Debugging preparation
Compilation tool: MDK470a
Development board: s3c2410
Debugging method: Get the hex file after compiling with mdk, convert it into bin file with hex2bin tool, download it via USB, and observe it on DNW
#define GPFCON (*(volatile unsigned long*)0x56000050) #define GPFDAT (*(volatile unsigned long*)0x56000054) //===PORT F GROUP //Port: GPF7 GPF6 GPF5 GPF4 GPF3 GPF2 GPF1 GPF0 //Signal: LED_1 LED_2 LED_3 LED_4 FS2_INT GPLD_INT1 KEY_INT BUT_INT1 //Set properties: output output output output EINT3 EINT2 EINT1 EINT0 //Binary value: 01 01 01 01 00 00 00 00 int i,nout; int Main() { GPFCON = 0x00005500; //GPFDAT = 0x00000050; nout=0x000000F0; while(1) { GPFDAT=nout & 0x00000070; for(i=0; i<1000000; i++); GPFDAT=nout & 0x00000030; for(i=0; i<1000000; i++); GPFDAT=nout & 0x00000010; for(i=0; i<1000000; i++); GPFDAT=nout & 0x00000000; for(i=0; i<1000000; i++); //led(); } //return(0); } Phenomenon: LED1~4 turn on and off in a cycle with equal time intervals #define GPFCON (*(volatile unsigned long*)0x56000050) #define GPFDAT (*(volatile unsigned long*)0x56000054) int i,nout; void led(void) { GPFDAT=nout & 0x00000070; for(i=0; i<1000000; i++); GPFDAT=nout & 0x00000030; for(i=0; i<1000000; i++); GPFDAT=nout & 0x00000010; for(i=0; i<1000000; i++); GPFDAT=nout & 0x00000000; } int Main() { GPFCON = 0x00005500; //GPFDAT = 0x00000050; nout=0x000000F0; while(1) { GPFDAT=nout & 0x00000070; //μãááLED1 for(i=0; i<1000000; i++); GPFDAT=nout & 0x00000030; //LED1/LED2 for(i=0; i<1000000; i++); GPFDAT=nout & 0x00000010; //LED1/LED2/LED3 for(i=0; i<1000000; i++); GPFDAT=nout & 0x00000000; //LED1/LED2/LED3/LED4 for(i=0; i<1000000; i++); //led(); } //return(0); } Phenomenon: LED1 and LED3 light up almost at the same time, LED2 lights up after a short while, LED4 lights up after a while, and then the cycle stops. Note: Code-2 just adds a function in front of Code-1, but does not call it. #define GPFCON (*(volatile unsigned long*)0x56000050) #define GPFDAT (*(volatile unsigned long*)0x56000054) int i,nout; void led(void) { GPFDAT=nout & 0x00000070; for(i=0; i<1000000; i++); GPFDAT=nout & 0x00000030; for(i=0; i<1000000; i++); GPFDAT=nout & 0x00000010; for(i=0; i<1000000; i++); GPFDAT=nout & 0x00000000; } int Main() { GPFCON = 0x00005500; //GPFDAT = 0x00000050; nout=0x000000F0; while(1) { /* GPFDAT=nout & 0x00000070; //μãááLED1 for(i=0; i<1000000; i++); GPFDAT=nout & 0x00000030; //LED1/LED2 for(i=0; i<1000000; i++); GPFDAT=nout & 0x00000010; //LED1/LED2/LED3 for(i=0; i<1000000; i++); GPFDAT=nout & 0x00000000; //LED1/LED2/LED3/LED4 for(i=0; i<1000000; i++); */ led(); } //return(0); } Phenomenon: Same as Code-2 phenomenon #define rGPFCON (*(volatile unsigned *)0x56000050) #define rGPFDAT (*(volatile unsigned *)0x56000054) //#define rGPFUP (*(volatile unsigned *)0x56000058) void port_init(void) { rGPFCON=0x00005500; // rGPFUP=0x0000ff; } void led_on(void) { int i,nout; nout=0x000000F0; rGPFDAT=nout & 0x00000070; for(i=0; i<100000; i++); rGPFDAT=nout & 0x00000030; for(i=0; i<100000; i++); rGPFDAT=nout & 0x00000010; for(i=0; i<100000; i++); rGPFDAT=nout & 0x00000000; for(i=0; i<100000; i++); } /* void led_off(void) { int i,nout; nout=0; rGPFDAT=0; for(i=0; i<100000; i++); rGPFDAT=nout | 0x00000080; for(i=0; i<100000; i++); rGPFDAT=nout | 0x00000040; for(i=0; i<100000; i++); rGPFDAT=nout | 0x00000020; for(i=0; i<100000; i++); rGPFDAT=nout | 0x00000000; for(i=0; i<100000; i++); } void led_on_off(void) { int i; rGPFDAT=0; for(i=0; i<100000; i++); rGPFDAT=0x000000F0; for(i=0; i<100000; i++); } */ int main(void) { // port_init(); rGPFCON=0x00005500; while(1) { led_on(); // led_off(); // led_on_off(); } return(0); } /* int i,nout; void led(void) { GPFDAT=nout & 0x00000070; for(i=0; i<1000000; i++); GPFDAT=nout & 0x00000030; for(i=0; i<1000000; i++); GPFDAT=nout & 0x00000010; for(i=0; i<1000000; i++); GPFDAT=nout & 0x00000000; } int Main() { GPFCON = 0x00005500; //GPFDAT = 0x00000050; nout=0x000000F0; while(1) { GPFDAT=nout & 0x00000070; for(i=0; i<1000000; i++); GPFDAT=nout & 0x00000030; for(i=0; i<1000000; i++); GPFDAT=nout & 0x00000010; for(i=0; i<1000000; i++); GPFDAT=nout & 0x00000000; for(i=0; i<1000000; i++); //led(); } //return(0); } */ Phenomenon: After downloading, the development board restarts directly when running y analyze At present, it seems that only Code-1 has achieved the desired effect. I have a lot of comments in the source code, but since they are all garbled when pasted, I deleted them all. Code-1 and Code-2 should look the same on the PC platform, but the running results are very different. It should not be caused by the development board cache. It is probably caused by the compiler. <1> Maybe MDK optimized the code, not the linker, but during compilation <2>Code-4 causes the board to reboot directly. What is the reason? It seems that I can't solve these two problems now. Let's put it aside for now and ask the teacher or the experts to help solve them next time.
Previous article:【ARM】s3c2410 interrupt processing example
Next article:【ARM】arm·ad converter
Recommended ReadingLatest update time:2024-11-17 13:37
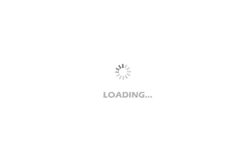
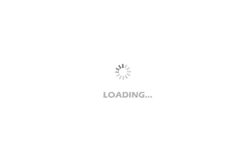
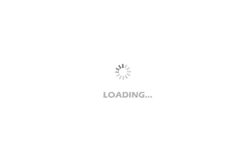
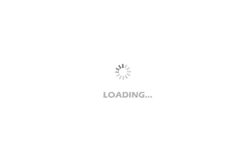
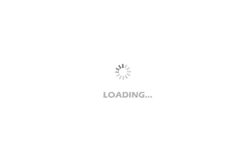
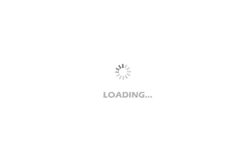
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- New breakthrough! Ultra-fast memory accelerates Intel Xeon 6-core processors
- New breakthrough! Ultra-fast memory accelerates Intel Xeon 6-core processors
- Consolidating vRAN sites onto a single server helps operators reduce total cost of ownership
- Consolidating vRAN sites onto a single server helps operators reduce total cost of ownership
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- Circuit Design_Buzzer Tips
- STTS751 High Accuracy Temperature Sensor Extension for MakeCode
- [Qinheng RISC-V core CH582] 1. Development environment installation
- [Sipeed LicheeRV 86 Panel Review] Using crosstool-ng to build a compiler for riscv64
- "Domestic Chip Exchange" is launched! How to do it is up to you!
- [Erha Image Recognition Artificial Intelligence Vision Sensor] Evaluation of Four Face Algorithms
- [ESP32-Audio-Kit Audio Development Board] - 5: Run "esp-adf" flash on Ubuntu 20.04
- Are the design formulas for a push-pull transformer correct?
- Do you know the function of OPA?
- [RVB2601 Creative Application Development] Handheld Game Console (3) Flying Bird