1Use the C library function printf.
step:
1) First you need to include the header file stdio.h.
2) Then define the file handle, which is actually an int variable encapsulated in a structure.
struct__FILE{inthandle;};
3) Define FILE__stdout; FILE is __FILE, which is defined by the stdio.h macro.
4) Implement the function
intfputc(intch,FILE*f){chartempch=ch;sendchar(tempch);returnch;}
5) Implement the function
intsendchar(intch){
if(ch=='n'){
while(!(console_tty_f->lsr&UART_LSR_THRE));
console_tty_f->dll_fifo=0x0d;
}
while(!(console_tty_f->lsr&UART_LSR_THRE));
return(console_tty_f->dll_fifo=ch);
}
As can be seen from the above code, printf is a blocking function. Waiting for the message to be sent may affect other processes. If you write a non-waiting print function, you can use the second method.
2 Use the C library function vsprintf and variable parameter function.
step:
1) Include the header files stdio.h and stdarg.h.
2) Write a variable parameter function.
voidprint(constchar*lpszFormat,...){charszBuffer[PRINT_BUF]={0};
va_listargs; enter; va_start(args, lpszFormat);
ret=vsprintf(szBuffer,lpszFormat,args);
va_end(args);
uart[console_uart].put_2_ring(console_uart,szBuffer,ret);
}
It can be seen that the library function vsprintf is used to format the input string and then send it when idle.
3. Complete parameter extraction and formatting by yourself.
step:
1) Define variable parameter list typedef char*va_list;
2) Define address alignment macro
#define_AUPBND(sizeof(int)-1)
#define_ADNBND(sizeof(int)-1)
#define_bnd(X,bnd)(((sizeof(X))+(bnd))&(~(bnd)))
3) Define variable length parameter extraction macro
#defineva_start(ap,A)(void)((ap)=(((char*)&(A))+(_bnd(A,_AUPBND))))
#defineva_arg(ap,T)(*(T*)(((ap)+=(_bnd(T,_AUPBND)))-(_bnd(T,_ADNBND)))
#defineva_end(ap)(void)0
4) Write a variable parameter function, as in step 2 of method 2.
5) Implement the vsprintf function. There are many implementation source codes, such as Linux, etc. But there is no support for floating points.
Previous article:Design of ARM matrix keyboard and its linux driver implementation
Next article:Video Acquisition and Processing System Based on FPGA and ARM
Recommended ReadingLatest update time:2024-11-16 10:35
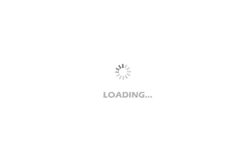
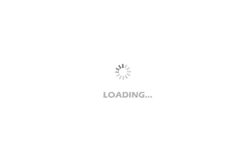
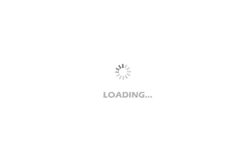
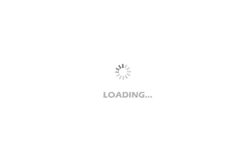
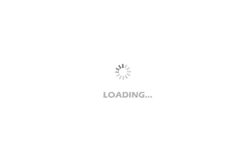
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- TI C6000 project creation steps
- Please teach me a peak detection circuit composed of an op amp
- EEWORLD University ---- Control system of 100 quantum bits
- What is the function of this solder joint on the PCB?
- Can I change my nickname in the forum?
- GD32L233C-START Review——03_2.CoreMark Test, RT-Thread-Nano Porting
- A detailed guide to the Industrial Internet of Things: From risks to benefits
- How to implement remote monitoring of wind turbines in wind farms?
- The difference between USART, USI, USCI and eUSCI serial communication modules in MSP430 microcontroller (updated on March 4, 2019)
- Answer questions to win prizes | ADI Technology Express - Industrial Automation Control and Edge Computing