Introduction: I recently started using the stm8s103k microcontroller, and today I will record the use of its timer 2. First of all, it needs to be explained that the stm8s manual says that TIM2 16-bit counts up, which is wrong.
The stm8s timing is easy to use. First, set the clock frequency of the timer. Once the clock period T of the timer is known, the timing time Tn is determined, that is, Tn = T * ARR (automatically load data).
The procedure is as follows:
1. MCU clock setting:
//fmaster=fcpu=2MHz
CLK_ECKR=0x00;
CLK_ICKR=0x01;
CLK_CMSR=0xe1;
CLK_SWR=0xe1;
CLK_CKDIVR=0x18;
2. Timer TIM2 initialization
//Timer 2 initialization fmaster/frequency division = 2M/2 = 1M, count once in 1us, interrupt once in 50us
void TIM2_Init(void)
{
_asm("sim"); //sim is to disable interrupts
TIM2_IER = 0x00; //Disable interrupt
TIM2_EGR = 0x01; //Allow update flag to be generated
TIM2_PSCR =0x01; //Set clock division 2M/2=1MHz---1us
TIM2_ARRH = 0x00; //0x32=50; Cycle = 50 times, reset timer 2 every 50us
TIM2_ARRL = 0x32; //ARR automatically loads value, decrements by 1 every 1us
TIM2_CNTRH=0x00; //initial value
TIM2_CNTRL=0x00;
TIM2_CR1 |= 0x81; //Start the timer
TIM2_IER |= 0x01; //Enable interrupt
_asm("rim"); //rim enables interrupt
}
3. Interrupt execution of program
@far @interrupt void TIM2_UPD_IRQHandler(void)
{
TIM2_SR1 &=~(0x01); //=0x0e; // Clear interrupt flag
PC_ODR=~PC_ODR; //You need to configure pc and pb as output ports first
PB_ODR=~PB_ODR;
}
4. Modify the interrupt vector table
Open the stm8_interrupt_vector.c file and add the following content:
extern @far @interrupt void TIM2_UPD_IRQHandler(void);
Modify the following line:
{0x82, NonHandledInterrupt},
for:
{0x82,(interrupt_handler_t)TIM2_UPD_IRQHandler},
That's it, you can try it.
During my operation, I encountered a very annoying problem, that is, the downloader often does not work, error number 30006, 30003.
In both cases, first confirm whether your stlink wiring is correct, and then measure the voltage of the 4 download pins. The correct voltage is: 5V, Gnd, NRST-5V, SWIM-0v (roughly like this). If there is no problem above, it may be that the downloader and the computer are not connected properly. You need to reconnect and confirm the software (Target-setting).
Previous article:STM8 learning nRF24L01
Next article:The TIMER2 timer cannot enter the interruption problem
Recommended ReadingLatest update time:2024-11-16 12:46
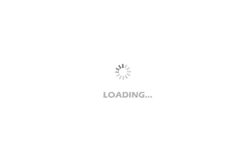
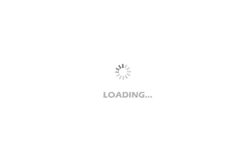
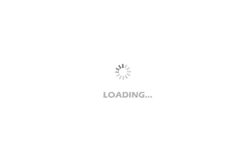
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Creating an embedded project using Rust
- I bought another development board with the highest integration, no doubt about it.
- Design Method of Low Noise Preamplifier Circuit
- [Elective course to get a gift] Keysight's new "Test and Measurement" series of courses, reservations open in the hot summer! Master-level lecturers live online~
- AD chip output digital signal problem
- Step-by-step tutorial | ESP32-Audio-Kit (8388 version) development environment setup video tutorial
- MSP430F5438A instruction cycle and clock cycle
- AVR assembler, paid help
- Allegro software design has been updated with the latest patch, but why can't I do 3D simulation?
- EEWORLD University ---- Embedded Linux Development Introduction Video