6. Relationship between anomalies and patterns
reset abnormally enters SVC mode
FIQ fast interrupt request exception enters fast interrupt mode, supports high-speed data transmission and channel processing (enters this mode when FIQ exception response)
IRQ interrupt request exception enters interrupt mode, which is used for general interrupt processing (enters this mode when IRQ exception response)
Prefetch prefetch abort, data abort exception enters abort mode to support virtual memory and/or memory protection
undef Undefined instruction exception enters undefined mode, supports software emulation of hardware coprocessor (enters this mode when responding to undefined instruction exception)
SWI software interrupt, reset exception enters management mode, operating system protection code (enters this mode when the system is reset and software interrupt response)
7. IRQ interrupt exception
1. The concept of interruption
What is interruption? Let's introduce it from an example in life. We are reading a book at home, and suddenly the phone rings. You put down the book, answer the phone, talk to the caller, then put down the phone and come back to continue reading your book. This is the phenomenon of "interruption" in life, that is, the normal working process is interrupted by external events.
In the processor, an interrupt is a process, that is, when the CPU encounters an external/internal emergency event that needs to be handled during the normal execution of the program, it temporarily interrupts (stops) the execution of the current program and turns to serve the event. After the service is completed, it returns to the pause point (breakpoint) to continue executing the original program. The program that serves the event is called an interrupt service program or interrupt handler.
Strictly speaking, the above description refers to interrupts caused by hardware events. Interrupts can also be caused by software methods, that is, special instructions are arranged in the program in advance. When the CPU executes such instructions, it switches to execute the corresponding pre-arranged program, and then returns to execute the original program. This can be called a soft interrupt. Taking soft interrupts into consideration, the following definition can be given to interrupts: an interrupt is a process in which the CPU inserts another program into the process of executing the current program due to hardware or software reasons. The occurrence of the interrupt process caused by hardware reasons is unpredictable, that is, random, while soft interrupts are arranged in advance.
2. Interrupt handling process
When an interrupt exception occurs, the entire processing flow is:
As shown in FIG:
An interrupt is generated when execution reaches 0x30000008
cpu executes 4 big steps and 3 small steps
1) Save CPSR to SPSR_irq2) According to the exception type, set the mode flag CPSR[4:0], CPU execution status CPSR[5]: T bit = 0 and disable interrupts3) Set the return address LR = 0x300000104) Point PC to the corresponding exception vector table address [interrupt IRQ: 0x00000018]
After entering the exception vector table, execute instruction b and jump to the exception handling function
The exception handling function needs to do the following
1) Correct the return address SUBS PC, LR_irq, #4, which is 0x3000000C2) Save the context register3) Jump into the interrupt processing function isr_proccess() and execute the interrupt processing program4) Restore the context register5) Return to context PC=LR
1.
The program returns to 0x3000000C and continues to execute
A more detailed explanation of interrupts will be provided in subsequent articles, so please pay attention to [A Bit of Linux].
8. SWI abnormality
1. SWI instruction
The format of the SWI instruction is:
SWI{condition} 24-bit immediate value
The SWI instruction is used to generate a software interrupt so that the user program can call the operating system's system routine. The operating system provides the corresponding system services in the SWI exception handler. The 24-bit immediate value in the instruction specifies the type of system routine called by the user program, and the relevant parameters are passed through general registers. When the 24-bit immediate value in the instruction is ignored, the type of system routine called by the user program is determined by the content of general register R0, and the parameters are passed through other general registers.
Example:
SWI 0x02 ; This instruction calls the system routine numbered 02 of the operating system.
1.
2. BKPT instruction
The format of the BKPT instruction is:
BKPT 16-bit immediate value
The BKPT instruction generates a software breakpoint interrupt, which can be used for program debugging.
3. Examples
The following is a code containing an exception vector table. The program value fills in the entries of the reset exception and the swi exception. Other entry addresses can be filled in at this position with the empty instruction nop.
area first, code, readonly
code32
entry; Exception vector table defined
vector
b reset_handler ; Jump to reset_handler
nop
b swi_handler ; SWI instruction exception jump address
nop
nop
nop
nop
nop
swi_handler ; swi handler code
; Exception handling first needs to push the stack to save the processor scene
mrs r0, cpsr
bic r0, r0, #0x1f
orr r0, r0, #0x10
msr cpsr_c, r0
;ldr r0, [lr, #-4]; Get the machine code of the SWI instruction. The instruction before lr is the swi instruction, and the subscript is in this instruction ;bic r0, r0, #0xff000000 ; Get the SWI NUMBER through the machine code
movs pc, lr ; lr > pc and spsr -> cpsr returns SVC -> USER
reset_handler ; Initialize SVC mode stack
ldr sp, =0x40001000; Change the current mode from SVC mode to USER mode
mrs r0, cpsr
bic r0, r0, #0x1f
orr r0, r0, #0x10
msr cpsr_c, r0; Initialize USER mode stack
ldr sp, =0x40000800
mov r0, #1
; USER SWI
swi 5 ; open APP USER This statement is triggered by the user program itself.
; Observe and record the changes of PC LR CPSR SPSR SP before and after the execution of the comparison instruction
; and think about what changes automatically occurred in the processor hardware after the exception occurred
add r1, r0, r0
stop
b stop
end
The operation process is as follows:
Mainly pay attention to observe the changes in the pattern before and after swi is executed. You can analyze it according to 4 big steps and 3 small steps.
4. How to jump and switch modes at the same time?
When returning from a SWI exception, we need to perform two actions:
Copy spsr to cpsr,
pc = lr jump back to the original position
Both actions must be executed, but if they are executed step by step, after spsr is copied back, the current mode will change back to usr mode, and the corresponding lr value will become lr_usr, and the value at this time is 0x0 [bl instruction has not been executed before], so how can we jump there?
We can use the following command
movs pc, lr
This command performs two actions simultaneously:
pc = lr
cpsr = spsr returns SVC -> USER
This enables simultaneous jumping and mode switching.
If the entry has been pushed using ldm, it can be restored with the following command:
LDMFD SP_excp!, {r0-r12, pc}^
See Chapter 5.
5. How to get the soft interrupt number?
To obtain the interrupt number of the swi instruction, we can only get the corresponding value from the swi machine code.
2. In order to get the content of the swi instruction, we must first find the address of this instruction,
and the value of lr is the address of the next instruction of the swi instruction, so we can get the soft interrupt number through the following code.
ldr r0, [lr, #-4]; Get the machine code of the SWI instruction. The instruction before lr is the swi instruction. The subscript is in the instruction.
bic r0, r0, #0xff000000 ; Get SWI NUMBER through machine code
6. System calls and swi
System calls
Linux applications have many system calls, such as open, read, socket, etc., which will actually trigger swi exceptions, triggering system calls sys_open, sys_read, etc. The kernel performs specific operations based on the value of swi.
Each system call has its own unique number. The identifier of the system call function is defined in the following files:
linux/arch/arm/kernel/calls.S
The content is as follows:
/* 0 */CALL(sys_restart_syscall)CALL(sys_exit)CALL(sys_fork)CALL(sys_read)CALL(sys_write)
…………/* 375 */CALL(sys_setns)CALL(sys_process_vm_readv)CALL(sys_process_vm_writev)CALL(sys_kcmp)CALL(sys_finit_module)#ifndef syscalls_counted.equ syscalls_padding, ((NR_syscalls + 3) & ~3) - NR_syscalls#define syscalls_counted#endif.rept syscalls_padding CALL(sys_ni_syscall).endr
SWI code snippet analysis
Search for vector_swi and find the entry function
archarmkernelentry-common.S
.align 5ENTRY(vector_swi)@ Save the scene
sub sp, sp, #S_FRAME_SIZE
stmia sp, {r0 - r12} @ Calling r0 - r12
add r8, sp, #S_PC
stmdb r8, {sp, lr}^ @ Calling sp, lr
mrs r8, spsr @ called from non-FIQ mode, so ok.str lr, [sp, #S_PC] @ Save calling PC
str r8, [sp, #S_PSR] @ Save CPSR
str r0, [sp, #S_OLD_R0] @ Save OLD_R0
zero_fp
@ Get the instruction address of swi and make sure it is swi instruction
ldr scno, [lr, #-4] @ get SWI instructionA710( and ip, scno, #0x0f000000 @ check for SWI )A710( teq ip, #0x0f000000 )A710( bne .Larm710bug )
@tbl is equal to the array table base address
get_thread_info tsk
adr tbl, sys_call_table @ load syscall table pointer
ldr ip, [tsk, #TI_FLAGS] @ check for syscall tracing
@ Clear the upper 8 bits
bic scno, scno, #0xff000000 @ mask off SWI op-code
@ #define __NR_SYSCALL_BASE 0x900000 The value of swi here is actually 0x900000 0x900001 ...so we need to clear the high bit 9eor scno, scno, #__NR_SYSCALL_BASE @ check OS number
@According to the index number, call the function in the tbl array
@ tbl: array table base address, scno: index value of sys_write() to be called lsl #2: shift left 2 bits, a function pointer occupies 4 bytes
Previous article:7. Learn ARM-GNU pseudo instructions, code compilation, and lds usage from scratch
Next article:5. Learn ARM-MRS, MSR, addressing operation, and atomic operation principles from scratch
Recommended ReadingLatest update time:2024-11-16 23:51
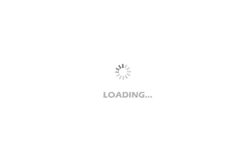
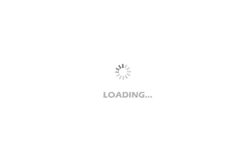
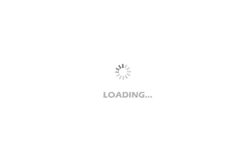
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- ODROID-GO handheld game console emulator based on ESP32
- [Liquid Level Sensor Evaluation] Re-understanding of Capacitive-Contactless Liquid Level Sensor
- LED PWM Dimming C Program
- FPGA introductory series experimental tutorial - key debounce control LED on and off
- Crazy Shell AI open source drone flight control machine code reading, compilation and burning
- TWS Headphones ANC Design Guide Basics 01
- What is c_int00 when programming DSP?
- Keil.STM32H7xx_DFP.2.2.0 installation package problem
- [Ultra-low power STM32U5 IoT Discovery Kit] - 2: wifi
- Why can't I debug CCS7.0 suddenly?