{
//Hope for input
BCLR(bit, GPIO_PDDR_REG(gpio_ptr));
}
}
//===========================================================================
//Function name: gpio_set
//Function returns: None
//Parameter description: port_pin: port number | pin number (e.g.: PORTB|(5) means pin 5 of port B)
// state: initial state of the pin (0 = low level, 1 = high level)
//Function summary: Set the pin state to low or high
//===========================================================================
void gpio_set(uint_16 port_pin, uint_8 state)
{
//Local variable declaration
GPIO_MemMapPtr gpio_ptr; //Declare port_ptr as a GPIO structure type pointer
gpio_ptr_bit(port_pin,&gpio_ptr,&bit); //Calculate the base address and the offset of the pin in the register
//Determine whether the pin is output 1 or 0 based on the parameter state
if (1==state)
{
BSET(bit,gpio_ptr->PDOR); //The corresponding position is 1
} else {
BCLR(bit,gpio_ptr->PDOR);//The corresponding position is 0
}
}
//===========================================================================
//Function name: gpio_get
//Function returns: the state of the specified pin (1 or 0)
//Parameter description: port_pin: port number | pin number (e.g.: PORTB|(5) means pin 5 of port B)
//Function summary: Get the status of the specified pin (1 or 0)
//===========================================================================
uint_8 gpio_get(uint_16 port_pin)
{
//Local variable declaration
GPIO_MemMapPtr gpio_ptr; //Declare port_ptr as a GPIO structure type pointer
gpio_ptr_bit(port_pin,&gpio_ptr,&bit); //Calculate the base address and the offset of the pin in the register
// Return the status of the pin
return ((BGET(bit,gpio_ptr->PDIR))>=1 ? 1:0);
}
//===========================================================================
//Function name: gpio_reverse
//Function returns: None
//Parameter description: port_pin: port number | pin number (e.g.: PORTB|(5) means pin 5 of port B)
//Function summary: Invert the output state of the specified pin.
//===========================================================================
void gpio_reverse(uint_16 port_pin)
{
//Local variable declaration
GPIO_MemMapPtr gpio_ptr; //Declare port_ptr as a GPIO structure type pointer
gpio_ptr_bit(port_pin,&gpio_ptr,&bit); //Calculate the base address and the offset of the pin in the register
//Reverse the output state of the specified pin
BSET(bit,gpio_ptr->PTOR);
}
//===========================================================================
//Function name: gpio_pull
//Function returns: None
//Parameter description: port_pin: port number | pin number (e.g.: PORTB|(5) means pin 5 of port B)
// pullselect: pin pull-up enable selection (0 = pull-up disabled, 1 = pull-up enabled)
//Function summary: Pull the specified pin high
//===========================================================================
void gpio_pull(uint_16 port_pin, uint_8 pullselect)
{
//Local variable declaration
uint_8 port; //port number
uint_8 pin; //pin number
gpio_port_pin_num(port_pin, &port, &pin); //parse out the port number and pin number
//Calculate the offset of the pin in the register
if (port < 4) //Port number is PORTA~PORTD
{
//The number of pins
bit = 8 * port + pin;
if (1==pullselect){BSET(bit,PORT_PUE0);}//Port pull-up enable low register 0 pull-up enable
else {BCLR(bit,PORT_PUE0);}//Pull-up disable (disabled)
}
else if(3 //The number of pins bit = 8 * (port - 4) + pin; if (1==pullselect){BSET(bit,PORT_PUE1);}//Port pull-up enable low register 1 pull-up enable else {BCLR(bit,PORT_PUE1);} } else //Port number is PORTI { //The number of pins bit = 8 * (port - 8) + pin; if (1==pullselect){BSET(bit,PORT_PUE2);}//Port pull-up enable low register 2 pull-up enable else {BCLR(bit,PORT_PUE2);} } } //----------------------The following is where the internal functions are stored---------------------------------------- //=========================================================================== //Function name: gpio_port_pin_num //Function returns: None //Parameter description: port_pin: port number | pin number (e.g.: PORTB|(5) means pin 5 of port B) // port: the parsed port number // pin: parsed pin number (0~8, the actual value is determined by the physical pin of the chip) //Function summary: Parse the parameter port_pin (e.g. PORTB|(5)) to get the specific port number and pin number, and pass them out through port and pin. // For example, PORTB|(5) is parsed as *port=PORTB, *pin=5). //Note: port and pin are address parameters. The purpose is to bring back the result. No value is required before calling the function. //=========================================================================== static void gpio_port_pin_num(uint_16 port_pin,uint_8* port,uint_8* pin) { *port = port_pin>>8; *pin = port_pin; } //=========================================================================== //Function name: gpio_ptr_bit //Function returns: None //Parameter description: port_pin: port number | pin number (e.g.: PORTB|(5) means pin 5 of port B) // gpio_ptr: parsed base address // bit: the offset of the pin in the register //Function summary: Parse the passed parameter port_pin (e.g. PORTB|(5)), parse the base address and the offset of the pin in the register, and pass it out through gpio_ptr and bit. // For example: PORTB|(5) is parsed as base address GPIOA_BASE_PTR, offset 13 //Note: port and pin are address parameters. The purpose is to bring back the result. No value is required before calling the function. //=========================================================================== // Parse the base address and the pin's offset in the register static void gpio_ptr_bit(uint_16 port_pin,GPIO_MemMapPtr* gpio_ptr,uint_32* bit) { uint_8 port; //port number uint_8 pin; //pin number gpio_port_pin_num(port_pin, &port, &pin); //parse out the port number and pin number //Calculate the offset of the pin in the register if (port < 4) //Port number is PORTA~PORTD { //The register base address where the port is located *gpio_ptr = GPIOA_BASE_PTR; //The number of pins *bit = 8 * port + pin; } else if(3 //The register base address where the port is located *gpio_ptr = GPIOB_BASE_PTR; //The number of pins *bit = 8 * (port - 4) + pin; } else //Port number is PORTI { //The register base address where the port is located *gpio_ptr = GPIOC_BASE_PTR; //The number of pins *bit = 8 * (port - 8) + pin; } } //----------------------------End of internal function---------------------------------------
Previous article:Freescale S12 series (based on MC9S12XET256MAA and/MC9S12XEP100) CAN data
Next article:NXP Freescale Kinetis KEA128 Study Notes 3--GPIO Module (Part 2)
Recommended ReadingLatest update time:2024-11-17 02:34
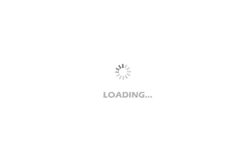
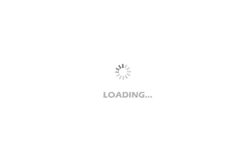
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- Rambus Launches Industry's First HBM 4 Controller IP: What Are the Technical Details Behind It?
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- EEWORLD University ---- Children's Toy "Black Box" -- BabyCareAssistant Demonstration Video
- [National Technology Low Power Series N32L43x Evaluation] 4. SPI-FLASH driver + fatfs file system transplantation + USB driver module...
- Anlu SparkRoad Development Board Review (7) MCU Soft Core IP Trial
- A DSP debugging and direct code generation method based on Matlab
- [Technical Tips] Power Supply PCB Design Skills and Case Analysis
- FPGA_100 Days Journey_VGA Design.pdf
- MakeCode graphical programming supports USB HID function
- EEWORLD University-How to improve the performance and reliability of motor drive and inverter applications? Introducing two simple and efficient methods
- The latest weekly evaluation intelligence, EE Evaluation Intelligence Bureau reports for you
- Pure hardware AC constant resistance load and INA related issues