interrupt processing is the same as returning from the undefined instruction exception interrupt processing:
MOV PC, LR_svc
Data Abort Exception:
When a data access abort occurs, the program needs to return to the problematic instruction and re-access the data. Therefore, the data access abort should return to the instruction that generated the data access abort abort, rather than the next instruction of the current instruction.
The data access exception interrupt is generated when the currently executed instruction is executed in the ALU. When the data access exception interrupt occurs, the value of the program counter pc has been updated, and it points to the third instruction after the current instruction (for ARM instructions, it points to the current instruction address plus 12 bytes; for Thumb instructions, it points to the current instruction address plus 6 bytes). At this time, the processor saves the value (pc-4) to lr_abt, which points to the second instruction after the current instruction, so the return operation can be implemented by the following instruction:
SUBS PC, LR_abt, #8
When each of the above exceptions occurs, the return address must be repaired according to the specific exception type. Once again, the return address of the interrupted program is saved in LR_excep in the corresponding exception mode.
2. Mode recovery
After an exception occurs, when entering the exception handling program, the data in the user program registers R0~R12 are saved in the stack under the exception mode. When returning after the exception handling, the data saved in the stack must be restored to the original R0~R12.
There is no doubt that during the exception handling process, it is necessary to ensure that the stack pointer SP_excep is the same at the entry and exit of the exception handling. Otherwise, the data restored to R0~R12 will be incorrect, and the execution scene will be inconsistent when returning to the interrupted program, causing problems. Although the execution scene is restored, it is still in exception mode at this time, and the status in CPSR is the status in exception mode.
Therefore, it is necessary to restore the saved state in SPSR_excep to CPSR. SPSR_excep is the state when the program is interrupted. When restoring SPSR_excep to CPSR, the CPU mode and state are switched from the exception mode back to the mode and state when the program was interrupted.
At this moment, the program scene is restored and the status is restored, but the value in PC still points to the address space in exception mode. We want the CPU to continue executing the interrupted program, so we need to manually change the value of PC to the return address when entering the exception. This address has been calculated at the entrance of the exception handling, so just set PC = LR_excep.
The above operations can be implemented step by step, but usually we can achieve all the above operations with one instruction:
LDMFD SP_excp!, {r0-r12, pc}^
Note: SP_excep is the SP in the corresponding exception mode, and the ^ symbol indicates restoring SPSR_excep to CPSR.
Previous article:7. Learn ARM-GNU pseudo instructions, code compilation, and lds usage from scratch
Next article:5. Learn ARM-MRS, MSR, addressing operation, and atomic operation principles from scratch
Recommended ReadingLatest update time:2024-11-16 23:53
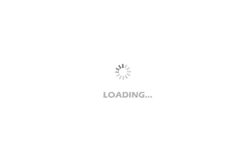
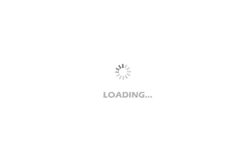
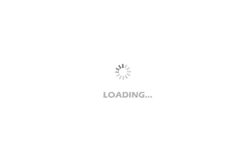
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Bluetooth, 4-Digit True RMS Digital Multimeter
- Zibee transparent transmission program development
- Download the 150,000+ word "RF and Microwave Technology Practical Handbook" for free here! (Internal Gift)
- World clock controlled by NXP LPC845
- Download the NI Practical Guide to Maximizing DC Measurement Performance
- PSRR Measurement of LDO
- [ESP32-Korvo Review] Part 2: Demo Experience + ESP-IDF Compilation Environment Installation
- Low EMI DC/DC Converter PCB Design
- I seldom deal with interfaces. Now that the speed is so high, I have to deal with single-ended signals and differential signals. If you see them, please share them.
- Banknote number recognition system based on ARM