1. If Statement
The if statement is a basic conditional selection statement in C51. It usually has three formats:
(1) if (expression) {statement;}
(2) if (expression) {statement 1;} else {statement 2;}
(3) if (expression 1) {statement 1;}
else if (expression 2) (statement 2;)
else if (expression 3) (statement 3;)
…
else if (expression n-1) (statement n-1;)
else {statement n}
【Example】 Usage of if statement.
(1) if (x!=y) printf("x=%d,y=%dn",x,y);
When executing the above statement, if x is not equal to y, the value of x and the value of y are output.
(2) if (x>y) max=x;
else max=y;
When executing the above statement, if x is greater than y, x is sent to the maximum value variable max. If x is not greater than y, y is sent to the maximum value variable max. The max variable gets the larger number of x and y.
(3) if (score>=90) printf(“Your result is an An”);
else if (score>=80) printf("Your result is an Bn");
else if (score>=70) printf("Your result is an Cn");
else if (score>=60) printf("Your result is an Dn");
else printf("Your result is an En");
After executing the above statement, five levels A, B, C, D, and E can be scored according to the score.
2. Switch/case Statement
If statements can be nested to implement a multi-branch structure, but the structure is complex. Switch is a multi-branch selection statement provided in C51 that specifically handles multi-branch structures. Its format is as follows:
switch (expression)
{case constant expression 1: {statement 1;}break;
case constant expression 2: {statement 2;}break;
…
case constant expression n: {statement n;}break;
default: {statement n+1;}
described as follows:
(1) The expression in the parentheses after switch can be an integer or character expression.
(2) When the value of the expression is equal to the value of the constant expression after a "case", the statement after the "case" is executed, and then the switch statement is exited when a break statement is encountered. If the value of the expression is different from the value of the constant expression after all cases, the statement after default is executed, and then the switch structure is exited.
(3) The value of each case constant expression must be different, otherwise there will be a contradiction.
(4) The order in which case statements and default statements appear has no effect on the execution process.
(5) Each case statement can be followed by a "break" or not. If there is a break statement, the switch structure will be exited when it is executed. If there is no break statement, the following statements will be executed in sequence until a break is encountered or the switch structure ends.
(6) Each case statement can be followed by one statement, multiple statements, or no statement. Statements can be enclosed in curly braces or not.
(7) Multiple cases can share a set of execution statements.
【Example】 Usage of switch/case statement.
The student scores are divided into A~D, corresponding to different percentage scores, and the corresponding percentages are required to be printed according to different grades. This can be achieved through the following switch/case statement.
…
switch(grade)
{
case 'A'; printf("90~100n"); break;
case 'B'; printf("80~90n"); break;
case 'C'; printf("70~80n"); break;
case 'D'; printf("60~70n"); break;
case 'E'; printf("<60n"); break;
default; printf("error"n)
}
3. while statement
The while statement is used in C51 to implement the while loop structure. Its format is as follows:
while (expression)
{statement;} /*loop body*/
The expression after the while statement is the condition for looping, and the following statement is the loop body. When the expression is non-zero (true), the statements in the loop body are executed repeatedly; when the expression is zero (false), the while loop is terminated, and the program will execute the next statement outside the loop structure. Its characteristics are: first judge the condition, then execute the loop body. Change the condition in the loop body, and then judge the condition again. If the condition is met, execute the loop body again. If the condition is not met, exit the loop. If the condition is not met for the first time, the loop body will not be executed once.
[Example] The following program uses the while statement to calculate and output the cumulative sum of 1 to 100.
#include #include void main(void) //Main function { int i,s=0; //define integer variables x and y i=1; SCON=0x52; //Serial port initialization TMOD=0x20; TH1=0XF3; TR1=1; while (i<=100) // accumulate the sum of 1 to 100 in s { s=s+i; i++; } printf("1+2+3……+100=%dn",s); while(1); } The result of program execution: 1+2+3…+100=5050 4. do while statement The do while statement is used in C51 to implement the until loop structure. Its format is as follows: do {statement;} /*loop body*/ while(expression); Its characteristics are: the statements in the loop body are executed first, and then the expression is judged. If the expression is true (true), the loop body is executed again, and then judged again, until an expression is not true (false), then the loop is exited and the next statement of the do while structure is executed. When the do while statement is executed, the statements in the loop body will be executed at least once. [Example] Use the do while statement to calculate and output the cumulative sum of 1 to 100. #include #include void main(void) //main function { int i,s=0; //define integer variables x and y i=1; SCON=0x52; //Serial port initialization TMOD=0x20; TH1=0XF3; TR1=1; do //Accumulate the sum of 1 to 100 in s { s=s+i; i++; } while (i<=100); printf("1+2+3……+100=%dn",s); while(1); } 5. for Statement for(expression1; expression2; expression3) {statement;} /*loop body*/ The for statement is followed by three expressions and its execution process is as follows: (1) First solve the value of expression 1. (2) Solve the value of expression 2. If the value of expression 2 is true, execute the statements in the loop and then execute the next step (3). If the value of expression 2 is false, end the for loop and go to the last step. (3) If the value of expression 2 is true, after executing the statements in the loop body, expression 3 is evaluated and then the process goes to step 4. (4) Go to (2) and continue execution. (5) Exit the for loop and execute the following statement. In a for loop, general expression 1 is an initial value expression, which is used to assign an initial value to the loop variable; expression 2 is a conditional expression, which is used to judge the loop variable; expression 3 is a loop variable update expression, which is used to update the value of the loop variable so that the loop variable can exit the loop if the condition is not met. [Example] Use the for statement to calculate and output the cumulative sum of 1 to 100. #include #include void main(void) //main function { int i,s=0; //define integer variables x and y SCON=0x52; //Serial port initialization TMOD=0x20; TH1=0XF3; TR1=1; for (i=1;i<=100;i++) s=s+i; // add the sum of 1 to 100 in s printf("1+2+3……+100=%dn",s); while(1); } 6. Nesting of loops A loop body is allowed to contain a complete loop structure, which is called loop nesting. The outer loop is called the outer loop, and the inner loop is called the inner loop. If the inner loop body contains a loop structure, it constitutes a multiple loop. In C51, three loop structures are allowed to be nested. [Example] Use nested structures to construct a delay program. void delay(unsigned int x) { unsigned char j; while(x--) {for (j=0;j<125;j++);} } Here, an inner loop is used to construct a benchmark delay, and the number of outer loops is set through parameters when calling, so that various delay relationships can be formed. 7. break and continue statements The break and continue statements are usually used in loop structures to jump out of the loop structure. However, there are some differences between the two statements, which are introduced below. 1) Break statement As mentioned above, the break statement can be used to jump out of the switch structure, allowing the program to continue executing the next statement after the switch structure. The break statement can also be used to jump out of the loop body, ending the loop early and then executing the statement following the loop structure. It cannot be used in any other statement except loop statements and switch statements. [Example 19] The following program is used to calculate the area of a circle. When the calculated area is greater than 100, the break statement jumps out of the loop. for (r=1; r<=10; r++) { area=pi*r*r; if (area>100) break; printf("%fn",area); } 2) Continue statement The continue statement is used in a loop structure to end the current loop, skip the unexecuted statements following continue in the loop body, and directly determine whether to execute the loop next time. The difference between the continue statement and the break statement is that the continue statement only ends the current loop instead of terminating the entire loop; the break statement ends the loop without further conditional judgment. 【Example 20】 Output the numbers between 100 and 200 that are not divisible by 3. for (i=100; i<=200; i++) { if (i%3= =0) continue; printf("%d ";i); } In the program, when i is divisible by 3, the continue statement is executed, the current loop ends, and the printf() function is skipped. The printf() function is only executed when i is divisible by 3. 8. return statement The return statement is usually placed at the end of a function to terminate the execution of the function and control the program to return to the location where the function was called. When returning, the return statement can also be used to bring back the return value. There are two formats of the return statement:
Previous article:Functions of single-chip microcomputer C language C51
Next article:Input and output of single chip microcomputer C language C51
Recommended ReadingLatest update time:2024-11-16 13:35
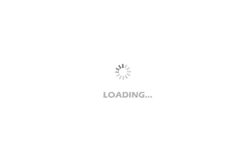
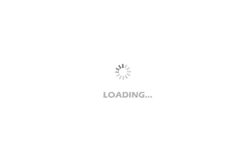
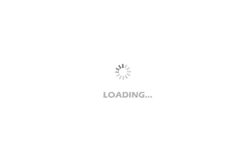
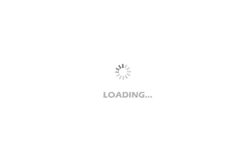
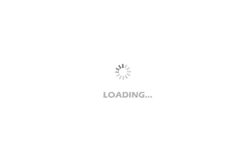
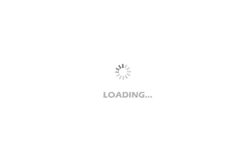
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Registration is open | TI live award-winning broadcast: Current and voltage measurement solutions in servo drives
- 【Development and application based on NUCLEO-F746ZG motor】9. Parameter configuration - system clock
- I make a bubble on time
- Playing with Zynq Serial 44——[ex63] Image smoothing processing of MT9V034 camera
- [2022 Digi-Key Innovation Design Competition] Material Unboxing STM32H745I-DISCO
- Supplementary chapter of motor PID control - Introduction to the serial port protocol of the Wildfire host computer
- 485 interface EMC circuit design scheme!
- Help! CCS7.3 enters the exit.c file after entering debug mode. I don't know how to solve it
- Share Gigabit Ethernet port circuit design
- [RVB2601 creative application development] 2. CH2601 serial port receiving