Function
1. Function definition
The general format of a function definition is as follows:
Function type Function name (formal parameter list) [reentrant][interrupt m][using n]
Formal parameter description
{
Local variable definition
Function body
}
The first part is called the header of the function, and the second part is called the tail of the function. The format is as follows:
1) Function type
The function type describes the type of value returned by the function.
2) Function name
The function name is the name that the user gives to the custom function for use when calling the function.
3). Formal parameter table
The formal parameter table is used to list the formal parameters for data transfer between the calling function and the called function.
[Example] Define a function max() that returns the maximum value of two integers.
int max(int x,int y)
{ int z;
z=x>y?x:y;
return (z);
}
It can also be used like this:
int max(x,y)
int x,y;
{ int z;
z=x>y?x:y;
return (z);
}
4) The reentrant modifier
This modifier is used to define a function as a reentrant function. A reentrant function is a function that allows recursive calls. A recursive call of a function means that when a function is being called and has not yet returned, it directly or indirectly calls itself. Ordinary functions cannot do this, only reentrant functions allow recursive calls.
Regarding reentrant functions, note the following:
(1) When a reentrant function modified with reentrant is called, bit type parameters are not allowed in the actual parameter list. Any operations on bit variables are not allowed in the function body, and bit type values cannot be returned.
(2) During compilation, the system creates a simulated stack area in the internal or external memory for the reentrant function, called the reentrant stack. The local variables and parameters of the reentrant function are placed in the reentrant stack, so that the reentrant function can be called recursively.
(3) In terms of parameter passing, actual parameters can be passed to the indirectly called reentrant function. Indirectly called functions without reentrant attributes cannot contain call parameters, but defined global variables can be used for parameter passing.
5). interrupt m modifier
Interrupt m is a very important modifier in C51 functions, because interrupt functions must be modified by it. In C51 programming, when the interrupt m modifier is used in function definition, the system converts the corresponding function into an interrupt function during compilation, automatically adds the program header and tail segments, and automatically arranges it in the corresponding position in the program memory according to the 51 system interrupt processing method.
In this modifier, the value of m is 0~31, and the corresponding interrupt conditions are as follows:
0——External interrupt 0
1——Timer/Counter T0
2——External interrupt 1
3——Timer/Counter T1
4——Serial port interrupt
5——Timer/Counter T2
Other values are reserved.
When writing 51 interrupt function, please note the following:
(1) Interrupt functions cannot pass parameters. If an interrupt function contains any parameter declaration, a compilation error will occur.
(2) The interrupt function has no return value. If you attempt to define a return value, you will not get the correct result. It is recommended to define the interrupt function as a void type to clearly indicate that there is no return value.
(3) In any case, the interrupt function cannot be called directly, otherwise a compilation error will occur. This is because the return of the interrupt function is completed by the RETI instruction of the 8051 microcontroller, and the RETI instruction affects the hardware interrupt system of the 8051 microcontroller. If the interrupt function is called directly without an actual interrupt, the operation result of the RETI instruction will produce a fatal error.
(4) If other functions are called in an interrupt function, the registers used by the called function must be the same as those used by the interrupt function. Otherwise, incorrect results will be generated.
(5) When the C51 compiler compiles an interrupt function, it will automatically add the corresponding content at the beginning and end of the program. Specifically, ACC, B, DPH, DPL, and PSW are pushed onto the stack at the beginning of the program and popped off at the end. If the interrupt function does not have the using n modifier, R0~R1 will also be pushed onto the stack at the beginning and popped off at the end. If the interrupt function has the using n modifier, the working register group select bit in PSW will also be modified after PSW is pushed onto the stack at the beginning.
(6) The C51 compiler generates an interrupt vector from the absolute address 8m+3, where m is the interrupt number, which is the number after interrupt. The vector contains an absolute jump to the entry address of the interrupt function.
(7) The interrupt function is best written at the end of the file, and the use of extern storage type declaration is prohibited to prevent other programs from calling it.
[Example] Write an interrupt service program to count the number of interrupts of external interrupt 0
extern int x;
void int0() interrupt 0 using 1
{
x++;
}
6) Using n modifier
The modifier using n is used to specify the working register group used inside this function, where n is a value from 0 to 3, indicating the register group number.
When using the using n modifier, note the following points:
(1) After adding using n, C51 automatically adds the following instructions at the beginning and end of the function during compilation.
{
PUSH PSW ; push the flag register onto the stack
MOV PSW, #Constant associated with register group number
…
POP PSW ; Pop the flag register from the stack
}
(2) The using n modifier cannot be used for functions that return values, because the return value of a C51 function is stored in a register. If the register set is changed, the return value will be incorrect.
2. Function calling and declaration
1. Function call
The general form of a function call is as follows:
function name(argument list);
For a function call with parameters, if the argument list contains multiple arguments, each argument is separated by a comma.
According to the position of the function call in the calling function, there are three types of function calls:
(1) Function statement: A statement that treats the called function as the main calling function.
(2) Function expression. A function is placed in an expression and appears as an operand. In this case, the called function is required to have a return statement to return a clear value to participate in the expression operation.
(3) Function parameters. The called function is used as a parameter of another function.
2. Declaration of custom functions
In C51, the general form of a function prototype is as follows:
[extern] function type function name (formal parameter list);
The function declaration tells the compiler the function name, function type, and the type, number, and order of its parameters so that the system can check them when the function is called. A semicolon is added after the function declaration.
If the declared function is inside a file, then extern is not used in the declaration. If the declared function is not inside a file but in another file, then extern must be used in the declaration to indicate that the function to be used is in another file.
【Example】Use of function
#include #include int max(int x,int y); //declare the max function void main(void) //main function { int a,b; SCON=0x52; //Serial port initialization TMOD=0x20; TH1=0XF3; TR1=1; scanf("please input a,b:%d,%d",&a,&b); printf("n"); printf("max is:%dn",max(a,b)); while(1); } int max(int x,int y) { int z; z=(x>=y?x:y); return(z); } [Example 24] Use of external functions Program serial_initial.c #include #include void serial_initial(void) //main function { SCON=0x52; //Serial port initialization TMOD=0x20; TH1=0XF3; TR1=1; } Program y1.c #include #include extern serial_initial(); void main(void) { int a,b; serial_initial(); scanf("please input a,b:%d,%d",&a,&b); printf("n"); printf("max is:%dn",a>=b?a:b); while(1); } 3. Nested and recursive functions 1. Nesting of functions In the process of calling a function, another function is called. The C51 compiler usually relies on the stack to pass parameters. The stack is set in the on-chip RAM, and the space of the on-chip RAM is limited, so the nesting depth is relatively limited, generally within a few layers. If there are too many layers, it will lead to insufficient stack space and errors. 【Example】 Nested function calls #include #include extern serial_initial(); int max(int a,int b) { int z; z=a>=b?a:b; return(z); } int add(int c,int d,int e,int f) { int result; result=max(c,d)+max(e,f); //Call function max return(result); } main() { int final; serial_initial(); final=add(7,5,2,8); printf("%d",final); while(1); } 2. Recursion of functions Recursive calling is a special case of nested calling. If a function is called directly or indirectly, it is called a recursive calling of the function. In the recursive calling of a function, it is necessary to avoid endless self-calling. The recursive calling should be ended through conditional control to limit the number of recursions. The following is an example of using recursive calls to find n!. 【Example】Recursively find the factorial of a number n!. In mathematical calculations, the factorial of a number n is equal to the number itself multiplied by the factorial of the number n-1, that is, n!=n´(n-1)!. Using the factorial of n-1 to represent the factorial of n is a recursive representation method. In programming, this is achieved through recursive function calls.
Previous article:Single chip microcomputer C language C51 structure data type
Next article:C language C51 statements for single chip microcomputers
Recommended ReadingLatest update time:2024-11-16 11:40
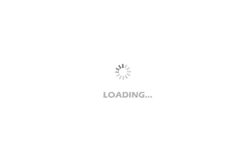
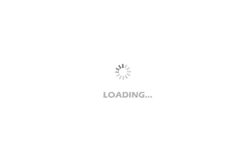
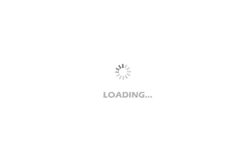
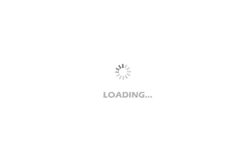
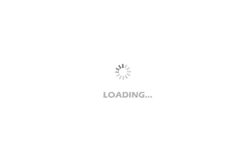
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Compile BLE_Chat under BLE_Examples with software, and fail to search for Bluetooth signal after running
- [RT-Thread reading notes] Solved the confusion of interruption
- How does LoRa achieve positioning?
- MPLAB cannot complete compilation
- ISE Tutorial
- Common Misunderstandings in PCB Differential Signal Design
- 【XMC4800 Relax EtherCAT Kit Review】+LWIP Application
- [NXP Rapid IoT Review] + Mobile Synchronizer 3
- Design of USB interface based on DSP
- How to test the third-order intermodulation intercept point (OPI3) of RF signal source and arbitrary waveform generator?